Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Compilation / BaseTemplateBuildProvider.cs / 5 / BaseTemplateBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Util; using System.Web.UI; internal abstract class BaseTemplateBuildProvider: InternalBuildProvider { private TemplateParser _parser; internal TemplateParser Parser { get { return _parser; } } internal override IAssemblyDependencyParser AssemblyDependencyParser { get { return _parser; } } private string _instantiatableFullTypeName; private string _intermediateFullTypeName; protected abstract TemplateParser CreateParser(); internal abstract BaseCodeDomTreeGenerator CreateCodeDomTreeGenerator(TemplateParser parser); protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { Debug.Assert(_parser != null); // Return the provider type and compiler params Type codeDomProviderType = _parser.CompilerType.CodeDomProviderType; // Create a code generator for the language CodeDomProvider codeDomProvider = CompilationUtil.CreateCodeDomProviderNonPublic( codeDomProviderType); // Create a designer mode codedom tree for the page BaseCodeDomTreeGenerator treeGenerator = CreateCodeDomTreeGenerator(_parser); treeGenerator.SetDesignerMode(); CodeCompileUnit ccu = treeGenerator.GetCodeDomTree(codeDomProvider, new StringResourceBuilder(), VirtualPathObject); linePragmasTable = treeGenerator.LinePragmasTable; // This code is used to see the full generated code in the debugger. Just uncomment and look at // generatedCode in the debugger. Don't check in with this code uncommented! #if TESTCODE Stream stream = new MemoryStream(); StreamWriter writer = new StreamWriter(stream, System.Text.Encoding.Unicode); codeDomProvider.GenerateCodeFromCompileUnit(ccu, writer, null /*CodeGeneratorOptions*/); writer.Flush(); stream.Seek(0, SeekOrigin.Begin); TextReader reader = new StreamReader(stream); string generatedCode = reader.ReadToEnd(); #endif return ccu; } public override CompilerType CodeCompilerType { get { Debug.Assert(_parser == null); _parser = CreateParser(); if (IgnoreParseErrors) _parser.IgnoreParseErrors = true; if (IgnoreControlProperties) _parser.IgnoreControlProperties = true; if (!ThrowOnFirstParseError) _parser.ThrowOnFirstParseError = false; _parser.Parse(ReferencedAssemblies, VirtualPathObject); // If the page is non-compiled, don't ask for a language if (!Parser.RequiresCompilation) return null; return _parser.CompilerType; } } internal override ICollection GetCompileWithDependencies() { // If there is a code besides file, return it if (_parser.CodeFileVirtualPath == null) return null; // no-compile pages should not have any compile with dependencies Debug.Assert(Parser.RequiresCompilation); return new SingleObjectCollection(_parser.CodeFileVirtualPath); } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // Don't generate any code for no-compile pages if (!Parser.RequiresCompilation) return; BaseCodeDomTreeGenerator treeGenerator = CreateCodeDomTreeGenerator(_parser); CodeCompileUnit ccu = treeGenerator.GetCodeDomTree(assemblyBuilder.CodeDomProvider, assemblyBuilder.StringResourceBuilder, VirtualPathObject); if (ccu != null) { // Add all the assemblies if (_parser.AssemblyDependencies != null) { foreach (Assembly assembly in _parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly, ccu); } } assemblyBuilder.AddCodeCompileUnit(this, ccu); } // Get the name of the generated type that can be instantiated. It may be null // in updatable compilation scenarios. _instantiatableFullTypeName = treeGenerator.GetInstantiatableFullTypeName(); // tell the assembly builder to generate a fast factory for this type if (_instantiatableFullTypeName != null) assemblyBuilder.GenerateTypeFactory(_instantiatableFullTypeName); _intermediateFullTypeName = treeGenerator.GetIntermediateFullTypeName(); } public override Type GetGeneratedType(CompilerResults results) { // No Type is generated for no-compile pages if (!Parser.RequiresCompilation) return null; // Figure out the Type that needs to be persisted string typeName; if (_instantiatableFullTypeName == null) { if (Parser.CodeFileVirtualPath != null) { // Updatable precomp of a code separation page: use the intermediate type typeName = _intermediateFullTypeName; } else { // Updatable precomp of a single page: use the base type, since nothing got compiled