Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / BorderGapMaskConverter.cs / 1 / BorderGapMaskConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Used to create a Gap in the Border for GroupBox style // //--------------------------------------------------------------------------- using System.Globalization; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Shapes; namespace System.Windows.Controls { ////// BorderGapMaskConverter class /// public class BorderGapMaskConverter : IMultiValueConverter { ////// Convert a value. /// /// values as produced by source binding /// target type /// converter parameter /// culture information ////// Converted value. /// Visual Brush that is used as the opacity mask for the Border /// in the style for GroupBox. /// public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture) { // // Parameter Validation // Type doubleType = typeof(double); if (parameter == null || values == null || values.Length != 3 || values[0] == null || values[1] == null || values[2] == null || !doubleType.IsAssignableFrom(values[0].GetType()) || !doubleType.IsAssignableFrom(values[1].GetType()) || !doubleType.IsAssignableFrom(values[2].GetType()) ) { return DependencyProperty.UnsetValue; } Type paramType = parameter.GetType(); if (!(doubleType.IsAssignableFrom(paramType) || typeof(string).IsAssignableFrom(paramType))) { return DependencyProperty.UnsetValue; } // // Conversion // double headerWidth = (double)values[0]; double borderWidth = (double)values[1]; double borderHeight = (double)values[2]; // Doesn't make sense to have a Grid // with 0 as width or height if (borderWidth == 0 || borderHeight == 0) { return null; } // Width of the line to the left of the header // to be used to set the width of the first column of the Grid double lineWidth; if (parameter is string) { lineWidth = Double.Parse(((string)parameter), NumberFormatInfo.InvariantInfo); } else { lineWidth = (double)parameter; } Grid grid = new Grid(); grid.Width = borderWidth; grid.Height = borderHeight; ColumnDefinition colDef1 = new ColumnDefinition(); ColumnDefinition colDef2 = new ColumnDefinition(); ColumnDefinition colDef3 = new ColumnDefinition(); colDef1.Width = new GridLength(lineWidth); colDef2.Width = new GridLength(headerWidth); colDef3.Width = new GridLength(1, GridUnitType.Star); grid.ColumnDefinitions.Add(colDef1); grid.ColumnDefinitions.Add(colDef2); grid.ColumnDefinitions.Add(colDef3); RowDefinition rowDef1 = new RowDefinition(); RowDefinition rowDef2 = new RowDefinition(); rowDef1.Height = new GridLength(borderHeight / 2); rowDef2.Height = new GridLength(1, GridUnitType.Star); grid.RowDefinitions.Add(rowDef1); grid.RowDefinitions.Add(rowDef2); Rectangle rectColumn1 = new Rectangle(); Rectangle rectColumn2 = new Rectangle(); Rectangle rectColumn3 = new Rectangle(); rectColumn1.Fill = Brushes.Black; rectColumn2.Fill = Brushes.Black; rectColumn3.Fill = Brushes.Black; Grid.SetRowSpan(rectColumn1, 2); Grid.SetRow(rectColumn1, 0); Grid.SetColumn(rectColumn1, 0); Grid.SetRow(rectColumn2, 1); Grid.SetColumn(rectColumn2, 1); Grid.SetRowSpan(rectColumn3, 2); Grid.SetRow(rectColumn3, 0); Grid.SetColumn(rectColumn3, 2); grid.Children.Add(rectColumn1); grid.Children.Add(rectColumn2); grid.Children.Add(rectColumn3); return (new VisualBrush(grid)); } ////// Not Supported /// /// value, as produced by target /// target types /// converter parameter /// culture information ///Nothing public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture) { return new object[] { Binding.DoNothing }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Used to create a Gap in the Border for GroupBox style // //--------------------------------------------------------------------------- using System.Globalization; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Shapes; namespace System.Windows.Controls { ////// BorderGapMaskConverter class /// public class BorderGapMaskConverter : IMultiValueConverter { ////// Convert a value. /// /// values as produced by source binding /// target type /// converter parameter /// culture information ////// Converted value. /// Visual Brush that is used as the opacity mask for the Border /// in the style for GroupBox. /// public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture) { // // Parameter Validation // Type doubleType = typeof(double); if (parameter == null || values == null || values.Length != 3 || values[0] == null || values[1] == null || values[2] == null || !doubleType.IsAssignableFrom(values[0].GetType()) || !doubleType.IsAssignableFrom(values[1].GetType()) || !doubleType.IsAssignableFrom(values[2].GetType()) ) { return DependencyProperty.UnsetValue; } Type paramType = parameter.GetType(); if (!