Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / DispatchRuntime.cs / 2 / DispatchRuntime.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Description; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Threading; using System.Transactions; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel.Diagnostics; using System.Web.Security; public sealed class DispatchRuntime { ServiceAuthorizationManager serviceAuthorizationManager; ReadOnlyCollectionexternalAuthorizationPolicies; AuditLogLocation securityAuditLogLocation; ConcurrencyMode concurrencyMode; bool suppressAuditFailure; AuditLevel serviceAuthorizationAuditLevel; AuditLevel messageAuthenticationAuditLevel; bool automaticInputSessionShutdown; ChannelDispatcher channelDispatcher; SynchronizedCollection inputSessionShutdownHandlers; EndpointDispatcher endpointDispatcher; IInstanceProvider instanceProvider; IInstanceContextProvider instanceContextProvider; InstanceContext singleton; bool ignoreTransactionMessageProperty; SynchronizedCollection messageInspectors; OperationCollection operations; IDispatchOperationSelector operationSelector; ClientRuntime proxyRuntime; ImmutableDispatchRuntime runtime; SynchronizedCollection instanceContextInitializers; bool isExternalPoliciesSet; bool isAuthorizationManagerSet; SynchronizationContext synchronizationContext; PrincipalPermissionMode principalPermissionMode; object roleProvider; Type type; DispatchOperation unhandled; bool transactionAutoCompleteOnSessionClose; bool impersonateCallerForAllOperations; bool releaseServiceInstanceOnTransactionComplete; SharedRuntimeState shared; internal DispatchRuntime(EndpointDispatcher endpointDispatcher) : this(new SharedRuntimeState(true)) { if (endpointDispatcher == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("endpointDispatcher"); this.endpointDispatcher = endpointDispatcher; DiagnosticUtility.DebugAssert(shared.IsOnServer, "Server constructor called on client?"); } internal DispatchRuntime(ClientRuntime proxyRuntime, SharedRuntimeState shared) : this(shared) { if (proxyRuntime == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("proxyRuntime"); this.proxyRuntime = proxyRuntime; this.instanceProvider = new CallbackInstanceProvider(); this.channelDispatcher = new ChannelDispatcher(shared); this.instanceContextProvider = InstanceContextProviderBase.GetProviderForMode(InstanceContextMode.PerSession, this); DiagnosticUtility.DebugAssert(!shared.IsOnServer, "Client constructor called on server?"); } DispatchRuntime(SharedRuntimeState shared) { this.shared = shared; this.operations = new OperationCollection(this); this.inputSessionShutdownHandlers = this.NewBehaviorCollection (); this.messageInspectors = this.NewBehaviorCollection (); this.instanceContextInitializers = this.NewBehaviorCollection (); this.synchronizationContext = ThreadBehavior.GetCurrentSynchronizationContext(); this.automaticInputSessionShutdown = true; this.principalPermissionMode = ServiceAuthorizationBehavior.DefaultPrincipalPermissionMode; this.securityAuditLogLocation = ServiceSecurityAuditBehavior.defaultAuditLogLocation; this.suppressAuditFailure = ServiceSecurityAuditBehavior.defaultSuppressAuditFailure; this.serviceAuthorizationAuditLevel = ServiceSecurityAuditBehavior.defaultServiceAuthorizationAuditLevel; this.messageAuthenticationAuditLevel = ServiceSecurityAuditBehavior.defaultMessageAuthenticationAuditLevel; this.unhandled = new DispatchOperation(this, "*", MessageHeaders.WildcardAction, MessageHeaders.WildcardAction); this.unhandled.InternalFormatter = MessageOperationFormatter.Instance; this.unhandled.InternalInvoker = new UnhandledActionInvoker(this); } public IInstanceContextProvider InstanceContextProvider { get { return this.instanceContextProvider; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); } lock (this.ThisLock) { this.InvalidateRuntime(); this.instanceContextProvider = value; } } } public InstanceContext SingletonInstanceContext { get { return