return Parser.BaseType; } } else { typeName = _instantiatableFullTypeName; } Debug.Assert(typeName != null); Type generatedType = results.CompiledAssembly.GetType(typeName); // It should always extend the required base type Debug.Assert(Parser.BaseType.IsAssignableFrom(generatedType)); return generatedType; } internal override BuildResultCompiledType CreateBuildResult(Type t) { return new BuildResultCompiledTemplateType(t); } public override ICollection VirtualPathDependencies { get { return _parser.SourceDependencies; } } internal override ICollection GetGeneratedTypeNames() { if (_parser.GeneratedClassName == null && _parser.BaseTypeName == null) { return null; } ArrayList collection = new ArrayList(); if (_parser.GeneratedClassName != null) { collection.Add(_parser.GeneratedClassName); } if (_parser.BaseTypeName != null) { collection.Add(Util.MakeFullTypeName(_parser.BaseTypeNamespace, _parser.BaseTypeName)); } return collection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Util; using System.Web.UI; internal abstract class BaseTemplateBuildProvider: InternalBuildProvider { private TemplateParser _parser; internal TemplateParser Parser { get { return _parser; } } internal override IAssemblyDependencyParser AssemblyDependencyParser { get { return _parser; } } private string _instantiatableFullTypeName; private string _intermediateFullTypeName; protected abstract TemplateParser CreateParser(); internal abstract BaseCodeDomTreeGenerator CreateCodeDomTreeGenerator(TemplateParser parser); protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { Debug.Assert(_parser != null); // Return the provider type and compiler params Type codeDomProviderType = _parser.CompilerType.CodeDomProviderType; // Create a code generator for the language CodeDomProvider codeDomProvider = CompilationUtil.CreateCodeDomProviderNonPublic( codeDomProviderType); // Create a designer mode codedom tree for the page BaseCodeDomTreeGenerator treeGenerator = CreateCodeDomTreeGenerator(_parser); treeGenerator.SetDesignerMode(); CodeCompileUnit ccu = treeGenerator.GetCodeDomTree(codeDomProvider, new StringResourceBuilder(), VirtualPathObject); linePragmasTable = treeGenerator.LinePragmasTable; // This code is used to see the full generated code in the debugger. Just uncomment and look at // generatedCode in the debugger. Don't check in with this code uncommented! #if TESTCODE Stream stream = new MemoryStream(); StreamWriter writer = new StreamWriter(stream, System.Text.Encoding.Unicode); codeDomProvider.GenerateCodeFromCompileUnit(ccu, writer, null /*CodeGeneratorOptions*/); writer.Flush(); stream.Seek(0, SeekOrigin.Begin); TextReader reader = new StreamReader(stream); string generatedCode = reader.ReadToEnd(); #endif return ccu; } public override CompilerType CodeCompilerType { get { Debug.Assert(_parser == null); _parser = CreateParser(); if (IgnoreParseErrors) _parser.IgnoreParseErrors = true; if (IgnoreControlProperties) _parser.IgnoreControlProperties = true; if (!ThrowOnFirstParseError) _parser.ThrowOnFirstParseError = false; _parser.Parse(ReferencedAssemblies, VirtualPathObject); // If the page is non-compiled, don't ask for a language if (!Parser.RequiresCompilation) return null; return _parser.CompilerType; } } internal override ICollection GetCompileWithDependencies() { // If there is a code besides file, return it if (_parser.CodeFileVirtualPath == null) return null; // no-compile pages should not have any compile with dependencies Debug.Assert(Parser.RequiresCompilation); return new SingleObjectCollection(_parser.CodeFileVirtualPath); } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // Don't generate any code for no-compile pages if (!Parser.RequiresCompilation) return; BaseCodeDomTreeGenerator treeGenerator = CreateCodeDomTreeGenerator(_parser); CodeCompileUnit ccu = treeGenerator.GetCodeDomTree(assemblyBuilder.CodeDomProvider, assemblyBuilder.StringResourceBuilder, VirtualPathObject); if (ccu != null) { // Add all the assemblies if (_parser.AssemblyDependencies != null) { foreach (Assembly assembly in _parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly, ccu); } } assemblyBuilder.AddCodeCompileUnit(this, ccu); } // Get the name of the generated type that can be instantiated. It may be null // in updatable compilation