Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlAuthorityBinding.cs / 1 / SamlAuthorityBinding.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Xml; using System.Xml.Serialization; using System.Runtime.Serialization; using System.IdentityModel.Selectors; [DataContract] public class SamlAuthorityBinding { XmlQualifiedName authorityKind; string binding; string location; [DataMember] bool isReadOnly = false; public SamlAuthorityBinding(XmlQualifiedName authorityKind, string binding, string location) { this.AuthorityKind = authorityKind; this.Binding = binding; this.Location = location; CheckObjectValidity(); } public SamlAuthorityBinding() { } [DataMember] public XmlQualifiedName AuthorityKind { get {return this.authorityKind; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (String.IsNullOrEmpty(value.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityKindMissingName)); this.authorityKind = value; } } [DataMember] public string Binding { get {return this.binding; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding)); this.binding = value; } } [DataMember] public string Location { get {return this.location; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation)); this.location = value; } } public bool IsReadOnly { get { return this.isReadOnly; } } public void MakeReadOnly() { this.isReadOnly = true; } void CheckObjectValidity() { if (this.authorityKind == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKind))); if (String.IsNullOrEmpty(authorityKind.Name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityKindMissingName))); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresBinding))); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingRequiresLocation))); } public virtual void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; string authKind = reader.GetAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(authKind)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingAuthorityKindOnRead))); string[] authKindParts = authKind.Split(':'); if (authKindParts.Length > 2) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingInvalidAuthorityKind))); string localName; string prefix; string nameSpace; if (authKindParts.Length == 2) { prefix = authKindParts[0]; localName = authKindParts[1]; } else { prefix = String.Empty; localName = authKindParts[0]; } nameSpace = reader.LookupNamespace(prefix); this.authorityKind = new XmlQualifiedName(localName, nameSpace); this.binding = reader.GetAttribute(dictionary.Binding, null); if (String.IsNullOrEmpty(this.binding)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingBindingOnRead))); this.location = reader.GetAttribute(dictionary.Location, null); if (String.IsNullOrEmpty(this.location)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAuthorityBindingMissingLocationOnRead))); if (reader.IsEmptyElement) { reader.MoveToContent(); reader.Read(); } else { reader.MoveToContent(); reader.Read(); reader.ReadEndElement(); } } public virtual void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AuthorityBinding, dictionary.Namespace); string prefix = null; if (!String.IsNullOrEmpty(this.authorityKind.Namespace)) { writer.WriteAttributeString(String.Empty, dictionary.NamespaceAttributePrefix.Value, null, this.authorityKind.Namespace); prefix = writer.LookupPrefix(this.authorityKind.Namespace); } writer.WriteStartAttribute(dictionary.AuthorityKind, null); if (String.IsNullOrEmpty(prefix)) writer.WriteString(this.authorityKind.Name); else writer.WriteString(prefix + ":" + this.authorityKind.Name); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Location, null); writer.WriteString(this.location); writer.WriteEndAttribute(); writer.WriteStartAttribute(dictionary.Binding, null); writer.WriteString(this.binding); writer.WriteEndAttribute(); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
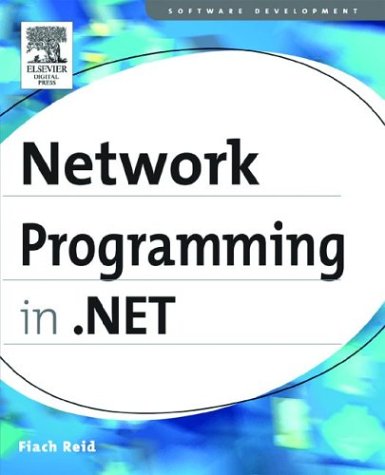
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeOfExpression.cs
- BaseResourcesBuildProvider.cs
- GenericEnumerator.cs
- Clause.cs
- XmlValidatingReaderImpl.cs
- DataServiceQueryException.cs
- CookieHandler.cs
- Group.cs
- PropertyConverter.cs
- LogRestartAreaEnumerator.cs
- BaseParaClient.cs
- EncoderNLS.cs
- PrintingPermission.cs
- InternalMappingException.cs
- WindowsEditBox.cs
- DragDrop.cs
- ExpressionBinding.cs
- UnknownWrapper.cs
- TimersDescriptionAttribute.cs
- BindingContext.cs
- FunctionNode.cs
- UTF32Encoding.cs
- IdentityReference.cs
- BufferModeSettings.cs
- UniqueEventHelper.cs
- OleDbParameterCollection.cs
- ClientEventManager.cs
- HtmlMeta.cs
- ScriptIgnoreAttribute.cs
- StorageMappingItemLoader.cs
- Journaling.cs
- HierarchicalDataTemplate.cs
- BooleanFunctions.cs
- WindowsEditBox.cs
- SystemResources.cs
- SelectionEditingBehavior.cs
- URLAttribute.cs
- Accessible.cs
- JumpItem.cs
- CodePropertyReferenceExpression.cs
- WSSecurityJan2004.cs
- DtdParser.cs
- QueryContinueDragEventArgs.cs
- WebPartZoneCollection.cs
- AssociationSet.cs
- Dictionary.cs
- LocalizedNameDescriptionPair.cs
- TagNameToTypeMapper.cs
- PersonalizationProviderCollection.cs
- MembershipPasswordException.cs
- CacheVirtualItemsEvent.cs
- HttpCacheVaryByContentEncodings.cs
- SapiGrammar.cs
- CriticalFinalizerObject.cs
- ResolveInfo.cs
- ErrorProvider.cs
- DoubleLink.cs
- EncoderNLS.cs
- QilLiteral.cs
- RawStylusInputCustomDataList.cs
- MDIClient.cs
- InvokeHandlers.cs
- FamilyCollection.cs
- _AuthenticationState.cs
- COAUTHIDENTITY.cs
- DataSourceControl.cs
- InArgumentConverter.cs
- DependencyObject.cs
- CompilerLocalReference.cs
- IPEndPoint.cs
- XamlGridLengthSerializer.cs
- TraceContextRecord.cs
- UnsafeNativeMethods.cs
- ListViewItemSelectionChangedEvent.cs
- DynamicPropertyHolder.cs
- ToolStripDropDownClosedEventArgs.cs
- DbConnectionInternal.cs
- StringBuilder.cs
- TextEffect.cs
- PassportIdentity.cs
- SerializerProvider.cs
- BuildProvider.cs
- ReaderOutput.cs
- AlgoModule.cs
- ObfuscationAttribute.cs
- SpStreamWrapper.cs
- WebResponse.cs
- TextEditorCharacters.cs
- Pen.cs
- MenuItem.cs
- HealthMonitoringSectionHelper.cs
- DiscoveryExceptionDictionary.cs
- ExpressionConverter.cs
- SecurityMessageProperty.cs
- EventPropertyMap.cs
- ProcessModelSection.cs
- Msec.cs
- TrimSurroundingWhitespaceAttribute.cs
- VariableAction.cs
- RoleService.cs