Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / PtsHost / CellParaClient.cs / 1 / CellParaClient.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Para client for cell // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- using MS.Internal.Text; using MS.Internal.Documents; using MS.Internal.PtsTable; using System.Security; using System.Windows; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Threading; using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Para client for cell /// internal sealed class CellParaClient : SubpageParaClient { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor. /// /// Cell paragraph. /// Table paraclient. internal CellParaClient(CellParagraph cellParagraph, TableParaClient tableParaClient) : base(cellParagraph) { _tableParaClient = tableParaClient; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Arrange. /// /// U offset component of the cell's visual. /// V offset component of the cell's visual. /// Table's rectangle.. (in page flow dir) /// Table's flow direction /// Page context ////// Critical, because: /// a) calls Critical function PTS.FsClearUpdateInfoInSubpage. /// b) calls Critical function PTS.FsTransformRectangle /// Safe - as the parameters passed in are Critical for set. /// [SecurityCritical, SecurityTreatAsSafe] internal void Arrange(int du, int dv, PTS.FSRECT rcTable, FlowDirection tableFlowDirection, PageContext pageContext) { // // Determine cell width based on column widths. // CalculatedColumn[] calculatedColumns = _tableParaClient.CalculatedColumns; Debug.Assert(calculatedColumns != null && (Cell.ColumnIndex + Cell.ColumnSpan) <= calculatedColumns.Length); double durCellSpacing = Table.InternalCellSpacing; double durCellWidth = -durCellSpacing; // find the width sum of all columns the cell spans int i = Cell.ColumnIndex + Cell.ColumnSpan - 1; do { durCellWidth += calculatedColumns[i].DurWidth + durCellSpacing; } while (--i >= ColumnIndex); if(tableFlowDirection != PageFlowDirection) { PTS.FSRECT pageRect = pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(PageFlowDirection), ref pageRect, ref rcTable, PTS.FlowDirectionToFswdir(tableFlowDirection), out rcTable)); } _rect.u = du + rcTable.u; _rect.v = dv + rcTable.v; _rect.du = TextDpi.ToTextDpi(durCellWidth); _rect.dv = TextDpi.ToTextDpi(_arrangeHeight); if(tableFlowDirection != PageFlowDirection) { PTS.FSRECT pageRect = pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(tableFlowDirection), ref pageRect, ref _rect, PTS.FlowDirectionToFswdir(PageFlowDirection), out _rect)); } _flowDirectionParent = tableFlowDirection; _flowDirection = (FlowDirection)Paragraph.Element.GetValue(FrameworkElement.FlowDirectionProperty); _pageContext = pageContext; OnArrange(); if(_paraHandle.Value != IntPtr.Zero) { PTS.Validate(PTS.FsClearUpdateInfoInSubpage(PtsContext.Context, _paraHandle.Value), PtsContext); } } ////// ValidateVisual. /// internal void ValidateVisual() { ValidateVisual(PTS.FSKUPDATE.fskupdNew); } ////// FormatCellFinite - Calls same apis that PTS does for formatting /// /// Subpage size. /// Subpage break record. /// Is an empty cell ok? /// Text Direction /// Suppress hard break before first para /// Format result /// dvr Used /// Resultant break record for end of page ////// Critical - as this calls the Critical function FormatParaFinite and Critical /// setter on _paraHandle.Value. /// [SecurityCritical] internal void FormatCellFinite(Size subpageSize, IntPtr breakRecordIn, bool isEmptyOk, uint fswdir, PTS.FSKSUPPRESSHARDBREAKBEFOREFIRSTPARA fsksuppresshardbreakbeforefirstparaIn, out PTS.FSFMTR fsfmtr, out int dvrUsed, out IntPtr breakRecordOut) { IntPtr pfspara; PTS.FSBBOX fsbbox; IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; int