Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / GridLength.cs / 1305600 / GridLength.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Grid length implementation // // See spec at http://avalon/layout/Specs/Star%20LengthUnit.mht // // History: // 09/24/2003 : olego - Created (in griddy prototype branch); // 10/27/2003 : olego - Ported from griddy prototype branch; // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; namespace System.Windows { ////// GridUnitType enum is used to indicate what kind of value the /// GridLength is holding. /// // Note: Keep the GridUnitType enum in [....] with the string representation // of units (GridLengthConverter._unitString). public enum GridUnitType { ////// The value indicates that content should be calculated without constraints. /// Auto = 0, ////// The value is expressed as a pixel. /// Pixel, ////// The value is expressed as a weighted proportion of available space. /// Star, } ////// GridLength is the type used for various length-like properties in the system, /// that explicitely support Star unit type. For example, "Width", "Height" /// properties of ColumnDefinition and RowDefinition used by Grid. /// [TypeConverter(typeof(GridLengthConverter))] public struct GridLength : IEquatable{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Constructor, initializes the GridLength as absolute value in pixels. /// /// Specifies the number of 'device-independent pixels' /// (96 pixels-per-inch). ////// If public GridLength(double pixels) : this(pixels, GridUnitType.Pixel) { } ///pixels parameter isdouble.NaN /// orpixels parameter isdouble.NegativeInfinity /// orpixels parameter isdouble.PositiveInfinity . ////// Constructor, initializes the GridLength and specifies what kind of value /// it will hold. /// /// Value to be stored by this GridLength /// instance. /// Type of the value to be stored by this GridLength /// instance. ////// If the ///type parameter isGridUnitType.Auto , /// then passed in value is ignored and replaced with0 . ////// If public GridLength(double value, GridUnitType type) { if (DoubleUtil.IsNaN(value)) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterNoNaN, "value")); } if (double.IsInfinity(value)) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterNoInfinity, "value")); } if ( type != GridUnitType.Auto && type != GridUnitType.Pixel && type != GridUnitType.Star ) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterUnknownGridUnitType, "type")); } _unitValue = (type == GridUnitType.Auto) ? 0.0 : value; _unitType = type; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ///value parameter isdouble.NaN /// orvalue parameter isdouble.NegativeInfinity /// orvalue parameter isdouble.PositiveInfinity . ////// Overloaded operator, compares 2 GridLength's. /// /// first GridLength to compare. /// second GridLength to compare. ///true if specified GridLengths have same value /// and unit type. public static bool operator == (GridLength gl1, GridLength gl2) { return ( gl1.GridUnitType == gl2.GridUnitType && gl1.Value == gl2.Value ); } ////// Overloaded operator, compares 2 GridLength's. /// /// first GridLength to compare. /// second GridLength to compare. ///true if specified GridLengths have either different value or /// unit type. public static bool operator != (GridLength gl1, GridLength gl2) { return ( gl1.GridUnitType != gl2.GridUnitType || gl1.Value != gl2.Value ); } ////// Compares this instance of GridLength with another object. /// /// Reference to an object for comparison. ///override public bool Equals(object oCompare) { if(oCompare is GridLength) { GridLength l = (GridLength)oCompare; return (this == l); } else return false; } /// true if this GridLength instance has the same value /// and unit type as oCompare./// Compares this instance of GridLength with another instance. /// /// Grid length instance to compare. ///public bool Equals(GridLength gridLength) { return (this == gridLength); } /// true if this GridLength instance has the same value /// and unit type as gridLength./// ////// public override int GetHashCode() { return ((int)_unitValue + (int)_unitType); } /// /// Returns public bool IsAbsolute { get { return (_unitType == GridUnitType.Pixel); } } ///true if this GridLength instance holds /// an absolute (pixel) value. ////// Returns public bool IsAuto { get { return (_unitType == GridUnitType.Auto); } } ///true if this GridLength instance is /// automatic (not specified). ////// Returns public bool IsStar { get { return (_unitType == GridUnitType.Star); } } ///true if this GridLength instance holds weighted propertion /// of available space. ////// Returns value part of this GridLength instance. /// public double Value { get { return ((_unitType == GridUnitType.Auto) ? 1.0 : _unitValue); } } ////// Returns unit type of this GridLength instance. /// public GridUnitType GridUnitType { get { return (_unitType); } } ////// Returns the string representation of this object. /// public override string ToString() { return GridLengthConverter.ToString(this, CultureInfo.InvariantCulture); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Returns initialized Auto GridLength value. /// public static GridLength Auto { get { return (s_auto); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private double _unitValue; // unit value storage private GridUnitType _unitType; // unit type storage // static instance of Auto GridLength private static readonly GridLength s_auto = new GridLength(1.0, GridUnitType.Auto); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
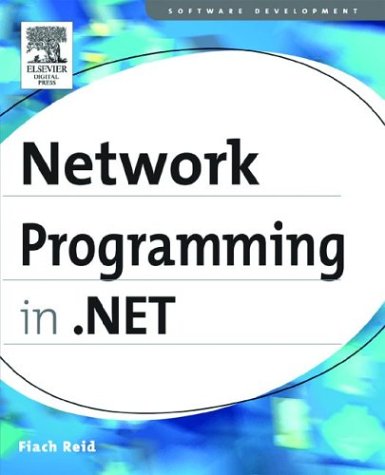
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NonBatchDirectoryCompiler.cs
- RectangleHotSpot.cs
- HtmlWindow.cs
- WindowsGraphics2.cs
- CodeValidator.cs
- MULTI_QI.cs
- COM2Enum.cs
- DbConnectionPoolIdentity.cs
- ReachUIElementCollectionSerializer.cs
- DrawingBrush.cs
- ThrowOnMultipleAssignment.cs
- SequenceQuery.cs
- EntryIndex.cs
- XmlNullResolver.cs
- ColumnCollection.cs
- WaitingCursor.cs
- LayoutEditorPart.cs
- MessageContractExporter.cs
- ResourceDefaultValueAttribute.cs
- AutomationElement.cs
- DynamicValueConverter.cs
- TreeNode.cs
- OpenFileDialog.cs
- Quad.cs
- WebEventCodes.cs
- SQLRoleProvider.cs
- IODescriptionAttribute.cs
- StringResourceManager.cs
- MenuEventArgs.cs
- ApplicationContext.cs
- WindowsListViewGroupSubsetLink.cs
- SignatureHelper.cs
- ScrollViewerAutomationPeer.cs
- SafeProcessHandle.cs
- FlowSwitchLink.cs
- RectangleF.cs
- ObjectStateManager.cs
- UnSafeCharBuffer.cs
- BooleanSwitch.cs
- FontDifferentiator.cs
- TimelineCollection.cs
- Currency.cs
- GlyphShapingProperties.cs
- SqlCachedBuffer.cs
- ThreadExceptionDialog.cs
- DemultiplexingClientMessageFormatter.cs
- InlineCollection.cs
- XPathScanner.cs
- PointConverter.cs
- WebBrowserNavigatingEventHandler.cs
- WhereQueryOperator.cs
- FillBehavior.cs
- UInt64.cs
- AssociationSetEnd.cs
- SafeProcessHandle.cs
- SectionInput.cs
- MethodCallConverter.cs
- ByteAnimation.cs
- InputLangChangeEvent.cs
- DesignerActionUIService.cs
- HttpApplication.cs
- EventProviderBase.cs
- IntranetCredentialPolicy.cs
- Baml2006ReaderSettings.cs
- Attachment.cs
- ContractAdapter.cs
- KeyValueConfigurationCollection.cs
- OrderPreservingSpoolingTask.cs
- GroupBox.cs
- NotFiniteNumberException.cs
- VolatileEnlistmentState.cs
- WindowsScrollBar.cs
- ISO2022Encoding.cs
- XmlDataSourceNodeDescriptor.cs
- OperationFormatStyle.cs
- AsyncStreamReader.cs
- BindingRestrictions.cs
- DataGridViewColumnEventArgs.cs
- TextContainerChangedEventArgs.cs
- adornercollection.cs
- XmlSchemaSimpleType.cs
- BuildDependencySet.cs
- TextTreeExtractElementUndoUnit.cs
- validation.cs
- SecurityTokenAuthenticator.cs
- AutomationPropertyInfo.cs
- XamlSerializerUtil.cs
- ListBox.cs
- TableColumn.cs
- ELinqQueryState.cs
- DataGridCell.cs
- TextTreeTextBlock.cs
- ViewValidator.cs
- FileStream.cs
- ResXResourceReader.cs
- XmlTextReaderImpl.cs
- CryptoHandle.cs
- PasswordPropertyTextAttribute.cs
- MenuItemStyle.cs
- DrawingContextDrawingContextWalker.cs