scenarios. _instantiatableFullTypeName = treeGenerator.GetInstantiatableFullTypeName(); // tell the assembly builder to generate a fast factory for this type if (_instantiatableFullTypeName != null) assemblyBuilder.GenerateTypeFactory(_instantiatableFullTypeName); _intermediateFullTypeName = treeGenerator.GetIntermediateFullTypeName(); } public override Type GetGeneratedType(CompilerResults results) { // No Type is generated for no-compile pages if (!Parser.RequiresCompilation) return null; // Figure out the Type that needs to be persisted string typeName; if (_instantiatableFullTypeName == null) { if (Parser.CodeFileVirtualPath != null) { // Updatable precomp of a code separation page: use the intermediate type typeName = _intermediateFullTypeName; } else { // Updatable precomp of a single page: use the base type, since nothing got compiled return Parser.BaseType; } } else { typeName = _instantiatableFullTypeName; } Debug.Assert(typeName != null); Type generatedType = results.CompiledAssembly.GetType(typeName); // It should always extend the required base type Debug.Assert(Parser.BaseType.IsAssignableFrom(generatedType)); return generatedType; } internal override BuildResultCompiledType CreateBuildResult(Type t) { return new BuildResultCompiledTemplateType(t); } public override ICollection VirtualPathDependencies { get { return _parser.SourceDependencies; } } internal override ICollection GetGeneratedTypeNames() { if (_parser.GeneratedClassName == null && _parser.BaseTypeName == null) { return null; } ArrayList collection = new ArrayList(); if (_parser.GeneratedClassName != null) { collection.Add(_parser.GeneratedClassName); } if (_parser.BaseTypeName != null) { collection.Add(Util.MakeFullTypeName(_parser.BaseTypeNamespace, _parser.BaseTypeName)); } return collection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
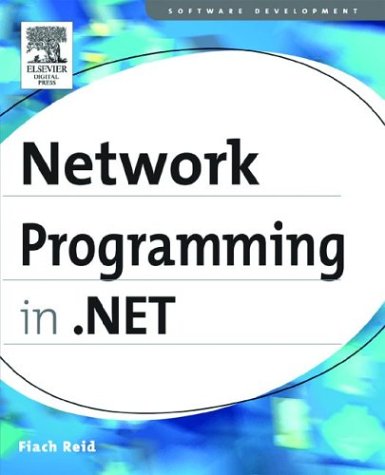
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ShutDownListener.cs
- ModuleConfigurationInfo.cs
- FixedSOMSemanticBox.cs
- DoubleUtil.cs
- StorageSetMapping.cs
- TripleDESCryptoServiceProvider.cs
- StorageRoot.cs
- DateTimeConstantAttribute.cs
- ClientFormsAuthenticationCredentials.cs
- OutputCacheModule.cs
- DBDataPermissionAttribute.cs
- Light.cs
- InternalBase.cs
- BuildDependencySet.cs
- WhileDesigner.xaml.cs
- SQlBooleanStorage.cs
- BoundColumn.cs
- Executor.cs
- GridViewDeleteEventArgs.cs
- XhtmlBasicFormAdapter.cs
- ActiveXHelper.cs
- TableLayoutColumnStyleCollection.cs
- X509Utils.cs
- GeneralTransform3DGroup.cs
- DynamicMethod.cs
- ExtractedStateEntry.cs
- OleDbSchemaGuid.cs
- ByteConverter.cs
- ConfigurationSectionCollection.cs
- StaticDataManager.cs
- CqlGenerator.cs
- ScrollEventArgs.cs
- ExpressionBindings.cs
- ScriptReferenceBase.cs
- EpmSourceTree.cs
- StateBag.cs
- FixUp.cs
- BaseProcessor.cs
- CompModSwitches.cs
- SafeBitVector32.cs
- RectValueSerializer.cs
- __TransparentProxy.cs
- ExpressionNode.cs
- FixedSchema.cs
- XmlWrappingReader.cs
- AdornerHitTestResult.cs
- Item.cs
- XamlToRtfWriter.cs
- InstancePersistenceException.cs
- DoubleLinkList.cs
- ZipIOFileItemStream.cs
- FreezableOperations.cs
- ResponseStream.cs
- DependencyPropertyKind.cs
- DataGridViewLinkColumn.cs
- DbConnectionPoolGroupProviderInfo.cs
- Rfc2898DeriveBytes.cs
- ManagementObjectSearcher.cs
- CheckBox.cs
- TemplatedAdorner.cs
- QueryAccessibilityHelpEvent.cs
- ArglessEventHandlerProxy.cs
- Input.cs
- TcpServerChannel.cs
- XmlnsDefinitionAttribute.cs
- DataGridColumnCollectionEditor.cs
- ToolStripDropDownDesigner.cs
- PageCodeDomTreeGenerator.cs
- FloaterParagraph.cs
- complextypematerializer.cs
- WebPartAuthorizationEventArgs.cs
- SecurityContext.cs
- WebBaseEventKeyComparer.cs
- BulletedListEventArgs.cs
- FileDialogCustomPlace.cs
- TrayIconDesigner.cs
- BrushConverter.cs
- ListItemCollection.cs
- SocketConnection.cs
- BounceEase.cs
- XmlCodeExporter.cs
- DbMetaDataColumnNames.cs
- XmlAttributes.cs
- FileVersion.cs
- BindingListCollectionView.cs
- CookieHandler.cs
- ObjectDataSourceSelectingEventArgs.cs
- DrawingDrawingContext.cs
- ToolboxItemCollection.cs
- Image.cs
- MetafileHeaderWmf.cs
- SystemColorTracker.cs
- ServiceModelEnumValidator.cs
- bidPrivateBase.cs
- LinqDataSourceDeleteEventArgs.cs
- RootCodeDomSerializer.cs
- lengthconverter.cs
- FontWeights.cs
- LayoutSettings.cs
- WpfKnownType.cs