Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / PackageController.cs / 1 / PackageController.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// Responsible for the lifecycle of the PackageDocument and the actions that can // be performed on it. // // // History: // 08/28/2005: [....]: Initial implementation. //----------------------------------------------------------------------------- using System; using System.IO.Packaging; using System.Security; using System.Windows.TrustUI; using System.Windows.Xps.Packaging; namespace MS.Internal.Documents.Application { ////// Responsible for the lifecycle of the PackageDocument and the actions that can /// be performed on it. /// internal class PackageController : IDocumentController { #region IDocumentController Members //------------------------------------------------------------------------- // IDocumentController Members //------------------------------------------------------------------------- ////// /// ////// /// Critical: /// - we are calling the ctor for TransactionalPackage /// TreatAsSafe: /// - safe by definition we are the one method responsibile for setting /// this value /// - the stream is the Workspace stream which is created based on the /// source stream /// [SecurityCritical, SecurityTreatAsSafe] bool IDocumentController.EnableEdit(Document document) { PackageDocument doc = (PackageDocument)document; if (doc.Workspace != null) { doc.Package.EnableEditMode(doc.Workspace); } return true; } ////// ////// /// Critical: /// - we are calling the ctor for TransactionalPackage /// - sets Package /// - creates DigitalSignatureProvider /// - creates DocumentSignatureManager /// TreatAsSafe: /// - safe by definition we are the one method responsible for setting /// this value /// - the stream is the source stream for this XpsDocument /// - source of package is our underlying stream which is protected /// - provider passed to DocumentSignatureManager is created in this /// method from a package created from the underlying stream /// [SecurityCritical, SecurityTreatAsSafe] bool IDocumentController.Open(Document document) { PackageDocument doc = (PackageDocument)document; TransactionalPackage package = new RestrictedTransactionalPackage( doc.Source); doc.Package = package; DocumentProperties.Current.SetXpsProperties(package.PackageProperties); DocumentSignatureManager.Initialize(new DigitalSignatureProvider(package)); // when signatures change (are added or removed) we can no longer simply copy bits on disk DocumentSignatureManager.Current.SignaturesChanged += new EventHandler( delegate ( object sender, EventArgs args) { if (doc.IsFileCopySafe) { Trace.SafeWrite( Trace.Signatures, "Disabling file copy for current document."); doc.IsFileCopySafe = false; } }); return true; } ////// ////// /// Critical: /// - we are calling the Rebind for TransactionalPackage /// TreatAsSafe: /// - safe by definition we are the one method responsibile for setting /// this value /// - the stream is the source stream for this XpsDocument /// - source of package is our underlying stream which is protected /// [SecurityCritical, SecurityTreatAsSafe] bool IDocumentController.Rebind(Document document) { PackageDocument doc = (PackageDocument)document; if (doc.IsRebindNeeded) { doc.Package.Rebind(doc.Source); // no rebinds are needed above us for documents as package doc.IsRebindNeeded = false; } return true; } ////// bool IDocumentController.SaveAsPreperation(Document document) { return ((IDocumentController)this).SavePreperation(document); } ////// /// ////// /// /// Critical: /// - we are calling the MergeChanges for TransactionalPackage /// TreatAsSafe: /// - safe by definition we are the one method responsibile for calling /// this member /// - the stream is the destination stream for this XpsDocument /// [SecurityCritical, SecurityTreatAsSafe] bool IDocumentController.SaveCommit(Document document) { PackageDocument doc = (PackageDocument)document; if (doc.Destination == null) { return false; } if (doc.Package.IsDirty || !doc.IsDestinationIdenticalToSource) { StreamProxy source = doc.Source as StreamProxy; StreamProxy destination = doc.Destination as StreamProxy; // this will catch the case where our source stream is our destination // stream, which would cause corruption of the package if (source.GetHashCode() == destination.GetHashCode()) { throw new InvalidOperationException( SR.Get(SRID.PackageControllerStreamCorruption)); } // this will catch the case where no one was able to copy the stream // thus the lengths will not match if (doc.Source.Length != doc.Destination.Length) { throw new InvalidOperationException( SR.Get(SRID.PackageControllerStreamCorruption)); } // Flush the package to ensure that the relationship parts are // written out before changes are merged doc.Package.Flush(); doc.Package.MergeChanges(doc.Destination); Trace.SafeWrite(Trace.File, "Destination Merged."); } else { Trace.SafeWrite(Trace.File, "Destination Merge Skipped (nothing to do)."); } return true; } ////// ////// /// This method severely breaks encapsulating the chain, however it does /// so because the requirement breaks encapsulation