Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / KeyTimeConverter.cs / 1 / KeyTimeConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeyTimeConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; namespace System.Windows { ////// /// public class KeyTimeConverter : TypeConverter { #region Data private static char[] _percentCharacter = new char[] { '%' }; #endregion ////// Returns whether or not this class can convert from a given type /// to an instance of a KeyTime. /// public override bool CanConvertFrom( ITypeDescriptorContext typeDescriptorContext, Type type) { if (type == typeof(string)) { return true; } else { return base.CanConvertFrom( typeDescriptorContext, type); } } ////// Returns whether or not this class can convert from an instance of a /// KeyTime to a given type. /// public override bool CanConvertTo( ITypeDescriptorContext typeDescriptorContext, Type type) { if ( type == typeof(InstanceDescriptor) || type == typeof(string)) { return true; } else { return base.CanConvertTo( typeDescriptorContext, type); } } ////// /// public override object ConvertFrom( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Uniform") { return KeyTime.Uniform; } else if (stringValue == "Paced") { return KeyTime.Paced; } else if (stringValue[stringValue.Length - 1] == _percentCharacter[0]) { stringValue = stringValue.TrimEnd(_percentCharacter); double doubleValue = (double)TypeDescriptor.GetConverter( typeof(double)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); if (doubleValue == 0.0) { return KeyTime.FromPercent(0.0); } else if (doubleValue == 100.0) { return KeyTime.FromPercent(1.0); } else { return KeyTime.FromPercent(doubleValue / 100.0); } } else { TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); return KeyTime.FromTimeSpan(timeSpanValue); } } return base.ConvertFrom( typeDescriptorContext, cultureInfo, value); } ////// /// ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeyTime, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if ( value != null && value is KeyTime) { KeyTime keyTime = (KeyTime)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; switch (keyTime.Type) { case KeyTimeType.Percent: mi = typeof(KeyTime).GetMethod("FromPercent", new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { keyTime.Percent }); case KeyTimeType.TimeSpan: mi = typeof(KeyTime).GetMethod("FromTimeSpan", new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { keyTime.TimeSpan }); case KeyTimeType.Uniform: mi = typeof(KeyTime).GetProperty("Uniform"); return new InstanceDescriptor(mi, null); case KeyTimeType.Paced: mi = typeof(KeyTime).GetProperty("Paced"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(String)) { switch (keyTime.Type) { case KeyTimeType.Uniform: return "Uniform"; case KeyTimeType.Paced: return "Paced"; case KeyTimeType.Percent: string returnValue = (string)TypeDescriptor.GetConverter( typeof(Double)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.Percent * 100.0, destinationType); return returnValue + _percentCharacter[0].ToString(); case KeyTimeType.TimeSpan: return TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.TimeSpan, destinationType); } } } return base.ConvertTo( typeDescriptorContext, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeyTimeConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; namespace System.Windows { ////// /// public class KeyTimeConverter : TypeConverter { #region Data private static char[] _percentCharacter = new char[] { '%' }; #endregion ////// Returns whether or not this class can convert from a given type /// to an instance of a KeyTime. /// public override bool CanConvertFrom( ITypeDescriptorContext typeDescriptorContext, Type type) { if (type == typeof(string)) { return true; } else { return base.CanConvertFrom( typeDescriptorContext, type); } } ////// Returns whether or not this class can convert from an instance of a /// KeyTime to a given type. /// public override bool CanConvertTo( ITypeDescriptorContext typeDescriptorContext, Type type) { if ( type == typeof(InstanceDescriptor) || type == typeof(string)) { return true; } else { return base.CanConvertTo( typeDescriptorContext, type); } } ////// /// public override object ConvertFrom( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Uniform") { return KeyTime.Uniform; } else if (stringValue == "Paced") { return KeyTime.Paced; } else if (stringValue[stringValue.Length - 1] == _percentCharacter[0]) { stringValue = stringValue.TrimEnd(_percentCharacter); double doubleValue = (double)TypeDescriptor.GetConverter( typeof(double)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); if (doubleValue == 0.0) { return KeyTime.FromPercent(0.0); } else if (doubleValue == 100.0) { return KeyTime.FromPercent(1.0); } else { return KeyTime.FromPercent(doubleValue / 100.0); } } else { TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); return KeyTime.FromTimeSpan(timeSpanValue); } } return base.ConvertFrom( typeDescriptorContext, cultureInfo, value); } ////// /// ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeyTime, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if ( value != null && value is KeyTime) { KeyTime keyTime = (KeyTime)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; switch (keyTime.Type) { case KeyTimeType.Percent: mi = typeof(KeyTime).GetMethod("FromPercent", new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { keyTime.Percent }); case KeyTimeType.TimeSpan: mi = typeof(KeyTime).GetMethod("FromTimeSpan", new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { keyTime.TimeSpan }); case KeyTimeType.Uniform: mi = typeof(KeyTime).GetProperty("Uniform"); return new InstanceDescriptor(mi, null); case KeyTimeType.Paced: mi = typeof(KeyTime).GetProperty("Paced"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(String)) { switch (keyTime.Type) { case KeyTimeType.Uniform: return "Uniform"; case KeyTimeType.Paced: return "Paced"; case KeyTimeType.Percent: string returnValue = (string)TypeDescriptor.GetConverter( typeof(Double)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.Percent * 100.0, destinationType); return returnValue + _percentCharacter[0].ToString(); case KeyTimeType.TimeSpan: return TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.TimeSpan, destinationType); } } } return base.ConvertTo( typeDescriptorContext, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
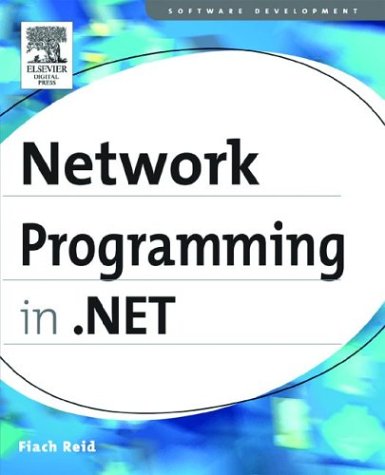
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbWrapper.cs
- UnsafeNativeMethods.cs
- HttpServerVarsCollection.cs
- DataListItem.cs
- NativeMethodsCLR.cs
- SendingRequestEventArgs.cs
- SqlMethodAttribute.cs
- BadImageFormatException.cs
- ResourceLoader.cs
- XmlSchemaResource.cs
- TokenFactoryCredential.cs
- TreeNode.cs
- ValidationErrorEventArgs.cs
- MatcherBuilder.cs
- ConstraintConverter.cs
- DispatchRuntime.cs
- PropertyMapper.cs
- CompiledQuery.cs
- Shape.cs
- DbMetaDataCollectionNames.cs
- _Rfc2616CacheValidators.cs
- DataGridViewComboBoxColumn.cs
- DeviceFilterDictionary.cs
- InheritanceContextHelper.cs
- WinEventHandler.cs
- PipelineModuleStepContainer.cs
- KeyValueConfigurationElement.cs
- Drawing.cs
- AppDomainResourcePerfCounters.cs
- SystemSounds.cs
- CqlParser.cs
- ToolboxControl.cs
- LineMetrics.cs
- Int32RectConverter.cs
- Win32Native.cs
- XmlBinaryReader.cs
- CompilerGlobalScopeAttribute.cs
- ISessionStateStore.cs
- HandlerFactoryWrapper.cs
- TextEditor.cs
- RepeaterItemCollection.cs
- DataBindingsDialog.cs
- DataGridViewRow.cs
- XmlnsDictionary.cs
- MimeFormatExtensions.cs
- Pen.cs
- CancellationScope.cs
- MasterPageParser.cs
- MessageProperties.cs
- HuffmanTree.cs
- Rectangle.cs
- GenerateTemporaryAssemblyTask.cs
- KeyValueSerializer.cs
- VirtualizingPanel.cs
- DrawingCollection.cs
- CookieHandler.cs
- MachineKeyConverter.cs
- DefaultHttpHandler.cs
- BindingBase.cs
- BinaryWriter.cs
- ItemList.cs
- RMPublishingDialog.cs
- StreamReader.cs
- FillRuleValidation.cs
- BitVector32.cs
- PrivilegedConfigurationManager.cs
- CodeSubDirectory.cs
- NetNamedPipeBinding.cs
- SQLInt64Storage.cs
- SolidColorBrush.cs
- Propagator.Evaluator.cs
- ListBoxItem.cs
- AnimationClockResource.cs
- OverrideMode.cs
- DashStyle.cs
- BitmapEffectInput.cs
- SQLBytesStorage.cs
- XPathAncestorQuery.cs
- BinHexEncoding.cs
- LinearGradientBrush.cs
- FamilyMapCollection.cs
- DateTimeSerializationSection.cs
- ZipIOExtraFieldZip64Element.cs
- WSSecurityXXX2005.cs
- ToolBarDesigner.cs
- NativeRecognizer.cs
- WebDisplayNameAttribute.cs
- CheckBoxPopupAdapter.cs
- HtmlImage.cs
- DataTableMapping.cs
- EncoderNLS.cs
- Int64Converter.cs
- DbException.cs
- ConstraintStruct.cs
- HttpSysSettings.cs
- ListBoxAutomationPeer.cs
- VectorCollectionConverter.cs
- ZipIOBlockManager.cs
- CollectionEditorDialog.cs
- FacetValues.cs