Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Policy / UnionCodeGroup.cs / 1 / UnionCodeGroup.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // UnionCodeGroup.cs // // Representation for code groups used for the policy mechanism // namespace System.Security.Policy { using System; using System.Security.Util; using System.Security; using System.Collections; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class UnionCodeGroup : CodeGroup, IUnionSemanticCodeGroup { internal UnionCodeGroup() : base() { } internal UnionCodeGroup( IMembershipCondition membershipCondition, PermissionSet permSet ) : base( membershipCondition, permSet ) { } public UnionCodeGroup( IMembershipCondition membershipCondition, PolicyStatement policy ) : base( membershipCondition, policy ) { } public override PolicyStatement Resolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); object usedEvidence = null; if (PolicyManager.CheckMembershipCondition(MembershipCondition, evidence, out usedEvidence)) { PolicyStatement thisPolicy = PolicyStatement; // PolicyStatement getter makes a copy for us // If any delay-evidence was used to generate this grant set, then we need to keep track of // that for potentially later forcing it to be verified. IDelayEvaluatedEvidence delayEvidence = usedEvidence as IDelayEvaluatedEvidence; bool delayEvidenceNeedsVerification = delayEvidence != null && !delayEvidence.IsVerified; if (delayEvidenceNeedsVerification) { thisPolicy.AddDependentEvidence(delayEvidence); } bool foundExclusiveChild = false; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext() && !foundExclusiveChild) { PolicyStatement childPolicy = PolicyManager.ResolveCodeGroup(enumerator.Current as CodeGroup, evidence); if (childPolicy != null) { thisPolicy.InplaceUnion(childPolicy); if ((childPolicy.Attributes & PolicyStatementAttribute.Exclusive) == PolicyStatementAttribute.Exclusive) { foundExclusiveChild = true; } } } return thisPolicy; } else { return null; } } ///PolicyStatement IUnionSemanticCodeGroup.InternalResolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { return this.PolicyStatement; } else { return null; } } public override CodeGroup ResolveMatchingCodeGroups( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { CodeGroup retGroup = this.Copy(); retGroup.Children = new ArrayList(); IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { CodeGroup matchingGroups = ((CodeGroup)enumerator.Current).ResolveMatchingCodeGroups( evidence ); // If the child has a policy, we are done. if (matchingGroups != null) { retGroup.AddChild( matchingGroups ); } } return retGroup; } else { return null; } } public override CodeGroup Copy() { UnionCodeGroup group = new UnionCodeGroup(); group.MembershipCondition = this.MembershipCondition; group.PolicyStatement = this.PolicyStatement; group.Name = this.Name; group.Description = this.Description; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { group.AddChild( (CodeGroup)enumerator.Current ); } return group; } public override String MergeLogic { get { return Environment.GetResourceString( "MergeLogic_Union" ); } } internal override String GetTypeName() { return "System.Security.Policy.UnionCodeGroup"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // UnionCodeGroup.cs // // Representation for code groups used for the policy mechanism // namespace System.Security.Policy { using System; using System.Security.Util; using System.Security; using System.Collections; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class UnionCodeGroup : CodeGroup, IUnionSemanticCodeGroup { internal UnionCodeGroup() : base() { } internal UnionCodeGroup( IMembershipCondition membershipCondition, PermissionSet permSet ) : base( membershipCondition, permSet ) { } public UnionCodeGroup( IMembershipCondition membershipCondition, PolicyStatement policy ) : base( membershipCondition, policy ) { } public override PolicyStatement Resolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); object usedEvidence = null; if (PolicyManager.CheckMembershipCondition(MembershipCondition, evidence, out usedEvidence)) { PolicyStatement thisPolicy = PolicyStatement; // PolicyStatement getter makes a copy for us // If any delay-evidence was used to generate this grant set, then we need to keep track of // that for potentially later forcing it to be verified. IDelayEvaluatedEvidence delayEvidence = usedEvidence as IDelayEvaluatedEvidence; bool