Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Compilation / ExpressionBuilder.cs / 1 / ExpressionBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.ComponentModel.Design; using System.Web.Configuration; using System.Reflection; using System.Web; using System.Web.Hosting; #if !FEATURE_PAL using System.Web.UI.Design; #endif // !FEATURE_PAL using System.Web.UI; using System.Web.Util; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class ExpressionBuilder { private static ExpressionBuilder dataBindingExpressionBuilder; internal virtual void BuildExpression(BoundPropertyEntry bpe, ControlBuilder controlBuilder, CodeExpression controlReference, CodeStatementCollection methodStatements, CodeStatementCollection statements, CodeLinePragma linePragma, ref bool hasTempObject) { CodeExpression codeExpression = GetCodeExpression(bpe, bpe.ParsedExpressionData, new ExpressionBuilderContext(controlBuilder.VirtualPath)); CodeDomUtility.CreatePropertySetStatements(methodStatements, statements, controlReference, bpe.Name, bpe.Type, codeExpression, linePragma); } internal static ExpressionBuilder GetExpressionBuilder(string expressionPrefix, VirtualPath virtualPath) { return GetExpressionBuilder(expressionPrefix, virtualPath, null); } internal static ExpressionBuilder GetExpressionBuilder(string expressionPrefix, VirtualPath virtualPath, IDesignerHost host) { // If there is no expressionPrefix, it's a v1 style databinding expression if (expressionPrefix.Length == 0) { if (dataBindingExpressionBuilder == null) { dataBindingExpressionBuilder = new DataBindingExpressionBuilder(); } return dataBindingExpressionBuilder; } CompilationSection config = null; // If we are in the designer, we need to access IWebApplication config instead #if !FEATURE_PAL // FEATURE_PAL does not support designer-based features if (host != null) { IWebApplication webapp = (IWebApplication)host.GetService(typeof(IWebApplication)); if (webapp != null) { config = webapp.OpenWebConfiguration(true).GetSection("system.web/compilation") as CompilationSection; } } #endif // !FEATURE_PAL // If we failed to get config from the designer, fall back on runtime config always if (config == null) { config = RuntimeConfig.GetConfig(virtualPath).Compilation; } System.Web.Configuration.ExpressionBuilder builder = config.ExpressionBuilders[expressionPrefix]; if (builder == null) { throw new HttpParseException(SR.GetString(SR.InvalidExpressionPrefix, expressionPrefix)); } Type expressionBuilderType = null; if (host != null) { // If we are in the designer, we have to use the type resolution service ITypeResolutionService ts = (ITypeResolutionService)host.GetService(typeof(ITypeResolutionService)); if (ts != null) { expressionBuilderType = ts.GetType(builder.Type); } } if (expressionBuilderType == null) { expressionBuilderType = builder.TypeInternal; } Debug.Assert(expressionBuilderType != null, "expressionBuilderType should not be null"); if (!typeof(ExpressionBuilder).IsAssignableFrom(expressionBuilderType)) { throw new HttpParseException(SR.GetString(SR.ExpressionBuilder_InvalidType, expressionBuilderType.FullName)); } ExpressionBuilder