Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / SevenBitStream.cs / 1305376 / SevenBitStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mime { using System; using System.IO; using System.Text; ////// This stream validates outgoing bytes to be within the /// acceptible range of 0 - 127. Writes will throw if a /// value > 127 is found. /// internal class SevenBitStream : DelegatedStream, IEncodableStream { ////// ctor. /// /// Underlying stream internal SevenBitStream(Stream stream) : base(stream) { } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write /// Callback to call when write completes /// State to pass to callback public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); IAsyncResult result = base.BeginWrite(buffer, offset, count, callback, state); return result; } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write public override void Write(byte[] buffer, int offset, int count) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); base.Write(buffer, offset, count); } // helper methods ////// Checks the data in the buffer for bytes > 127. /// /// Buffer containing data /// Offset within buffer to start checking /// Count of bytes to check void CheckBytes(byte[] buffer, int offset, int count) { for (int i = count; i < offset + count; i++) { if (buffer[i] > 127) throw new FormatException(SR.GetString(SR.Mail7BitStreamInvalidCharacter)); } } public int DecodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } //nothing to "encode" here so all we're actually doing is folding public int EncodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } public Stream GetStream() { return this; } public string GetEncodedString() { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
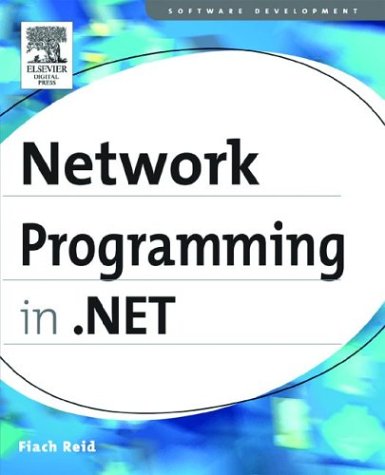
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymLanguageVendor.cs
- PolyBezierSegmentFigureLogic.cs
- FixedHyperLink.cs
- NestPullup.cs
- BooleanFunctions.cs
- MailMessageEventArgs.cs
- BulletChrome.cs
- StreamingContext.cs
- _LoggingObject.cs
- ProcessThread.cs
- UrlAuthFailedErrorFormatter.cs
- CriticalHandle.cs
- FlagsAttribute.cs
- Expression.cs
- DeploymentExceptionMapper.cs
- CommonDialog.cs
- InitializerFacet.cs
- ZeroOpNode.cs
- TableAdapterManagerHelper.cs
- DataSysAttribute.cs
- CatalogPart.cs
- LinkedResourceCollection.cs
- BasicCellRelation.cs
- BorderGapMaskConverter.cs
- DataGridRelationshipRow.cs
- ObjectSecurity.cs
- Cell.cs
- RoutedPropertyChangedEventArgs.cs
- VersionValidator.cs
- DiscoveryReferences.cs
- InputLanguageEventArgs.cs
- DataGridViewComboBoxCell.cs
- AppDomainProtocolHandler.cs
- AsyncOperationManager.cs
- ContourSegment.cs
- DataGridItemEventArgs.cs
- CellParaClient.cs
- ExternalDataExchangeService.cs
- CodePageUtils.cs
- SecurityTokenValidationException.cs
- GPRECTF.cs
- Nodes.cs
- StreamWithDictionary.cs
- NavigatorInput.cs
- CombinedGeometry.cs
- FieldAccessException.cs
- EntityContainerEntitySet.cs
- PointLight.cs
- BindableTemplateBuilder.cs
- Context.cs
- GZipDecoder.cs
- MailDefinition.cs
- BitmapEditor.cs
- RelatedPropertyManager.cs
- BamlLocalizableResource.cs
- SqlNotificationRequest.cs
- Label.cs
- basenumberconverter.cs
- SplitterPanelDesigner.cs
- ImageButton.cs
- ObjectHandle.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- AppSecurityManager.cs
- HttpApplicationFactory.cs
- GenerateScriptTypeAttribute.cs
- KeyedPriorityQueue.cs
- updatecommandorderer.cs
- UpdateProgress.cs
- MaterializeFromAtom.cs
- NetworkInformationPermission.cs
- XamlGridLengthSerializer.cs
- XomlCompilerResults.cs
- OleDbException.cs
- DataTemplate.cs
- SectionXmlInfo.cs
- X509Extension.cs
- CodeArrayCreateExpression.cs
- XamlTreeBuilderBamlRecordWriter.cs
- MsmqQueue.cs
- ProtectedUri.cs
- Hash.cs
- CheckBoxPopupAdapter.cs
- DataGridTableCollection.cs
- FieldToken.cs
- ConfigurationValue.cs
- ClusterRegistryConfigurationProvider.cs
- PassportAuthenticationModule.cs
- DispatcherFrame.cs
- Propagator.Evaluator.cs
- ConfigurationConverterBase.cs
- UidPropertyAttribute.cs
- ResXResourceReader.cs
- ProviderSettings.cs
- WebBrowserNavigatedEventHandler.cs
- DataGridViewRow.cs
- GlobalProxySelection.cs
- PrePostDescendentsWalker.cs
- DynamicExpression.cs
- Journal.cs
- SocketInformation.cs