delayEvidenceNeedsVerification = delayEvidence != null && !delayEvidence.IsVerified; if (delayEvidenceNeedsVerification) { thisPolicy.AddDependentEvidence(delayEvidence); } bool foundExclusiveChild = false; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext() && !foundExclusiveChild) { PolicyStatement childPolicy = PolicyManager.ResolveCodeGroup(enumerator.Current as CodeGroup, evidence); if (childPolicy != null) { thisPolicy.InplaceUnion(childPolicy); if ((childPolicy.Attributes & PolicyStatementAttribute.Exclusive) == PolicyStatementAttribute.Exclusive) { foundExclusiveChild = true; } } } return thisPolicy; } else { return null; } } /// PolicyStatement IUnionSemanticCodeGroup.InternalResolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { return this.PolicyStatement; } else { return null; } } public override CodeGroup ResolveMatchingCodeGroups( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { CodeGroup retGroup = this.Copy(); retGroup.Children = new ArrayList(); IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { CodeGroup matchingGroups = ((CodeGroup)enumerator.Current).ResolveMatchingCodeGroups( evidence ); // If the child has a policy, we are done. if (matchingGroups != null) { retGroup.AddChild( matchingGroups ); } } return retGroup; } else { return null; } } public override CodeGroup Copy() { UnionCodeGroup group = new UnionCodeGroup(); group.MembershipCondition = this.MembershipCondition; group.PolicyStatement = this.PolicyStatement; group.Name = this.Name; group.Description = this.Description; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { group.AddChild( (CodeGroup)enumerator.Current ); } return group; } public override String MergeLogic { get { return Environment.GetResourceString( "MergeLogic_Union" ); } } internal override String GetTypeName() { return "System.Security.Policy.UnionCodeGroup"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
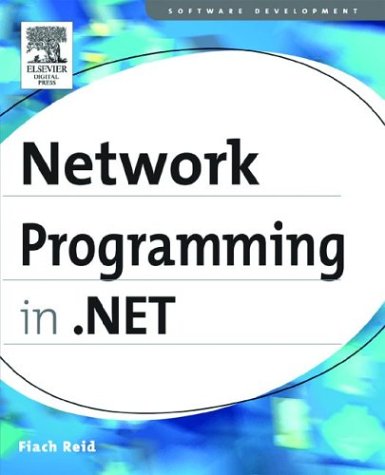
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuildManager.cs
- SafeFileMappingHandle.cs
- Compiler.cs
- DataGridViewControlCollection.cs
- DataControlLinkButton.cs
- processwaithandle.cs
- HandleCollector.cs
- HtmlTitle.cs
- UnconditionalPolicy.cs
- OdbcConnectionFactory.cs
- BitmapSourceSafeMILHandle.cs
- ProxyElement.cs
- Group.cs
- SecurityCookieModeValidator.cs
- SqlLiftIndependentRowExpressions.cs
- bidPrivateBase.cs
- KoreanCalendar.cs
- ZoneButton.cs
- InputLanguageSource.cs
- SessionParameter.cs
- TransformValueSerializer.cs
- PiiTraceSource.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- SettingsSavedEventArgs.cs
- MimeTypeMapper.cs
- SqlDependencyListener.cs
- Slider.cs
- DataBoundControlHelper.cs
- itemelement.cs
- NameService.cs
- ConnectionManager.cs
- HttpFileCollection.cs
- AjaxFrameworkAssemblyAttribute.cs
- FormsAuthenticationEventArgs.cs
- DataSourceSelectArguments.cs
- COM2ICategorizePropertiesHandler.cs
- XmlAttributeCollection.cs
- OutputCacheProfileCollection.cs
- Function.cs
- Visual.cs
- StandardOleMarshalObject.cs
- PageCache.cs
- SecurityTokenSerializer.cs
- SecurityTokenResolver.cs
- GeneralTransform3DGroup.cs
- DataGridCell.cs
- IResourceProvider.cs
- ChangePassword.cs
- GACMembershipCondition.cs
- ProfileSection.cs
- Number.cs
- ContentFileHelper.cs
- EnvironmentPermission.cs
- RuleSettings.cs
- FlowDocumentReader.cs
- StorageSetMapping.cs
- CqlParser.cs
- AlgoModule.cs
- WebReferenceCollection.cs
- DynamicScriptObject.cs
- DataProtection.cs
- SplitContainer.cs
- NativeActivityMetadata.cs
- ProviderCollection.cs
- AttachedPropertiesService.cs
- RoutingService.cs
- SafeArrayTypeMismatchException.cs
- CreateUserErrorEventArgs.cs
- PeerCustomResolverSettings.cs
- SerializationObjectManager.cs
- TextRangeEditTables.cs
- CharConverter.cs
- MexHttpsBindingCollectionElement.cs
- SoapExtensionTypeElementCollection.cs
- MultipartIdentifier.cs
- GcHandle.cs
- UIInitializationException.cs
- StringToken.cs
- RepeatInfo.cs
- OleDbEnumerator.cs
- TreeViewAutomationPeer.cs
- DataSetMappper.cs
- QueueException.cs
- HttpModuleAction.cs
- LayoutDump.cs
- InvalidPropValue.cs
- HelpHtmlBuilder.cs
- DataRelation.cs
- VoiceSynthesis.cs
- BitmapImage.cs
- UnionCodeGroup.cs
- EventArgs.cs
- DecoderReplacementFallback.cs
- EventInfo.cs
- TemplatingOptionsDialog.cs
- MenuItemBinding.cs
- StorageEndPropertyMapping.cs
- SimplePropertyEntry.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- DelayedRegex.cs