Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / MruCache.cs / 1 / MruCache.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Collections.Generic; using System.Diagnostics; class MruCachewhere TKey : class where TValue : class { LinkedList mruList; Dictionary items; int lowWatermark; int highWatermark; CacheEntry mruEntry; public MruCache(int watermark) : this(watermark * 4 / 5, watermark) { } // // The cache will grow until the high watermark. At which point, the least recently used items // will be purge until the cache's size is reduced to low watermark // public MruCache(int lowWatermark, int highWatermark) : this(lowWatermark, highWatermark, null) { } public MruCache(int lowWatermark, int highWatermark, IEqualityComparer comparer) { DiagnosticUtility.DebugAssert(lowWatermark <= highWatermark, ""); DiagnosticUtility.DebugAssert(lowWatermark > 0, ""); this.lowWatermark = lowWatermark; this.highWatermark = highWatermark; this.mruList = new LinkedList (); if (comparer == null) { this.items = new Dictionary (); } else { this.items = new Dictionary (comparer); } } public void Add(TKey key, TValue value) { DiagnosticUtility.DebugAssert(null != key, ""); // if anything goes wrong (duplicate entry, etc) we should // clear our caches so that we don't get out of sync bool success = false; try { if (this.items.Count == this.highWatermark) { // If the cache is full, purge enough LRU items to shrink the // cache down to the low watermark int countToPurge = this.highWatermark - this.lowWatermark; for (int i = 0; i < countToPurge; i++) { TKey keyRemove = this.mruList.Last.Value; this.mruList.RemoveLast(); TValue item = this.items[keyRemove].value; this.items.Remove(keyRemove); OnSingleItemRemoved(item); } } // Add the new entry to the cache and make it the MRU element CacheEntry entry; entry.node = this.mruList.AddFirst(key); entry.value = value; this.items.Add(key, entry); this.mruEntry = entry; success = true; } finally { if (!success) { this.Clear(); } } } public void Clear() { this.mruList.Clear(); this.items.Clear(); this.mruEntry.value = null; this.mruEntry.node = null; } public bool Remove(TKey key) { DiagnosticUtility.DebugAssert(null != key, ""); CacheEntry entry; if (this.items.TryGetValue(key, out entry)) { this.items.Remove(key); OnSingleItemRemoved(entry.value); this.mruList.Remove(entry.node); if (object.ReferenceEquals(this.mruEntry.node, entry.node)) { this.mruEntry.value = null; this.mruEntry.node = null; } return true; } return false; } protected virtual void OnSingleItemRemoved(TValue item) { } // // If found, make the entry most recently used // public bool TryGetValue(TKey key, out TValue value) { // first check our MRU item if (this.mruEntry.node != null && key != null && key.Equals(this.mruEntry.node.Value)) { value = this.mruEntry.value; return true; } CacheEntry entry; bool found = this.items.TryGetValue(key, out entry); value = entry.value; // Move the node to the head of the MRU list if it's not already there if (found && this.mruList.Count > 1 && !object.ReferenceEquals(this.mruList.First, entry.node)) { this.mruList.Remove(entry.node); this.mruList.AddFirst(entry.node); this.mruEntry = entry; } return found; } struct CacheEntry { internal TValue value; internal LinkedListNode node; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
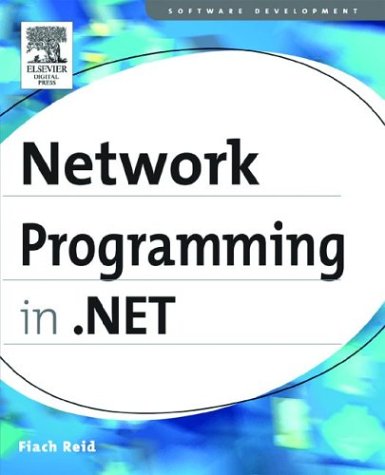
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TripleDESCryptoServiceProvider.cs
- TagMapInfo.cs
- TransformedBitmap.cs
- ProviderMetadataCachedInformation.cs
- UICuesEvent.cs
- PathFigureCollectionConverter.cs
- Serializer.cs
- Help.cs
- SortDescriptionCollection.cs
- TextOptions.cs
- ThicknessAnimationBase.cs
- ValidatedControlConverter.cs
- BulletChrome.cs
- HashCryptoHandle.cs
- DrawingDrawingContext.cs
- FloaterParaClient.cs
- GuidTagList.cs
- DiscoveryClientRequestChannel.cs
- CustomErrorsSectionWrapper.cs
- UnicastIPAddressInformationCollection.cs
- CustomCategoryAttribute.cs
- PlanCompiler.cs
- TrustLevelCollection.cs
- ExpressionCopier.cs
- ResolveNameEventArgs.cs
- PerformanceCounterPermissionEntry.cs
- XPathDocumentIterator.cs
- PrinterUnitConvert.cs
- IHttpResponseInternal.cs
- TreeViewEvent.cs
- Camera.cs
- StreamReader.cs
- MonikerHelper.cs
- HtmlWindowCollection.cs
- SqlInternalConnectionTds.cs
- TypeNameConverter.cs
- List.cs
- ToolbarAUtomationPeer.cs
- BitmapImage.cs
- WebBrowsableAttribute.cs
- Event.cs
- DataFormat.cs
- DbParameterHelper.cs
- AggregateNode.cs
- HttpListenerRequest.cs
- OleCmdHelper.cs
- DataControlPagerLinkButton.cs
- TextLineBreak.cs
- KeyTimeConverter.cs
- Avt.cs
- SqlCharStream.cs
- PropertyMap.cs
- GridViewEditEventArgs.cs
- SecurityException.cs
- EntityParameter.cs
- RotateTransform3D.cs
- WebSysDescriptionAttribute.cs
- BitSet.cs
- CustomDictionarySources.cs
- RawStylusActions.cs
- ErrorHandler.cs
- SiteIdentityPermission.cs
- HasActivatableWorkflowEvent.cs
- StatusBarPanel.cs
- DiagnosticSection.cs
- ClientRolePrincipal.cs
- IxmlLineInfo.cs
- AppSettingsReader.cs
- ImportCatalogPart.cs
- ThrowHelper.cs
- ModuleElement.cs
- XmlArrayAttribute.cs
- EventTrigger.cs
- PeerCollaborationPermission.cs
- DocumentGridPage.cs
- SqlXmlStorage.cs
- MenuCommand.cs
- DiagnosticTrace.cs
- ProfileService.cs
- URI.cs
- HostingEnvironmentSection.cs
- TraversalRequest.cs
- fixedPageContentExtractor.cs
- ClientScriptItemCollection.cs
- WindowHideOrCloseTracker.cs
- ADMembershipUser.cs
- QueryCursorEventArgs.cs
- FormViewDeleteEventArgs.cs
- TcpAppDomainProtocolHandler.cs
- JsonObjectDataContract.cs
- XmlUtil.cs
- LocalizedNameDescriptionPair.cs
- WebReferenceCollection.cs
- ThreadStateException.cs
- ClientRuntimeConfig.cs
- LassoSelectionBehavior.cs
- RNGCryptoServiceProvider.cs
- TextEditorContextMenu.cs
- InvalidComObjectException.cs
- ToolStripTemplateNode.cs