Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Automation / Peers / ComboBoxAutomationPeer.cs / 1 / ComboBoxAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ComboBoxAutomationPeer: SelectorAutomationPeer, IValueProvider, IExpandCollapseProvider { /// public ComboBoxAutomationPeer(ComboBox owner): base(owner) {} /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { // Use the same peer as ListBox return new ListBoxItemAutomationPeer(item, this); } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.ComboBox; } /// override protected string GetClassNameCore() { return "ComboBox"; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if (pattern == PatternInterface.Value) { ComboBox owner = (ComboBox)Owner; if (owner.IsEditable) iface = this; } else if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } /// protected override ListGetChildrenCore() { List children = base.GetChildrenCore(); // include text box part into children collection ComboBox owner = (ComboBox)Owner; TextBox textBox = owner.EditableTextBoxSite; if (textBox != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(textBox); if (peer != null) { if (children == null) children = new List (); children.Insert(0, peer); } } return children; } #region Value /// /// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IValueProvider.SetValue(string val) { if (val == null) throw new ArgumentNullException("val"); ComboBox owner = (ComboBox)Owner; if (!owner.IsEnabled) throw new ElementNotEnabledException(); owner.Text = val; } ///Value of a value control, as a a string. string IValueProvider.Value { get { return ((ComboBox)(((ComboBoxAutomationPeer)this).Owner)).Text; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IValueProvider.IsReadOnly { get { ComboBox owner = (ComboBox)Owner; return !owner.IsEnabled || owner.IsReadOnly; } } #endregion Value #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = true; } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = false; } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; return owner.IsDropDownOpen ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ComboBoxAutomationPeer: SelectorAutomationPeer, IValueProvider, IExpandCollapseProvider { /// public ComboBoxAutomationPeer(ComboBox owner): base(owner) {} /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { // Use the same peer as ListBox return new ListBoxItemAutomationPeer(item, this); } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.ComboBox; } /// override protected string GetClassNameCore() { return "ComboBox"; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if (pattern == PatternInterface.Value) { ComboBox owner = (ComboBox)Owner; if (owner.IsEditable) iface = this; } else if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } /// protected override ListGetChildrenCore() { List children = base.GetChildrenCore(); // include text box part into children collection ComboBox owner = (ComboBox)Owner; TextBox textBox = owner.EditableTextBoxSite; if (textBox != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(textBox); if (peer != null) { if (children == null) children = new List (); children.Insert(0, peer); } } return children; } #region Value /// /// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IValueProvider.SetValue(string val) { if (val == null) throw new ArgumentNullException("val"); ComboBox owner = (ComboBox)Owner; if (!owner.IsEnabled) throw new ElementNotEnabledException(); owner.Text = val; } ///Value of a value control, as a a string. string IValueProvider.Value { get { return ((ComboBox)(((ComboBoxAutomationPeer)this).Owner)).Text; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IValueProvider.IsReadOnly { get { ComboBox owner = (ComboBox)Owner; return !owner.IsEnabled || owner.IsReadOnly; } } #endregion Value #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = true; } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = false; } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; return owner.IsDropDownOpen ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
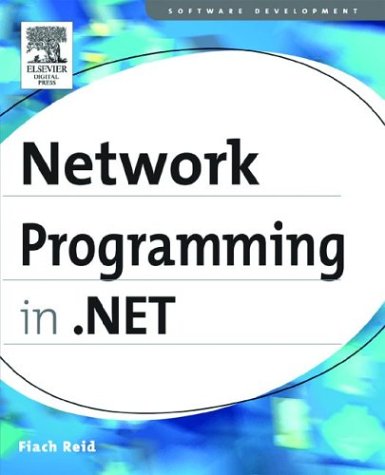
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceLockException.cs
- BindingsCollection.cs
- Geometry3D.cs
- BuildProvider.cs
- XmlSiteMapProvider.cs
- PowerModeChangedEventArgs.cs
- MessageSecurityVersion.cs
- Vector3DCollection.cs
- HierarchicalDataSourceConverter.cs
- ObjectListCommandEventArgs.cs
- SmiRequestExecutor.cs
- RotationValidation.cs
- FillRuleValidation.cs
- GraphicsPath.cs
- ResourceDictionary.cs
- ProfilePropertySettings.cs
- SemanticBasicElement.cs
- PolicyManager.cs
- HtmlSelectionListAdapter.cs
- ScriptControlDescriptor.cs
- TextRangeSerialization.cs
- IntPtr.cs
- DBBindings.cs
- ModelTreeEnumerator.cs
- StorageComplexPropertyMapping.cs
- ErrorWrapper.cs
- HtmlHead.cs
- StoreAnnotationsMap.cs
- DescendantQuery.cs
- CommentEmitter.cs
- ButtonRenderer.cs
- Material.cs
- CommentEmitter.cs
- FormViewInsertEventArgs.cs
- StateDesignerConnector.cs
- PathFigureCollectionValueSerializer.cs
- SocketPermission.cs
- IndexObject.cs
- InvokeFunc.cs
- TypeLibConverter.cs
- CollectionViewGroup.cs
- VirtualPath.cs
- MDIControlStrip.cs
- TextDecoration.cs
- LoginCancelEventArgs.cs
- RightsManagementPermission.cs
- COM2ICategorizePropertiesHandler.cs
- TraceInternal.cs
- ReadOnlyKeyedCollection.cs
- XmlSchemaInclude.cs
- ClaimTypes.cs
- SchemaImporterExtensionElementCollection.cs
- ThreadStaticAttribute.cs
- SerializationInfo.cs
- NameValueFileSectionHandler.cs
- TraceHandlerErrorFormatter.cs
- AddressAccessDeniedException.cs
- SerialPinChanges.cs
- SecondaryViewProvider.cs
- ResourceDisplayNameAttribute.cs
- MultilineStringConverter.cs
- MILUtilities.cs
- SafeTokenHandle.cs
- NotifyInputEventArgs.cs
- Latin1Encoding.cs
- ContractCodeDomInfo.cs
- Deflater.cs
- HostingEnvironment.cs
- PackageDigitalSignature.cs
- ApplicationActivator.cs
- Durable.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- EventLogger.cs
- UnsafeNativeMethodsTablet.cs
- HttpConfigurationContext.cs
- SqlCacheDependencySection.cs
- dbenumerator.cs
- ContextProperty.cs
- FlowDocumentFormatter.cs
- CaseStatementSlot.cs
- ModifiableIteratorCollection.cs
- LoaderAllocator.cs
- LinqDataSourceView.cs
- Main.cs
- PlanCompiler.cs
- NoResizeSelectionBorderGlyph.cs
- Button.cs
- TableCell.cs
- Function.cs
- UnescapedXmlDiagnosticData.cs
- PackageRelationshipCollection.cs
- ViewStateException.cs
- DataRelationPropertyDescriptor.cs
- LayoutTable.cs
- XPathDescendantIterator.cs
- PropertyInfoSet.cs
- StylusButtonEventArgs.cs
- CodeRemoveEventStatement.cs
- PerfCounterSection.cs
- XmlDataImplementation.cs