expressionBuilder = (ExpressionBuilder)HttpRuntime.FastCreatePublicInstance(expressionBuilderType); return expressionBuilder; } // // Public API // public virtual bool SupportsEvaluate { get { return false; } } public virtual object ParseExpression(string expression, Type propertyType, ExpressionBuilderContext context) { return null; } public abstract CodeExpression GetCodeExpression(BoundPropertyEntry entry, object parsedData, ExpressionBuilderContext context); public virtual object EvaluateExpression(object target, BoundPropertyEntry entry, object parsedData, ExpressionBuilderContext context) { return null; } } }
Link Menu
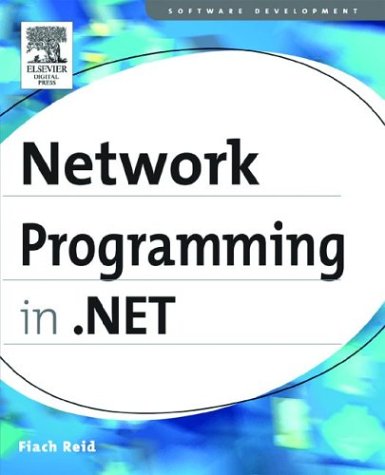
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Avt.cs
- ValueChangedEventManager.cs
- Geometry.cs
- NavigationProgressEventArgs.cs
- Aes.cs
- AssemblyAttributes.cs
- ProgressBarBrushConverter.cs
- ColorAnimationUsingKeyFrames.cs
- ReadWriteObjectLock.cs
- ListSortDescriptionCollection.cs
- CodeMethodInvokeExpression.cs
- NonClientArea.cs
- ResourceManagerWrapper.cs
- WebPartZoneBase.cs
- HttpWrapper.cs
- SystemEvents.cs
- TileBrush.cs
- LayoutManager.cs
- DoubleAnimationUsingKeyFrames.cs
- DomainConstraint.cs
- UrlPropertyAttribute.cs
- GraphicsPathIterator.cs
- CodeCommentStatement.cs
- SpecialNameAttribute.cs
- ComponentCollection.cs
- Literal.cs
- DataListGeneralPage.cs
- ValidationSummary.cs
- DictionaryEntry.cs
- XmlHierarchicalDataSourceView.cs
- UriExt.cs
- WorkflowPersistenceService.cs
- XmlSignatureManifest.cs
- XmlCharacterData.cs
- oledbmetadatacollectionnames.cs
- EndpointIdentity.cs
- DEREncoding.cs
- DataTemplateKey.cs
- BindingCompleteEventArgs.cs
- HeaderedContentControl.cs
- AssertFilter.cs
- _NegoStream.cs
- VisualProxy.cs
- EventListenerClientSide.cs
- GatewayDefinition.cs
- RegexRunnerFactory.cs
- ProxyGenerationError.cs
- FlowPosition.cs
- DecimalConverter.cs
- EntityStoreSchemaFilterEntry.cs
- MenuItemBindingCollection.cs
- BrowserDefinition.cs
- RepeaterItemCollection.cs
- NameNode.cs
- CustomCategoryAttribute.cs
- PreviewPageInfo.cs
- DesignSurfaceServiceContainer.cs
- DockEditor.cs
- Normalization.cs
- LoginView.cs
- SqlAggregateChecker.cs
- SqlCacheDependencyDatabase.cs
- SuppressMessageAttribute.cs
- MessageEnumerator.cs
- ValidationEventArgs.cs
- ConstructorArgumentAttribute.cs
- X509CertificateChain.cs
- XmlDictionary.cs
- WebPartRestoreVerb.cs
- MessageBodyDescription.cs
- ThrowHelper.cs
- ToolStripLabel.cs
- DataSourceXmlSubItemAttribute.cs
- CommandConverter.cs
- CustomPopupPlacement.cs
- TextTreeTextBlock.cs
- PhysicalAddress.cs
- ClientCultureInfo.cs
- Registry.cs
- DbParameterCollection.cs
- Accessors.cs
- TraceHandlerErrorFormatter.cs
- MouseButton.cs
- ListBindingConverter.cs
- MappingException.cs
- InvokeGenerator.cs
- AnnotationResourceCollection.cs
- TraceUtils.cs
- Inline.cs
- WizardSideBarListControlItem.cs
- HttpHandlerAction.cs
- OdbcTransaction.cs
- Pens.cs
- CfgArc.cs
- BitmapEffectDrawing.cs
- HandleRef.cs
- DataGridRowDetailsEventArgs.cs
- XmlElementCollection.cs
- NaturalLanguageHyphenator.cs
- LogSwitch.cs