this.singleton; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); } lock (this.ThisLock) { this.InvalidateRuntime(); this.singleton = value; } } } public ConcurrencyMode ConcurrencyMode { get { return this.concurrencyMode; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.concurrencyMode = value; } } } public AuditLogLocation SecurityAuditLogLocation { get { return this.securityAuditLogLocation; } set { if (!AuditLogLocationHelper.IsDefined(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); lock (this.ThisLock) { this.InvalidateRuntime(); this.securityAuditLogLocation = value; } } } public bool SuppressAuditFailure { get { return this.suppressAuditFailure; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.suppressAuditFailure = value; } } } public AuditLevel ServiceAuthorizationAuditLevel { get { return this.serviceAuthorizationAuditLevel; } set { if (!AuditLevelHelper.IsDefined(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); lock (this.ThisLock) { this.InvalidateRuntime(); this.serviceAuthorizationAuditLevel = value; } } } public AuditLevel MessageAuthenticationAuditLevel { get { return this.messageAuthenticationAuditLevel; } set { if (!AuditLevelHelper.IsDefined(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); lock (this.ThisLock) { this.InvalidateRuntime(); this.messageAuthenticationAuditLevel = value; } } } internal bool RequiresAuthorization { get { return (this.isAuthorizationManagerSet || this.isExternalPoliciesSet || AuditLevel.Success == (this.serviceAuthorizationAuditLevel & AuditLevel.Success)); } } public ReadOnlyCollection ExternalAuthorizationPolicies { get { return this.externalAuthorizationPolicies; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.externalAuthorizationPolicies = value; this.isExternalPoliciesSet = true; } } } public ServiceAuthorizationManager ServiceAuthorizationManager { get { return this.serviceAuthorizationManager; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.serviceAuthorizationManager = value; this.isAuthorizationManagerSet = true; } } } public bool AutomaticInputSessionShutdown { get { return this.automaticInputSessionShutdown; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.automaticInputSessionShutdown = value; } } } public ChannelDispatcher ChannelDispatcher { get { return this.channelDispatcher ?? this.endpointDispatcher.ChannelDispatcher; } } public ClientRuntime CallbackClientRuntime { get { if (this.proxyRuntime == null) { lock (this.ThisLock) { if (this.proxyRuntime == null) { this.proxyRuntime = new ClientRuntime(this, this.shared); } } } return this.proxyRuntime; } } public EndpointDispatcher EndpointDispatcher { get { return this.endpointDispatcher; } } internal bool HasMatchAllOperation { get { lock (this.ThisLock) { return !(this.unhandled.Invoker is UnhandledActionInvoker); } } } public bool ImpersonateCallerForAllOperations { get { return this.impersonateCallerForAllOperations; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.impersonateCallerForAllOperations = value; } } } public SynchronizedCollection InputSessionShutdownHandlers { get { return this.inputSessionShutdownHandlers; } } public bool IgnoreTransactionMessageProperty { get { return this.ignoreTransactionMessageProperty; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.ignoreTransactionMessageProperty = value; } } } public IInstanceProvider InstanceProvider { get { return this.instanceProvider; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.instanceProvider = value; } } } internal bool EnableFaults { get { if (this.IsOnServer) { ChannelDispatcher channelDispatcher = this.ChannelDispatcher; return (channelDispatcher != null) && channelDispatcher.EnableFaults; } else { return this.shared.EnableFaults; } } } internal bool IsOnServer { get { return this.shared.IsOnServer; } } internal bool ManualAddressing { get { if (this.IsOnServer) { ChannelDispatcher channelDispatcher = this.ChannelDispatcher; return (channelDispatcher != null) && channelDispatcher.ManualAddressing; } else { return this.shared.ManualAddressing; } } } internal int MaxCallContextInitializers { get { lock (this.ThisLock) { int max = 0; for (int i=0; i MessageInspectors { get { return this.messageInspectors; } } public SynchronizedKeyedCollection Operations { get { return this.operations; } } public IDispatchOperationSelector OperationSelector { get { return this.operationSelector; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.operationSelector = value; } } } // Internal access to CallbackClientRuntime, but this one doesn't create on demand internal ClientRuntime ClientRuntime { get { return this.proxyRuntime; } } public bool ReleaseServiceInstanceOnTransactionComplete { get { return this.releaseServiceInstanceOnTransactionComplete; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.releaseServiceInstanceOnTransactionComplete = value; } } } public SynchronizedCollection InstanceContextInitializers { get { return this.instanceContextInitializers; } } public SynchronizationContext SynchronizationContext { get { return this.synchronizationContext; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.synchronizationContext = value; } } } internal object ThisLock { get { return this.shared; } } public PrincipalPermissionMode PrincipalPermissionMode { get { return this.principalPermissionMode; } set { if (!PrincipalPermissionModeHelper.IsDefined(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); lock (this.ThisLock) { this.InvalidateRuntime(); this.principalPermissionMode = value; } } } internal bool IsRoleProviderSet { get { return this.roleProvider != null; } } public RoleProvider RoleProvider { get { return (RoleProvider)this.roleProvider; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.roleProvider = value; } } } public bool TransactionAutoCompleteOnSessionClose { get { return this.transactionAutoCompleteOnSessionClose; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.transactionAutoCompleteOnSessionClose = value; } } } public Type Type { get { return this.type; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.type = value; } } } public DispatchOperation UnhandledDispatchOperation { get { return this.unhandled; } set { if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); lock (this.ThisLock) { this.InvalidateRuntime(); this.unhandled = value; } } } public bool ValidateMustUnderstand { get { return this.shared.ValidateMustUnderstand; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.shared.ValidateMustUnderstand = value; } } } internal DispatchOperationRuntime GetOperation(ref Message message) { ImmutableDispatchRuntime runtime = this.GetRuntime(); return runtime.GetOperation(ref message); } internal ImmutableDispatchRuntime GetRuntime() { ImmutableDispatchRuntime runtime = this.runtime; if (runtime != null) { return runtime; } else { return GetRuntimeCore(); } } ImmutableDispatchRuntime GetRuntimeCore() { lock (this.ThisLock) { if (this.runtime == null) this.runtime = new ImmutableDispatchRuntime(this); return this.runtime; } } internal void InvalidateRuntime() { lock (this.ThisLock) { this.shared.ThrowIfImmutable(); this.runtime = null; } } internal void LockDownProperties() { this.shared.LockDownProperties(); } internal SynchronizedCollection NewBehaviorCollection () { return new DispatchBehaviorCollection (this); } class DispatchBehaviorCollection : SynchronizedCollection { DispatchRuntime outer; internal DispatchBehaviorCollection(DispatchRuntime outer) : base(outer.ThisLock) { this.outer = outer; } protected override void ClearItems() { this.outer.InvalidateRuntime(); base.ClearItems(); } protected override void InsertItem(int index, T item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } this.outer.InvalidateRuntime(); base.InsertItem(index, item); } protected override void RemoveItem(int index) { this.outer.InvalidateRuntime(); base.RemoveItem(index); } protected override void SetItem(int index, T