Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / Atom10FormatterFactory.cs / 1305376 / Atom10FormatterFactory.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a formatter factory for ATOM 1.0. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System.Diagnostics; using System.IO; using System.ServiceModel.Syndication; using System.Text; using System.Xml; ///Provides support for serializing responses in ATOM 1.0 format. ////// For more information, see http://tools.ietf.org/html/rfc4287. /// internal sealed class Atom10FormatterFactory : SyndicationFormatterFactory { ///Creates a new instance of the ///class. A new instance of the internal override SyndicationFeedFormatter CreateSyndicationFeedFormatter() { return new Atom10FeedFormatter(); } ///class. /// Creates a new instance of the /// Theclass with the specified /// instance. /// to serialize. /// /// A new instance of the internal override SyndicationFeedFormatter CreateSyndicationFeedFormatter(SyndicationFeed feedToWrite) { Debug.Assert(feedToWrite != null, "feedToWrite != null"); return new Atom10FeedFormatter(feedToWrite); } ///class with the specified /// instance. /// Creates a new instance of the ///class. A new instance of the internal override SyndicationItemFormatter CreateSyndicationItemFormatter() { return new Atom10ItemFormatter(); } ///class. /// Creates a new instance of the /// Theclass with the specified /// instance. /// to serialize. /// A new instance of the internal override SyndicationItemFormatter CreateSyndicationItemFormatter(SyndicationItem itemToWrite) { Debug.Assert(itemToWrite != null, "itemToWrite != null"); string value; if (itemToWrite.AttributeExtensions.TryGetValue(SyndicationSerializer.QualifiedNullAttribute, out value) && value == XmlConstants.XmlTrueLiteral) { return null; } return new Atom10ItemFormatter(itemToWrite); } ///class. /// Creates an /// Stream over which to read (the reader should close it when it's done with it). /// Encoding of the stream, possibly null. ///over the specified with the given /// , to be used with an appropriate formatter. /// A new internal override XmlReader CreateReader(Stream stream, Encoding encoding) { Debug.Assert(stream != null, "stream != null"); return XmlUtil.CreateXmlReader(stream, encoding); } ///instance. /// Creates an /// Stream over which to write (the writer should close it when it's done with it). /// Encoding of the stream. ///into the specified with the given /// , to be used with an appropriate formatter. /// A new internal override XmlWriter CreateWriter(Stream stream, Encoding encoding) { Debug.Assert(stream != null, "stream != null"); Debug.Assert(encoding != null, "encoding != null"); return XmlUtil.CreateXmlWriterAndWriteProcessingInstruction(stream, encoding); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //instance. // Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a formatter factory for ATOM 1.0. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System.Diagnostics; using System.IO; using System.ServiceModel.Syndication; using System.Text; using System.Xml; ///Provides support for serializing responses in ATOM 1.0 format. ////// For more information, see http://tools.ietf.org/html/rfc4287. /// internal sealed class Atom10FormatterFactory : SyndicationFormatterFactory { ///Creates a new instance of the ///class. A new instance of the internal override SyndicationFeedFormatter CreateSyndicationFeedFormatter() { return new Atom10FeedFormatter(); } ///class. /// Creates a new instance of the /// Theclass with the specified /// instance. /// to serialize. /// /// A new instance of the internal override SyndicationFeedFormatter CreateSyndicationFeedFormatter(SyndicationFeed feedToWrite) { Debug.Assert(feedToWrite != null, "feedToWrite != null"); return new Atom10FeedFormatter(feedToWrite); } ///class with the specified /// instance. /// Creates a new instance of the ///class. A new instance of the internal override SyndicationItemFormatter CreateSyndicationItemFormatter() { return new Atom10ItemFormatter(); } ///class. /// Creates a new instance of the /// Theclass with the specified /// instance. /// to serialize. /// A new instance of the internal override SyndicationItemFormatter CreateSyndicationItemFormatter(SyndicationItem itemToWrite) { Debug.Assert(itemToWrite != null, "itemToWrite != null"); string value; if (itemToWrite.AttributeExtensions.TryGetValue(SyndicationSerializer.QualifiedNullAttribute, out value) && value == XmlConstants.XmlTrueLiteral) { return null; } return new Atom10ItemFormatter(itemToWrite); } ///class. /// Creates an /// Stream over which to read (the reader should close it when it's done with it). /// Encoding of the stream, possibly null. ///over the specified with the given /// , to be used with an appropriate formatter. /// A new internal override XmlReader CreateReader(Stream stream, Encoding encoding) { Debug.Assert(stream != null, "stream != null"); return XmlUtil.CreateXmlReader(stream, encoding); } ///instance. /// Creates an /// Stream over which to write (the writer should close it when it's done with it). /// Encoding of the stream. ///into the specified with the given /// , to be used with an appropriate formatter. /// A new internal override XmlWriter CreateWriter(Stream stream, Encoding encoding) { Debug.Assert(stream != null, "stream != null"); Debug.Assert(encoding != null, "encoding != null"); return XmlUtil.CreateXmlWriterAndWriteProcessingInstruction(stream, encoding); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.instance.
