Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / IO / Packaging / contentDescriptor.cs / 1305600 / contentDescriptor.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Types of keys and data in the element table that is used // by XamlFilter and initialized by the generated function // InitElementDictionary. // // History: // 02/26/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; namespace MS.Internal.IO.Packaging { ////// Representation of a fully-qualified XML name for a XAML element. /// internal class ElementTableKey { ////// Constructor. /// internal ElementTableKey(string xmlNamespace, string baseName) { if (xmlNamespace == null) { throw new ArgumentNullException("xmlNamespace"); } if (baseName == null) { throw new ArgumentNullException("baseName"); } _xmlNamespace = xmlNamespace; _baseName = baseName; } ////// Equality test. /// public override bool Equals( object other ) { if (other == null) return false; // Standard behavior. if (other.GetType() != GetType()) return false; // Note that because of the GetType() checking above, the casting must be valid. ElementTableKey otherElement = (ElementTableKey)other; return ( String.CompareOrdinal(BaseName,otherElement.BaseName) == 0 && String.CompareOrdinal(XmlNamespace,otherElement.XmlNamespace) == 0 ); } ////// Hash on all name components. /// public override int GetHashCode() { return XmlNamespace.GetHashCode() ^ BaseName.GetHashCode(); } ////// XML namespace. /// internal string XmlNamespace { get { return _xmlNamespace; } } ////// Local name. /// internal string BaseName { get { return _baseName; } } private string _baseName; private string _xmlNamespace; public static readonly string XamlNamespace = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"; public static readonly string FixedMarkupNamespace = "http://schemas.microsoft.com/xps/2005/06"; } ///Content-location information for an element. internal class ContentDescriptor { ////// The name of the key to the value of _xamlElementContentDescriptorDictionary in the resource file. /// internal const string ResourceKeyName = "Dictionary"; ////// The name of the resource containing the definition of XamlFilter._xamlElementContentDescriptorDictionary. /// internal const string ResourceName = "ElementTable"; ////// Standard constructor. /// internal ContentDescriptor( bool hasIndexableContent, bool isInline, string contentProp, string titleProp) { HasIndexableContent = hasIndexableContent; IsInline = isInline; ContentProp = contentProp; TitleProp = titleProp; } ////// Constructor with default settings for all but HasIndexableContent. /// ////// Currently, this constructor is always passed false, since in this case the other values are "don't care". /// It would make sense to use it with HasIndexableContent=true, however. /// internal ContentDescriptor( bool hasIndexableContent) { HasIndexableContent = hasIndexableContent; IsInline = false; ContentProp = null; TitleProp = null; } ////// Whether indexable at all. /// ////// ContentDescriptor properties are read-write because at table creation time these properties /// are discovered and stored incrementally. /// internal bool HasIndexableContent { get { return _hasIndexableContent; } set { _hasIndexableContent = value; } } ////// Block or inline. /// internal bool IsInline { get { return _isInline; } set { _isInline = value; } } ////// Attribute in which to find content or null. /// internal string ContentProp { get { return _contentProp; } set { _contentProp = value; } } ////// Attribute in which to find a title rather than the real content. /// internal string TitleProp { get { return _titleProp; } set { _titleProp = value; } } private bool _hasIndexableContent; private bool _isInline; private string _contentProp; private string _titleProp; } } // namespace MS.Internal.IO.Packaging // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Types of keys and data in the element table that is used // by XamlFilter and initialized by the generated function // InitElementDictionary. // // History: // 02/26/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; namespace MS.Internal.IO.Packaging { ////// Representation of a fully-qualified XML name for a XAML element. /// internal class ElementTableKey { ////// Constructor. /// internal ElementTableKey(string xmlNamespace, string baseName) { if (xmlNamespace == null) { throw new ArgumentNullException("xmlNamespace"); } if (baseName == null) { throw new ArgumentNullException("baseName"); } _xmlNamespace = xmlNamespace; _baseName = baseName; } ////// Equality test. /// public override bool Equals( object other ) { if (other == null) return false; // Standard behavior. if (other.GetType() != GetType()) return false; // Note that because of the GetType() checking above, the casting must be valid. ElementTableKey otherElement = (ElementTableKey)other; return ( String.CompareOrdinal(BaseName,otherElement.BaseName) == 0 && String.CompareOrdinal(XmlNamespace,otherElement.XmlNamespace) == 0 ); } ////// Hash on all name components. /// public override int GetHashCode() { return