Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / RMPublishingDialog.cs / 1 / RMPublishingDialog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // RMPublishingDialog is the RM publishing dialog. // // History: // 08/01/05 - [....] created // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Stops compiler from warning about unknown warnings using Microsoft.Win32; using MS.Internal.Permissions; using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Drawing; using System.Globalization; using System.IO; using System.Security; using System.Security.Permissions; using System.Security.RightsManagement; using System.Text; using System.Text.RegularExpressions; using System.Windows; using System.Windows.Forms; using System.Windows.TrustUI; using MS.Internal.Documents.Application; namespace MS.Internal.Documents { ////// The publishing dialog. /// internal sealed partial class RMPublishingDialog : DialogBaseForm { //----------------------------------------------------- // Constructors //----------------------------------------------------- #region Constructors ////// Creates a new Publishing dialog for the given user with the given list of /// already granted rights. /// /// A dictionary of rights already granted on the /// document. This should be null if the document is not protected, and an /// empty dictionary if it is but no grants exist. ////// Critical - 1) A user object that contains critical information is passed in /// as an argument, but it is not saved except as a string to /// display in the UI. /// 2) The critical data _rmLicenses is initialized from the grant /// dictionary passed in as an argument. /// [SecurityCritical] internal RMPublishingDialog( RightsManagementUser user, IDictionarygrantDictionary) { checkBoxValidUntil.Checked = false; datePickerValidUntil.Enabled = false; // The DateTimePicker displays validity dates in the local time // zone, so the date displayed is not necessarily the value that is // returned by the ValidUntil property. datePickerValidUntil.MinDate = DateTime.Today.AddDays(1); datePickerValidUntil.Value = DateTime.Today.AddDays(1); textBoxPermissionsContact.Enabled = true; checkBoxPermissionsContact.Checked = true; textBoxPermissionsContact.Text = user.Name; // Check if referral info is already specified in a license if (grantDictionary != null && grantDictionary.Count != 0) { IEnumerator licenseEnumerator = grantDictionary.Values.GetEnumerator(); licenseEnumerator.MoveNext(); Uri referralUri = licenseEnumerator.Current.ReferralInfoUri; string address = AddressUtility.GetEmailAddressFromMailtoUri(referralUri); // If there was an address previously specified, use it if (!string.IsNullOrEmpty(address)) { textBoxPermissionsContact.Text = address; } // Otherwise disable the checkbox else { checkBoxPermissionsContact.Checked = false; } } if (grantDictionary != null) { _isAlreadyProtected = true; radioButtonPermissions.Checked = true; _rmLicenses = new List (grantDictionary.Values); DateTime validUntil = DateTime.MaxValue; foreach (RightsManagementLicense license in grantDictionary.Values) { if (license.ValidUntil > DateTime.UtcNow && license.ValidUntil < validUntil) { validUntil = license.ValidUntil; } } DateTime localValidUntil = DateTimePicker.MaximumDateTime; // Convert the UTC time to local time if necessary if (validUntil < DateTime.MaxValue) { localValidUntil = validUntil.ToLocalTime(); } if (localValidUntil < DateTimePicker.MaximumDateTime) { checkBoxValidUntil.Checked = true; datePickerValidUntil.Enabled = true; datePickerValidUntil.Value = localValidUntil; } } rightsTable.InitializeRightsTable(user.Name, grantDictionary); UpdateAnyoneEnabled(); // Determine the list of available templates. InitializeTemplates(); // Add templates to the UI (ComboBox). PopulateTemplateUI(); // Attach our event handlers for the Save/SaveAs buttons here. // We do this here so that the event handlers can be Critical (and not TAS) to prevent // them from being invoked in other ways... buttonSave.Click += new System.EventHandler(this.buttonSave_Click); buttonSaveAs.Click += new System.EventHandler(this.buttonSaveAs_Click); // Update the radiobuttons to their initial state, which will also toggle the // Save buttons appropriately. UpdateRadioButtonState(); // check if we can save the document with the current filename _canSave = false; DocumentManager documentManager = DocumentManager.CreateDefault(); if (documentManager != null) { _canSave = documentManager.CanSave; } buttonSave.Enabled = _canSave; } #endregion Constructors //------------------------------------------------------ // Internal Properties //----------------------------------------------------- #region Internal Properties /// /// A list of all the RM licenses to grant for this document. /// ////// Critical - 1) This function returns critical data including e-mail addresses /// of users to whom permissions are granted and the permissions /// granted to each user. /// 2) It accesses the critical data stored in _rmLicenses. /// internal IListLicenses { [SecurityCritical] get { return (radioButtonPermissions.Checked) ? _rmLicenses : null; } } /// /// The time until when the protection on the document is valid, in the /// UTC time zone. /// internal DateTime? ValidUntil { get { if (checkBoxValidUntil.Checked) { return datePickerValidUntil.Value.ToUniversalTime(); } else { return null; } } } ////// The address to contact for more permissions on the document. This /// may return null if none was specified. /// ////// Critical - 1) Returns private contact information (e-mail address) for users /// internal Uri ReferralUri { [SecurityCritical] get { return _referralUri; } } ////// Indicates the type of Save operation chosen. /// internal bool IsSaveAs { get { return _isSaveAs; } } ////// The uri of the currently selected template. /// Will be null if no template is currently selected. /// ////// Critical /// 1) Accesses SecurityCritical field _templates. /// internal Uri Template { [SecurityCritical] get { // Check if a valid template was selected (we assume that the first template // is a placeholder for "" if (radioButtonTemplate.Checked && (comboBoxTemplates.SelectedIndex < _templates.Count)) { return _templates[comboBoxTemplates.SelectedIndex].Template; } else { // Since template was not found return the empty string. return null; } } } #endregion Internal Properties //------------------------------------------------------ // Private Methods //------------------------------------------------------ #region Private Methods /// /// Generates RightsManagementLicense objects for each grant specified by /// the user. /// ///A list of RightsManagementLicense objects ////// Critical /// 1) Uses SecurityCritical setter properties to set up the /// RightsManagementLicense. This is safe because we are setting /// properties of the license based on direct user input. The /// user input is entered in a WinForms dialog that can't be /// tampered with from code that is running in Partial Trust and /// Avalon based. /// 2) Calls Critical function GetUsernameFromRow to determine the /// username from the UI data. /// 3) Calls Critical function GetUserFromUserName to create the user /// objects representing each row in the table. /// 4) Calls Critical function GetPermissionsFromRow. The data /// returned from this critical call is protected for set. /// 5) Returns critical data - user names and permissions granted - /// corresponding to data entered in the dialog. /// [SecurityCritical] private IListCreateRightsManagementLicenses() { List licenseList = new List (); // Enumerate through all the rows that correspond with users foreach (DataGridViewRow row in rightsTable.Rows) { if (row != null) { RightsManagementLicense rmLicense = new RightsManagementLicense(); string userName = RightsTable.GetUsernameFromRow(row); rmLicense.LicensedUser = GetUserFromUserName(userName); rmLicense.LicensePermissions = RightsTable.GetPermissionsFromRow(row); rmLicense.ValidFrom = DateTime.MinValue; rmLicense.ValidUntil = DateTime.MaxValue; if (ReferralUri != null) { rmLicense.ReferralInfoName = textBoxPermissionsContact.Text; rmLicense.ReferralInfoUri = ReferralUri; } // If the user for the current license has not been given // owner rights, and the dialog contains an expiration // date, set the ValidFrom and ValidUntil dates in the // license from the dialog if (!rmLicense.HasPermission(RightsManagementPermissions.AllowOwner) && ValidUntil.HasValue) { rmLicense.ValidFrom = DateTime.UtcNow; rmLicense.ValidUntil = ValidUntil.Value; } licenseList.Add(rmLicense); } } return licenseList; } /// /// Gets the template path from the registry and builds a list of /// ServerSideTemplates. /// ////// Critical /// 1) Calls GetTemplatePath and sets _templatePath. /// 2) Calls GetXmlTemplates and sets _templates. /// TreatAsSafe /// 1) GetTemplatePath is used to acquire a value directly from the registry /// and the result is stored in _templatePath. Since the value is acquired /// directly and _templatePath is marked SecurityCritical this is safe. /// 2) GetXmlTemplates is used to acquire a list of templates from the file /// system. Since the list is then converted to a ReadOnlyCollection, /// and stored in a SecurityCritical field, _templates, this is safe. /// [SecurityCritical, SecurityTreatAsSafe] private void InitializeTemplates() { Listtemplates = null; // Get the template path setting from the registry. _templatePath = GetTemplatePath(); // Get the template file paths // This method will return an empty list if the path is invalid. templates = GetXmlTemplates(); // Insert the default case, templates.Insert(0, new ServerSideTemplate(null)); // Assign ReadOnlyCollection _templates = new ReadOnlyCollection (templates); } /// /// Sets up the ComboBox with data and handlers. /// ////// Critical /// 1) Accessing SecurityCritical field _templates /// TreatAsSafe /// 1) Assigning _templates to be displayed in the UI. /// [SecurityCritical, SecurityTreatAsSafe] private void PopulateTemplateUI() { // Set the template list as the ComboBox source. comboBoxTemplates.DataSource = _templates; // Add a handler to the SelectedValueChanged event to ensure that // the correct controls are enabled/disabled depending on whether // a template has been selected. comboBoxTemplates.SelectedValueChanged += new EventHandler(comboBox1_SelectedValueChanged); // Setup initial index value. comboBox1_SelectedValueChanged(null, null); } ////// Used to get the value of HKCU\Software\Microsoft\XPSViewer\Common\DRM\AdminTemplatePath /// ///The contents of the key, otherwise string.Empty. ////// Critical /// 1) Asserts for the RegistryPermission to determine if the requested /// key's value is available. /// [SecurityCritical] private Uri GetTemplatePath() { string path = string.Empty; (new RegistryPermission( RegistryPermissionAccess.Read, _registryBaseForRMTemplates)) .Assert(); // BlessedAssert try { using (RegistryKey defaultRMKey = Registry.CurrentUser.OpenSubKey( _registryLocationForRMTemplates)) { // Ensure key exists if (defaultRMKey != null) { // If the requested key is available then attempt to get the value. object keyValue = defaultRMKey.GetValue(_registryValueNameForRMTemplatePath); if ((keyValue != null) && (defaultRMKey.GetValueKind(_registryValueNameForRMTemplatePath) == RegistryValueKind.String)) { path = (string)keyValue; } } } } finally { RegistryPermission.RevertAssert(); } Uri newUri = null; // Attempt to build a new Uri of the found path value. if (!string.IsNullOrEmpty(path)) { try { newUri = new Uri(path); } catch (UriFormatException e) { // The two exceptions we could have are UriFormatException and // ArgumentNullException. Since we've validated against ArgumentNullException // we only have to catch UriFormatException here. This will silently catch // the exception and fail to create a Uri (or fill in UI template values). Trace.SafeWrite( Trace.Rights, "Exception Hit: {0}", e); } } return newUri; } ////// Builds a list of ServerSideTemplates based on any xml files found in the /// _templatePath directory. /// ///Collection of ServerSideTemplates, each one representing a /// template found in the _templatePath directory. ////// Critical /// 1) Asserts for FileIOPermission to get the list of files. /// 2) Accesses SecurityCritical field _templatePath /// 2) Calls ServerSideTemplate.BuildTemplateList /// [SecurityCritical] private ListGetXmlTemplates() { List templateList = new List (); // Only look for files if a path was found in the registry. if (_templatePath != null) { // Save the path local to this method to guarantee it does not // change between the assert and the file access string templateLocalPath = _templatePath.LocalPath; // Assert for directory listing. // FileIOPermissionAccess.Read required for file access // FileIOPermissionAccess.PathDiscovery required for file listing. (new FileIOPermission( FileIOPermissionAccess.Read | FileIOPermissionAccess.PathDiscovery, templateLocalPath)) .Assert(); try { DirectoryInfo dir = new DirectoryInfo(templateLocalPath); // Ensure that the required path exists. if (dir.Exists) { // Add each .xml file into the list foreach (FileInfo file in dir.GetFiles("*.xml")) { templateList.Add(new Uri(file.FullName)); } } } // Any exception above represents a failure to load templates. // Failing to load them is not a fatal error, so we can safely // continue with an empty list. #pragma