(doubleType.IsAssignableFrom(paramType) || typeof(string).IsAssignableFrom(paramType))) { return DependencyProperty.UnsetValue; } // // Conversion // double headerWidth = (double)values[0]; double borderWidth = (double)values[1]; double borderHeight = (double)values[2]; // Doesn't make sense to have a Grid // with 0 as width or height if (borderWidth == 0 || borderHeight == 0) { return null; } // Width of the line to the left of the header // to be used to set the width of the first column of the Grid double lineWidth; if (parameter is string) { lineWidth = Double.Parse(((string)parameter), NumberFormatInfo.InvariantInfo); } else { lineWidth = (double)parameter; } Grid grid = new Grid(); grid.Width = borderWidth; grid.Height = borderHeight; ColumnDefinition colDef1 = new ColumnDefinition(); ColumnDefinition colDef2 = new ColumnDefinition(); ColumnDefinition colDef3 = new ColumnDefinition(); colDef1.Width = new GridLength(lineWidth); colDef2.Width = new GridLength(headerWidth); colDef3.Width = new GridLength(1, GridUnitType.Star); grid.ColumnDefinitions.Add(colDef1); grid.ColumnDefinitions.Add(colDef2); grid.ColumnDefinitions.Add(colDef3); RowDefinition rowDef1 = new RowDefinition(); RowDefinition rowDef2 = new RowDefinition(); rowDef1.Height = new GridLength(borderHeight / 2); rowDef2.Height = new GridLength(1, GridUnitType.Star); grid.RowDefinitions.Add(rowDef1); grid.RowDefinitions.Add(rowDef2); Rectangle rectColumn1 = new Rectangle(); Rectangle rectColumn2 = new Rectangle(); Rectangle rectColumn3 = new Rectangle(); rectColumn1.Fill = Brushes.Black; rectColumn2.Fill = Brushes.Black; rectColumn3.Fill = Brushes.Black; Grid.SetRowSpan(rectColumn1, 2); Grid.SetRow(rectColumn1, 0); Grid.SetColumn(rectColumn1, 0); Grid.SetRow(rectColumn2, 1); Grid.SetColumn(rectColumn2, 1); Grid.SetRowSpan(rectColumn3, 2); Grid.SetRow(rectColumn3, 0); Grid.SetColumn(rectColumn3, 2); grid.Children.Add(rectColumn1); grid.Children.Add(rectColumn2); grid.Children.Add(rectColumn3); return (new VisualBrush(grid)); } ////// Not Supported /// /// value, as produced by target /// target types /// converter parameter /// culture information ///Nothing public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture) { return new object[] { Binding.DoNothing }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
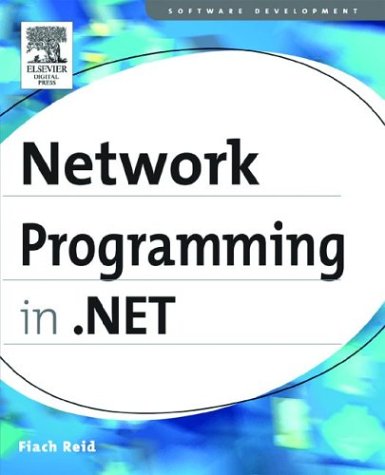
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeIndexerExpression.cs
- ListControlDataBindingHandler.cs
- XmlHelper.cs
- BindingCompleteEventArgs.cs
- TcpClientSocketManager.cs
- TerminatorSinks.cs
- DesignerVerb.cs
- SqlFacetAttribute.cs
- ExpressionBuilder.cs
- TemplatedAdorner.cs
- PasswordRecovery.cs
- DSASignatureFormatter.cs
- BlurEffect.cs
- PasswordBoxAutomationPeer.cs
- EntityContainerEmitter.cs
- ReadOnlyAttribute.cs
- DataGridViewRowsAddedEventArgs.cs
- HighlightVisual.cs
- ClientScriptManagerWrapper.cs
- ForEachAction.cs
- BaseTemplateBuildProvider.cs
- XmlSchemaSimpleContentExtension.cs
- SecurityTokenInclusionMode.cs
- TextTreeRootNode.cs
- LinqDataView.cs
- CrossAppDomainChannel.cs
- XmlSchema.cs
- EventMap.cs
- KeyGestureValueSerializer.cs
- TextServicesCompartmentContext.cs
- SchemaTypeEmitter.cs
- CounterNameConverter.cs
- TaskDesigner.cs
- OutputCacheSettingsSection.cs
- altserialization.cs
- WebBrowserPermission.cs
- LiteralText.cs
- HandlerFactoryWrapper.cs
- TypeConverterHelper.cs
- _WinHttpWebProxyDataBuilder.cs
- HttpRuntime.cs
- IsolatedStorageFile.cs
- LinkClickEvent.cs
- SessionSwitchEventArgs.cs
- DelegatingHeader.cs
- RepeaterItem.cs
- ServiceModelEnumValidatorAttribute.cs
- MailMessage.cs
- DataRecordInternal.cs
- XmlMembersMapping.cs
- KeyBinding.cs
- SmtpTransport.cs
- EventLogQuery.cs
- PrivilegedConfigurationManager.cs
- Set.cs
- RuntimeWrappedException.cs
- Hashtable.cs
- CheckBoxList.cs
- DbConnectionPoolGroup.cs
- TextElementEnumerator.cs
- AdRotator.cs
- CompiledIdentityConstraint.cs
- DataGridViewRowConverter.cs
- SubtreeProcessor.cs
- DisplayInformation.cs
- BrowserDefinition.cs
- EditorPartCollection.cs
- OlePropertyStructs.cs
- CompositeTypefaceMetrics.cs
- InvalidComObjectException.cs
- IgnoreDeviceFilterElement.cs
- GridLengthConverter.cs
- IssuedTokenServiceCredential.cs
- ExistsInCollection.cs
- webeventbuffer.cs
- COM2PictureConverter.cs
- CodeLinePragma.cs
- xml.cs
- XmlSchemaExporter.cs
- MsmqBindingElementBase.cs
- CleanUpVirtualizedItemEventArgs.cs
- FileDialog_Vista_Interop.cs
- RuntimeVariablesExpression.cs
- QuadraticBezierSegment.cs
- Geometry3D.cs
- SmiConnection.cs
- SerializationHelper.cs
- XmlImplementation.cs
- ICspAsymmetricAlgorithm.cs
- TransformerInfoCollection.cs
- AttributeQuery.cs
- UndirectedGraph.cs
- FunctionDescription.cs
- DbInsertCommandTree.cs
- Transaction.cs
- SetIndexBinder.cs
- SystemUdpStatistics.cs
- PropertyChangeTracker.cs
- DocumentXmlWriter.cs
- RefreshPropertiesAttribute.cs