Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / SizeKeyFrameCollection.cs / 1 / SizeKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameSizeAnimation /// to animate a Size property value along a set of key frames. /// public class SizeKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static SizeKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new SizeKeyFrameCollection. /// public SizeKeyFrameCollection() : base() { _keyFrames = new List< SizeKeyFrame>(2); } #endregion #region Static Methods ////// An empty SizeKeyFrameCollection. /// public static SizeKeyFrameCollection Empty { get { if (s_emptyCollection == null) { SizeKeyFrameCollection emptyCollection = new SizeKeyFrameCollection(); emptyCollection._keyFrames = new List< SizeKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this SizeKeyFrameCollection. /// ///The copy public new SizeKeyFrameCollection Clone() { return (SizeKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SizeKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the SizeKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of SizeKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the SizeKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the SizeKeyFrames in the collection to an /// array of SizeKeyFrames. /// public void CopyTo(SizeKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a SizeKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((SizeKeyFrame)keyFrame); } ////// Adds a SizeKeyFrame to the collection. /// public int Add(SizeKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all SizeKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given SizeKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((SizeKeyFrame)keyFrame); } ////// Returns true of the collection contains the given SizeKeyFrame. /// public bool Contains(SizeKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given SizeKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((SizeKeyFrame)keyFrame); } ////// Returns the index of a given SizeKeyFrame in the collection. /// public int IndexOf(SizeKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a SizeKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (SizeKeyFrame)keyFrame); } ////// Inserts a SizeKeyFrame into a specific location in the collection. /// public void Insert(int index, SizeKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a SizeKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((SizeKeyFrame)keyFrame); } ////// Removes a SizeKeyFrame from the collection. /// public void Remove(SizeKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the SizeKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the SizeKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (SizeKeyFrame)value; } } ////// Gets or sets the SizeKeyFrame at a given index. /// public SizeKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "SizeKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameSizeAnimation /// to animate a Size property value along a set of key frames. /// public class SizeKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static SizeKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new SizeKeyFrameCollection. /// public SizeKeyFrameCollection() : base() { _keyFrames = new List< SizeKeyFrame>(2); } #endregion #region Static Methods ////// An empty SizeKeyFrameCollection. /// public static SizeKeyFrameCollection Empty { get { if (s_emptyCollection == null) { SizeKeyFrameCollection emptyCollection = new SizeKeyFrameCollection(); emptyCollection._keyFrames = new List< SizeKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this SizeKeyFrameCollection. /// ///The copy public new SizeKeyFrameCollection Clone() { return (SizeKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SizeKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { SizeKeyFrameCollection sourceCollection = (SizeKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< SizeKeyFrame>(count); for (int i = 0; i < count; i++) { SizeKeyFrame keyFrame = (SizeKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the SizeKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of SizeKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the SizeKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the SizeKeyFrames in the collection to an /// array of SizeKeyFrames. /// public void CopyTo(SizeKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a SizeKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((SizeKeyFrame)keyFrame); } ////// Adds a SizeKeyFrame to the collection. /// public int Add(SizeKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all SizeKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given SizeKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((SizeKeyFrame)keyFrame); } ////// Returns true of the collection contains the given SizeKeyFrame. /// public bool Contains(SizeKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given SizeKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((SizeKeyFrame)keyFrame); } ////// Returns the index of a given SizeKeyFrame in the collection. /// public int IndexOf(SizeKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a SizeKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (SizeKeyFrame)keyFrame); } ////// Inserts a SizeKeyFrame into a specific location in the collection. /// public void Insert(int index, SizeKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a SizeKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((SizeKeyFrame)keyFrame); } ////// Removes a SizeKeyFrame from the collection. /// public void Remove(SizeKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the SizeKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the SizeKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (SizeKeyFrame)value; } } ////// Gets or sets the SizeKeyFrame at a given index. /// public SizeKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "SizeKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
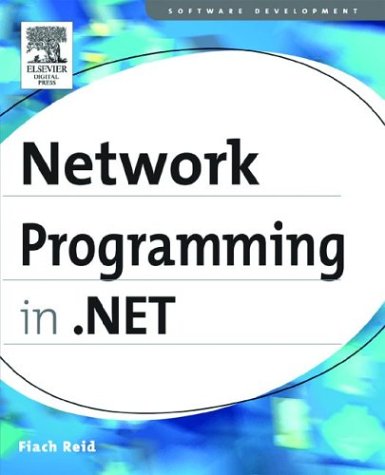
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InfiniteTimeSpanConverter.cs
- XamlLoadErrorInfo.cs
- HistoryEventArgs.cs
- safemediahandle.cs
- Matrix3DStack.cs
- ResXResourceReader.cs
- MailMessage.cs
- MethodBuilder.cs
- ConfigXmlSignificantWhitespace.cs
- IxmlLineInfo.cs
- ColumnCollection.cs
- CapabilitiesRule.cs
- PackWebRequest.cs
- FloaterBaseParagraph.cs
- HttpCacheParams.cs
- OleDbCommand.cs
- ExpressionBuilderCollection.cs
- DecimalConstantAttribute.cs
- TraceHandler.cs
- SQLString.cs
- CompilerParameters.cs
- FrameworkPropertyMetadata.cs
- XmlElementAttributes.cs
- TargetPerspective.cs
- DiagnosticTrace.cs
- Transactions.cs
- MethodBuilderInstantiation.cs
- FontUnit.cs
- NetworkInformationException.cs
- WCFServiceClientProxyGenerator.cs
- AuthenticationService.cs
- PbrsForward.cs
- BatchParser.cs
- DynamicMethod.cs
- KeyValueConfigurationElement.cs
- ParserOptions.cs
- ActivityExecutorSurrogate.cs
- Token.cs
- ParserOptions.cs
- Point3DAnimationUsingKeyFrames.cs
- SystemTcpStatistics.cs
- GenericParameterDataContract.cs
- ZipFileInfo.cs
- AdRotator.cs
- OleDbDataReader.cs
- XmlMapping.cs
- StylusLogic.cs
- HandleCollector.cs
- TrackPointCollection.cs
- FindCompletedEventArgs.cs
- SByteConverter.cs
- WebPermission.cs
- CopyCodeAction.cs
- DialogResultConverter.cs
- DataBoundControlHelper.cs
- DataComponentMethodGenerator.cs
- DataKey.cs
- BinHexEncoding.cs
- SoapCodeExporter.cs
- Ray3DHitTestResult.cs
- InitializationEventAttribute.cs
- OdbcFactory.cs
- ScriptingRoleServiceSection.cs
- InternalConfigRoot.cs
- XmlByteStreamWriter.cs
- UnsettableComboBox.cs
- CanExecuteRoutedEventArgs.cs
- NegotiateStream.cs
- EventLog.cs
- ListViewDeleteEventArgs.cs
- DictionaryItemsCollection.cs
- TextElementEnumerator.cs
- UnmanagedHandle.cs
- DataBindingHandlerAttribute.cs
- DrawingGroup.cs
- WmpBitmapDecoder.cs
- ConsoleEntryPoint.cs
- WCFModelStrings.Designer.cs
- ErrorEventArgs.cs
- UnsafeNativeMethodsMilCoreApi.cs
- StylusButtonEventArgs.cs
- InputBuffer.cs
- Token.cs
- SynchronousChannel.cs
- CompiledQuery.cs
- MarkupWriter.cs
- ClientBuildManagerCallback.cs
- UIServiceHelper.cs
- VariableQuery.cs
- RegexCaptureCollection.cs
- AnnotationDocumentPaginator.cs
- CardSpaceException.cs
- QueryableDataSource.cs
- RenderingEventArgs.cs
- BooleanStorage.cs
- MenuItemBinding.cs
- AdapterUtil.cs
- AttributeCollection.cs
- DatasetMethodGenerator.cs
- ListViewAutomationPeer.cs