Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / MethodSet.cs / 1305376 / MethodSet.cs
using System; using System.Collections; using System.Runtime.InteropServices; using WbemClient_v1; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Represents the set of methods available in the collection. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class MethodDataCollection : ICollection, IEnumerable { private ManagementObject parent; private class enumLock { } //used to lock usage of BeginMethodEnum/NextMethod internal MethodDataCollection(ManagementObject parent) : base() { this.parent = parent; } // //ICollection // ///using System; /// using System.Management; /// /// // This sample demonstrates enumerate all methods in a ManagementClass object. /// class Sample_MethodDataCollection /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("win32_logicaldisk"); /// MethodDataCollection diskMethods = diskClass.Methods; /// foreach (MethodData method in diskMethods) { /// Console.WriteLine("Method = " + method.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates enumerate all methods in a ManagementClass object. /// Class Sample_MethodDataCollection /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("win32_logicaldisk") /// Dim diskMethods As MethodDataCollection = diskClass.Methods /// Dim method As MethodData /// For Each method In diskMethods /// Console.WriteLine("Method = " & method.Name) /// Next method /// Return 0 /// End Function /// End Class ///
////// ///Represents the number of objects in the ///. /// public int Count { get { int i = 0; IWbemClassObjectFreeThreaded inParameters = null, outParameters = null; string methodName; int status = (int)ManagementStatus.Failed; lock(typeof(enumLock)) { try { status = parent.wbemObject.BeginMethodEnumeration_(0); if (status >= 0) { methodName = ""; // Condition primer to branch into the while loop. while (methodName != null && status >= 0 && status != (int)tag_WBEMSTATUS.WBEM_S_NO_MORE_DATA) { methodName = null; inParameters = null; outParameters = null; status = parent.wbemObject.NextMethod_(0, out methodName, out inParameters, out outParameters); if (status >= 0 && status != (int)tag_WBEMSTATUS.WBEM_S_NO_MORE_DATA) i++; } parent.wbemObject.EndMethodEnumeration_(); // Ignore status. } } catch (COMException e) { ManagementException.ThrowWithExtendedInfo(e); } } // lock if ((status & 0xfffff000) == 0x80041000) { ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); } else if ((status & 0x80000000) != 0) { Marshal.ThrowExceptionForHR(status); } return i; } } ///The number of objects in the ///. /// ///Indicates whether the object is synchronized. ////// public bool IsSynchronized { get { return false; } } ////// if the object is synchronized; /// otherwise, . /// ///Represents the object to be used for synchronization. ////// public object SyncRoot { get { return this; } } ///The object to be used for synchronization. ////// ///Copies the ///into an array. /// /// The array to which to copy the collection. /// The index from which to start. public void CopyTo(Array array, int index) { //Use an enumerator to get the MethodData objects and attach them into the target array foreach (MethodData m in this) array.SetValue(m, index++); } ///Copies the ///into an array. /// /// The destination array to which to copy theCopies the ///to a specialized /// array. objects. /// The index in the destination array from which to start the copy. public void CopyTo(MethodData[] methodArray, int index) { CopyTo((Array)methodArray, index); } // // IEnumerable // IEnumerator IEnumerable.GetEnumerator() { return (IEnumerator)(new MethodDataEnumerator(parent)); } /// /// ///Returns an enumerator for the ///. /// ///Each call to this method /// returns a new enumerator on the collection. Multiple enumerators can be obtained /// for the same method collection. However, each enumerator takes a snapshot /// of the collection, so changes made to the collection after the enumerator was /// obtained are not reflected. ///An public MethodDataEnumerator GetEnumerator() { return new MethodDataEnumerator(parent); } //Enumerator class ///to enumerate through the collection. /// ///Represents the enumerator for ////// objects in the . /// public class MethodDataEnumerator : IEnumerator { private ManagementObject parent; private ArrayList methodNames; //can't use simple array because we don't know the size... private IEnumerator en; //Internal constructor //Because WMI doesn't provide a "GetMethodNames" for methods similar to "GetNames" for properties, //We have to walk the methods list and cache the names here. //We lock to ensure that another thread doesn't interfere in the Begin/Next sequence. internal MethodDataEnumerator(ManagementObject parent) { this.parent = parent; methodNames = new ArrayList(); IWbemClassObjectFreeThreaded inP = null, outP = null; string tempMethodName; int status = (int)ManagementStatus.Failed; lock(typeof(enumLock)) { try { status = parent.wbemObject.BeginMethodEnumeration_(0); if (status >= 0) { tempMethodName = ""; // Condition primer to branch into the while loop. while (tempMethodName != null && status >= 0 && status != (int)tag_WBEMSTATUS.WBEM_S_NO_MORE_DATA) { tempMethodName = null; status = parent.wbemObject.NextMethod_(0, out tempMethodName, out inP, out outP); if (status >= 0 && status != (int)tag_WBEMSTATUS.WBEM_S_NO_MORE_DATA) methodNames.Add(tempMethodName); } parent.wbemObject.EndMethodEnumeration_(); // Ignore status. } } catch (COMException e) { ManagementException.ThrowWithExtendedInfo(e); } en = methodNames.GetEnumerator(); } if ((status & 0xfffff000) == 0x80041000) { ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); } else if ((status & 0x80000000) != 0) { Marshal.ThrowExceptionForHR(status); } } ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate all methods in /// // Win32_LogicalDisk class using MethodDataEnumerator object. /// /// class Sample_MethodDataEnumerator /// { /// public static int Main(string[] args) /// { /// ManagementClass diskClass = new ManagementClass("win32_logicaldisk"); /// MethodDataCollection.MethodDataEnumerator diskEnumerator = /// diskClass.Methods.GetEnumerator(); /// while(diskEnumerator.MoveNext()) /// { /// MethodData method = diskEnumerator.Current; /// Console.WriteLine("Method = " + method.Name); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate all methods in /// ' Win32_LogicalDisk class using MethodDataEnumerator object. /// /// Class Sample_MethodDataEnumerator /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("win32_logicaldisk") /// Dim diskEnumerator As _ /// MethodDataCollection.MethodDataEnumerator = _ /// diskClass.Methods.GetEnumerator() /// While diskEnumerator.MoveNext() /// Dim method As MethodData = diskEnumerator.Current /// Console.WriteLine("Method = " & method.Name) /// End While /// Return 0 /// End Function /// End Class ///
///object IEnumerator.Current { get { return (object)this.Current; } } /// /// ///Returns the current ///in the /// enumeration. The current public MethodData Current { get { return new MethodData(parent, (string)en.Current); } } ///item in the collection. /// ///Moves to the next element in the ///enumeration. public bool MoveNext () { return en.MoveNext(); } /// if the enumerator was successfully advanced to the next method; if the enumerator has passed the end of the collection. /// public void Reset() { en.Reset(); } }//MethodDataEnumerator // //Methods // ///Resets the enumerator to the beginning of the ///enumeration. /// /// The name of the method requested. ///Returns the specified ///from the . A public virtual MethodData this[string methodName] { get { if (null == methodName) throw new ArgumentNullException ("methodName"); return new MethodData(parent, methodName); } } ///instance containing all information about the specified method. /// /// The name of the method to remove from the collection. ///Removes a ///from the . /// public virtual void Remove(string methodName) { if (parent.GetType() == typeof(ManagementObject)) //can't remove methods from instance throw new InvalidOperationException(); int status = (int)ManagementStatus.Failed; try { status = parent.wbemObject.DeleteMethod_(methodName); } catch (COMException e) { ManagementException.ThrowWithExtendedInfo(e); } if ((status & 0xfffff000) == 0x80041000) { ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); } else if ((status & 0x80000000) != 0) { Marshal.ThrowExceptionForHR(status); } } //This variant takes only a method name and assumes a void method with no in/out parameters ////// Removing ///objects from the /// can only be done when the class has no /// instances. Any other case will result in an exception. /// ///Adds a ///to the . /// /// The name of the method to add. ///Adds a ///to the . This overload will /// add a new method with no parameters to the collection. /// public virtual void Add(string methodName) { Add(methodName, null, null); } //This variant takes the full information, i.e. the method name and in & out param objects ///Adding ///objects to the can only /// be done when the class has no instances. Any other case will result in an /// exception. /// /// The name of the method to add. /// TheAdds a ///to the . This overload will add a new method with the /// specified parameter objects to the collection. holding the input parameters to the method. /// The holding the output parameters to the method. /// /// public virtual void Add(string methodName, ManagementBaseObject inParameters, ManagementBaseObject outParameters) { IWbemClassObjectFreeThreaded wbemIn = null, wbemOut = null; if (parent.GetType() == typeof(ManagementObject)) //can't add methods to instance throw new InvalidOperationException(); if (inParameters != null) wbemIn = inParameters.wbemObject; if (outParameters != null) wbemOut = outParameters.wbemObject; int status = (int)ManagementStatus.Failed; try { status = parent.wbemObject.PutMethod_(methodName, 0, wbemIn, wbemOut); } catch (COMException e) { ManagementException.ThrowWithExtendedInfo(e); } if ((status & 0xfffff000) == 0x80041000) { ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); } else if ((status & 0x80000000) != 0) { Marshal.ThrowExceptionForHR(status); } } }//MethodDataCollection } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Adding ///objects to the can only be /// done when the class has no instances. Any other case will result in an /// exception.