dvrTopSpace; PTS.FSPAP fspap; if(CellParagraph.StructuralCache.DtrList != null && breakRecordIn != null) { CellParagraph.InvalidateStructure(TextContainerHelper.GetCPFromElement(CellParagraph.StructuralCache.TextContainer, CellParagraph.Element, ElementEdge.BeforeStart)); } // Ensures segment is created for paragraph fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); PTS.FSRECT rectCell; rectCell = new PTS.FSRECT(); rectCell.u = rectCell.v = 0; rectCell.du = TextDpi.ToTextDpi(subpageSize.Width); rectCell.dv = TextDpi.ToTextDpi(subpageSize.Height); // Suppress top space if cell is broken, but not otherwise bool suppressTopSpace = (breakRecordIn != IntPtr.Zero) ? true : false; CellParagraph.FormatParaFinite(this, breakRecordIn, PTS.FromBoolean(true), IntPtr.Zero, PTS.FromBoolean(isEmptyOk), PTS.FromBoolean(suppressTopSpace), fswdir, ref rectCell, null, PTS.FSKCLEAR.fskclearNone, fsksuppresshardbreakbeforefirstparaIn, out fsfmtr, out pfspara, out breakRecordOut, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } _paraHandle.Value = pfspara; } ////// FormatCellBottomless /// /// Text Direction /// Width of cell (height is specified by row props) /// bottomless format result /// dvr Used ////// Critical - as this calls the Critical setter on _paraHandle.Value. /// Safe - as pfspara which it is set to is generated in the function. /// [SecurityCritical, SecurityTreatAsSafe] internal void FormatCellBottomless(uint fswdir, double width, out PTS.FSFMTRBL fmtrbl, out int dvrUsed) { IntPtr pfspara; PTS.FSBBOX fsbbox; IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; int dvrTopSpace; int fPageBecomesUninterruptable; PTS.FSPAP fspap; if(CellParagraph.StructuralCache.DtrList != null) { CellParagraph.InvalidateStructure(TextContainerHelper.GetCPFromElement(CellParagraph.StructuralCache.TextContainer, CellParagraph.Element, ElementEdge.BeforeStart)); } fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); CellParagraph.FormatParaBottomless(this, PTS.FromBoolean(false), fswdir, 0, TextDpi.ToTextDpi(width), 0, null, PTS.FSKCLEAR.fskclearNone, PTS.FromBoolean(true), out fmtrbl, out pfspara, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace, out fPageBecomesUninterruptable); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } _paraHandle.Value = pfspara; } ////// UpdateBottomlessCell /// /// Text Direction /// Width of cell (height is specified by row props) /// bottomless format result /// dvr Used ////// Critical - as this calls Critical function SubpageParagraph.UpdateBottomlessPara. /// Safe - as the pointer parameter passed in is Critical for set. /// [SecurityCritical, SecurityTreatAsSafe] internal void UpdateBottomlessCell(uint fswdir, double width, out PTS.FSFMTRBL fmtrbl, out int dvrUsed) { IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; PTS.FSBBOX fsbbox; int dvrTopSpace; int fPageBecomesUninterruptable; PTS.FSPAP fspap; fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); CellParagraph.UpdateBottomlessPara(_paraHandle.Value, this, PTS.FromBoolean(false), fswdir, 0, TextDpi.ToTextDpi(width), 0, null, PTS.FSKCLEAR.fskclearNone, PTS.FromBoolean(true), out fmtrbl, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace, out fPageBecomesUninterruptable); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } } ////// /// /// /// /// ///internal Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition, Rect visibleRect) { Geometry geometry = null; // Find out if cell is selected. We consider cell selected if its end tag is crossed by selection. // The asymmetry is important here - it allows to use only normalized positions // and still be able to select individual cells. // Note that this logic is an assumption in textselection unit expansion mechanism // (TexSelection.ExtendSelectionToStructuralUnit method). if (endPosition.CompareTo(Cell.StaticElementEnd) >= 0) { geometry = new RectangleGeometry(_rect.FromTextDpi()); } else { SubpageParagraphResult paragraphResult = (SubpageParagraphResult)(CreateParagraphResult()); ReadOnlyCollection colResults = paragraphResult.Columns; Transform transform; transform = new TranslateTransform(-TextDpi.FromTextDpi(ContentRect.u), -TextDpi.FromTextDpi(ContentRect.v)); visibleRect = transform.TransformBounds(visibleRect); transform = null; geometry = TextDocumentView.GetTightBoundingGeometryFromTextPositionsHelper(colResults[0].Paragraphs, paragraphResult.FloatingElements, startPosition, endPosition, 0.0, visibleRect); if (geometry != null) { // restrict geometry to the cell's content rect boundary. // because of end-of-line / end-of-para simulation calculated geometry could be larger. Rect viewport = new Rect(0, 0, TextDpi.FromTextDpi(ContentRect.du), TextDpi.FromTextDpi(ContentRect.dv)); CaretElement.ClipGeometryByViewport(ref geometry, viewport); transform = new TranslateTransform(TextDpi.FromTextDpi(ContentRect.u), TextDpi.FromTextDpi(ContentRect.v)); CaretElement.AddTransformToGeometry(geometry, transform); } } return (geometry); } /// /// Calculates width of cell /// /// Table owner ///Cell's width internal double CalculateCellWidth(TableParaClient tableParaClient) { Debug.Assert(tableParaClient != null); CalculatedColumn[] calculatedColumns = tableParaClient.CalculatedColumns; Debug.Assert( calculatedColumns != null && (Cell.ColumnIndex + Cell.ColumnSpan) <= calculatedColumns.Length); double durCellSpacing = Table.InternalCellSpacing; double durCellWidth = -durCellSpacing; // find the width sum of all columns the cell spans int i = Cell.ColumnIndex + Cell.ColumnSpan - 1; do { durCellWidth += calculatedColumns[i].DurWidth + durCellSpacing; } while (--i >= Cell.ColumnIndex); Debug.Assert(0 <= durCellWidth); return durCellWidth; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Cell. /// internal TableCell Cell { get { return (CellParagraph.Cell); } } ////// Table. /// internal Table Table { get { return (Cell.Table); } } ////// Cell paragraph. /// internal CellParagraph CellParagraph { get { return (CellParagraph)_paragraph; } } ////// Returns column index. /// internal int ColumnIndex { get { return (Cell.ColumnIndex); } } ////// Sets height for arrange. /// internal double ArrangeHeight { set { _arrangeHeight = value; } } ////// Table para client /// internal TableParaClient TableParaClient { get { return (_tableParaClient); } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private double _arrangeHeight; // height for arrange private TableParaClient _tableParaClient; #endregion Private Fields } internal class CellInfo { ////// C'tor - Just needs the table and cell para clients. /// /// Table para client. /// Cell Para client. internal CellInfo(TableParaClient tpc, CellParaClient cpc) { _rectTable = new Rect(TextDpi.FromTextDpi(tpc.Rect.u), TextDpi.FromTextDpi(tpc.Rect.v), TextDpi.FromTextDpi(tpc.Rect.du), TextDpi.FromTextDpi(tpc.Rect.dv)); _rectCell = new Rect(TextDpi.FromTextDpi(cpc.Rect.u), TextDpi.FromTextDpi(cpc.Rect.v), TextDpi.FromTextDpi(cpc.Rect.du), TextDpi.FromTextDpi(cpc.Rect.dv)); _autofitWidth = tpc.AutofitWidth; _columnWidths = new double[tpc.CalculatedColumns.Length]; for(int index = 0; index < tpc.CalculatedColumns.Length; index++) { _columnWidths[index] = tpc.CalculatedColumns[index].DurWidth; } _cell = cpc.Cell; } ////// Adjusts cells rectangles by a given amount /// /// Table para client. internal void Adjust(Point ptAdjust) { _rectTable.X += ptAdjust.X; _rectTable.Y += ptAdjust.Y; _rectCell.X += ptAdjust.X; _rectCell.Y += ptAdjust.Y; } ////// Cell info is for /// internal TableCell Cell { get { return (_cell); } } ////// Widths of columns in table /// internal double[] TableColumnWidths { get { return (_columnWidths); } } ////// Autofit Width of table /// internal double TableAutofitWidth { get { return (_autofitWidth); } } ////// Area for table /// internal Rect TableArea { get { return (_rectTable); } } ////// Area for cell /// internal Rect CellArea { get { return (_rectCell); } } private Rect _rectCell; private Rect _rectTable; private TableCell _cell; private double[] _columnWidths; private double _autofitWidth; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Para client for cell // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- using MS.Internal.Text; using MS.Internal.Documents; using MS.Internal.PtsTable; using System.Security; using System.Windows; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Threading; using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Para client for cell /// internal sealed class CellParaClient : SubpageParaClient { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor. /// /// Cell paragraph. /// Table paraclient. internal CellParaClient(CellParagraph cellParagraph, TableParaClient tableParaClient) : base(cellParagraph) { _tableParaClient = tableParaClient; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Arrange. /// /// U offset component of the cell's visual. /// V offset component of the cell's visual. /// Table's rectangle.. (in page flow dir) /// Table's flow direction /// Page context ////// Critical, because: /// a) calls Critical function PTS.FsClearUpdateInfoInSubpage. /// b) calls Critical function PTS.FsTransformRectangle /// Safe - as the parameters passed in are Critical for set. /// [SecurityCritical, SecurityTreatAsSafe] internal void Arrange(int du, int dv, PTS.FSRECT rcTable, FlowDirection tableFlowDirection, PageContext pageContext) { // // Determine cell width based on column widths. // CalculatedColumn[] calculatedColumns = _tableParaClient.CalculatedColumns; Debug.Assert(calculatedColumns != null && (Cell.ColumnIndex + Cell.ColumnSpan) <= calculatedColumns.Length); double durCellSpacing = Table.InternalCellSpacing; double durCellWidth = -durCellSpacing; // find the width sum of all columns the cell spans int i = Cell.ColumnIndex + Cell.ColumnSpan - 1; do { durCellWidth += calculatedColumns[i].DurWidth + durCellSpacing; } while (--i >= ColumnIndex); if(tableFlowDirection != PageFlowDirection) { PTS.FSRECT pageRect = pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(PageFlowDirection), ref pageRect, ref rcTable, PTS.FlowDirectionToFswdir(tableFlowDirection), out rcTable)); } _rect.u = du + rcTable.u; _rect.v = dv + rcTable.v; _rect.du = TextDpi.ToTextDpi(durCellWidth); _rect.dv = TextDpi.ToTextDpi(_arrangeHeight); if(tableFlowDirection != PageFlowDirection) { PTS.FSRECT pageRect = pageContext.PageRect; PTS.Validate(PTS.FsTransformRectangle(PTS.FlowDirectionToFswdir(tableFlowDirection), ref pageRect, ref _rect, PTS.FlowDirectionToFswdir(PageFlowDirection), out _rect)); } _flowDirectionParent = tableFlowDirection; _flowDirection = (FlowDirection)Paragraph.Element.GetValue(FrameworkElement.FlowDirectionProperty); _pageContext = pageContext; OnArrange(); if(_paraHandle.Value != IntPtr.Zero) { PTS.Validate(PTS.FsClearUpdateInfoInSubpage(PtsContext.Context, _paraHandle.Value), PtsContext); } } ////// ValidateVisual. /// internal void ValidateVisual() { ValidateVisual(PTS.FSKUPDATE.fskupdNew); } ////// FormatCellFinite - Calls same apis that PTS does for formatting /// /// Subpage size. /// Subpage break record. /// Is an empty cell ok? /// Text Direction /// Suppress hard break before first para /// Format result /// dvr Used /// Resultant break record for end of page ////// Critical - as this