warning suppress 56500 // suppress PreSharp Warning 56500: Avoid `swallowing errors by catching non-specific exceptions.. catch (Exception e) { // Nothing should be done here, as we purposely catch all exceptions. // If a directory fails to open, either from a system problem, or // the registry value being set to garbage we should just fail // to find a value (or populate the list) silently. Trace.SafeWrite( Trace.Rights, "Exception Hit: {0}", e); } finally { FileIOPermission.RevertAssert(); } } return ServerSideTemplate.BuildTemplateList(templateList); } /// /// Gets a User object from a user's name. /// /// The name of the user. ///A User object corresponding to the name ////// Critical /// 1) Calling SecurityCritical function CreateUser /// [SecurityCritical] private static RightsManagementUser GetUserFromUserName(string userName) { if (RightsTable.IsEveryone(userName)) { return RightsManagementUser.AnyoneRightsManagementUser; } else { // XPS Viewer doesn't allow document authors to explicitly define the authentication type of the consumers. // So we are using WindowsPassport Authentication type as means of enabling both Passport and Windows // consumers return RightsManagementUser.CreateUser( userName, AuthenticationType.WindowsPassport); } } ////// Validates the Referral address entered into the dialog and prompts /// the user if the address is invalid. If the address is valid, /// _referralUri is updated. /// /// ////// SecurityCritical: Modifies critical _referralUri field. /// ///A bool reflecting the validity of the address. [SecurityCritical] private bool ValidateReferralAddress() { bool ok = true; _referralUri = null; // If the permissions contact check box is checked, parse the // referral information to make a mailto URI. if (checkBoxPermissionsContact.Checked) { string permissionsAddress = textBoxPermissionsContact.Text.Trim(); if (!string.IsNullOrEmpty(permissionsAddress)) { _referralUri = AddressUtility.GenerateMailtoUri(permissionsAddress); ok = true; } // If no e-mail address was entered, we should show an // error dialog box and not let the form be closed else { System.Windows.MessageBox.Show( string.Format( CultureInfo.CurrentCulture, SR.Get(SRID.RightsManagementWarnErrorNoReferralAddress), textBoxPermissionsContact.Text), SR.Get(SRID.RightsManagementWarnErrorTitle), MessageBoxButton.OK, MessageBoxImage.Exclamation); textBoxPermissionsContact.Focus(); textBoxPermissionsContact.SelectAll(); ok = false; } } return ok; } ////// Sets up a button on which an icon will be displayed, including all /// styling to apply to the button. /// /// The button /// The icon to put on the button /// The text associated with the button private void SetupIconButton(Button button, Icon icon, string text, string tooltip) { ImageList images = new ImageList(); // The button is square, so the padding will be the same both // horizontally and vertically. int totalPadding = button.Padding.Horizontal + button.Margin.Horizontal; // Add one pixel of padding to ensure the image is not cut off by // the Windows button bevel totalPadding++; // Calculate the image size by subtracting the padding from the // size of the whole button images.ImageSize = System.Drawing.Size.Subtract( button.Size, new System.Drawing.Size(totalPadding, totalPadding)); // Ensure the highest color icon is selected images.ColorDepth = ColorDepth.Depth32Bit; // Set the button to use the icon images.Images.Add(icon); button.ImageList = images; button.ImageIndex = 0; // Buttons with icons should look just like the icon image (with no // border or beveling). When the user focuses on it using Tab or // mouses over it, the button's beveling and border should return // until the user leaves the button again. // Set the button to appear flat with no border button.FlatStyle = FlatStyle.Flat; button.FlatAppearance.BorderSize = 0; // Set up event handlers to change the button's style when it is // focused or the mouse hovers over it button.MouseEnter += new EventHandler(OnIconButtonEnter); button.GotFocus += new EventHandler(OnIconButtonEnter); button.MouseLeave += new EventHandler(OnIconButtonLeave); button.LostFocus += new EventHandler(OnIconButtonLeave); // Since the button has no text, we need to set the AccessibleName button.AccessibleName = text; // Set the button's tooltip text. _toolTip.SetToolTip(button, tooltip); } ////// Invoked when a setting is changed; this will enable/disable the Save/SaveAs buttons /// so that the settings