Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Rectangle.cs / 1 / Rectangle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; ////// /// [ TypeConverterAttribute(typeof(RectangleConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct Rectangle { ////// Stores the location and size of a rectangular region. For /// more advanced region functions use a ////// object. /// /// /// public static readonly Rectangle Empty = new Rectangle(); private int x; private int y; private int width; private int height; ////// Stores the location and size of a rectangular region. For /// more advanced region functions use a ////// object. /// /// /// public Rectangle(int x, int y, int width, int height) { this.x = x; this.y = y; this.width = width; this.height = height; } ////// Initializes a new instance of the ////// class with the specified location and size. /// /// /// public Rectangle(Point location, Size size) { this.x = location.X; this.y = location.Y; this.width = size.Width; this.height = size.Height; } ////// Initializes a new instance of the Rectangle class with the specified location /// and size. /// ////// /// Creates a new // !! Not in C++ version public static Rectangle FromLTRB(int left, int top, int right, int bottom) { return new Rectangle(left, top, right - left, bottom - top); } ///with /// the specified location and size. /// /// /// [Browsable(false)] public Point Location { get { return new Point(X, Y); } set { X = value.X; Y = value.Y; } } ////// Gets or sets the coordinates of the /// upper-left corner of the rectangular region represented by this ///. /// /// /// Gets or sets the size of this [Browsable(false)] public Size Size { get { return new Size(Width, Height); } set { this.Width = value.Width; this.Height = value.Height; } } ///. /// /// /// Gets or sets the x-coordinate of the /// upper-left corner of the rectangular region defined by this public int X { get { return x; } set { x = value; } } ///. /// /// /// Gets or sets the y-coordinate of the /// upper-left corner of the rectangular region defined by this public int Y { get { return y; } set { y = value; } } ///. /// /// /// Gets or sets the width of the rectangular /// region defined by this public int Width { get { return width; } set { width = value; } } ///. /// /// /// Gets or sets the width of the rectangular /// region defined by this public int Height { get { return height; } set { height = value; } } ///. /// /// /// [Browsable(false)] public int Left { get { return X; } } ////// Gets the x-coordinate of the upper-left corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Top { get { return Y; } } ////// Gets the y-coordinate of the upper-left corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Right { get { return X + Width; } } ////// Gets the x-coordinate of the lower-right corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Bottom { get { return Y + Height; } } ////// Gets the y-coordinate of the lower-right corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public bool IsEmpty { get { return height == 0 && width == 0 && x == 0 && y == 0; // C++ uses this definition: // return(Width <= 0 )|| (Height <= 0); } } ////// Tests whether this ///has a /// or a of 0. /// /// /// public override bool Equals(object obj) { if (!(obj is Rectangle)) return false; Rectangle comp = (Rectangle)obj; return(comp.X == this.X) && (comp.Y == this.Y) && (comp.Width == this.Width) && (comp.Height == this.Height); } ////// Tests whether ///is a with /// the same location and size of this Rectangle. /// /// /// public static bool operator ==(Rectangle left, Rectangle right) { return (left.X == right.X && left.Y == right.Y && left.Width == right.Width && left.Height == right.Height); } ////// Tests whether two ////// objects have equal location and size. /// /// /// public static bool operator !