Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / PropertyConverter.cs / 1 / PropertyConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public static class PropertyConverter { private static readonly Type[] s_parseMethodTypes = new Type[] { typeof(string) }; private static readonly Type[] s_parseMethodTypesWithSOP = new Type[] { typeof(string), typeof(IServiceProvider) }; /* * Contains helpers to convert properties from strings to their types and vice versa. */ /* * Converts a persisted enumeration value into its numeric value. * Hyphen characters in the persisted format are converted to underscores. */ ////// public static object EnumFromString(Type enumType, string value) { try { return Enum.Parse(enumType, value, true); } catch { return null; } } /* * Converts a numeric enumerated value into its persisted form, which is the * code name with underscores replaced by hyphens. */ ////// public static string EnumToString(Type enumType, object enumValue) { string value = Enum.Format(enumType, enumValue, "G"); // return value.Replace('_','-'); } /* * Converts the persisted string into an object using the object's * FromString method. */ ////// public static object ObjectFromString(Type objType, MemberInfo propertyInfo, string value) { if (value == null) return null; // Blank valued bools don't map with FromString. Return null to allow // caller to interpret. if (objType.Equals(typeof(bool)) && value.Length == 0) { return null; } bool useParseMethod = true; object ret = null; try { if (objType.IsEnum) { useParseMethod = false; ret = EnumFromString(objType, value); } else if (objType.Equals(typeof(string))) { useParseMethod = false; ret = value; } else { PropertyDescriptor pd = null; if (propertyInfo != null) { pd = TypeDescriptor.GetProperties(propertyInfo.ReflectedType)[propertyInfo.Name]; } if (pd != null) { TypeConverter converter = pd.Converter; if (converter != null && converter.CanConvertFrom(typeof(string))) { useParseMethod = false; ret = converter.ConvertFromInvariantString(value); } } } } catch { } if (useParseMethod) { // resort to Parse static method on the type // First try Parse(string, IServiceProvider); MethodInfo methodInfo = objType.GetMethod("Parse", s_parseMethodTypesWithSOP); if (methodInfo != null) { object[] parameters = new object[2]; parameters[0] = value; parameters[1] = CultureInfo.InvariantCulture; try { ret = Util.InvokeMethod(methodInfo, null, parameters); } catch { } } else { // Try the simpler: Parse(string); methodInfo = objType.GetMethod("Parse", s_parseMethodTypes); if (methodInfo != null) { object[] parameters = new object[1]; parameters[0] = value; try { ret = Util.InvokeMethod(methodInfo, null, parameters); } catch { } } } } if (ret == null) { // Unhandled... throw an exception, so user sees an error at parse time // Note that we don't propagate inner exceptions here, since they usually // do not give any information about where the bad value existed on // the object being initialized, whereas, our exception gives that // information. throw new HttpException(SR.GetString(SR.Type_not_creatable_from_string, objType.FullName, value, propertyInfo.Name)); } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public static class PropertyConverter { private static readonly Type[] s_parseMethodTypes = new Type[] { typeof(string) }; private static readonly Type[] s_parseMethodTypesWithSOP = new Type[] { typeof(string), typeof(IServiceProvider) }; /* * Contains helpers to convert properties from strings to their types and vice versa. */ /* * Converts a persisted enumeration value into its numeric value. * Hyphen characters in the persisted format are converted to underscores. */ ////// public static object EnumFromString(Type enumType, string value) { try { return Enum.Parse(enumType, value, true); } catch { return null; } } /* * Converts a numeric enumerated value into its persisted form, which is the * code name with underscores replaced by hyphens. */ ////// public static string EnumToString(Type enumType, object enumValue) { string value = Enum.Format(enumType, enumValue, "G"); // return value.Replace('_','-'); } /* * Converts the persisted string into an object using the object's * FromString method. */ ////// public static object ObjectFromString(Type objType, MemberInfo propertyInfo, string value) { if (value == null) return null; // Blank valued bools don't map with FromString. Return null to allow // caller to interpret. if (objType.Equals(typeof(bool)) && value.Length == 0) { return null; } bool useParseMethod = true; object ret = null; try { if (objType.IsEnum) { useParseMethod = false; ret = EnumFromString(objType, value); } else if (objType.Equals(typeof(string))) { useParseMethod = false; ret = value; } else { PropertyDescriptor pd = null; if (propertyInfo != null) { pd = TypeDescriptor.GetProperties(propertyInfo.ReflectedType)[propertyInfo.Name]; } if (pd != null) { TypeConverter converter = pd.Converter; if (converter != null && converter.CanConvertFrom(typeof(string))) { useParseMethod = false; ret = converter.ConvertFromInvariantString(value); } } } } catch { } if (useParseMethod) { // resort to Parse static method on the type // First try Parse(string, IServiceProvider); MethodInfo methodInfo = objType.GetMethod("Parse", s_parseMethodTypesWithSOP); if (methodInfo != null) { object[] parameters = new object[2]; parameters[0] = value; parameters[1] = CultureInfo.InvariantCulture; try { ret = Util.InvokeMethod(methodInfo, null, parameters); } catch { } } else { // Try the simpler: Parse(string); methodInfo = objType.GetMethod("Parse", s_parseMethodTypes); if (methodInfo != null) { object[] parameters = new object[1]; parameters[0] = value; try { ret = Util.InvokeMethod(methodInfo, null, parameters); } catch { } } } } if (ret == null) { // Unhandled... throw an exception, so user sees an error at parse time // Note that we don't propagate inner exceptions here, since they usually // do not give any information about where the bad value existed on // the object being initialized, whereas, our exception gives that // information. throw new HttpException(SR.GetString(SR.Type_not_creatable_from_string, objType.FullName, value, propertyInfo.Name)); } return ret; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
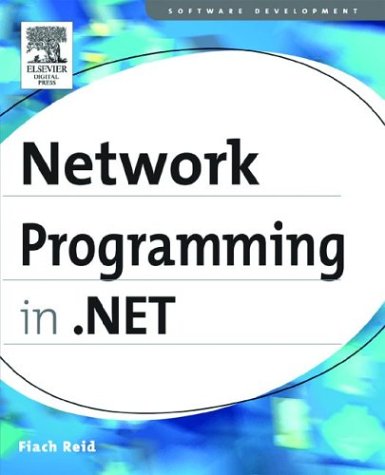
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartChrome.cs
- TransformationRules.cs
- SoapExtensionReflector.cs
- PathFigureCollection.cs
- WebPartConnection.cs
- ResetableIterator.cs
- UIElementCollection.cs
- SerializationObjectManager.cs
- Header.cs
- BitmapSource.cs
- HttpCookie.cs
- GroupQuery.cs
- SQLStringStorage.cs
- FieldAccessException.cs
- Table.cs
- SQLDecimal.cs
- CodeTryCatchFinallyStatement.cs
- util.cs
- BindingGroup.cs
- Permission.cs
- ExtenderControl.cs
- SwitchLevelAttribute.cs
- PersistNameAttribute.cs
- ListView.cs
- MarkupCompilePass2.cs
- TransformedBitmap.cs
- FactoryMaker.cs
- WindowsScroll.cs
- MenuAutomationPeer.cs
- ToolTipService.cs
- TypeConverterHelper.cs
- ArgIterator.cs
- ModifyActivitiesPropertyDescriptor.cs
- DesignerForm.cs
- ParagraphResult.cs
- Dynamic.cs
- DocumentPageViewAutomationPeer.cs
- CapabilitiesRule.cs
- XmlSerializerImportOptions.cs
- ClientSettingsSection.cs
- EntityDataSourceWizardForm.cs
- ManipulationDelta.cs
- ITextView.cs
- RestHandler.cs
- GridViewCellAutomationPeer.cs
- SpecialFolderEnumConverter.cs
- ThreadExceptionDialog.cs
- ColorConvertedBitmapExtension.cs
- DefaultTextStoreTextComposition.cs
- InvokeBinder.cs
- WebBrowserPermission.cs
- WebPartVerb.cs
- ProcessThread.cs
- Message.cs
- ItemCollection.cs
- DataGridViewRowHeaderCell.cs
- XmlSchemaExternal.cs
- MenuEventArgs.cs
- ConstructorBuilder.cs
- RightsManagementInformation.cs
- OdbcStatementHandle.cs
- HttpResponse.cs
- TextDecorationCollectionConverter.cs
- FilterUserControlBase.cs
- DataException.cs
- CallContext.cs
- TimerTable.cs
- Block.cs
- Attributes.cs
- RenderDataDrawingContext.cs
- ContainerActivationHelper.cs
- ListenerSessionConnection.cs
- MultilineStringConverter.cs
- x509utils.cs
- SqlDataSource.cs
- UIElement3D.cs
- NativeMethods.cs
- ComponentManagerBroker.cs
- DataRelationPropertyDescriptor.cs
- ChildTable.cs
- Helper.cs
- FormViewCommandEventArgs.cs
- AdCreatedEventArgs.cs
- MobileControlsSectionHelper.cs
- AutomationPropertyInfo.cs
- TableCell.cs
- DbUpdateCommandTree.cs
- ScriptModule.cs
- PropertyIDSet.cs
- SafePointer.cs
- SqlStatistics.cs
- TaskFormBase.cs
- GeneralTransformGroup.cs
- XPathDocumentNavigator.cs
- CriticalExceptions.cs
- QueryFunctions.cs
- loginstatus.cs
- TextCompositionEventArgs.cs
- XhtmlConformanceSection.cs
- Panel.cs