may be committed to disk. /// /// A bool value used to determine the state of save private void ToggleSave(bool isSaveAllowed) { buttonSave.Enabled = isSaveAllowed && _canSave; buttonSaveAs.Enabled = isSaveAllowed; } ////// Handler for grid selection change -- enables/disables the Remove button /// as appropriate. /// /// Event sender (not used) /// Event arguments (not used) private void rightsTable_SelectionChanged(object sender, System.EventArgs e) { bool allowRemove = false; // make sure that an item is selected if (rightsTable.SelectedRows.Count == 1) { // retrieve the selected row DataGridViewRow row = rightsTable.SelectedRows[0]; // if the row's index is greater than 0, then (and only then) allow remove // (the owner, who can't be removed, is at index 0) if (row.Index > 0) { allowRemove = true; } } buttonRemoveUser.Enabled = allowRemove; } ////// Handler for the "restrict permissions" radio buttons change event. /// /// Event sender (not used) /// Event arguments (not used) private void radioButton_CheckedChanged(object sender, System.EventArgs e) { UpdateRadioButtonState(); } ////// Rotate the visible pane as appropriate. Toggle the Save/SaveAs buttons depending /// on the current radio button selection. /// private void UpdateRadioButtonState() { if (this.radioButtonUnrestricted.Checked) { flowLayoutPanelUnrestricted.Visible = true; flowLayoutPanelPermissions.Visible = false; flowLayoutPanelTemplate.Visible = false; // If the document was already protected allow the owner unrestrict // the permissions. ToggleSave(_isAlreadyProtected); } else if (this.radioButtonPermissions.Checked) { flowLayoutPanelUnrestricted.Visible = false; flowLayoutPanelPermissions.Visible = true; flowLayoutPanelTemplate.Visible = false; ToggleSave(true); } else if (this.radioButtonTemplate.Checked) { flowLayoutPanelUnrestricted.Visible = false; flowLayoutPanelPermissions.Visible = false; flowLayoutPanelTemplate.Visible = true; // Enable save buttons if a template is selected. ToggleSave(comboBoxTemplates.SelectedIndex > 0); } } ////// Handler for the "valid until" checkbox change event. /// /// Event sender (not used) /// Event arguments (not used) private void checkBoxValidUntil_CheckedChanged(object sender, EventArgs e) { datePickerValidUntil.Enabled = checkBoxValidUntil.Checked; } ////// Handler for the "request permissions from" checkbox change event. /// /// Event sender (not used) /// Event arguments (not used) private void checkBoxPermissionsContact_CheckedChanged(object sender, EventArgs e) { textBoxPermissionsContact.Enabled = checkBoxPermissionsContact.Checked; } ////// Handler for when the Add User button is clicked. /// /// Event sender (not used) /// Event arguments (not used) ////// Critical /// 1) Asserts for UI permission to change TextBoxes focus state. /// TreatAsSafe /// 1) Assert is limited to TextBox.Focus which only alters the current /// UI focus, and does not affect data. /// [SecurityCritical, SecurityTreatAsSafe] private void buttonAddUser_Click(object sender, EventArgs e) { string userNames = textBoxUserName.Text; string[] userNameArray = null; if (string.IsNullOrEmpty(userNames)) { System.Windows.MessageBox.Show( SR.Get(SRID.RightsManagementWarnErrorNoAddress), SR.Get(SRID.RightsManagementWarnErrorTitle), MessageBoxButton.OK, MessageBoxImage.Exclamation); } userNameArray = userNames.Split( SR.Get(SRID.RMPublishingUserSeparator).ToCharArray(), StringSplitOptions.RemoveEmptyEntries); foreach (string userName in userNameArray) { string trimmedName = userName.Trim(); if (!string.IsNullOrEmpty(trimmedName)) { rightsTable.AddUser(new RightsTableUser(trimmedName)); } } // Clear the text box so the user can enter a new username. textBoxUserName.Clear(); (new UIPermission(UIPermissionWindow.AllWindows)) .Assert(); // BlessedAssert try { textBoxUserName.Focus(); } finally { UIPermission.RevertAssert(); } UpdateAnyoneEnabled(); } ////// Handler for when the People Picker button is clicked. /// ////// Critical: Calls SecurityCritical code (PeoplePickerWrapper.Show) /// TreatAsSafe: This call can be considered safe; the Show method /// invokes unmanaged code to display the Active Directory /// "People Picker" dialog and returns a selected user's /// e-mail address which is then displayed in the /// publishing dialog's username entry textbox. /// /// Event sender (not used) /// Event arguments (not used) [SecurityCritical, SecurityTreatAsSafe] private void buttonPeoplePicker_Click(object