Link Menu
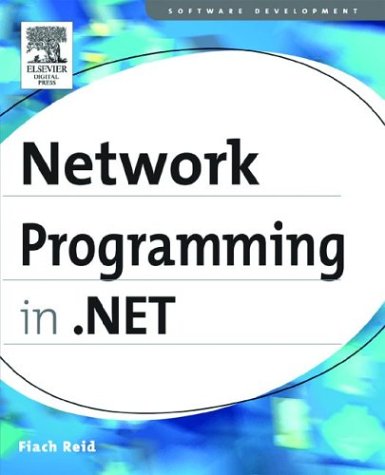
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeWindow.cs
- CustomAttributeFormatException.cs
- RoleGroupCollectionEditor.cs
- PropertyStore.cs
- XmlStreamStore.cs
- XmlLinkedNode.cs
- Int32AnimationBase.cs
- OpenTypeLayoutCache.cs
- XPathQilFactory.cs
- Hash.cs
- XsltLibrary.cs
- WindowsIdentity.cs
- Membership.cs
- SystemIPGlobalStatistics.cs
- TypedReference.cs
- ResizingMessageFilter.cs
- XamlToRtfWriter.cs
- Menu.cs
- DataGridRelationshipRow.cs
- RuntimeArgumentHandle.cs
- OptimalTextSource.cs
- MultiAsyncResult.cs
- WSSecurityOneDotZeroSendSecurityHeader.cs
- ViewDesigner.cs
- RewritingProcessor.cs
- SemaphoreFullException.cs
- DataServiceException.cs
- EventProvider.cs
- Common.cs
- SystemIPInterfaceProperties.cs
- DefaultSerializationProviderAttribute.cs
- oledbmetadatacollectionnames.cs
- CompilationUnit.cs
- XmlRawWriter.cs
- DockPanel.cs
- KeyInterop.cs
- SqlCommandBuilder.cs
- CreateUserErrorEventArgs.cs
- LoadRetryHandler.cs
- ScriptComponentDescriptor.cs
- EventLogPermission.cs
- EventListener.cs
- JpegBitmapDecoder.cs
- ReflectionPermission.cs
- ItemType.cs
- EditableTreeList.cs
- WebServiceResponse.cs
- TokenBasedSet.cs
- Stroke.cs
- ContractNamespaceAttribute.cs
- ArrayList.cs
- base64Transforms.cs
- DocumentPage.cs
- QuadraticBezierSegment.cs
- WindowsFormsHostAutomationPeer.cs
- DesignerActionList.cs
- XmlDocumentType.cs
- hresults.cs
- ConstantSlot.cs
- SinglePhaseEnlistment.cs
- util.cs
- KeyValuePair.cs
- FileLevelControlBuilderAttribute.cs
- PersistenceIOParticipant.cs
- ClosureBinding.cs
- PerformanceCounter.cs
- DebuggerAttributes.cs
- WizardForm.cs
- TableCell.cs
- ListItemViewControl.cs
- BehaviorEditorPart.cs
- EntityChangedParams.cs
- GridViewEditEventArgs.cs
- CachingParameterInspector.cs
- ManagementNamedValueCollection.cs
- SafeRightsManagementPubHandle.cs
- Point3D.cs
- ConfigurationManagerInternalFactory.cs
- AssemblyFilter.cs
- StringSource.cs
- MessageUtil.cs
- SQLByteStorage.cs
- PreviewPageInfo.cs
- EntityContainerEntitySet.cs
- EntitySetRetriever.cs
- TextStore.cs
- DisplayMemberTemplateSelector.cs
- DefaultMemberAttribute.cs
- userdatakeys.cs
- EdmType.cs
- ExceptionRoutedEventArgs.cs
- TdsParser.cs
- ImageCodecInfoPrivate.cs
- AstNode.cs
- SvcMapFileLoader.cs
- ISFClipboardData.cs
- securestring.cs
- DragEvent.cs
- xamlnodes.cs
- HtmlTableRow.cs