=(Rectangle left, Rectangle right) { return !(left == right); } ////// Tests whether two ////// objects differ in location or size. /// /// /// Converts a RectangleF to a Rectangle by performing a ceiling operation on /// all the coordinates. /// public static Rectangle Ceiling(RectangleF value) { return new Rectangle((int)Math.Ceiling(value.X), (int)Math.Ceiling(value.Y), (int)Math.Ceiling(value.Width), (int)Math.Ceiling(value.Height)); } ////// /// Converts a RectangleF to a Rectangle by performing a truncate operation on /// all the coordinates. /// public static Rectangle Truncate(RectangleF value) { return new Rectangle((int)value.X, (int)value.Y, (int)value.Width, (int)value.Height); } ////// /// Converts a RectangleF to a Rectangle by performing a round operation on /// all the coordinates. /// public static Rectangle Round(RectangleF value) { return new Rectangle((int)Math.Round(value.X), (int)Math.Round(value.Y), (int)Math.Round(value.Width), (int)Math.Round(value.Height)); } ////// /// public bool Contains(int x, int y) { return this.X <= x && x < this.X + this.Width && this.Y <= y && y < this.Y + this.Height; } ////// Determines if the specfied point is contained within the /// rectangular region defined by this ///. /// /// /// public bool Contains(Point pt) { return Contains(pt.X, pt.Y); } ////// Determines if the specfied point is contained within the /// rectangular region defined by this ///. /// /// /// public bool Contains(Rectangle rect) { return(this.X <= rect.X) && ((rect.X + rect.Width) <= (this.X + this.Width)) && (this.Y <= rect.Y) && ((rect.Y + rect.Height) <= (this.Y + this.Height)); } // !! Not in C++ version ////// Determines if the rectangular region represented by /// ///is entirely contained within the rectangular region represented by /// this . /// /// /// public override int GetHashCode() { return(int)((UInt32)X ^ (((UInt32)Y << 13) | ((UInt32)Y >> 19)) ^ (((UInt32)Width << 26) | ((UInt32)Width >> 6)) ^ (((UInt32)Height << 7) | ((UInt32)Height >> 25))); } ///[To be supplied.] ////// /// public void Inflate(int width, int height) { this.X -= width; this.Y -= height; this.Width += 2*width; this.Height += 2*height; } ////// Inflates this ////// by the specified amount. /// /// /// Inflates this public void Inflate(Size size) { Inflate(size.Width, size.Height); } ///by the specified amount. /// /// /// // !! Not in C++ public static Rectangle Inflate(Rectangle rect, int x, int y) { Rectangle r = rect; r.Inflate(x, y); return r; } ////// Creates a ////// that is inflated by the specified amount. /// /// Creates a Rectangle that represents the intersection between this Rectangle and rect. /// public void Intersect(Rectangle rect) { Rectangle result = Rectangle.Intersect(rect, this); this.X = result.X; this.Y = result.Y; this.Width = result.Width; this.Height = result.Height; } ////// /// Creates a rectangle that represents the intersetion between a and /// b. If there is no intersection, null is returned. /// public static Rectangle Intersect(Rectangle a, Rectangle b) { int x1 = Math.Max(a.X, b.X); int x2 = Math.Min(a.X + a.Width, b.X + b.Width); int y1 = Math.Max(a.Y, b.Y); int y2 = Math.Min(a.Y + a.Height, b.Y + b.Height); if (x2 >= x1 && y2 >= y1) { return new Rectangle(x1, y1, x2 - x1, y2 - y1); } return Rectangle.Empty; } ////// /// Determines if this rectangle intersets with rect. /// public bool IntersectsWith(Rectangle rect) { return(rect.X < this.X + this.Width) && (this.X < (rect.X + rect.Width)) && (rect.Y < this.Y + this.Height) && (this.Y < rect.Y + rect.Height); } ////// /// public static Rectangle Union(Rectangle a, Rectangle b) { int x1 = Math.Min(a.X, b.X); int x2 = Math.Max(a.X + a.Width, b.X + b.Width); int y1 = Math.Min(a.Y, b.Y); int y2 = Math.Max(a.Y + a.Height, b.Y + b.Height); return new Rectangle(x1, y1, x2 - x1, y2 - y1); } ////// Creates a rectangle that represents the union between a and /// b. /// ////// /// public void Offset(Point pos) { Offset(pos.X, pos.Y); } ////// Adjusts the location of this rectangle by the specified amount. /// ////// /// Adjusts the location of this rectangle by the specified amount. /// public void Offset(int x, int y) { this.X += x; this.Y += y; } ////// /// public override string ToString() { return "{X=" + X.ToString(CultureInfo.CurrentCulture) + ",Y=" + Y.ToString(CultureInfo.CurrentCulture) + ",Width=" + Width.ToString(CultureInfo.CurrentCulture) + ",Height=" + Height.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Converts the attributes of this ///to a /// human readable string. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; ////// /// [ TypeConverterAttribute(typeof(RectangleConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct Rectangle { ////// Stores the location and size of a rectangular region. For /// more advanced region functions use a ////// object. /// /// /// public static readonly Rectangle Empty = new Rectangle(); private int x; private int y; private int width; private int height; ////// Stores the location and size of a rectangular region. For /// more advanced region functions use a ////// object. /// /// /// public Rectangle(int x, int y, int width, int height) { this.x = x; this.y = y; this.width = width; this.height = height; } ////// Initializes a new instance of the ////// class with the specified location and size. /// /// /// public Rectangle(Point location, Size size) { this.x = location.X; this.y = location.Y; this.width = size.Width; this.height = size.Height; } ////// Initializes a new instance of the Rectangle class with the specified location /// and size. /// ////// /// Creates a new // !! Not in C++ version public static Rectangle FromLTRB(int left, int top, int right, int bottom) { return new Rectangle(left, top, right - left, bottom - top); } ///with /// the specified location and size. /// /// /// [Browsable(false)] public Point Location { get { return new Point(X, Y); } set { X = value.X; Y = value.Y; } } ////// Gets or sets the coordinates of the /// upper-left corner of the rectangular region represented by this ///. /// /// /// Gets or sets the size of this [Browsable(false)] public Size Size { get { return new Size(Width, Height); } set { this.Width = value.Width; this.Height = value.Height; } } ///. /// /// /// Gets or sets the x-coordinate of the /// upper-left corner of the rectangular region defined by this public int X { get { return x; } set { x = value; } } ///. /// /// /// Gets or sets the y-coordinate of the /// upper-left corner of the rectangular region defined by this public int Y { get { return y; } set { y = value; } } ///. /// /// /// Gets or sets the width of the rectangular /// region defined by this public int Width { get { return width; } set { width = value; } } ///. /// /// /// Gets or sets the width of the rectangular /// region defined by this public int Height { get { return height; } set { height = value; } } ///. /// /// /// [Browsable(false)] public int Left { get { return X; } } ////// Gets the x-coordinate of the upper-left corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Top { get { return Y; } } ////// Gets the y-coordinate of the upper-left corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Right { get { return X + Width; } } ////// Gets the x-coordinate of the lower-right corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public int Bottom { get { return Y + Height; } } ////// Gets the y-coordinate of the lower-right corner of the /// rectangular region defined by this ///. /// /// /// [Browsable(false)] public bool IsEmpty { get { return height == 0 && width == 0 && x == 0 && y == 0; // C++ uses this definition: // return(Width <= 0 )|| (Height <= 0); } } ////// Tests whether this ///has a /// or a of 0. /// /// /// public override bool Equals(object obj) { if (!