Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Misc / SecurityUtils.cs / 1 / SecurityUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ #if WINFORMS_NAMESPACE namespace System.Windows.Forms #elif DRAWING_NAMESPACE namespace System.Drawing #elif WINFORMS_PUBLIC_GRAPHICS_LIBRARY namespace System.Internal #elif SYSTEM_NAMESPACE namespace System #else namespace System.Windows.Forms #endif { using System; using System.Reflection; using System.Diagnostics.CodeAnalysis; using System.Security; using System.Security.Permissions; ////// Useful methods to securely call 'dangerous' managed APIs (especially reflection). /// See http://wiki/default.aspx/Microsoft.Projects.DotNetClient.SecurityConcernsAroundReflection /// for more information specifically about why we need to be careful about reflection invocations. /// internal static class SecurityUtils { private static bool HasReflectionPermission { get { try { (new ReflectionPermission(PermissionState.Unrestricted)).Demand(); return true; } catch (SecurityException) { } return false; } } ////// This helper method provides safe access to Activator.CreateInstance. /// NOTE: This overload will work only with public .ctors. /// internal static object SecureCreateInstance(Type type) { return SecureCreateInstance(type, null); } ////// This helper method provides safe access to Activator.CreateInstance. /// NOTE: This overload will work only with public .ctors. /// internal static object SecureCreateInstance(Type type, object[] args) { if (type == null) { throw new ArgumentNullException("type"); } // The only case we need to worry about is when the type is in the same assembly // as us. In all other cases, reflection will take care of security. if (type.Assembly == typeof(SecurityUtils).Assembly && !(type.IsPublic || type.IsNestedPublic)) { (new ReflectionPermission(PermissionState.Unrestricted)).Demand(); } return Activator.CreateInstance(type, args); } ////// This helper method provides safe access to Activator.CreateInstance. /// Set allowNonPublic to true if you want non public ctors to be used. /// internal static object SecureCreateInstance(Type type, object[] args, bool allowNonPublic) { if (type == null) { throw new ArgumentNullException("type"); } BindingFlags flags = BindingFlags.Instance | BindingFlags.Public | BindingFlags.CreateInstance; // The only case we need to worry about is when the type is in the same assembly // as us. In all other cases, reflection will take care of security. if (type.Assembly == typeof(SecurityUtils).Assembly) { // if it's an internal type, we demand reflection permission. if (!(type.IsPublic || type.IsNestedPublic)) { (new ReflectionPermission(PermissionState.Unrestricted)).Demand(); } else if (allowNonPublic && !HasReflectionPermission) { // Someone is trying to instantiate a public type in *our* assembly, but does not // have full reflection permission. We shouldn't pass BindingFlags.NonPublic in this case. // The reason we don't directly demand the permission here is because we don't know whether // a public nr non-public .ctor will be invoked. We want to allow the public .ctor case to // succeed. allowNonPublic = false; } } if (allowNonPublic) { flags |= BindingFlags.NonPublic; } return Activator.CreateInstance(type, flags, null, args, null); } ////// Helper method to safely invoke a .ctor. You should prefer SecureCreateInstance to this. /// Set allowNonPublic to true if you want non public ctors to be used. /// internal static object SecureConstructorInvoke(Type type, Type[] argTypes, object[] args, bool allowNonPublic) { return SecureConstructorInvoke(type, argTypes, args, allowNonPublic, BindingFlags.Default); } ////// Helper method to safely invoke a .ctor. You should prefer SecureCreateInstance to this. /// Set