XmlNamespace.GetHashCode() ^ BaseName.GetHashCode(); } ////// XML namespace. /// internal string XmlNamespace { get { return _xmlNamespace; } } ////// Local name. /// internal string BaseName { get { return _baseName; } } private string _baseName; private string _xmlNamespace; public static readonly string XamlNamespace = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"; public static readonly string FixedMarkupNamespace = "http://schemas.microsoft.com/xps/2005/06"; } ///Content-location information for an element. internal class ContentDescriptor { ////// The name of the key to the value of _xamlElementContentDescriptorDictionary in the resource file. /// internal const string ResourceKeyName = "Dictionary"; ////// The name of the resource containing the definition of XamlFilter._xamlElementContentDescriptorDictionary. /// internal const string ResourceName = "ElementTable"; ////// Standard constructor. /// internal ContentDescriptor( bool hasIndexableContent, bool isInline, string contentProp, string titleProp) { HasIndexableContent = hasIndexableContent; IsInline = isInline; ContentProp = contentProp; TitleProp = titleProp; } ////// Constructor with default settings for all but HasIndexableContent. /// ////// Currently, this constructor is always passed false, since in this case the other values are "don't care". /// It would make sense to use it with HasIndexableContent=true, however. /// internal ContentDescriptor( bool hasIndexableContent) { HasIndexableContent = hasIndexableContent; IsInline = false; ContentProp = null; TitleProp = null; } ////// Whether indexable at all. /// ////// ContentDescriptor properties are read-write because at table creation time these properties /// are discovered and stored incrementally. /// internal bool HasIndexableContent { get { return _hasIndexableContent; } set { _hasIndexableContent = value; } } ////// Block or inline. /// internal bool IsInline { get { return _isInline; } set { _isInline = value; } } ////// Attribute in which to find content or null. /// internal string ContentProp { get { return _contentProp; } set { _contentProp = value; } } ////// Attribute in which to find a title rather than the real content. /// internal string TitleProp { get { return _titleProp; } set { _titleProp = value; } } private bool _hasIndexableContent; private bool _isInline; private string _contentProp; private string _titleProp; } } // namespace MS.Internal.IO.Packaging // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
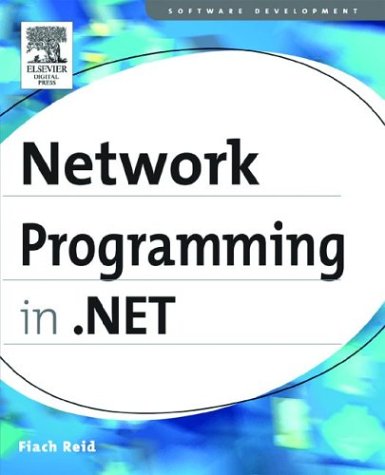
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextParagraphProperties.cs
- NativeMethods.cs
- MsmqInputSessionChannelListener.cs
- XmlSerializerSection.cs
- ComponentEditorPage.cs
- DataRowExtensions.cs
- DataTemplate.cs
- SafeSecurityHandles.cs
- ScriptReference.cs
- ComponentResourceManager.cs
- InvalidContentTypeException.cs
- UserControl.cs
- ArithmeticException.cs
- ConfigurationManagerInternalFactory.cs
- AssemblyNameProxy.cs
- XmlILConstructAnalyzer.cs
- _ServiceNameStore.cs
- DatagridviewDisplayedBandsData.cs
- TagNameToTypeMapper.cs
- EntityTypeBase.cs
- PathFigureCollection.cs
- Composition.cs
- util.cs
- AssemblyNameProxy.cs
- KeyEventArgs.cs
- Attributes.cs
- ScriptResourceInfo.cs
- AutoGeneratedField.cs
- ObjectDataSourceSelectingEventArgs.cs
- Typography.cs
- RepeaterItem.cs
- IChannel.cs
- CodeActivity.cs
- ReadOnlyState.cs
- SystemIPGlobalProperties.cs
- DataGridViewCellStyleConverter.cs
- TargetException.cs
- RegularExpressionValidator.cs
- NegotiateStream.cs
- Pen.cs
- DataGridHyperlinkColumn.cs
- GenericsNotImplementedException.cs
- UnionCqlBlock.cs
- NullableDecimalAverageAggregationOperator.cs
- OleServicesContext.cs
- LineGeometry.cs
- XmlUnspecifiedAttribute.cs
- ProfileGroupSettings.cs
- AxHost.cs
- XamlBrushSerializer.cs
- COM2FontConverter.cs
- XmlSiteMapProvider.cs
- TextProperties.cs
- UriTemplatePathPartiallyEquivalentSet.cs
- CodeLabeledStatement.cs
- Pen.cs
- EnumerableValidator.cs
- DeclarativeCatalogPart.cs
- DbFunctionCommandTree.cs
- TemplateLookupAction.cs
- DeferredRunTextReference.cs
- exports.cs
- CancellationHandler.cs
- PrefixHandle.cs
- WindowsScrollBar.cs
- SymbolMethod.cs
- ExpandSegment.cs
- FontStyle.cs
- Memoizer.cs
- XappLauncher.cs
- PropertyOverridesDialog.cs
- SafeTokenHandle.cs
- InvalidCardException.cs
- SystemInfo.cs
- SqlCacheDependencySection.cs
- FieldCollectionEditor.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- EmptyControlCollection.cs
- SupportingTokenAuthenticatorSpecification.cs
- ObjectPersistData.cs
- ObjectReferenceStack.cs
- UrlMapping.cs
- DecoratedNameAttribute.cs
- LinearGradientBrush.cs
- ArrayConverter.cs
- ZoneMembershipCondition.cs
- CodeTypeReference.cs
- WorkflowServiceBehavior.cs
- OutputCacheProfileCollection.cs
- CertificateElement.cs
- WhileDesigner.xaml.cs
- FileRecordSequenceCompletedAsyncResult.cs
- PointHitTestResult.cs
- XmlSerializer.cs
- BaseTemplatedMobileComponentEditor.cs
- RoleManagerModule.cs
- RangeValidator.cs
- Utility.cs
- TrackingServices.cs
- DataGridRelationshipRow.cs