Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Effects / Generated / DropShadowEffect.cs / 1 / DropShadowEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class DropShadowEffect : Effect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DropShadowEffect Clone() { return (DropShadowEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DropShadowEffect CloneCurrentValue() { return (DropShadowEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ShadowDepthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(ShadowDepthProperty); } private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(ColorProperty); } private static void DirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(DirectionProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(OpacityProperty); } private static void BlurRadiusPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(BlurRadiusProperty); } private static void RenderingBiasPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(RenderingBiasProperty); } #region Public Properties ////// ShadowDepth - double. Default value is 5.0. /// public double ShadowDepth { get { return (double) GetValue(ShadowDepthProperty); } set { SetValueInternal(ShadowDepthProperty, value); } } ////// Color - Color. Default value is Colors.Black. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Direction - double. Default value is 315.0. /// public double Direction { get { return (double) GetValue(DirectionProperty); } set { SetValueInternal(DirectionProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// BlurRadius - double. Default value is 5.0. /// public double BlurRadius { get { return (double) GetValue(BlurRadiusProperty); } set { SetValueInternal(BlurRadiusProperty, value); } } ////// RenderingBias - RenderingBias. Default value is RenderingBias.Performance. /// public RenderingBias RenderingBias { get { return (RenderingBias) GetValue(RenderingBiasProperty); } set { SetValueInternal(RenderingBiasProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DropShadowEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hShadowDepthAnimations = GetAnimationResourceHandle(ShadowDepthProperty, channel); DUCE.ResourceHandle hColorAnimations = GetAnimationResourceHandle(ColorProperty, channel); DUCE.ResourceHandle hDirectionAnimations = GetAnimationResourceHandle(DirectionProperty, channel); DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hBlurRadiusAnimations = GetAnimationResourceHandle(BlurRadiusProperty, channel); // Pack & send command packet DUCE.MILCMD_DROPSHADOWEFFECT data; unsafe { data.Type = MILCMD.MilCmdDropShadowEffect; data.Handle = _duceResource.GetHandle(channel); if (hShadowDepthAnimations.IsNull) { data.ShadowDepth = ShadowDepth; } data.hShadowDepthAnimations = hShadowDepthAnimations; if (hColorAnimations.IsNull) { data.Color = CompositionResourceManager.ColorToMilColorF(Color); } data.hColorAnimations = hColorAnimations; if (hDirectionAnimations.IsNull) { data.Direction = Direction; } data.hDirectionAnimations = hDirectionAnimations; if (hOpacityAnimations.IsNull) { data.Opacity = Opacity; } data.hOpacityAnimations = hOpacityAnimations; if (hBlurRadiusAnimations.IsNull) { data.BlurRadius = BlurRadius; } data.hBlurRadiusAnimations = hBlurRadiusAnimations; data.RenderingBias = RenderingBias; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_DROPSHADOWEFFECT)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_DROPSHADOWEFFECT)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DropShadowEffect.ShadowDepth property. /// public static readonly DependencyProperty ShadowDepthProperty; ////// The DependencyProperty for the DropShadowEffect.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DropShadowEffect.Direction property. /// public static readonly DependencyProperty DirectionProperty; ////// The DependencyProperty for the DropShadowEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the DropShadowEffect.BlurRadius property. /// public static readonly DependencyProperty BlurRadiusProperty; ////// The DependencyProperty for the DropShadowEffect.RenderingBias property. /// public static readonly DependencyProperty RenderingBiasProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_ShadowDepth = 5.0; internal static Color s_Color = Colors.Black; internal const double c_Direction = 315.0; internal const double c_Opacity = 1.0; internal const double c_BlurRadius = 5.0; internal const RenderingBias c_RenderingBias = RenderingBias.Performance; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DropShadowEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(DropShadowEffect); ShadowDepthProperty = RegisterProperty("ShadowDepth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(ShadowDepthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Black, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); DirectionProperty = RegisterProperty("Direction", typeof(double), typeofThis, 315.0, new PropertyChangedCallback(DirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); BlurRadiusProperty = RegisterProperty("BlurRadius", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(BlurRadiusPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); RenderingBiasProperty = RegisterProperty("RenderingBias", typeof(RenderingBias), typeofThis, RenderingBias.Performance, new PropertyChangedCallback(RenderingBiasPropertyChanged), new ValidateValueCallback(System.Windows.Media.Effects.ValidateEnums.IsRenderingBiasValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class DropShadowEffect : Effect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DropShadowEffect Clone() { return (DropShadowEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DropShadowEffect CloneCurrentValue() { return (DropShadowEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ShadowDepthPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(ShadowDepthProperty); } private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(ColorProperty); } private static void DirectionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(DirectionProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(OpacityProperty); } private static void BlurRadiusPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(BlurRadiusProperty); } private static void RenderingBiasPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DropShadowEffect target = ((DropShadowEffect) d); target.PropertyChanged(RenderingBiasProperty); } #region Public Properties ////// ShadowDepth - double. Default value is 5.0. /// public double ShadowDepth { get { return (double) GetValue(ShadowDepthProperty); } set { SetValueInternal(ShadowDepthProperty, value); } } ////// Color - Color. Default value is Colors.Black. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Direction - double. Default value is 315.0. /// public double Direction { get { return (double) GetValue(DirectionProperty); } set { SetValueInternal(DirectionProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } ////// BlurRadius - double. Default value is 5.0. /// public double BlurRadius { get { return (double) GetValue(BlurRadiusProperty); } set { SetValueInternal(BlurRadiusProperty, value); } } ////// RenderingBias - RenderingBias. Default value is RenderingBias.Performance. /// public RenderingBias RenderingBias { get { return (RenderingBias) GetValue(RenderingBiasProperty); } set { SetValueInternal(RenderingBiasProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DropShadowEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hShadowDepthAnimations = GetAnimationResourceHandle(ShadowDepthProperty, channel); DUCE.ResourceHandle hColorAnimations = GetAnimationResourceHandle(ColorProperty, channel); DUCE.ResourceHandle hDirectionAnimations = GetAnimationResourceHandle(DirectionProperty, channel); DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hBlurRadiusAnimations = GetAnimationResourceHandle(BlurRadiusProperty, channel); // Pack & send command packet DUCE.MILCMD_DROPSHADOWEFFECT data; unsafe { data.Type = MILCMD.MilCmdDropShadowEffect; data.Handle = _duceResource.GetHandle(channel); if (hShadowDepthAnimations.IsNull) { data.ShadowDepth = ShadowDepth; } data.hShadowDepthAnimations = hShadowDepthAnimations; if (hColorAnimations.IsNull) { data.Color = CompositionResourceManager.ColorToMilColorF(Color); } data.hColorAnimations = hColorAnimations; if (hDirectionAnimations.IsNull) { data.Direction = Direction; } data.hDirectionAnimations = hDirectionAnimations; if (hOpacityAnimations.IsNull) { data.Opacity = Opacity; } data.hOpacityAnimations = hOpacityAnimations; if (hBlurRadiusAnimations.IsNull) { data.BlurRadius = BlurRadius; } data.hBlurRadiusAnimations = hBlurRadiusAnimations; data.RenderingBias = RenderingBias; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_DROPSHADOWEFFECT)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_DROPSHADOWEFFECT)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DropShadowEffect.ShadowDepth property. /// public static readonly DependencyProperty ShadowDepthProperty; ////// The DependencyProperty for the DropShadowEffect.