allowNonPublic to true if you want non public ctors to be used. /// The 'extraFlags' parameter is used to pass in any other flags you need, /// besides Public, NonPublic and Instance. /// internal static object SecureConstructorInvoke(Type type, Type[] argTypes, object[] args, bool allowNonPublic, BindingFlags extraFlags) { if (type == null) { throw new ArgumentNullException("type"); } BindingFlags flags = BindingFlags.Instance | BindingFlags.Public | extraFlags; // The only case we need to worry about is when the type is in the same assembly // as us. In all other cases, reflection will take care of security. if (type.Assembly == typeof(SecurityUtils).Assembly) { // if it's an internal type, we demand reflection permission. if (!(type.IsPublic || type.IsNestedPublic)) { (new ReflectionPermission(PermissionState.Unrestricted)).Demand(); } else if (allowNonPublic && !HasReflectionPermission) { // Someone is trying to invoke a ctor on a public type in *our* assembly, but does not // have full reflection permission. We shouldn't pass BindingFlags.NonPublic in this case. allowNonPublic = false; } } if (allowNonPublic) { flags |= BindingFlags.NonPublic; } ConstructorInfo ctor = type.GetConstructor(flags, null, argTypes, null); if (ctor != null) { return ctor.Invoke(args); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
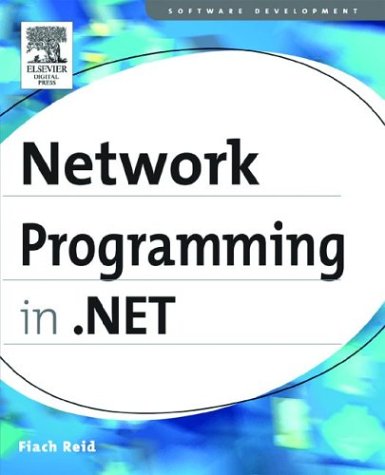
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HwndSourceParameters.cs
- PerfService.cs
- VerificationAttribute.cs
- HttpConfigurationContext.cs
- GraphicsPath.cs
- SmtpCommands.cs
- entityreference_tresulttype.cs
- DbConnectionPoolCounters.cs
- MarkupWriter.cs
- TextRangeBase.cs
- DbProviderSpecificTypePropertyAttribute.cs
- SchemaImporter.cs
- LinqDataSourceView.cs
- FlowchartDesigner.xaml.cs
- Thickness.cs
- EntitySqlQueryBuilder.cs
- TranslateTransform3D.cs
- BitSet.cs
- FontStyle.cs
- ArcSegment.cs
- ScrollEventArgs.cs
- PointAnimation.cs
- ChameleonKey.cs
- IndentedTextWriter.cs
- SqlRowUpdatedEvent.cs
- DefaultBinder.cs
- DateBoldEvent.cs
- UnsafePeerToPeerMethods.cs
- ScalarConstant.cs
- CodeAttributeArgumentCollection.cs
- CanExecuteRoutedEventArgs.cs
- SecurityCapabilities.cs
- TypeConstant.cs
- JoinElimination.cs
- SecurityUtils.cs
- FacetValueContainer.cs
- ZipIOExtraFieldPaddingElement.cs
- ErrorsHelper.cs
- ProxyManager.cs
- FlagsAttribute.cs
- DesignerCapabilities.cs
- Table.cs
- DispatcherBuilder.cs
- AtomMaterializerLog.cs
- AlphaSortedEnumConverter.cs
- ListBindingHelper.cs
- KeyboardDevice.cs
- cookiecollection.cs
- Dispatcher.cs
- ViewStateModeByIdAttribute.cs
- OdbcConnection.cs
- InputScopeConverter.cs
- OleDbConnectionInternal.cs
- MetadataFile.cs
- Rfc4050KeyFormatter.cs
- WebPartEventArgs.cs
- DataGridViewCellParsingEventArgs.cs
- MatrixKeyFrameCollection.cs
- FrameworkElementAutomationPeer.cs
- SqlException.cs
- Brushes.cs
- SubMenuStyleCollection.cs
- Directory.cs
- XmlDownloadManager.cs
- ComNativeDescriptor.cs
- BufferedGraphicsContext.cs
- AppDomainResourcePerfCounters.cs
- ThaiBuddhistCalendar.cs
- Style.cs
- ChangeBlockUndoRecord.cs
- ArrayList.cs
- SqlBooleanizer.cs
- tooltip.cs
- Accessible.cs
- ManagedFilter.cs
- Vector3DKeyFrameCollection.cs
- DataBindingHandlerAttribute.cs
- CqlParserHelpers.cs
- _NegotiateClient.cs
- Atom10FormatterFactory.cs
- ConnectionProviderAttribute.cs
- TerminatorSinks.cs
- DrawListViewItemEventArgs.cs
- CallbackBehaviorAttribute.cs
- TemplatedAdorner.cs
- XamlFilter.cs
- LayoutTable.cs
- ResourceCategoryAttribute.cs
- BindingOperations.cs
- XhtmlMobileTextWriter.cs
- JsonSerializer.cs
- GatewayIPAddressInformationCollection.cs
- UnauthorizedWebPart.cs
- DetailsViewRow.cs
- UInt64Storage.cs
- TypeConverter.cs
- ToolStripProgressBar.cs
- TaskForm.cs
- Setter.cs
- M3DUtil.cs