calls the Critical function FormatParaFinite and Critical /// setter on _paraHandle.Value. /// [SecurityCritical] internal void FormatCellFinite(Size subpageSize, IntPtr breakRecordIn, bool isEmptyOk, uint fswdir, PTS.FSKSUPPRESSHARDBREAKBEFOREFIRSTPARA fsksuppresshardbreakbeforefirstparaIn, out PTS.FSFMTR fsfmtr, out int dvrUsed, out IntPtr breakRecordOut) { IntPtr pfspara; PTS.FSBBOX fsbbox; IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; int dvrTopSpace; PTS.FSPAP fspap; if(CellParagraph.StructuralCache.DtrList != null && breakRecordIn != null) { CellParagraph.InvalidateStructure(TextContainerHelper.GetCPFromElement(CellParagraph.StructuralCache.TextContainer, CellParagraph.Element, ElementEdge.BeforeStart)); } // Ensures segment is created for paragraph fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); PTS.FSRECT rectCell; rectCell = new PTS.FSRECT(); rectCell.u = rectCell.v = 0; rectCell.du = TextDpi.ToTextDpi(subpageSize.Width); rectCell.dv = TextDpi.ToTextDpi(subpageSize.Height); // Suppress top space if cell is broken, but not otherwise bool suppressTopSpace = (breakRecordIn != IntPtr.Zero) ? true : false; CellParagraph.FormatParaFinite(this, breakRecordIn, PTS.FromBoolean(true), IntPtr.Zero, PTS.FromBoolean(isEmptyOk), PTS.FromBoolean(suppressTopSpace), fswdir, ref rectCell, null, PTS.FSKCLEAR.fskclearNone, fsksuppresshardbreakbeforefirstparaIn, out fsfmtr, out pfspara, out breakRecordOut, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } _paraHandle.Value = pfspara; } ////// FormatCellBottomless /// /// Text Direction /// Width of cell (height is specified by row props) /// bottomless format result /// dvr Used ////// Critical - as this calls the Critical setter on _paraHandle.Value. /// Safe - as pfspara which it is set to is generated in the function. /// [SecurityCritical, SecurityTreatAsSafe] internal void FormatCellBottomless(uint fswdir, double width, out PTS.FSFMTRBL fmtrbl, out int dvrUsed) { IntPtr pfspara; PTS.FSBBOX fsbbox; IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; int dvrTopSpace; int fPageBecomesUninterruptable; PTS.FSPAP fspap; if(CellParagraph.StructuralCache.DtrList != null) { CellParagraph.InvalidateStructure(TextContainerHelper.GetCPFromElement(CellParagraph.StructuralCache.TextContainer, CellParagraph.Element, ElementEdge.BeforeStart)); } fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); CellParagraph.FormatParaBottomless(this, PTS.FromBoolean(false), fswdir, 0, TextDpi.ToTextDpi(width), 0, null, PTS.FSKCLEAR.fskclearNone, PTS.FromBoolean(true), out fmtrbl, out pfspara, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace, out fPageBecomesUninterruptable); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } _paraHandle.Value = pfspara; } ////// UpdateBottomlessCell /// /// Text Direction /// Width of cell (height is specified by row props) /// bottomless format result /// dvr Used ////// Critical - as this calls Critical function SubpageParagraph.UpdateBottomlessPara. /// Safe - as the pointer parameter passed in is Critical for set. /// [SecurityCritical, SecurityTreatAsSafe] internal void UpdateBottomlessCell(uint fswdir, double width, out PTS.FSFMTRBL fmtrbl, out int dvrUsed) { IntPtr pmcsclientOut; PTS.FSKCLEAR fskclearOut; PTS.FSBBOX fsbbox; int dvrTopSpace; int fPageBecomesUninterruptable; PTS.FSPAP fspap; fspap = new PTS.FSPAP(); CellParagraph.GetParaProperties(ref fspap); CellParagraph.UpdateBottomlessPara(_paraHandle.Value, this, PTS.FromBoolean(false), fswdir, 0, TextDpi.ToTextDpi(width), 0, null, PTS.FSKCLEAR.fskclearNone, PTS.FromBoolean(true), out fmtrbl, out dvrUsed, out fsbbox, out pmcsclientOut, out fskclearOut, out dvrTopSpace, out fPageBecomesUninterruptable); if (pmcsclientOut != IntPtr.Zero) { MarginCollapsingState