Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripRenderEventArgs.cs / 1 / ToolStripRenderEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Drawing; ////// /// ToolStripRenderEventArgs /// public class ToolStripRenderEventArgs : EventArgs { private ToolStrip toolStrip = null; private Graphics graphics = null; private Rectangle affectedBounds = Rectangle.Empty; private Color backColor = Color.Empty; ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip) { this.toolStrip = toolStrip; this.graphics = g; this.affectedBounds = new Rectangle(Point.Empty, toolStrip.Size); } ////// /// This class represents all the information to render the toolStrip /// public ToolStripRenderEventArgs(Graphics g, ToolStrip toolStrip, Rectangle affectedBounds, Color backColor) { this.toolStrip = toolStrip; this.affectedBounds = affectedBounds; this.graphics = g; this.backColor = backColor; } ////// /// the bounds to draw in /// public Rectangle AffectedBounds { get { return affectedBounds; } } ////// /// the back color to draw with. /// public Color BackColor { get { if (backColor == Color.Empty) { // get the user specified color backColor = toolStrip.RawBackColor; if (backColor == Color.Empty) { if (toolStrip is ToolStripDropDown) { backColor = SystemColors.Menu; } else if (toolStrip is MenuStrip) { backColor = SystemColors.MenuBar; } else { backColor = SystemColors.Control; } } } return backColor; } } ////// /// the graphics object to draw with /// public Graphics Graphics { get { return graphics; } } ////// /// Represents which toolStrip was affected by the click /// public ToolStrip ToolStrip { get { return toolStrip; } } ///public Rectangle ConnectedArea { get { ToolStripDropDown dropDown = toolStrip as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem ownerItem = dropDown.OwnerItem as ToolStripDropDownItem; if (ownerItem is MdiControlStrip.SystemMenuItem) { // there's no connected rect between a system menu item and a dropdown. return Rectangle.Empty; } if (ownerItem !=null && ownerItem.ParentInternal != null && !ownerItem.IsOnDropDown) { // translate the item into our coordinate system. Rectangle itemBounds = new Rectangle(toolStrip.PointToClient(ownerItem.TranslatePoint(Point.Empty, ToolStripPointType.ToolStripItemCoords, ToolStripPointType.ScreenCoords)), ownerItem.Size); Rectangle bounds = ToolStrip.Bounds; Rectangle overlap = ToolStrip.ClientRectangle; overlap.Inflate(1,1); if (overlap.IntersectsWith(itemBounds)) { switch (ownerItem.DropDownDirection) { case ToolStripDropDownDirection.AboveLeft: case ToolStripDropDownDirection.AboveRight: // Consider... the shadow effect interferes with the connected area. return Rectangle.Empty; // return new Rectangle(itemBounds.X+1, bounds.Height -2, itemBounds.Width -2, 2); case ToolStripDropDownDirection.BelowRight: case ToolStripDropDownDirection.BelowLeft: overlap.Intersect(itemBounds); if (overlap.Height == 2) { return new Rectangle(itemBounds.X+1, 0, itemBounds.Width -2, 2); } // if its overlapping more than one pixel, this means we've pushed it to obscure // the menu item. in this case pretend it's not connected. return Rectangle.Empty; case ToolStripDropDownDirection.Right: case ToolStripDropDownDirection.Left: return Rectangle.Empty; // Consider... the shadow effect interferes with the connected area. // return new Rectangle(bounds.Width-2, 1, 2, itemBounds.Height-2); } } } } return Rectangle.Empty; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
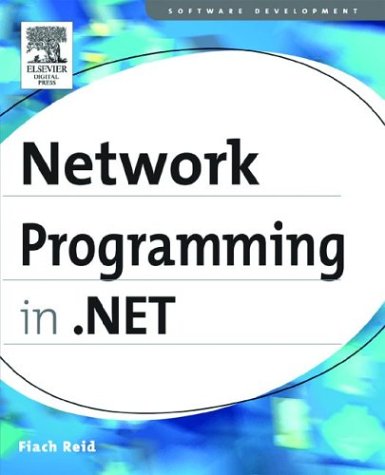
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UidPropertyAttribute.cs
- PropertyMetadata.cs
- XmlWriterTraceListener.cs
- EpmCustomContentDeSerializer.cs
- Opcode.cs
- ConfigXmlDocument.cs
- DesignerCategoryAttribute.cs
- EventSourceCreationData.cs
- HttpApplicationStateWrapper.cs
- Matrix.cs
- NetworkStream.cs
- Attachment.cs
- PropertyMap.cs
- WebBrowserSiteBase.cs
- SwitchAttribute.cs
- WebScriptMetadataInstanceContextProvider.cs
- AxisAngleRotation3D.cs
- ToolBarButton.cs
- SecurityDescriptor.cs
- WasNotInstalledException.cs
- ZipIOFileItemStream.cs
- ServiceBuildProvider.cs
- HttpPostedFile.cs
- UserPreferenceChangingEventArgs.cs
- nulltextcontainer.cs
- TextFormatter.cs
- VariableBinder.cs
- XmlSerializerVersionAttribute.cs
- CatalogPartCollection.cs
- WizardForm.cs
- Transform3DCollection.cs
- HtmlTitle.cs
- RuleSettings.cs
- EntityContainer.cs
- SchemaMapping.cs
- CacheSection.cs
- ArrayConverter.cs
- CheckBoxPopupAdapter.cs
- OleDbCommand.cs
- FloatAverageAggregationOperator.cs
- RichTextBox.cs
- KeyToListMap.cs
- ScriptReferenceEventArgs.cs
- WindowsRegion.cs
- SqlUnionizer.cs
- PropertyGridView.cs
- XmlNamespaceMappingCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- DocumentReference.cs
- ToolStripDropTargetManager.cs
- SecurityKeyEntropyMode.cs
- ProtocolsSection.cs
- MsmqIntegrationBindingCollectionElement.cs
- HitTestWithPointDrawingContextWalker.cs
- DataServiceKeyAttribute.cs
- MessageQueuePermissionAttribute.cs
- Size.cs
- CodeDirectionExpression.cs
- LineSegment.cs
- InvokePattern.cs
- TablePatternIdentifiers.cs
- TerminatorSinks.cs
- DockAndAnchorLayout.cs
- StreamGeometry.cs
- WorkflowIdleElement.cs
- DictionaryContent.cs
- ScrollChangedEventArgs.cs
- SqlCommandBuilder.cs
- StaticExtensionConverter.cs
- ViewLoader.cs
- _SSPIWrapper.cs
- CustomAttributeBuilder.cs
- ToolZone.cs
- ItemsControlAutomationPeer.cs
- LineMetrics.cs
- SizeAnimationUsingKeyFrames.cs
- WebPartVerbsEventArgs.cs
- XmlCharacterData.cs
- ResourceProviderFactory.cs
- IgnoreSectionHandler.cs
- ToolStripArrowRenderEventArgs.cs
- DataGridViewIntLinkedList.cs
- ProviderUtil.cs
- VisualTreeUtils.cs
- Queue.cs
- LinkTarget.cs
- DataGridViewRowEventArgs.cs
- EnumBuilder.cs
- PointCollectionConverter.cs
- PeerHelpers.cs
- LinkedResourceCollection.cs
- DataPagerField.cs
- HMACSHA256.cs
- QilReference.cs
- MenuItemBinding.cs
- XsltLibrary.cs
- Drawing.cs
- GlyphsSerializer.cs
- Part.cs
- Paragraph.cs