Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / GeneralTransform3DGroup.cs / 1305600 / GeneralTransform3DGroup.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransform3DGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// GeneralTransform3D group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransform3DGroup : GeneralTransform3D { #region Constructors ////// Default Constructor /// public GeneralTransform3DGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point3D inPoint, out Point3D result) { result = inPoint; // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return false; } bool pointTransformed = true; // transform the point through each of the transforms for (int i = 0, count = children.Count; i < count; i++) { if (children._collection[i].TryTransform(inPoint, out result) == false) { pointTransformed = false; break; } inPoint = result; } return pointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect3D TransformBounds(Rect3D rect) { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return rect; } Rect3D result = rect; for (int i = 0, count = children.Count; i < count; i++) { result = children._collection[i].TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform3D Inverse { get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } GeneralTransform3DGroup group = new GeneralTransform3DGroup(); for (int i = children.Count - 1; i >= 0; i--) { GeneralTransform3D g = children._collection[i].Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform3D AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } Matrix3D matrix = Matrix3D.Identity; for (int i = 0, count = children.Count; i < count; i++) { Transform3D t = children._collection[i].AffineTransform; t.Append(ref matrix); } return new MatrixTransform3D(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransform3DGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// GeneralTransform3D group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransform3DGroup : GeneralTransform3D { #region Constructors ////// Default Constructor /// public GeneralTransform3DGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point3D inPoint, out Point3D result) { result = inPoint; // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return false; } bool pointTransformed = true; // transform the point through each of the transforms for (int i = 0, count = children.Count; i < count; i++) { if (children._collection[i].TryTransform(inPoint, out result) == false) { pointTransformed = false; break; } inPoint = result; } return pointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect3D TransformBounds(Rect3D rect) { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return rect; } Rect3D result = rect; for (int i = 0, count = children.Count; i < count; i++) { result = children._collection[i].TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform3D Inverse { get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } GeneralTransform3DGroup group = new GeneralTransform3DGroup(); for (int i = children.Count - 1; i >= 0; i--) { GeneralTransform3D g = children._collection[i].Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform3D AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } Matrix3D matrix = Matrix3D.Identity; for (int i = 0, count = children.Count; i < count; i++) { Transform3D t = children._collection[i].AffineTransform; t.Append(ref matrix); } return new MatrixTransform3D(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
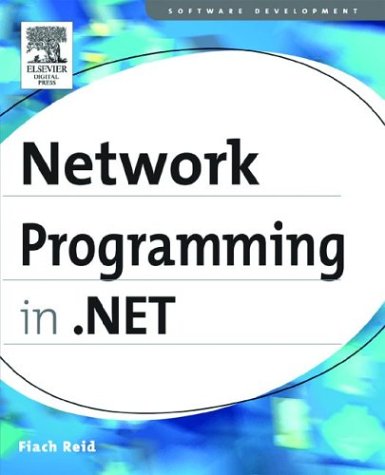
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GetIndexBinder.cs
- XPathNavigator.cs
- PageHandlerFactory.cs
- AxisAngleRotation3D.cs
- RotateTransform3D.cs
- _NestedSingleAsyncResult.cs
- DataGridViewToolTip.cs
- ConstraintStruct.cs
- CompiledRegexRunner.cs
- KeyPullup.cs
- ContextQuery.cs
- ComplexLine.cs
- XmlBindingWorker.cs
- XPathEmptyIterator.cs
- AnnotationMap.cs
- InstalledFontCollection.cs
- SafeCryptContextHandle.cs
- ItemDragEvent.cs
- ErrorActivity.cs
- SynthesizerStateChangedEventArgs.cs
- UnmanagedHandle.cs
- HMACSHA256.cs
- SqlXml.cs
- OuterGlowBitmapEffect.cs
- StrokeCollection2.cs
- NativeBuffer.cs
- ProtocolInformationReader.cs
- ScaleTransform.cs
- GridPatternIdentifiers.cs
- TransformationRules.cs
- NeutralResourcesLanguageAttribute.cs
- ViewGenerator.cs
- BitmapCache.cs
- TextParaLineResult.cs
- TextEditorTyping.cs
- XmlSchemaRedefine.cs
- TableStyle.cs
- XmlDownloadManager.cs
- GroupDescription.cs
- MethodSet.cs
- ExceptionValidationRule.cs
- QueuePathDialog.cs
- ItemDragEvent.cs
- XmlWrappingReader.cs
- SqlNode.cs
- ObjectConverter.cs
- ModelTreeEnumerator.cs
- MemberInfoSerializationHolder.cs
- SecUtil.cs
- sqlstateclientmanager.cs
- CodeAttributeArgument.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ConfigurationLocation.cs
- ExpressionLexer.cs
- ProcessHost.cs
- TraceContext.cs
- HandlerBase.cs
- FileResponseElement.cs
- SmiRequestExecutor.cs
- PagePropertiesChangingEventArgs.cs
- GridPatternIdentifiers.cs
- Int16.cs
- CLSCompliantAttribute.cs
- NamespaceInfo.cs
- DummyDataSource.cs
- InfoCardBaseException.cs
- XmlSchemaAttributeGroup.cs
- WindowsAuthenticationModule.cs
- CollectionViewGroup.cs
- CancellationHandlerDesigner.cs
- WebPartsPersonalization.cs
- ColumnHeader.cs
- XPathAxisIterator.cs
- DrawingContextDrawingContextWalker.cs
- XmlValueConverter.cs
- DataGridItemAttachedStorage.cs
- XsltContext.cs
- ReadWriteObjectLock.cs
- GridSplitter.cs
- CookieParameter.cs
- CommandSet.cs
- VersionPair.cs
- UxThemeWrapper.cs
- HMACRIPEMD160.cs
- TargetInvocationException.cs
- DataGridItemEventArgs.cs
- HierarchicalDataBoundControl.cs
- Byte.cs
- TextBoxBase.cs
- SingleKeyFrameCollection.cs
- SkewTransform.cs
- EmissiveMaterial.cs
- ConfigXmlSignificantWhitespace.cs
- EncodingInfo.cs
- Maps.cs
- Trace.cs
- AsyncDataRequest.cs
- SqlFormatter.cs
- ProfileBuildProvider.cs
- EvidenceTypeDescriptor.cs