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DropShadowEffect.Direction property. /// public static readonly DependencyProperty DirectionProperty; ////// The DependencyProperty for the DropShadowEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; ////// The DependencyProperty for the DropShadowEffect.BlurRadius property. /// public static readonly DependencyProperty BlurRadiusProperty; ////// The DependencyProperty for the DropShadowEffect.RenderingBias property. /// public static readonly DependencyProperty RenderingBiasProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_ShadowDepth = 5.0; internal static Color s_Color = Colors.Black; internal const double c_Direction = 315.0; internal const double c_Opacity = 1.0; internal const double c_BlurRadius = 5.0; internal const RenderingBias c_RenderingBias = RenderingBias.Performance; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DropShadowEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(DropShadowEffect); ShadowDepthProperty = RegisterProperty("ShadowDepth", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(ShadowDepthPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.Black, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); DirectionProperty = RegisterProperty("Direction", typeof(double), typeofThis, 315.0, new PropertyChangedCallback(DirectionPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); BlurRadiusProperty = RegisterProperty("BlurRadius", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(BlurRadiusPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); RenderingBiasProperty = RegisterProperty("RenderingBias", typeof(RenderingBias), typeofThis, RenderingBias.Performance, new PropertyChangedCallback(RenderingBiasPropertyChanged), new ValidateValueCallback(System.Windows.Media.Effects.ValidateEnums.IsRenderingBiasValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
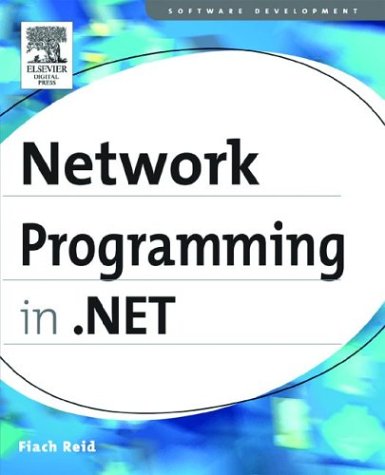
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScriptControl.cs
- SqlTransaction.cs
- Transactions.cs
- DataContractJsonSerializerOperationFormatter.cs
- WsdlInspector.cs
- BitmapCacheBrush.cs
- DataGridViewCheckBoxCell.cs
- XmlException.cs
- ManualWorkflowSchedulerService.cs
- SctClaimSerializer.cs
- LinqMaximalSubtreeNominator.cs
- FixedStringLookup.cs
- StreamUpgradeAcceptor.cs
- SelectionEditingBehavior.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- ForEachAction.cs
- Pair.cs
- DocumentPageHost.cs
- ScopelessEnumAttribute.cs
- SqlBinder.cs
- IntegrationExceptionEventArgs.cs
- OpenFileDialog.cs
- FixedPageAutomationPeer.cs
- NumericExpr.cs
- ArrayList.cs
- AssociationSetEnd.cs
- WriteTimeStream.cs
- EventDescriptor.cs
- Accessible.cs
- ExtensibleClassFactory.cs
- ScrollChrome.cs
- ListViewItem.cs
- CaseStatementSlot.cs
- DiagnosticTraceSource.cs
- TextPointerBase.cs
- DataGridCellAutomationPeer.cs
- CircleHotSpot.cs
- SponsorHelper.cs
- ModelTreeManager.cs
- DesignerWithHeader.cs
- WriteFileContext.cs
- ToolStrip.cs
- Peer.cs
- _SafeNetHandles.cs
- SamlSubject.cs
- SrgsElement.cs
- TextTreePropertyUndoUnit.cs
- SystemColors.cs
- RegexNode.cs
- XmlSchemaDocumentation.cs
- RankException.cs
- CatalogPartChrome.cs
- DataGridViewCellCollection.cs
- Msmq4PoisonHandler.cs
- Events.cs
- CallInfo.cs
- TextComposition.cs
- Merger.cs
- WebConfigurationHost.cs
- GACIdentityPermission.cs
- RoutedCommand.cs
- X509AsymmetricSecurityKey.cs
- XamlVector3DCollectionSerializer.cs
- Size3DConverter.cs
- ModifierKeysValueSerializer.cs
- SerializationInfoEnumerator.cs
- followingsibling.cs
- autovalidator.cs
- HtmlInputPassword.cs
- TreeView.cs
- WindowsTokenRoleProvider.cs
- Control.cs
- HexParser.cs
- RefExpr.cs
- PathTooLongException.cs
- SqlCommandSet.cs
- CalendarSelectionChangedEventArgs.cs
- DataGridViewControlCollection.cs
- XmlEntity.cs
- ResourceSet.cs
- Win32SafeHandles.cs
- CodeObjectCreateExpression.cs
- RightNameExpirationInfoPair.cs
- WinEventQueueItem.cs
- SettingsProperty.cs
- ElementFactory.cs
- MethodRental.cs
- UserControl.cs
- GridViewCellAutomationPeer.cs
- DrawingContextWalker.cs
- TracingConnectionInitiator.cs
- ToolBarButtonClickEvent.cs
- ColumnReorderedEventArgs.cs
- ReadOnlyDataSource.cs
- StringToken.cs
- ContainerActivationHelper.cs
- HtmlElementCollection.cs
- SymbolType.cs
- DataSourceHelper.cs
- ManagementObjectSearcher.cs