Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / EvidenceTypeDescriptor.cs / 1305376 / EvidenceTypeDescriptor.cs
// ==--== // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // using System; using System.Diagnostics; using System.Diagnostics.Contracts; using System.Runtime.Serialization; namespace System.Security.Policy { ////// Descriptor stored in the Evidence collection to detail the information we have about a type of /// evidence. This descriptor also stores any evidence that's been generated of the specific type. /// [Serializable] internal sealed class EvidenceTypeDescriptor { [NonSerialized] private bool m_hostCanGenerate; [NonSerialized] private bool m_generated; private EvidenceBase m_hostEvidence; private EvidenceBase m_assemblyEvidence; // EvidenceTypeDescriptors are stored in Evidence indexed by the type they describe, so this // information is redundant. We keep it around in checked builds to help debugging, but we can drop // it from retial builds. #if _DEBUG [NonSerialized] private Type m_evidenceType; #endif // _DEBUG public EvidenceTypeDescriptor() { } ////// Make a deep copy of a type descriptor /// private EvidenceTypeDescriptor(EvidenceTypeDescriptor descriptor) { Contract.Assert(descriptor != null); m_hostCanGenerate = descriptor.m_hostCanGenerate; if (descriptor.m_assemblyEvidence != null) { m_assemblyEvidence = descriptor.m_assemblyEvidence.Clone() as EvidenceBase; } if (descriptor.m_hostEvidence != null) { m_hostEvidence = descriptor.m_hostEvidence.Clone() as EvidenceBase; } #if _DEBUG m_evidenceType = descriptor.m_evidenceType; #endif // _DEBUG } ////// Evidence of this type supplied by the assembly /// public EvidenceBase AssemblyEvidence { get { return m_assemblyEvidence; } set { Contract.Assert(value != null); #if _DEBUG Contract.Assert(CheckEvidenceType(value), "Incorrect type of AssemblyEvidence set"); #endif m_assemblyEvidence = value; } } ////// Flag indicating that we've already attempted to generate this type of evidence /// public bool Generated { get { return m_generated; } set { Contract.Assert(value, "Attempt to clear the Generated flag"); m_generated = value; } } ////// Has the HostSecurityManager has told us that it can potentially generate evidence of this type /// public bool HostCanGenerate { get { return m_hostCanGenerate; } set { Contract.Assert(value, "Attempt to clear HostCanGenerate flag"); m_hostCanGenerate = value; } } ////// Evidence of this type supplied by the CLR or the host /// public EvidenceBase HostEvidence { get { return m_hostEvidence; } set { Contract.Assert(value != null); #if _DEBUG Contract.Assert(CheckEvidenceType(value), "Incorrect type of HostEvidence set"); #endif m_hostEvidence = value; } } #if _DEBUG ////// Verify that evidence being stored in this descriptor is of the correct type /// private bool CheckEvidenceType(EvidenceBase evidence) { Contract.Assert(evidence != null); ILegacyEvidenceAdapter legacyAdapter = evidence as ILegacyEvidenceAdapter; Type storedType = legacyAdapter == null ? evidence.GetType() : legacyAdapter.EvidenceType; return m_evidenceType == null || m_evidenceType.IsAssignableFrom(storedType); } #endif // _DEBUG ////// Make a deep copy of this descriptor /// public EvidenceTypeDescriptor Clone() { return new EvidenceTypeDescriptor(this); } #if _DEBUG ////// Set the type that this evidence descriptor refers to. /// internal void SetEvidenceType(Type evidenceType) { Contract.Assert(evidenceType != null); Contract.Assert(m_evidenceType == null, "Attempt to reset evidence type"); m_evidenceType = evidenceType; } #endif // _DEBUG } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==--== // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // using System; using System.Diagnostics; using System.Diagnostics.Contracts; using System.Runtime.Serialization; namespace System.Security.Policy { ////// Descriptor stored in the Evidence collection to detail the information we have about a type of /// evidence. This descriptor also stores any evidence that's been generated of the specific type. /// [Serializable] internal sealed class EvidenceTypeDescriptor { [NonSerialized] private bool m_hostCanGenerate; [NonSerialized] private bool m_generated; private EvidenceBase m_hostEvidence; private EvidenceBase m_assemblyEvidence; // EvidenceTypeDescriptors are stored in Evidence indexed by the type they describe, so this // information is redundant. We keep it around in checked builds to help debugging, but we can drop // it from retial builds. #if _DEBUG [NonSerialized] private Type m_evidenceType; #endif // _DEBUG public EvidenceTypeDescriptor() { } ////// Make a deep copy of a type descriptor /// private EvidenceTypeDescriptor(EvidenceTypeDescriptor descriptor) { Contract.Assert(descriptor != null); m_hostCanGenerate = descriptor.m_hostCanGenerate; if (descriptor.m_assemblyEvidence != null) { m_assemblyEvidence = descriptor.m_assemblyEvidence.Clone() as EvidenceBase; } if (descriptor.m_hostEvidence != null) { m_hostEvidence = descriptor.m_hostEvidence.Clone() as EvidenceBase; } #if _DEBUG m_evidenceType = descriptor.m_evidenceType; #endif // _DEBUG } ////// Evidence of this type supplied by the assembly /// public EvidenceBase AssemblyEvidence { get { return m_assemblyEvidence; } set { Contract.Assert(value != null); #if _DEBUG Contract.Assert(CheckEvidenceType(value), "Incorrect type of AssemblyEvidence set"); #endif m_assemblyEvidence = value; } } ////// Flag indicating that we've already attempted to generate this type of evidence /// public bool Generated { get { return m_generated; } set { Contract.Assert(value, "Attempt to clear the Generated flag"); m_generated = value; } } ////// Has the HostSecurityManager has told us that it can potentially generate evidence of this type /// public bool HostCanGenerate { get { return m_hostCanGenerate; } set { Contract.Assert(value, "Attempt to clear HostCanGenerate flag"); m_hostCanGenerate = value; } } ////// Evidence of this type supplied by the CLR or the host /// public EvidenceBase HostEvidence { get { return m_hostEvidence; } set { Contract.Assert(value != null); #if _DEBUG Contract.Assert(CheckEvidenceType(value), "Incorrect type of HostEvidence set"); #endif m_hostEvidence = value; } } #if _DEBUG ////// Verify that evidence being stored in this descriptor is of the correct type /// private bool CheckEvidenceType(EvidenceBase evidence) { Contract.Assert(evidence != null); ILegacyEvidenceAdapter legacyAdapter = evidence as ILegacyEvidenceAdapter; Type storedType = legacyAdapter == null ? evidence.GetType() : legacyAdapter.EvidenceType; return m_evidenceType == null || m_evidenceType.IsAssignableFrom(storedType); } #endif // _DEBUG ////// Make a deep copy of this descriptor /// public EvidenceTypeDescriptor Clone() { return new EvidenceTypeDescriptor(this); } #if _DEBUG ////// Set the type that this evidence descriptor refers to. /// internal void SetEvidenceType(Type evidenceType) { Contract.Assert(evidenceType != null); Contract.Assert(m_evidenceType == null, "Attempt to reset evidence type"); m_evidenceType = evidenceType; } #endif // _DEBUG } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
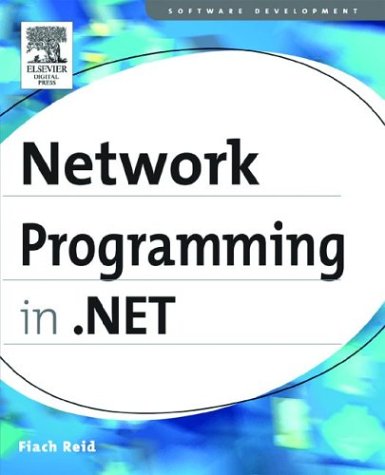
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataRowView.cs
- DnsPermission.cs
- AddInControllerImpl.cs
- ColorMap.cs
- Path.cs
- GeometryConverter.cs
- MaskInputRejectedEventArgs.cs
- CommandBindingCollection.cs
- FlowLayout.cs
- TextEditor.cs
- WindowsStartMenu.cs
- DataException.cs
- UnsettableComboBox.cs
- FunctionDescription.cs
- StartUpEventArgs.cs
- StringConverter.cs
- FontUnitConverter.cs
- TemplateBuilder.cs
- PagesChangedEventArgs.cs
- NullRuntimeConfig.cs
- NamespaceEmitter.cs
- Codec.cs
- InputMethod.cs
- SecurityRuntime.cs
- QuadraticBezierSegment.cs
- XmlQueryType.cs
- RuntimeHandles.cs
- PathTooLongException.cs
- WebControlAdapter.cs
- SoapFault.cs
- UpdateCommand.cs
- XmlTextReaderImplHelpers.cs
- EnumMember.cs
- DoWorkEventArgs.cs
- Inflater.cs
- LocalBuilder.cs
- HttpAsyncResult.cs
- ChannelPool.cs
- ConcurrencyMode.cs
- StrongNameIdentityPermission.cs
- PackagePartCollection.cs
- TypeResolver.cs
- ConfigUtil.cs
- RuleCache.cs
- ShapingEngine.cs
- DesignerActionGlyph.cs
- PathFigureCollection.cs
- DefaultPerformanceCounters.cs
- MetadataPropertyvalue.cs
- DynamicPropertyReader.cs
- Attachment.cs
- AccessViolationException.cs
- CapabilitiesAssignment.cs
- BindingEditor.xaml.cs
- XpsS0ValidatingLoader.cs
- AdapterDictionary.cs
- BrowserDefinitionCollection.cs
- CharacterBuffer.cs
- TemplateField.cs
- TransformerInfoCollection.cs
- SemaphoreSecurity.cs
- SqlMethods.cs
- GuidelineCollection.cs
- GridViewColumnHeaderAutomationPeer.cs
- FileUpload.cs
- ItemDragEvent.cs
- OpenTypeCommon.cs
- MatrixUtil.cs
- _ListenerRequestStream.cs
- BitmapEffectOutputConnector.cs
- Logging.cs
- ListViewItem.cs
- RSAOAEPKeyExchangeFormatter.cs
- SqlError.cs
- CheckedPointers.cs
- DataGridDetailsPresenterAutomationPeer.cs
- QuaternionRotation3D.cs
- FacetDescriptionElement.cs
- EnumMemberAttribute.cs
- PropertyValueUIItem.cs
- XmlSchemaDatatype.cs
- DocumentViewerAutomationPeer.cs
- ImageSourceValueSerializer.cs
- EntityType.cs
- WebControlsSection.cs
- Size.cs
- BuilderInfo.cs
- IPAddress.cs
- Point3DCollectionValueSerializer.cs
- StringConcat.cs
- SafeFindHandle.cs
- FixedSOMPageElement.cs
- SelectionChangedEventArgs.cs
- FormViewPageEventArgs.cs
- JsonXmlDataContract.cs
- Constant.cs
- OutputScope.cs
- PersistChildrenAttribute.cs
- InvokeDelegate.cs
- ProgressBarRenderer.cs