mcs = PtsContext.HandleToObject(pmcsclientOut) as MarginCollapsingState; PTS.ValidateHandle(mcs); dvrUsed += mcs.Margin; mcs.Dispose(); pmcsclientOut = IntPtr.Zero; } } ////// /// /// /// /// ///internal Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition, Rect visibleRect) { Geometry geometry = null; // Find out if cell is selected. We consider cell selected if its end tag is crossed by selection. // The asymmetry is important here - it allows to use only normalized positions // and still be able to select individual cells. // Note that this logic is an assumption in textselection unit expansion mechanism // (TexSelection.ExtendSelectionToStructuralUnit method). if (endPosition.CompareTo(Cell.StaticElementEnd) >= 0) { geometry = new RectangleGeometry(_rect.FromTextDpi()); } else { SubpageParagraphResult paragraphResult = (SubpageParagraphResult)(CreateParagraphResult()); ReadOnlyCollection colResults = paragraphResult.Columns; Transform transform; transform = new TranslateTransform(-TextDpi.FromTextDpi(ContentRect.u), -TextDpi.FromTextDpi(ContentRect.v)); visibleRect = transform.TransformBounds(visibleRect); transform = null; geometry = TextDocumentView.GetTightBoundingGeometryFromTextPositionsHelper(colResults[0].Paragraphs, paragraphResult.FloatingElements, startPosition, endPosition, 0.0, visibleRect); if (geometry != null) { // restrict geometry to the cell's content rect boundary. // because of end-of-line / end-of-para simulation calculated geometry could be larger. Rect viewport = new Rect(0, 0, TextDpi.FromTextDpi(ContentRect.du), TextDpi.FromTextDpi(ContentRect.dv)); CaretElement.ClipGeometryByViewport(ref geometry, viewport); transform = new TranslateTransform(TextDpi.FromTextDpi(ContentRect.u), TextDpi.FromTextDpi(ContentRect.v)); CaretElement.AddTransformToGeometry(geometry, transform); } } return (geometry); } /// /// Calculates width of cell /// /// Table owner ///Cell's width internal double CalculateCellWidth(TableParaClient tableParaClient) { Debug.Assert(tableParaClient != null); CalculatedColumn[] calculatedColumns = tableParaClient.CalculatedColumns; Debug.Assert( calculatedColumns != null && (Cell.ColumnIndex + Cell.ColumnSpan) <= calculatedColumns.Length); double durCellSpacing = Table.InternalCellSpacing; double durCellWidth = -durCellSpacing; // find the width sum of all columns the cell spans int i = Cell.ColumnIndex + Cell.ColumnSpan - 1; do { durCellWidth += calculatedColumns[i].DurWidth + durCellSpacing; } while (--i >= Cell.ColumnIndex); Debug.Assert(0 <= durCellWidth); return durCellWidth; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Cell. /// internal TableCell Cell { get { return (CellParagraph.Cell); } } ////// Table. /// internal Table Table { get { return (Cell.Table); } } ////// Cell paragraph. /// internal CellParagraph CellParagraph { get { return (CellParagraph)_paragraph; } } ////// Returns column index. /// internal int ColumnIndex { get { return (Cell.ColumnIndex); } } ////// Sets height for arrange. /// internal double ArrangeHeight { set { _arrangeHeight = value; } } ////// Table para client /// internal TableParaClient TableParaClient { get { return (_tableParaClient); } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private double _arrangeHeight; // height for arrange private TableParaClient _tableParaClient; #endregion Private Fields } internal class CellInfo { ////// C'tor - Just needs the table and cell para clients. /// /// Table para client. /// Cell Para client. internal CellInfo(TableParaClient tpc, CellParaClient cpc) { _rectTable = new Rect(TextDpi.FromTextDpi(tpc.Rect.u), TextDpi.FromTextDpi(tpc.Rect.v), TextDpi.FromTextDpi(tpc.Rect.du), TextDpi.FromTextDpi(tpc.Rect.dv)); _rectCell = new