Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / SinglePhaseEnlistment.cs / 1305376 / SinglePhaseEnlistment.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Diagnostics; using System.Threading; using System.Transactions; using System.Transactions.Diagnostics; public class SinglePhaseEnlistment : Enlistment { internal SinglePhaseEnlistment( InternalEnlistment enlistment ) : base(enlistment) { } public void Aborted() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Aborted ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Aborted( this.internalEnlistment, null ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } } // Changing the e paramater name would be a breaking change for little benefit. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public void Aborted( Exception e ) { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Aborted ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Aborted( this.internalEnlistment, e ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } } public void Committed() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Committed" ); EnlistmentCallbackPositiveTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Committed ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Committed( this.internalEnlistment ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Committed" ); } } public void InDoubt() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } lock( this.internalEnlistment.SyncRoot ) { if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.InDoubt ); } this.internalEnlistment.State.InDoubt( this.internalEnlistment, null ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } } // Changing the e paramater name would be a breaking change for little benefit. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public void InDoubt( Exception e ) { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } lock( this.internalEnlistment.SyncRoot ) { if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.InDoubt ); } this.internalEnlistment.State.InDoubt( this.internalEnlistment, e ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Diagnostics; using System.Threading; using System.Transactions; using System.Transactions.Diagnostics; public class SinglePhaseEnlistment : Enlistment { internal SinglePhaseEnlistment( InternalEnlistment enlistment ) : base(enlistment) { } public void Aborted() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Aborted ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Aborted( this.internalEnlistment, null ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } } // Changing the e paramater name would be a breaking change for little benefit. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public void Aborted( Exception e ) { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Aborted ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Aborted( this.internalEnlistment, e ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Aborted" ); } } public void Committed() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Committed" ); EnlistmentCallbackPositiveTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.Committed ); } lock( this.internalEnlistment.SyncRoot ) { this.internalEnlistment.State.Committed( this.internalEnlistment ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.Committed" ); } } public void InDoubt() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } lock( this.internalEnlistment.SyncRoot ) { if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.InDoubt ); } this.internalEnlistment.State.InDoubt( this.internalEnlistment, null ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } } // Changing the e paramater name would be a breaking change for little benefit. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public void InDoubt( Exception e ) { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } lock( this.internalEnlistment.SyncRoot ) { if ( DiagnosticTrace.Warning ) { EnlistmentCallbackNegativeTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), this.internalEnlistment.EnlistmentTraceId, EnlistmentCallback.InDoubt ); } this.internalEnlistment.State.InDoubt( this.internalEnlistment, e ); } if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "SinglePhaseEnlistment.InDoubt" ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
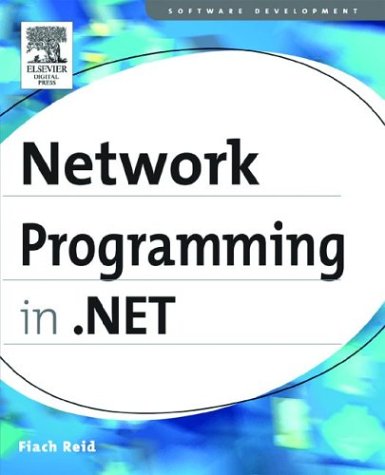
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LineUtil.cs
- BreakSafeBase.cs
- WebPartCloseVerb.cs
- RoleGroupCollection.cs
- HelpFileFileNameEditor.cs
- RequestCachingSection.cs
- PropertyGridCommands.cs
- EmissiveMaterial.cs
- TextContainerChangedEventArgs.cs
- MaxSessionCountExceededException.cs
- SmtpCommands.cs
- XamlValidatingReader.cs
- ListViewItem.cs
- SerializationSectionGroup.cs
- HttpStreamXmlDictionaryWriter.cs
- KoreanLunisolarCalendar.cs
- MenuRendererClassic.cs
- EmptyControlCollection.cs
- ParseNumbers.cs
- BitSet.cs
- HtmlControlPersistable.cs
- BrowserCapabilitiesFactoryBase.cs
- FocusManager.cs
- DataAdapter.cs
- RegisteredHiddenField.cs
- ProxyAttribute.cs
- TimeSpanParse.cs
- EventItfInfo.cs
- CodeObject.cs
- EdmConstants.cs
- ReadOnlyHierarchicalDataSource.cs
- TemplateInstanceAttribute.cs
- TableLayoutRowStyleCollection.cs
- TextAnchor.cs
- ServerIdentity.cs
- EncoderBestFitFallback.cs
- Avt.cs
- Column.cs
- VoiceChangeEventArgs.cs
- UnconditionalPolicy.cs
- MachineKeyConverter.cs
- columnmapfactory.cs
- MergeFilterQuery.cs
- InfoCardSymmetricAlgorithm.cs
- CapabilitiesSection.cs
- NumericUpDownAcceleration.cs
- AuthenticationConfig.cs
- DataSourceIDConverter.cs
- EntityDataSourceMemberPath.cs
- DefaultValueConverter.cs
- CodeTypeOfExpression.cs
- DataViewManagerListItemTypeDescriptor.cs
- RawStylusInputCustomData.cs
- DataBindingCollectionEditor.cs
- TextBoxBase.cs
- SeekableReadStream.cs
- DmlSqlGenerator.cs
- Block.cs
- webeventbuffer.cs
- TextSelectionProcessor.cs
- ContainerVisual.cs
- DialogWindow.cs
- CodeMethodReturnStatement.cs
- ItemAutomationPeer.cs
- XpsFilter.cs
- CfgParser.cs
- DetailsViewActionList.cs
- ManageRequest.cs
- PackageProperties.cs
- ListDictionaryInternal.cs
- SafeSecurityHelper.cs
- UriTemplateVariableQueryValue.cs
- XmlSerializer.cs
- EventLogPermission.cs
- Keywords.cs
- SQLBytes.cs
- XmlMtomReader.cs
- SearchForVirtualItemEventArgs.cs
- BinarySerializer.cs
- cookiecontainer.cs
- SynchronizedInputPattern.cs
- EntityDataSourceValidationException.cs
- CanExecuteRoutedEventArgs.cs
- WindowsGraphicsCacheManager.cs
- XmlAttributes.cs
- CodeVariableDeclarationStatement.cs
- PerformanceCounterPermissionEntry.cs
- MessageHeaderDescription.cs
- BasicCellRelation.cs
- LogArchiveSnapshot.cs
- ColumnMap.cs
- AnnotationComponentManager.cs
- SqlFormatter.cs
- XPathMessageFilterElementCollection.cs
- BasicKeyConstraint.cs
- _ContextAwareResult.cs
- WebException.cs
- Point.cs
- ObjectDataProvider.cs
- ToolStripTextBox.cs