and the original design /// of the class only consider streams not properties. The requirement is /// that as we transition to/from a protected document we copy properties /// between the layers. It was least risk to compromise the design here /// then extend it. /// ////// Critical: /// - we construct UmcSuppressedProperties /// - we call DocumentProperties.Copy /// TreatAsSafe: /// - EncryptedPackage (which require RightsManagementPermission) is the /// only type for the UmcSuppressProperties ctor; this is safe because this /// is the correct source for the data /// - copy is safe; it's only critical because of the data we are passing /// [SecurityCritical, SecurityTreatAsSafe] bool IDocumentController.SavePreperation(Document document) { // we don't have to check packageDoc for because we are only responsible // for PackageDocuments PackageDocument packageDoc = (PackageDocument)document; RightsDocument rightsDoc = document.Dependency as RightsDocument; if (rightsDoc != null) { bool isDestinationProtected = rightsDoc.IsDestinationProtected(); bool isSourceProtected = rightsDoc.IsSourceProtected(); // the only time we don't need to copy properties is when // neither source nor destination is protected as OPC properties // are copied as parts // if the source was protected and the destination is not // then we need to copy properties to the package if (isSourceProtected && !isDestinationProtected) { DocumentProperties.Copy( new SuppressedProperties(rightsDoc.SourcePackage), packageDoc.Package.PackageProperties); } // if the source was not protected and the destination is // then we need to copy properties from the package to the envelope if (!isSourceProtected && isDestinationProtected) { DocumentProperties.Copy( packageDoc.Package.PackageProperties, new SuppressedProperties(rightsDoc.DestinationPackage)); } // if they were both protected we need to copy properties // from the old envelope to the new if (isDestinationProtected && isSourceProtected) { DocumentProperties.Copy( new SuppressedProperties(rightsDoc.SourcePackage), new SuppressedProperties(rightsDoc.DestinationPackage)); } } return true; } #endregion IDocumentController Members #region IChainOfResponsibiltyNodeMembers //-------------------------------------------------------------------------- // IChainOfResponsibiltyNode Members //------------------------------------------------------------------------- /// /// bool IChainOfResponsibiltyNode/// .IsResponsible(Document subject) { return subject is PackageDocument; } #endregion IChainOfResponsibiltyNode Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
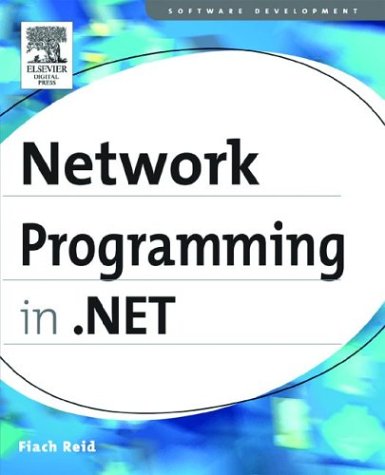
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlParameter.cs
- CompositeScriptReference.cs
- FrameworkElementFactory.cs
- ProtocolViolationException.cs
- _FixedSizeReader.cs
- Vector3DConverter.cs
- DesignerDataSourceView.cs
- ReadOnlyMetadataCollection.cs
- ResourceReader.cs
- ResXResourceReader.cs
- WebBrowserDocumentCompletedEventHandler.cs
- WCFServiceClientProxyGenerator.cs
- SigningProgress.cs
- TypeLoadException.cs
- InvokeMethodActivity.cs
- TextAutomationPeer.cs
- WebPartConnectionsCancelVerb.cs
- DocumentViewerBase.cs
- PolyQuadraticBezierSegment.cs
- ExceptionHelpers.cs
- WebPartCatalogAddVerb.cs
- BindValidationContext.cs
- ClassImporter.cs
- CodePageUtils.cs
- ServiceDocument.cs
- BitArray.cs
- ApplicationInfo.cs
- WindowsComboBox.cs
- TemplatedWizardStep.cs
- Compress.cs
- ObjectIDGenerator.cs
- AuthenticationConfig.cs
- BaseDataBoundControl.cs
- PackageDigitalSignatureManager.cs
- Control.cs
- InternalCache.cs
- Line.cs
- ExtenderHelpers.cs
- ExchangeUtilities.cs
- SiteOfOriginPart.cs
- DesignerToolboxInfo.cs
- X509Utils.cs
- ListView.cs
- TdsRecordBufferSetter.cs
- FilteredReadOnlyMetadataCollection.cs
- WebPartVerbCollection.cs
- MexHttpBindingElement.cs
- JavaScriptObjectDeserializer.cs
- ModelItemExtensions.cs
- HtmlInputCheckBox.cs
- XmlStreamStore.cs
- WebPartZone.cs
- SBCSCodePageEncoding.cs
- XmlResolver.cs
- BackgroundFormatInfo.cs
- BufferBuilder.cs
- _BufferOffsetSize.cs
- LinkButton.cs
- HttpListenerException.cs
- RuntimeWrappedException.cs
- SpellerInterop.cs
- VBCodeProvider.cs
- XmlWriterSettings.cs
- HtmlMeta.cs
- LicenseException.cs
- Matrix3DStack.cs
- UserValidatedEventArgs.cs
- StandardTransformFactory.cs
- DefaultEventAttribute.cs
- LocalizabilityAttribute.cs
- CollectionAdapters.cs
- CodeSnippetCompileUnit.cs
- DesignerForm.cs
- XamlSerializationHelper.cs
- EntityViewGenerationConstants.cs
- DrawingVisual.cs
- SqlVisitor.cs
- ObjectAssociationEndMapping.cs
- controlskin.cs
- WmfPlaceableFileHeader.cs
- ErrorItem.cs
- xdrvalidator.cs
- Codec.cs
- XmlWhitespace.cs
- PersonalizableAttribute.cs
- RandomNumberGenerator.cs
- WebPartZoneBase.cs
- DynamicResourceExtension.cs
- objectresult_tresulttype.cs
- SocketElement.cs
- JoinTreeSlot.cs
- ControlValuePropertyAttribute.cs
- TimeSpanValidatorAttribute.cs
- ExceptionHandler.cs
- ToolStripItemTextRenderEventArgs.cs
- SafeSecurityHelper.cs
- SupportsEventValidationAttribute.cs
- LambdaCompiler.cs
- BindingValueChangedEventArgs.cs
- PartialTrustVisibleAssembliesSection.cs