Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / GenericEnumerator.cs / 1 / GenericEnumerator.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GenericEnumerator.cs //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Windows; using MS.Utility; #if PRESENTATION_CORE using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #else using SR=System.Windows.SR; using SRID=System.Windows.SRID; #endif namespace MS.Internal { ////// GenericEnumerator /// internal class GenericEnumerator : IEnumerator { #region Delegates internal delegate int GetGenerationIDDelegate(); #endregion #region Constructors private GenericEnumerator() { } internal GenericEnumerator(IList array, GetGenerationIDDelegate getGenerationID) { _array = array; _count = _array.Count; _position = -1; _getGenerationID = getGenerationID; _originalGenerationID = _getGenerationID(); } #endregion #region Private private void VerifyCurrent() { if ( (-1 == _position) || (_position >= _count)) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); } } #endregion #region IEnumerator ////// Returns the object at the current location of the key times list. /// Use the strongly typed version instead. /// object IEnumerator.Current { get { VerifyCurrent(); return _current; } } ////// Move to the next value in the key times list /// ///true if succeeded, false if at the end of the list public bool MoveNext() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } _position++; if (_position >= _count) { _position = _count; return false; } else { Debug.Assert(_position >= 0); _current = _array[_position]; return true; } } ////// Move to the position before the first value in the list. /// public void Reset() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } else { _position = -1; } } #endregion #region Data private IList _array; private object _current; private int _count; private int _position; private int _originalGenerationID; private GetGenerationIDDelegate _getGenerationID; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GenericEnumerator.cs //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Windows; using MS.Utility; #if PRESENTATION_CORE using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #else using SR=System.Windows.SR; using SRID=System.Windows.SRID; #endif namespace MS.Internal { ////// GenericEnumerator /// internal class GenericEnumerator : IEnumerator { #region Delegates internal delegate int GetGenerationIDDelegate(); #endregion #region Constructors private GenericEnumerator() { } internal GenericEnumerator(IList array, GetGenerationIDDelegate getGenerationID) { _array = array; _count = _array.Count; _position = -1; _getGenerationID = getGenerationID; _originalGenerationID = _getGenerationID(); } #endregion #region Private private void VerifyCurrent() { if ( (-1 == _position) || (_position >= _count)) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); } } #endregion #region IEnumerator ////// Returns the object at the current location of the key times list. /// Use the strongly typed version instead. /// object IEnumerator.Current { get { VerifyCurrent(); return _current; } } ////// Move to the next value in the key times list /// ///true if succeeded, false if at the end of the list public bool MoveNext() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } _position++; if (_position >= _count) { _position = _count; return false; } else { Debug.Assert(_position >= 0); _current = _array[_position]; return true; } } ////// Move to the position before the first value in the list. /// public void Reset() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } else { _position = -1; } } #endregion #region Data private IList _array; private object _current; private int _count; private int _position; private int _originalGenerationID; private GetGenerationIDDelegate _getGenerationID; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
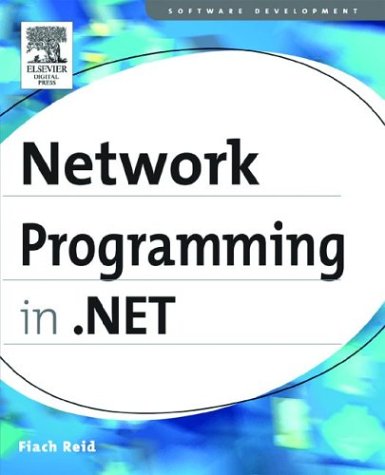
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateMachineSubscriptionManager.cs
- NetworkCredential.cs
- OverrideMode.cs
- _TLSstream.cs
- ChtmlTextWriter.cs
- PeerToPeerException.cs
- HostedAspNetEnvironment.cs
- ApplicationFileParser.cs
- EventLogEntry.cs
- HttpWrapper.cs
- DataGridViewColumnCollection.cs
- ViewValidator.cs
- ErrorFormatterPage.cs
- ParsedAttributeCollection.cs
- TraceUtility.cs
- SlipBehavior.cs
- ComponentChangedEvent.cs
- PasswordPropertyTextAttribute.cs
- ObjectTag.cs
- MouseButtonEventArgs.cs
- PanelDesigner.cs
- SizeKeyFrameCollection.cs
- FixUp.cs
- PathFigure.cs
- WindowsTreeView.cs
- Italic.cs
- EDesignUtil.cs
- XmlSchemaAnnotated.cs
- ResolveNameEventArgs.cs
- TypedElement.cs
- ThemeDirectoryCompiler.cs
- Border.cs
- XDRSchema.cs
- BitSet.cs
- SqlMethodCallConverter.cs
- DbDataAdapter.cs
- XmlSchemaSet.cs
- GeometryGroup.cs
- TimeoutException.cs
- ContentIterators.cs
- TemplatedWizardStep.cs
- OdbcPermission.cs
- MachineKeyConverter.cs
- BaseResourcesBuildProvider.cs
- ManipulationCompletedEventArgs.cs
- BaseCodeDomTreeGenerator.cs
- CommonProperties.cs
- AttachInfo.cs
- ServiceSettingsResponseInfo.cs
- ECDiffieHellmanCng.cs
- ColorComboBox.cs
- InheritanceService.cs
- MethodToken.cs
- RecordManager.cs
- ProvidePropertyAttribute.cs
- SizeFConverter.cs
- BoundConstants.cs
- TextBounds.cs
- ActiveXContainer.cs
- SqlUserDefinedTypeAttribute.cs
- SiteMapPath.cs
- ReadOnlyHierarchicalDataSource.cs
- Vector3D.cs
- SqlDataAdapter.cs
- GrowingArray.cs
- TextTreeRootNode.cs
- CustomAttributeBuilder.cs
- SessionParameter.cs
- InvalidOperationException.cs
- HttpProcessUtility.cs
- ADMembershipProvider.cs
- DropSource.cs
- FunctionQuery.cs
- StylusPointCollection.cs
- ProfileProvider.cs
- ExpressionBinding.cs
- RootBrowserWindowAutomationPeer.cs
- HtmlUtf8RawTextWriter.cs
- EventsTab.cs
- AmbientProperties.cs
- SuppressIldasmAttribute.cs
- OperationCanceledException.cs
- DefaultTraceListener.cs
- Point3DAnimation.cs
- ObjectDataSourceEventArgs.cs
- XsltArgumentList.cs
- ArraySet.cs
- UrlSyndicationContent.cs
- PropertyToken.cs
- XmlNamespaceManager.cs
- DbQueryCommandTree.cs
- BitHelper.cs
- SystemIPGlobalStatistics.cs
- XmlSchemaRedefine.cs
- MemoryResponseElement.cs
- DragDropHelper.cs
- ColumnClickEvent.cs
- SecurityTokenAttachmentMode.cs
- SHA256.cs
- SecurityState.cs