sender, EventArgs e) { //Invoke the People Picker, which should return a user-selected //e-mail address or an empty array if nothing was selected. PeoplePickerWrapper picker = new PeoplePickerWrapper(); String[] userNames = picker.Show(this.Handle); if (userNames != null && userNames.Length > 0) { textBoxUserName.Text = string.Join( SR.Get(SRID.RMPublishingUserSeparator), userNames); } } ////// Handler for when the Anyone button is clicked. /// /// Event sender (not used) /// Event arguments (not used) private void buttonEveryone_Click(object sender, EventArgs e) { rightsTable.AddUser(new RightsTableUser(SR.Get(SRID.RMPublishingAnyoneUserDisplay))); UpdateAnyoneEnabled(); } ////// Handler for when the remove button is clicked. It removes the row of the /// sender from the table /// /// Event sender (button clicked) /// Event arguments (not used) private void buttonRemoveUser_Click(object sender, EventArgs e) { rightsTable.DeleteUser(); UpdateAnyoneEnabled(); } ////// Handler for when the Save button is clicked. /// ////// SecurityCritical : Calls SecurityCritical Methods: /// - ValidateReferralAddress() /// - CreateRightsManagementLicenses() /// /// Touches SecurityCritical field _rmLicenses /// /// /// Event sender (not used) /// Event arguments (not used) [SecurityCritical] private void buttonSave_Click(object sender, EventArgs e) { // Check the Referral Address, and if it's OK we can continue to // set the IsSaveAs flag and close the dialog. if (ValidateReferralAddress()) { _rmLicenses = CreateRightsManagementLicenses(); DialogResult = DialogResult.OK; _isSaveAs = false; this.Close(); } } ////// Handler for when the Save As button is clicked. /// ////// SecurityCritical : Calls SecurityCritical Methods: /// - ValidateReferralAddress() /// - CreateRightsManagementLicenses() /// /// Touches SecurityCritical field _rmLicenses /// /// /// Event sender (not used) /// Event arguments (not used) [SecurityCritical] private void buttonSaveAs_Click(object sender, EventArgs e) { // Check the Referral Address, and if it's OK we can continue to // set the IsSaveAs flag and close the dialog. if (ValidateReferralAddress()) { _rmLicenses = CreateRightsManagementLicenses(); DialogResult = DialogResult.OK; _isSaveAs = true; this.Close(); } } ////// Handler for when the user name text box gains focus. This sets the dialog's /// accept button to the Add User button. /// /// Event sender (not used) /// Event arguments (not used) private void textBoxUserName_GotFocus(object sender, System.EventArgs e) { this.AcceptButton = buttonAddUser; } ////// Handler for when the user name text box loses focus. This sets the dialog's /// accept button to the Save button. /// /// Event sender (not used) /// Event arguments (not used) private void textBoxUserName_LostFocus(object sender, System.EventArgs e) { this.AcceptButton = buttonSaveAs; } ////// Used to enable/disable controls based on the selected template. /// /// Event sender (not used) /// Event arguments (not used) private void comboBox1_SelectedValueChanged(object sender, EventArgs e) { ToggleSave(comboBoxTemplates.SelectedIndex > 0); } ////// Sets the sending button's style to Standard when it is focused or /// moused over. /// /// The button /// Event args (not used) private static void OnIconButtonEnter(object sender, EventArgs e) { Button button = sender as Button; if (button != null) { button.FlatStyle = FlatStyle.Standard; } } ////// Sets the sending button's style to the Flat style when tab or mouse /// focus leaves. /// /// The button /// Event args (not used) private static void OnIconButtonLeave(object sender, EventArgs e) { Button button = sender as Button; if (button != null) { button.FlatStyle = FlatStyle.Flat; } } ////// Enables or disables the Everyone button depending on whether Everyone /// is already in the table. /// private void UpdateAnyoneEnabled() { buttonEveryone.Enabled = !rightsTable.AnyoneUserPresent; } #endregion Private Methods //----------------------------------------------------- // Private Properties //------------------------------------------------------ #region Private Properties #endregion Private Properties //----------------------------------------------------- // Private Fields //----------------------------------------------------- #region Private Fields ////// The list of licenses represented in this dialog /// ////// Critical - This contains a list of all users to whom rights were granted /// as well as the rights