Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Permissions / IsolatedStoragePermission.cs / 1 / IsolatedStoragePermission.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Security.Permissions { using System; using System.IO; using System.Security; using System.Security.Util; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum IsolatedStorageContainment { None = 0x00, DomainIsolationByUser = 0x10, ApplicationIsolationByUser = 0x15, AssemblyIsolationByUser = 0x20, DomainIsolationByMachine = 0x30, AssemblyIsolationByMachine = 0x40, ApplicationIsolationByMachine = 0x45, DomainIsolationByRoamingUser = 0x50, AssemblyIsolationByRoamingUser = 0x60, ApplicationIsolationByRoamingUser = 0x65, AdministerIsolatedStorageByUser = 0x70, //AdministerIsolatedStorageByMachine = 0x80, UnrestrictedIsolatedStorage = 0xF0 }; [Serializable, SecurityPermissionAttribute( SecurityAction.InheritanceDemand, ControlEvidence = true, ControlPolicy = true )] [System.Runtime.InteropServices.ComVisible(true)] abstract public class IsolatedStoragePermission : CodeAccessPermission, IUnrestrictedPermission { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- ///internal long m_userQuota; /// internal long m_machineQuota; /// internal long m_expirationDays; /// internal bool m_permanentData; /// internal IsolatedStorageContainment m_allowed; //----------------------------------------------------- // // CONSTRUCTORS // //----------------------------------------------------- protected IsolatedStoragePermission(PermissionState state) { if (state == PermissionState.Unrestricted) { m_userQuota = Int64.MaxValue; m_machineQuota = Int64.MaxValue; m_expirationDays = Int64.MaxValue ; m_permanentData = true; m_allowed = IsolatedStorageContainment.UnrestrictedIsolatedStorage; } else if (state == PermissionState.None) { m_userQuota = 0; m_machineQuota = 0; m_expirationDays = 0; m_permanentData = false; m_allowed = IsolatedStorageContainment.None; } else { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidPermissionState")); } } internal IsolatedStoragePermission(IsolatedStorageContainment UsageAllowed, long ExpirationDays, bool PermanentData) { m_userQuota = 0; // typical demand won't include quota m_machineQuota = 0; // typical demand won't include quota m_expirationDays = ExpirationDays; m_permanentData = PermanentData; m_allowed = UsageAllowed; } internal IsolatedStoragePermission(IsolatedStorageContainment UsageAllowed, long ExpirationDays, bool PermanentData, long UserQuota) { m_machineQuota = 0; m_userQuota = UserQuota; m_expirationDays = ExpirationDays; m_permanentData = PermanentData; m_allowed = UsageAllowed; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- // properties public long UserQuota { set{ m_userQuota = value; } get{ return m_userQuota; } } #if false internal long MachineQuota { set{ m_machineQuota = value; } get{ return m_machineQuota; } } internal long ExpirationDays { set{ m_expirationDays = value; } get{ return m_expirationDays; } } internal bool PermanentData { set{ m_permanentData = value; } get{ return m_permanentData; } } #endif public IsolatedStorageContainment UsageAllowed { set{ m_allowed = value; } get{ return m_allowed; } } //------------------------------------------------------ // // CODEACCESSPERMISSION IMPLEMENTATION // //------------------------------------------------------ public bool IsUnrestricted() { return m_allowed == IsolatedStorageContainment.UnrestrictedIsolatedStorage; } //----------------------------------------------------- // // INTERNAL METHODS // //------------------------------------------------------ internal static long min(long x,long y) {return x>y?y:x;} internal static long max(long x,long y) {return x 0) { esd.AddAttribute(_strUserQuota, (m_userQuota).ToString(CultureInfo.InvariantCulture)) ; } if (m_machineQuota>0) { esd.AddAttribute(_strMachineQuota, (m_machineQuota).ToString(CultureInfo.InvariantCulture)) ; } if (m_expirationDays>0) { esd.AddAttribute( _strExpiry, (m_expirationDays).ToString(CultureInfo.InvariantCulture)) ; } if (m_permanentData) { esd.AddAttribute(_strPermDat, (m_permanentData).ToString()) ; } } else { esd.AddAttribute( "Unrestricted", "true" ); } return esd; } public override void FromXml(SecurityElement esd) { CodeAccessPermission.ValidateElement( esd, this ); m_allowed = IsolatedStorageContainment.None; // default if no match if (XMLUtil.IsUnrestricted(esd)) { m_allowed = IsolatedStorageContainment.UnrestrictedIsolatedStorage; } else { String allowed = esd.Attribute( "Allowed" ); if (allowed != null) m_allowed = (IsolatedStorageContainment)Enum.Parse( typeof( IsolatedStorageContainment ), allowed ); } if (m_allowed == IsolatedStorageContainment.UnrestrictedIsolatedStorage) { m_userQuota = Int64.MaxValue; m_machineQuota = Int64.MaxValue; m_expirationDays = Int64.MaxValue ; m_permanentData = true; } else { String param; param = esd.Attribute (_strUserQuota) ; m_userQuota = param != null ? Int64.Parse(param, CultureInfo.InvariantCulture) : 0 ; param = esd.Attribute (_strMachineQuota) ; m_machineQuota = param != null ? Int64.Parse(param, CultureInfo.InvariantCulture) : 0 ; param = esd.Attribute (_strExpiry) ; m_expirationDays = param != null ? Int64.Parse(param, CultureInfo.InvariantCulture) : 0 ; param = esd.Attribute (_strPermDat) ; m_permanentData = param != null ? (Boolean.Parse(param)) : false ; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
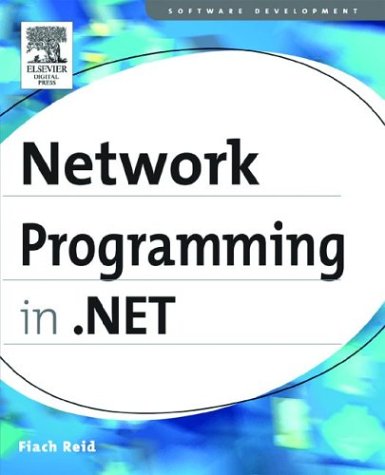
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationAttributes.cs
- ReadOnlyHierarchicalDataSourceView.cs
- AnnotationService.cs
- BooleanConverter.cs
- InputBuffer.cs
- PackWebResponse.cs
- VoiceInfo.cs
- DesignerCommandAdapter.cs
- SQLConvert.cs
- _LoggingObject.cs
- SamlSecurityTokenAuthenticator.cs
- XmlSchemaException.cs
- SwitchLevelAttribute.cs
- LoginName.cs
- FlowDocument.cs
- LocalValueEnumerator.cs
- GroupQuery.cs
- TabletDeviceInfo.cs
- EpmContentDeSerializerBase.cs
- BulletedListEventArgs.cs
- PreviewPrintController.cs
- Crypto.cs
- DBCommand.cs
- HuffCodec.cs
- SmtpFailedRecipientException.cs
- XmlSchemaChoice.cs
- StyleXamlTreeBuilder.cs
- QueryRewriter.cs
- RouteParser.cs
- VersionedStreamOwner.cs
- CatalogPart.cs
- autovalidator.cs
- StringArrayConverter.cs
- MimeMapping.cs
- basenumberconverter.cs
- CaseStatementProjectedSlot.cs
- GeneralTransform2DTo3DTo2D.cs
- TransactionState.cs
- Serializer.cs
- TableCellsCollectionEditor.cs
- initElementDictionary.cs
- LayoutEvent.cs
- ParenthesizePropertyNameAttribute.cs
- hresults.cs
- FacetChecker.cs
- XmlSchemaCompilationSettings.cs
- ConfigurationLocation.cs
- TTSEngineProxy.cs
- MetaType.cs
- Matrix3DValueSerializer.cs
- TypeForwardedToAttribute.cs
- SafeMILHandleMemoryPressure.cs
- Claim.cs
- TemplateApplicationHelper.cs
- LicFileLicenseProvider.cs
- Descriptor.cs
- DetailsViewRow.cs
- ButtonFieldBase.cs
- FileDetails.cs
- _UriTypeConverter.cs
- ProjectionQueryOptionExpression.cs
- ColumnHeader.cs
- TextOutput.cs
- DynamicMethod.cs
- Int16Animation.cs
- ExecutorLocksHeldException.cs
- BitmapDecoder.cs
- FileUpload.cs
- ViewLoader.cs
- CompilerError.cs
- HierarchicalDataBoundControlAdapter.cs
- ControlBuilderAttribute.cs
- SyndicationDeserializer.cs
- WindowsGrip.cs
- CountAggregationOperator.cs
- LayoutEvent.cs
- ConfigXmlWhitespace.cs
- PageBreakRecord.cs
- SecurityProtocolCorrelationState.cs
- AuthorizationSection.cs
- BoolExpr.cs
- StyleModeStack.cs
- Frame.cs
- RichTextBoxAutomationPeer.cs
- HTMLTextWriter.cs
- AvTraceFormat.cs
- SQLInt32.cs
- SQLUtility.cs
- Token.cs
- UnsafeNetInfoNativeMethods.cs
- FontStretchConverter.cs
- CorrelationManager.cs
- StoryFragments.cs
- HttpApplication.cs
- QilExpression.cs
- Ray3DHitTestResult.cs
- Input.cs
- QueryTask.cs
- Image.cs
- ChannelManager.cs