Link Menu
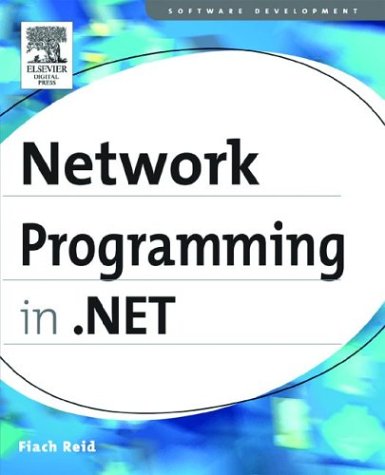
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationInteropProvider.cs
- XhtmlBasicFormAdapter.cs
- AuthorizationRuleCollection.cs
- FileUpload.cs
- Visual.cs
- BaseResourcesBuildProvider.cs
- SerializationStore.cs
- Scanner.cs
- SqlDelegatedTransaction.cs
- BaseDataList.cs
- GridViewRowEventArgs.cs
- SemaphoreFullException.cs
- RequestTimeoutManager.cs
- Baml6ConstructorInfo.cs
- Selection.cs
- PackageController.cs
- XMLSyntaxException.cs
- ScrollContentPresenter.cs
- AvtEvent.cs
- FontDriver.cs
- Helper.cs
- MultiView.cs
- EUCJPEncoding.cs
- DesignerForm.cs
- XmlStreamStore.cs
- BaseHashHelper.cs
- ViewManager.cs
- ClaimTypes.cs
- Mutex.cs
- StandardOleMarshalObject.cs
- ReachIDocumentPaginatorSerializerAsync.cs
- ConnectivityStatus.cs
- Point3DAnimation.cs
- WindowsMenu.cs
- SizeAnimationBase.cs
- WebDisplayNameAttribute.cs
- OleDbConnectionInternal.cs
- ValuePatternIdentifiers.cs
- XmlCustomFormatter.cs
- TextServicesPropertyRanges.cs
- WindowVisualStateTracker.cs
- SiteMapNodeCollection.cs
- ContentFilePart.cs
- DataStorage.cs
- RichTextBoxConstants.cs
- ListenerAdapterBase.cs
- DiagnosticsConfiguration.cs
- TextStore.cs
- Rect.cs
- IdentitySection.cs
- ReplacementText.cs
- InputLangChangeEvent.cs
- SqlSupersetValidator.cs
- ValidationEventArgs.cs
- FillErrorEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- SmuggledIUnknown.cs
- PrintPreviewControl.cs
- WebScriptServiceHostFactory.cs
- MarkupExtensionParser.cs
- CharConverter.cs
- UniqueEventHelper.cs
- AttributeEmitter.cs
- HwndProxyElementProvider.cs
- TemplateBindingExpression.cs
- XmlWriter.cs
- DoubleCollectionConverter.cs
- MarshalByRefObject.cs
- StringAnimationUsingKeyFrames.cs
- Aggregates.cs
- SliderAutomationPeer.cs
- CheckedPointers.cs
- SignatureDescription.cs
- CompilationRelaxations.cs
- DiffuseMaterial.cs
- XslAst.cs
- TabPage.cs
- XPathAxisIterator.cs
- Certificate.cs
- IgnorePropertiesAttribute.cs
- OleDbCommand.cs
- cookiecontainer.cs
- TypeBuilder.cs
- XmlDataLoader.cs
- PageParser.cs
- WrapPanel.cs
- BulletedList.cs
- KeyEvent.cs
- IPHostEntry.cs
- WebBrowserBase.cs
- DataTablePropertyDescriptor.cs
- ExpressionBuilder.cs
- XmlReader.cs
- XmlReaderSettings.cs
- DataService.cs
- ServiceModelExtensionElement.cs
- DrawingAttributeSerializer.cs
- ColorContextHelper.cs
- CodeRegionDirective.cs
- DesignerSelectionListAdapter.cs