item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } this.outer.InvalidateRuntime(); base.SetItem(index, item); } } class OperationCollection : SynchronizedKeyedCollection { DispatchRuntime outer; internal OperationCollection(DispatchRuntime outer) : base(outer.ThisLock) { this.outer = outer; } protected override void ClearItems() { this.outer.InvalidateRuntime(); base.ClearItems(); } protected override string GetKeyForItem(DispatchOperation item) { return item.Name; } protected override void InsertItem(int index, DispatchOperation item) { if (item == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); if (item.Parent != this.outer) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SFxMismatchedOperationParent)); this.outer.InvalidateRuntime(); base.InsertItem(index, item); } protected override void RemoveItem(int index) { this.outer.InvalidateRuntime(); base.RemoveItem(index); } protected override void SetItem(int index, DispatchOperation item) { if (item == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); if (item.Parent != this.outer) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SFxMismatchedOperationParent)); this.outer.InvalidateRuntime(); base.SetItem(index, item); } } internal class UnhandledActionInvoker : IOperationInvoker { DispatchRuntime dispatchRuntime; public UnhandledActionInvoker(DispatchRuntime dispatchRuntime) { this.dispatchRuntime = dispatchRuntime; } public bool IsSynchronous { get { return true; } } public object[] AllocateInputs() { return new object[1]; } public object Invoke(object instance, object[] inputs, out object[] outputs) { outputs = EmptyArray
Link Menu
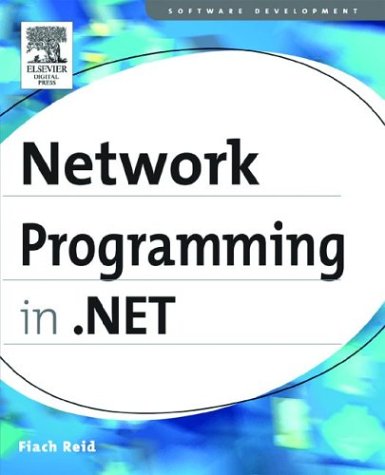
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeParameter.cs
- DummyDataSource.cs
- FastEncoder.cs
- AuthenticateEventArgs.cs
- XsdDateTime.cs
- Decimal.cs
- AssemblyCache.cs
- DetailsView.cs
- DefaultEvaluationContext.cs
- XPathDocumentNavigator.cs
- ChangeProcessor.cs
- StringValidatorAttribute.cs
- AsyncStreamReader.cs
- WebPartMovingEventArgs.cs
- WorkflowQueue.cs
- DataRowCollection.cs
- PageCodeDomTreeGenerator.cs
- Imaging.cs
- ProtocolException.cs
- DBDataPermission.cs
- Effect.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- DeploymentSection.cs
- X509LogoTypeExtension.cs
- ImageList.cs
- ListBindingConverter.cs
- PostBackOptions.cs
- RectangleHotSpot.cs
- TcpTransportBindingElement.cs
- SecureEnvironment.cs
- ComboBoxHelper.cs
- OperationAbortedException.cs
- ObjectDataSourceEventArgs.cs
- DesignerActionHeaderItem.cs
- ElementHostPropertyMap.cs
- DataServiceRequestOfT.cs
- DataRelationCollection.cs
- MenuItemBindingCollection.cs
- HierarchicalDataBoundControlAdapter.cs
- XmlUrlEditor.cs
- SmtpCommands.cs
- StringInfo.cs
- XXXInfos.cs
- TransformationRules.cs
- DayRenderEvent.cs
- GC.cs
- UITypeEditor.cs
- HashCryptoHandle.cs
- DataGrid.cs
- TextReader.cs
- Rfc2898DeriveBytes.cs
- StyleSheetDesigner.cs
- VoiceSynthesis.cs
- SafePEFileHandle.cs
- ProcessModuleCollection.cs
- CharacterHit.cs
- _IPv6Address.cs
- MaskDesignerDialog.cs
- DispatcherExceptionEventArgs.cs
- HashCodeCombiner.cs
- DesignDataSource.cs
- GeneratedCodeAttribute.cs
- WebBrowserBase.cs
- DashStyle.cs
- Char.cs
- HtmlWindow.cs
- WrappedIUnknown.cs
- GridViewCellAutomationPeer.cs
- DesignDataSource.cs
- AngleUtil.cs
- UserPersonalizationStateInfo.cs
- ListBindingHelper.cs
- RequestedSignatureDialog.cs
- CompilationRelaxations.cs
- PageCodeDomTreeGenerator.cs
- ProcessProtocolHandler.cs
- HostedController.cs
- EnumMemberAttribute.cs
- HtmlTernaryTree.cs
- EntityDataSourceContextCreatingEventArgs.cs
- OpenTypeLayout.cs
- PropertyCondition.cs
- ApplicationContext.cs
- SqlUdtInfo.cs
- EntityTypeEmitter.cs
- Constants.cs
- ScrollItemPatternIdentifiers.cs
- DecoderFallback.cs
- RequestStatusBarUpdateEventArgs.cs
- DataGridHeaderBorder.cs
- Message.cs
- URIFormatException.cs
- GAC.cs
- TextEncodedRawTextWriter.cs
- CreateCardRequest.cs
- RtfToXamlReader.cs
- odbcmetadatafactory.cs
- FilterQuery.cs
- Blend.cs
- SizeAnimationClockResource.cs