(obj is Rectangle)) return false; Rectangle comp = (Rectangle)obj; return(comp.X == this.X) && (comp.Y == this.Y) && (comp.Width == this.Width) && (comp.Height == this.Height); } ////// Tests whether ///is a with /// the same location and size of this Rectangle. /// /// /// public static bool operator ==(Rectangle left, Rectangle right) { return (left.X == right.X && left.Y == right.Y && left.Width == right.Width && left.Height == right.Height); } ////// Tests whether two ////// objects have equal location and size. /// /// /// public static bool operator !=(Rectangle left, Rectangle right) { return !(left == right); } ////// Tests whether two ////// objects differ in location or size. /// /// /// Converts a RectangleF to a Rectangle by performing a ceiling operation on /// all the coordinates. /// public static Rectangle Ceiling(RectangleF value) { return new Rectangle((int)Math.Ceiling(value.X), (int)Math.Ceiling(value.Y), (int)Math.Ceiling(value.Width), (int)Math.Ceiling(value.Height)); } ////// /// Converts a RectangleF to a Rectangle by performing a truncate operation on /// all the coordinates. /// public static Rectangle Truncate(RectangleF value) { return new Rectangle((int)value.X, (int)value.Y, (int)value.Width, (int)value.Height); } ////// /// Converts a RectangleF to a Rectangle by performing a round operation on /// all the coordinates. /// public static Rectangle Round(RectangleF value) { return new Rectangle((int)Math.Round(value.X), (int)Math.Round(value.Y), (int)Math.Round(value.Width), (int)Math.Round(value.Height)); } ////// /// public bool Contains(int x, int y) { return this.X <= x && x < this.X + this.Width && this.Y <= y && y < this.Y + this.Height; } ////// Determines if the specfied point is contained within the /// rectangular region defined by this ///. /// /// /// public bool Contains(Point pt) { return Contains(pt.X, pt.Y); } ////// Determines if the specfied point is contained within the /// rectangular region defined by this ///. /// /// /// public bool Contains(Rectangle rect) { return(this.X <= rect.X) && ((rect.X + rect.Width) <= (this.X + this.Width)) && (this.Y <= rect.Y) && ((rect.Y + rect.Height) <= (this.Y + this.Height)); } // !! Not in C++ version ////// Determines if the rectangular region represented by /// ///is entirely contained within the rectangular region represented by /// this . /// /// /// public override int GetHashCode() { return(int)((UInt32)X ^ (((UInt32)Y << 13) | ((UInt32)Y >> 19)) ^ (((UInt32)Width << 26) | ((UInt32)Width >> 6)) ^ (((UInt32)Height << 7) | ((UInt32)Height >> 25))); } ///[To be supplied.] ////// /// public void Inflate(int width, int height) { this.X -= width; this.Y -= height; this.Width += 2*width; this.Height += 2*height; } ////// Inflates this ////// by the specified amount. /// /// /// Inflates this public void Inflate(Size size) { Inflate(size.Width, size.Height); } ///by the specified amount. /// /// /// // !! Not in C++ public static Rectangle Inflate(Rectangle rect, int x, int y) { Rectangle r = rect; r.Inflate(x, y); return r; } ////// Creates a ////// that is inflated by the specified amount. /// /// Creates a Rectangle that represents the intersection between this Rectangle and rect. /// public void Intersect(Rectangle rect) { Rectangle result = Rectangle.Intersect(rect, this); this.X = result.X; this.Y = result.Y; this.Width = result.Width; this.Height = result.Height; } ////// /// Creates a rectangle that represents the intersetion between a and /// b. If there is no intersection, null is returned. /// public static Rectangle Intersect(Rectangle a, Rectangle b) { int x1 = Math.Max(a.X, b.X); int x2 = Math.Min(a.X + a.Width, b.X + b.Width); int y1 = Math.Max(a.Y, b.Y); int y2 = Math.Min(a.Y + a.Height, b.Y + b.Height); if (x2 >= x1 && y2 >= y1) { return new Rectangle(x1, y1, x2 - x1, y2 - y1); } return Rectangle.Empty; } ////// /// Determines if this rectangle intersets with rect. /// public bool IntersectsWith(Rectangle rect) { return(rect.X < this.X + this.Width) && (this.X < (rect.X + rect.Width)) && (rect.Y < this.Y + this.Height) && (this.Y < rect.Y + rect.Height); } ////// /// public static Rectangle Union(Rectangle a, Rectangle b) { int x1 = Math.Min(a.X, b.X); int x2 = Math.Max(a.X + a.Width, b.X + b.Width); int y1 = Math.Min(a.Y, b.Y); int y2 = Math.Max(a.Y + a.Height, b.Y + b.Height); return new Rectangle(x1, y1, x2 - x1, y2 - y1); } ////// Creates a rectangle that represents the union between a and /// b. /// ////// /// public void Offset(Point pos) { Offset(pos.X, pos.Y); } ////// Adjusts the location of this rectangle by the specified amount. /// ////// /// Adjusts the location of this rectangle by the specified amount. /// public void Offset(int x, int y) { this.X += x; this.Y += y; } ////// /// public override string ToString() { return "{X=" + X.ToString(CultureInfo.CurrentCulture) + ",Y=" + Y.ToString(CultureInfo.CurrentCulture) + ",Width=" + Width.ToString(CultureInfo.CurrentCulture) + ",Height=" + Height.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Converts the attributes of this ///to a /// human readable string. ///
Link Menu
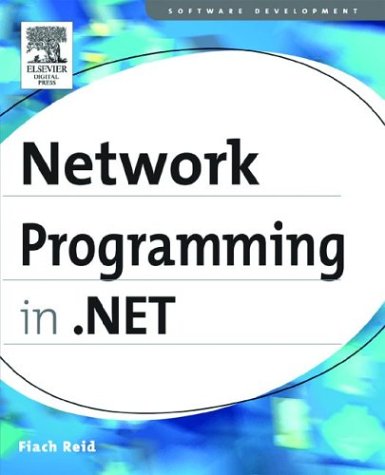
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PhonemeEventArgs.cs
- HTMLTextWriter.cs
- TextAdaptor.cs
- NativeMethods.cs
- LogicalExpressionEditor.cs
- RootContext.cs
- DependencyObjectPropertyDescriptor.cs
- CodeDefaultValueExpression.cs
- OdbcStatementHandle.cs
- WindowsUpDown.cs
- HttpServerVarsCollection.cs
- Form.cs
- PriorityBindingExpression.cs
- ShimAsPublicXamlType.cs
- CancellationTokenRegistration.cs
- Html32TextWriter.cs
- ParallelEnumerable.cs
- TraceUtils.cs
- HtmlTable.cs
- TransformConverter.cs
- URL.cs
- Base64Decoder.cs
- RenderData.cs
- Line.cs
- RegexReplacement.cs
- NotifyInputEventArgs.cs
- InputGestureCollection.cs
- WindowsListViewGroupSubsetLink.cs
- TransformerInfo.cs
- storepermissionattribute.cs
- Container.cs
- EnumerableWrapperWeakToStrong.cs
- SID.cs
- XmlDocumentType.cs
- DataMisalignedException.cs
- AdornerHitTestResult.cs
- MimeMapping.cs
- Stack.cs
- ForwardPositionQuery.cs
- UInt32Storage.cs
- PropertySegmentSerializationProvider.cs
- EmptyImpersonationContext.cs
- CacheSection.cs
- MetadataArtifactLoader.cs
- EventMappingSettings.cs
- WindowsListViewGroupSubsetLink.cs
- DocumentSchemaValidator.cs
- TransformerTypeCollection.cs
- FileInfo.cs
- RemoteWebConfigurationHostServer.cs
- DBCommandBuilder.cs
- ToolStripCustomTypeDescriptor.cs
- SecurityManager.cs
- DetailsViewRowCollection.cs
- CustomError.cs
- SQLInt16.cs
- TextBox.cs
- StructuredProperty.cs
- WebScriptEnablingElement.cs
- HostingEnvironmentSection.cs
- WinCategoryAttribute.cs
- NotifyInputEventArgs.cs
- ToolStripDropTargetManager.cs
- XPathNodeIterator.cs
- WebBrowserNavigatingEventHandler.cs
- SqlUtils.cs
- MSAAEventDispatcher.cs
- ColorConvertedBitmap.cs
- ImagingCache.cs
- StackBuilderSink.cs
- DateTimeFormat.cs
- EndOfStreamException.cs
- EdmMember.cs
- AddInToken.cs
- FeatureSupport.cs
- SortAction.cs
- Point.cs
- ConfigXmlDocument.cs
- File.cs
- DataListItemCollection.cs
- FontSizeConverter.cs
- LinqDataSource.cs
- BevelBitmapEffect.cs
- OleDbDataReader.cs
- MessageContractImporter.cs
- DispatcherTimer.cs
- SystemParameters.cs
- DispatcherHookEventArgs.cs
- ContainerAction.cs
- DataBindingCollectionConverter.cs
- SizeF.cs
- PropVariant.cs
- CodeNamespaceImport.cs
- SimpleWebHandlerParser.cs
- DoubleStorage.cs
- PropertyDescriptorCollection.cs
- CodeRemoveEventStatement.cs
- HttpDebugHandler.cs
- TemplateContentLoader.cs
- WhiteSpaceTrimStringConverter.cs