granted. /// [SecurityCritical] private IList_rmLicenses; /// /// The URI to contact for permissions. /// ////// Critical - Personally identifiable information /// [SecurityCritical] private Uri _referralUri; ////// A bool indicating the type of Save operation chosen. /// private bool _isSaveAs; ////// A bool indicating if the document was protected before it was loaded this time. /// private bool _isAlreadyProtected; ////// The path of the templates, as defined in the registry. /// ////// Critical - value is set with information obtained under elevation. /// [SecurityCritical] private Uri _templatePath; ////// List of files which are to be considered valid templates. /// ////// Critical - System information that should only be displayed by /// trusted sources. /// [SecurityCritical] private ReadOnlyCollection_templates; /// /// Critical /// 1) Data is used in elevation. Untrusted callers should not be able /// to change the value of this object. /// /// TreatAsSafe /// 1) Marked critical only to audit changes. /// [SecurityCritical, SecurityTreatAsSafe] private const string _registryBaseForRMTemplates = @"HKEY_CURRENT_USER\Software\Microsoft\XPSViewer\Common\DRM\"; ////// Critical /// 1) Data is used in elevation. Untrusted callers should not be able /// to change the value of this object. /// /// TreatAsSafe /// 1) Marked critical only to audit changes. /// [SecurityCritical, SecurityTreatAsSafe] private const string _registryLocationForRMTemplates = @"Software\Microsoft\XPSViewer\Common\DRM"; ////// Critical /// 1) Data is used in elevation. Untrusted callers should not be able /// to change the value of this object. /// /// TreatAsSafe /// 1) Marked critical only to audit changes. /// [SecurityCritical, SecurityTreatAsSafe] private const string _registryValueNameForRMTemplatePath = "AdminTemplatePath"; ////// Indicates whether you can can save replacing the current file. /// This is false for web based documents. /// private bool _canSave = false; #endregion Private Fields //------------------------------------------------------ // Protected Methods //------------------------------------------------------ #region Protected Methods ////// ApplyResources override. Called to apply dialog resources. /// protected override void ApplyResources() { base.ApplyResources(); Text = SR.Get(SRID.RMPublishingTitle); radioButtonUnrestricted.Text = SR.Get(SRID.RMPublishingUnrestrictedRadio); radioButtonPermissions.Text = SR.Get(SRID.RMPublishingPermissionsRadio); radioButtonTemplate.Text = SR.Get(SRID.RMPublishingTemplateRadio); groupBoxMainContent.Text = SR.Get(SRID.RMPublishingMainContentGroupLabel); textBoxUnrestrictedText.Text = SR.Get(SRID.RMPublishingUnrestrictedText); labelSelectTemplate.Text = SR.Get(SRID.RMPublishingSelectTemplate); // Set the text for the Add User button first so we can use it to // calculate the size of the image buttons buttonAddUser.Text = SR.Get(SRID.RMPublishingAddUserButton); // Each of the image buttons is a square, and the height will be // the same as the text button System.Drawing.Size imageButtonSize = new System.Drawing.Size( buttonAddUser.Size.Height, buttonAddUser.Size.Height); // Set the Add User button's tooltip _toolTip.SetToolTip(buttonAddUser, SR.Get(SRID.RMPublishingAddUserButtonToolTip)); buttonPeoplePicker.Size = imageButtonSize; buttonEveryone.Size = imageButtonSize; buttonRemoveUser.Size = imageButtonSize; // Set the button icons and text SetupIconButton( buttonPeoplePicker, Resources.RMPublishingPeoplePicker, SR.Get(SRID.RMPublishingPeoplePickerButton), SR.Get(SRID.RMPublishingPeoplePickerButtonToolTip)); SetupIconButton( buttonEveryone, Resources.RMPublishingEveryone, SR.Get(SRID.RMPublishingAnyoneButton), SR.Get(SRID.RMPublishingAnyoneButtonToolTip)); SetupIconButton( buttonRemoveUser, Resources.RMPublishingRemove, SR.Get(SRID.RMPublishingRemoveButton), SR.Get(SRID.RMPublishingRemoveButtonToolTip)); checkBoxValidUntil.Text = SR.Get(SRID.RMPublishingExpiresOn); checkBoxPermissionsContact.Text = SR.Get(SRID.RMPublishingRequestPermissionsFrom); buttonSave.Text = SR.Get(SRID.RMPublishingSaveButton); _toolTip.SetToolTip(buttonSave, SR.Get(SRID.RMPublishingSaveButtonToolTip)); buttonSaveAs.Text = SR.Get(SRID.RMPublishingSaveAsButton); _toolTip.SetToolTip(buttonSaveAs, SR.Get(SRID.RMPublishingSaveAsButtonToolTip)); buttonCancel.Text = SR.Get(SRID.RMPublishingCancelButton); } #endregion Protected Methods //------------------------------------------------------ // Nested Classes //------------------------------------------------------ #region Nested Classes //------------------------------------------------------ // // ServerSideTemplate // //------------------------------------------------------ ////// Represents a template to be displayed in the UI. /// private class ServerSideTemplate { ////// Constructor. /// /// Reference to the template file. If null thecase /// will be used. /// /// Critical /// 1) Creates a ServerSideTemplate, which contains file system data. /// [SecurityCritical] public ServerSideTemplate(Uri filename) { _template = filename; } ////// Gets the actual full filename to the template. /// ////// Critical /// 1) Provides access to the Uri of the template file. /// public Uri Template { [SecurityCritical] get { return _template; } } ////// Used to determine what to display in the UI. /// ///Returns the name of the template (without path or extension). ////// Critical /// 1) Accesses SecurityCritical _template field. /// TreatAsSafe /// 2) Removes all path and extension information from the Uri /// and only provides the filename for the UI. /// (This is needed for databinding). /// [SecurityCritical, SecurityTreatAsSafe] public override string ToString() { if (_template == null) { return SR.Get(SRID.ServerSideTemplateDisplayNone); } // This will remove the path and extension information to make // a screen readable string. Here we use LocalPath instead of // AbsolutePath since its result is unescaped (only the filename // is used so the rest of the path doesn't matter). return Path.GetFileNameWithoutExtension(_template.LocalPath); } ////// Builds a List of ServerSideTemplate's from a list of Uri file references. /// /// List of files to match. ///The list of ServerSideTemplates ////// Critical /// 1) Calls the ServerSideTemplate constructor. /// [SecurityCritical] public static ListBuildTemplateList(List files) { List templates = new List (); if (files != null) { foreach (Uri file in files) { templates.Add(new ServerSideTemplate(file)); } } return templates; } #region Private Fields /// /// Critical /// 1) Provides access to the Uri of the template file. /// [SecurityCritical] private Uri _template; #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
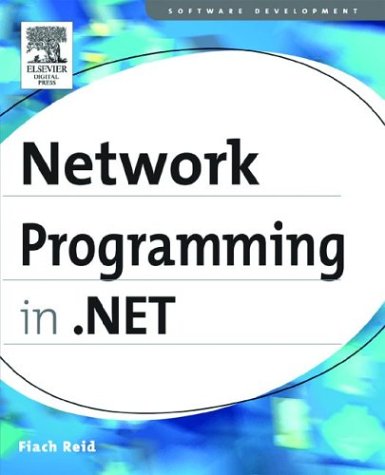
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapReflector.cs
- ToolStripGripRenderEventArgs.cs
- ExplicitDiscriminatorMap.cs
- SafeEventHandle.cs
- processwaithandle.cs
- VirtualPath.cs
- UrlPath.cs
- FixUpCollection.cs
- WebBaseEventKeyComparer.cs
- Cursor.cs
- ValidationContext.cs
- Convert.cs
- HMACSHA512.cs
- GeometryConverter.cs
- XmlEntityReference.cs
- SEHException.cs
- EventLogger.cs
- RevocationPoint.cs
- infer.cs
- NetworkStream.cs
- SymbolType.cs
- Misc.cs
- LinqDataSourceEditData.cs
- ClockController.cs
- ProgramPublisher.cs
- OleDbErrorCollection.cs
- ColumnCollection.cs
- BoolLiteral.cs
- CommonObjectSecurity.cs
- DrawingContextDrawingContextWalker.cs
- ElementAction.cs
- Mappings.cs
- ObjectRef.cs
- DependencyProperty.cs
- CodeAccessSecurityEngine.cs
- XmlSchemaAny.cs
- X509WindowsSecurityToken.cs
- PropertyConverter.cs
- Pair.cs
- PageStatePersister.cs
- FrameworkContentElement.cs
- DataKey.cs
- RegistryPermission.cs
- StateBag.cs
- ModuleBuilderData.cs
- XpsResourcePolicy.cs
- FigureParaClient.cs
- PluralizationService.cs
- ResourceLoader.cs
- infer.cs
- DbConnectionInternal.cs
- FixedDSBuilder.cs
- GridViewRow.cs
- Variant.cs
- ListItemsCollectionEditor.cs
- BitmapSourceSafeMILHandle.cs
- SessionStateItemCollection.cs
- precedingsibling.cs
- SafeNativeMethods.cs
- WSHttpBinding.cs
- ClientScriptManager.cs
- CodeStatementCollection.cs
- SqlColumnizer.cs
- AliasedSlot.cs
- BuildManagerHost.cs
- XomlCompilerResults.cs
- PolicyVersionConverter.cs
- WindowsGrip.cs
- ControlDesignerState.cs
- EventPrivateKey.cs
- InteropAutomationProvider.cs
- DataExpression.cs
- SqlCharStream.cs
- Subtree.cs
- InstanceValue.cs
- PointAnimationBase.cs
- RSAPKCS1SignatureDeformatter.cs
- TextTreeRootNode.cs
- DiscoveryService.cs
- TheQuery.cs
- RawTextInputReport.cs
- RectValueSerializer.cs
- ImageListStreamer.cs
- Hashtable.cs
- SQLString.cs
- HttpListenerContext.cs
- IconBitmapDecoder.cs
- DrawItemEvent.cs
- SqlServices.cs
- ComAdminWrapper.cs
- OutputCacheProfile.cs
- Message.cs
- DbgUtil.cs
- FlowPanelDesigner.cs
- SrgsRule.cs
- XmlSchemaImport.cs
- ApplicationTrust.cs
- AutomationPropertyInfo.cs
- RC2.cs
- AutomationIdentifierGuids.cs