Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / PaintEvent.cs / 1305376 / PaintEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using System.Runtime.InteropServices; ////// /// // NOTE: Please keep this class consistent with PrintPageEventArgs. public class PaintEventArgs : EventArgs, IDisposable { ////// Provides data for the ////// event. /// /// Graphics object with which painting should be done. /// private Graphics graphics = null; // See ResetGraphics() private GraphicsState savedGraphicsState = null; ////// DC (Display context) for obtaining the graphics object. Used to delay getting the graphics /// object until absolutely necessary (for perf reasons) /// private readonly IntPtr dc = IntPtr.Zero; IntPtr oldPal = IntPtr.Zero; ////// Rectangle into which all painting should be done. /// private readonly Rectangle clipRect; //private Control paletteSource; #if DEBUG static readonly TraceSwitch PaintEventFinalizationSwitch = new TraceSwitch("PaintEventFinalization", "Tracks the creation and finalization of PaintEvent objects"); internal static string GetAllocationStack() { if (PaintEventFinalizationSwitch.TraceVerbose) { return Environment.StackTrace; } else { return "Enabled 'PaintEventFinalization' trace switch to see stack of allocation"; } } private string AllocationSite = PaintEventArgs.GetAllocationStack(); #endif ////// /// public PaintEventArgs(Graphics graphics, Rectangle clipRect) { if( graphics == null ){ throw new ArgumentNullException("graphics"); } this.graphics = graphics; this.clipRect = clipRect; } // Internal version of constructor for performance // We try to avoid getting the graphics object until needed internal PaintEventArgs(IntPtr dc, Rectangle clipRect) { Debug.Assert(dc != IntPtr.Zero, "dc is not initialized."); this.dc = dc; this.clipRect = clipRect; } ////// Initializes a new instance of the ///class with the specified graphics and /// clipping rectangle. /// ~PaintEventArgs() { Dispose(false); } /// /// /// public Rectangle ClipRectangle { get { return clipRect; } } ////// Gets the /// rectangle in which to paint. /// ////// Gets the HDC this paint event is connected to. If there is no associated /// HDC, or the GDI+ Graphics object has been created (meaning GDI+ now owns the /// HDC), 0 is returned. /// /// internal IntPtr HDC { get { if (graphics == null) return dc; else return IntPtr.Zero; } } ////// /// public System.Drawing.Graphics Graphics { get { if (graphics == null && dc != IntPtr.Zero) { oldPal = Control.SetUpPalette(dc, false /*force*/, false /*realize*/); graphics = Graphics.FromHdcInternal(dc); graphics.PageUnit = GraphicsUnit.Pixel; savedGraphicsState = graphics.Save(); // See ResetGraphics() below } return graphics; } } // We want a way to dispose the GDI+ Graphics, but we don't want to create one // simply to dispose it // cpr: should be internal ////// Gets the ////// object used to /// paint. /// /// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes /// of the resources (other than memory) used by the ///. /// protected virtual void Dispose(bool disposing) { #if DEBUG Debug.Assert(disposing, "PaintEvent object should be explicitly disposed. Potential GDI multithreading lock issue. Allocation stack:\r\n" + AllocationSite); #endif if (disposing) { //only dispose the graphics object if we created it via the dc. if (graphics != null && dc != IntPtr.Zero) { graphics.Dispose(); } } if (oldPal != IntPtr.Zero && dc != IntPtr.Zero) { SafeNativeMethods.SelectPalette(new HandleRef(this, dc), new HandleRef(this, oldPal), 0); oldPal = IntPtr.Zero; } } // If ControlStyles.AllPaintingInWmPaint, we call this method // after OnPaintBackground so it appears to OnPaint that it's getting a fresh // Graphics. We want to make sure AllPaintingInWmPaint is purely an optimization, // and doesn't change behavior, so we need to make sure any clipping regions established // in OnPaintBackground don't apply to OnPaint. See ASURT 44682. internal void ResetGraphics() { if (graphics != null) { Debug.Assert(dc == IntPtr.Zero || savedGraphicsState != null, "Called ResetGraphics more than once?"); if (savedGraphicsState != null) { graphics.Restore(savedGraphicsState); savedGraphicsState = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
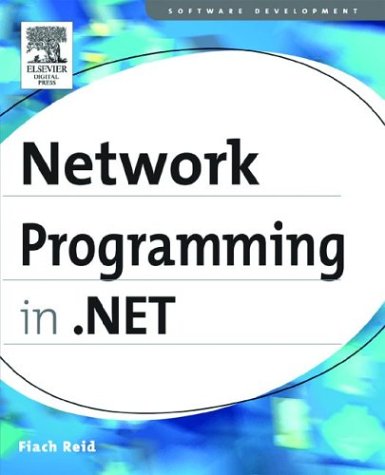
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HebrewNumber.cs
- CheckBoxPopupAdapter.cs
- AnimationException.cs
- LocalizabilityAttribute.cs
- shaperfactory.cs
- InitializingNewItemEventArgs.cs
- FocusChangedEventArgs.cs
- SBCSCodePageEncoding.cs
- X509Certificate2Collection.cs
- ExecutionEngineException.cs
- KeyMatchBuilder.cs
- RawKeyboardInputReport.cs
- RayHitTestParameters.cs
- PenCursorManager.cs
- FixedFlowMap.cs
- AuthenticationService.cs
- VerificationException.cs
- OleDbErrorCollection.cs
- SecurityDescriptor.cs
- TextAutomationPeer.cs
- XmlEventCache.cs
- ConstrainedDataObject.cs
- ProtectedConfigurationProviderCollection.cs
- HebrewNumber.cs
- Calendar.cs
- Int64Storage.cs
- ErrorHandler.cs
- IriParsingElement.cs
- CodeBlockBuilder.cs
- EntitySetBaseCollection.cs
- RegexTree.cs
- ErrorWebPart.cs
- XmlSchemaSimpleContentRestriction.cs
- ComponentSerializationService.cs
- CollectionViewGroupInternal.cs
- TypeExtensionConverter.cs
- SettingsPropertyNotFoundException.cs
- InkCanvas.cs
- ConditionedDesigner.cs
- IdentityManager.cs
- BindableTemplateBuilder.cs
- TrackingProfile.cs
- DrawListViewItemEventArgs.cs
- NonDualMessageSecurityOverHttp.cs
- DataGridViewRowPrePaintEventArgs.cs
- RuntimeResourceSet.cs
- MeasureData.cs
- ToolStripSeparator.cs
- Tablet.cs
- OracleException.cs
- HttpResponse.cs
- SafeRightsManagementHandle.cs
- Convert.cs
- DataGridViewCellLinkedList.cs
- RawStylusInputReport.cs
- ScaleTransform3D.cs
- AnimationClockResource.cs
- TimeoutConverter.cs
- WebPartManagerDesigner.cs
- EventPrivateKey.cs
- TreeSet.cs
- ChunkedMemoryStream.cs
- ConfigurationSection.cs
- Variable.cs
- Module.cs
- httpserverutility.cs
- ExitEventArgs.cs
- CaretElement.cs
- DataGrid.cs
- DataGridLinkButton.cs
- CompatibleIComparer.cs
- EndpointAddressProcessor.cs
- ScaleTransform3D.cs
- IInstanceTable.cs
- wgx_exports.cs
- MemberMemberBinding.cs
- StylusShape.cs
- SerializableTypeCodeDomSerializer.cs
- ZeroOpNode.cs
- GridView.cs
- RotateTransform.cs
- BaseParser.cs
- AccessedThroughPropertyAttribute.cs
- HTMLTagNameToTypeMapper.cs
- SizeF.cs
- XmlLangPropertyAttribute.cs
- HtmlControlPersistable.cs
- SoapRpcMethodAttribute.cs
- InheritanceContextHelper.cs
- ErrorTableItemStyle.cs
- SelectionItemPattern.cs
- XmlAttribute.cs
- DoWorkEventArgs.cs
- ChannelServices.cs
- TrustLevel.cs
- EventTrigger.cs
- M3DUtil.cs
- ScriptIgnoreAttribute.cs
- DataViewSetting.cs
- XhtmlMobileTextWriter.cs