Rect(TextDpi.FromTextDpi(cpc.Rect.u), TextDpi.FromTextDpi(cpc.Rect.v), TextDpi.FromTextDpi(cpc.Rect.du), TextDpi.FromTextDpi(cpc.Rect.dv)); _autofitWidth = tpc.AutofitWidth; _columnWidths = new double[tpc.CalculatedColumns.Length]; for(int index = 0; index < tpc.CalculatedColumns.Length; index++) { _columnWidths[index] = tpc.CalculatedColumns[index].DurWidth; } _cell = cpc.Cell; } ////// Adjusts cells rectangles by a given amount /// /// Table para client. internal void Adjust(Point ptAdjust) { _rectTable.X += ptAdjust.X; _rectTable.Y += ptAdjust.Y; _rectCell.X += ptAdjust.X; _rectCell.Y += ptAdjust.Y; } ////// Cell info is for /// internal TableCell Cell { get { return (_cell); } } ////// Widths of columns in table /// internal double[] TableColumnWidths { get { return (_columnWidths); } } ////// Autofit Width of table /// internal double TableAutofitWidth { get { return (_autofitWidth); } } ////// Area for table /// internal Rect TableArea { get { return (_rectTable); } } ////// Area for cell /// internal Rect CellArea { get { return (_rectCell); } } private Rect _rectCell; private Rect _rectTable; private TableCell _cell; private double[] _columnWidths; private double _autofitWidth; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
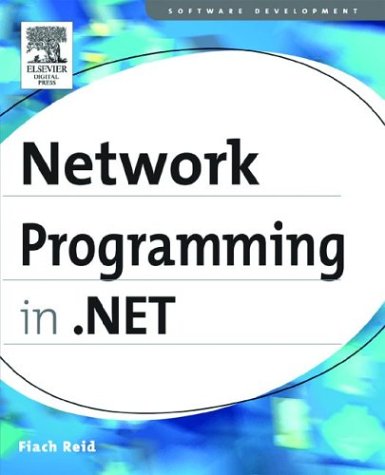
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ControlPropertyNameConverter.cs
- HttpCapabilitiesBase.cs
- DuplicateContext.cs
- SecurityChannelFaultConverter.cs
- Schema.cs
- DiscoveryExceptionDictionary.cs
- HandlerFactoryCache.cs
- DictionaryManager.cs
- VirtualPathUtility.cs
- FloaterBaseParagraph.cs
- XmlSchemaObjectCollection.cs
- PropertyCollection.cs
- RelationshipConstraintValidator.cs
- ToolBarButton.cs
- PersonalizationState.cs
- BooleanFacetDescriptionElement.cs
- CredentialCache.cs
- WebPartDisplayModeCollection.cs
- NestedContainer.cs
- SelectionProviderWrapper.cs
- OdbcCommand.cs
- Highlights.cs
- SamlAttribute.cs
- KeyboardNavigation.cs
- ModifyActivitiesPropertyDescriptor.cs
- CrossAppDomainChannel.cs
- TemplateXamlParser.cs
- MultipartContentParser.cs
- SiteMapDataSourceView.cs
- Form.cs
- Pts.cs
- XPathDocumentNavigator.cs
- XmlDesignerDataSourceView.cs
- DependencyPropertyAttribute.cs
- LinkClickEvent.cs
- TagPrefixCollection.cs
- _BaseOverlappedAsyncResult.cs
- TrackingQuery.cs
- StyleSelector.cs
- ClassDataContract.cs
- HttpCacheParams.cs
- TeredoHelper.cs
- DelimitedListTraceListener.cs
- MatrixAnimationUsingPath.cs
- MatrixAnimationBase.cs
- AutomationPropertyInfo.cs
- UrlAuthorizationModule.cs
- TargetException.cs
- NetworkStream.cs
- CompositionTarget.cs
- TimeBoundedCache.cs
- RequestedSignatureDialog.cs
- OleDbPropertySetGuid.cs
- TcpAppDomainProtocolHandler.cs
- TrustLevelCollection.cs
- CompositeTypefaceMetrics.cs
- ChtmlTextBoxAdapter.cs
- DataBinder.cs
- _TransmitFileOverlappedAsyncResult.cs
- TimelineGroup.cs
- NamedElement.cs
- BrushProxy.cs
- PlanCompiler.cs
- NavigationProperty.cs
- SourceChangedEventArgs.cs
- WindowsClaimSet.cs
- DesignTimeTemplateParser.cs
- ClientEventManager.cs
- ParameterCollection.cs
- TagMapInfo.cs
- PrePrepareMethodAttribute.cs
- Schema.cs
- TemplatedControlDesigner.cs
- PolicyLevel.cs
- TextFormatterContext.cs
- DataControlImageButton.cs
- Freezable.cs
- BitArray.cs
- PersistenceIOParticipant.cs
- WebUtil.cs
- ColorMatrix.cs
- RayMeshGeometry3DHitTestResult.cs
- RuntimeEnvironment.cs
- NodeLabelEditEvent.cs
- StackOverflowException.cs
- PlatformCulture.cs
- TimeSpan.cs
- WebPartZone.cs
- PartialCachingControl.cs
- AssemblyResourceLoader.cs
- UnsupportedPolicyOptionsException.cs
- RecordConverter.cs
- Decoder.cs
- WrappedOptions.cs
- CollectionBuilder.cs
- ServerType.cs
- TransactionScope.cs
- PeerNameResolver.cs
- TimeManager.cs
- InputLanguageSource.cs