Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / OleAutBinder.cs / 1 / OleAutBinder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // This class represents the Ole Automation binder. // #define DISPLAY_DEBUG_INFO namespace System { using System; using System.Runtime.InteropServices; using System.Reflection; using Microsoft.Win32; using CultureInfo = System.Globalization.CultureInfo; // Made serializable in anticipation of this class eventually having state. [Serializable()] internal class OleAutBinder : DefaultBinder { // ChangeType // This binder uses OLEAUT to change the type of the variant. public override Object ChangeType(Object value, Type type, CultureInfo cultureInfo) { Variant myValue = new Variant(value); if (cultureInfo == null) cultureInfo = CultureInfo.CurrentCulture; #if DISPLAY_DEBUG_INFO Console.Write("In OleAutBinder::ChangeType converting variant of type: "); Console.Write(myValue.VariantType); Console.Write(" to type: "); Console.WriteLine(type.Name); #endif if (type.IsByRef) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Striping byref from the type to convert to."); #endif type = type.GetElementType(); } // If we are trying to convert from an object to another type then we don't // need the OLEAUT change type, we can just use the normal COM+ mechanisms. if (!type.IsPrimitive && type.IsInstanceOfType(value)) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Source variant can be assigned to destination type"); #endif return value; } Type srcType = value.GetType(); // Handle converting primitives to enums. if (type.IsEnum && srcType.IsPrimitive) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Converting primitive to enum"); #endif return Enum.Parse(type, value.ToString()); } // Special case the convertion from DBNull. if (srcType == typeof(DBNull)) { // The requested type is a DBNull so no convertion is required. if (type == typeof(DBNull)) return value; // Visual J++ supported converting from DBNull to null so customers // have requested (via a CDCR) that DBNull be convertible to null. // We don't however allow this when converting to a value class, since null // doesn't make sense for these, or to object since this would change existing // semantics. if ((type.IsClass && type != typeof(Object)) || type.IsInterface) return null; } #if FEATURE_COMINTEROP // Use the OA variant lib to convert primitive types. try { #if DISPLAY_DEBUG_INFO Console.WriteLine("Using OAVariantLib.ChangeType() to do the conversion"); #endif // Specify the LocalBool flag to have BOOL values converted to local language rather // than 0 or -1. Object RetObj = OAVariantLib.ChangeType(myValue, type, OAVariantLib.LocalBool, cultureInfo).ToObject(); #if DISPLAY_DEBUG_INFO Console.WriteLine("Object returned from ChangeType is of type: " + RetObj.GetType().Name); #endif return RetObj; } #if DISPLAY_DEBUG_INFO catch(NotSupportedException e) #else catch(NotSupportedException) #endif { #if DISPLAY_DEBUG_INFO Console.Write("Exception thrown: "); Console.WriteLine(e); #endif throw new COMException(Environment.GetResourceString("Interop.COM_TypeMismatch"), unchecked((int)0x80020005)); } #else // !FEATURE_COMINTEROP return base.ChangeType(value, type, cultureInfo); #endif // !FEATURE_COMINTEROP } #if !FEATURE_COMINTEROP // CanChangeType public override bool CanChangeType(Object value, Type type, CultureInfo cultureInfo) { Variant myValue = new Variant(value); if (cultureInfo == null) cultureInfo = CultureInfo.CurrentCulture; #if DISPLAY_DEBUG_INFO Console.Write("In OleAutBinder::CanChangeType converting variant of type: "); Console.Write(myValue.VariantType); Console.Write(" to type: "); Console.WriteLine(type.Name); #endif // If we are trying to convert to variant then there is nothing to do. if (type == typeof(Variant)) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Variant being changed to type variant is always legal"); #endif return true; } if (type.IsByRef) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Striping byref from the type to convert to."); #endif type = type.GetElementType(); } // If we are trying to convert from an object to another type then we don't // need the OLEAUT change type, we can just use the normal COM+ mechanisms. if (!type.IsPrimitive && type.IsInstanceOfType(value)) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Source variant can be assigned to destination type"); #endif return true; } // Handle converting primitives to enums. if (type.IsEnum && value.GetType().IsPrimitive) { #if DISPLAY_DEBUG_INFO Console.WriteLine("Converting primitive to enum"); #endif return true; } return base.CanChangeType(value, type, cultureInfo); } #endif // FEATURE_COMINTEROP } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
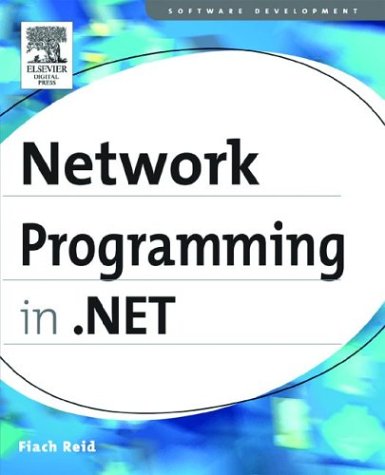
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HuffCodec.cs
- DataBinder.cs
- SettingsAttributes.cs
- ToolStripCollectionEditor.cs
- ParallelLoopState.cs
- Button.cs
- OverflowException.cs
- DeferredElementTreeState.cs
- ToolStripDropDownItem.cs
- MessageAction.cs
- XmlValidatingReaderImpl.cs
- XmlAnyElementAttribute.cs
- SynchronizedMessageSource.cs
- SerialReceived.cs
- AppSettingsExpressionEditor.cs
- DataSourceControlBuilder.cs
- TreeNodeEventArgs.cs
- ChannelCredentials.cs
- XmlSchemaCompilationSettings.cs
- QueryStringParameter.cs
- EntityKey.cs
- QilExpression.cs
- XpsColorContext.cs
- EntityDataSourceMemberPath.cs
- EntryWrittenEventArgs.cs
- SQLGuidStorage.cs
- Stack.cs
- XmlExpressionDumper.cs
- SizeChangedInfo.cs
- followingquery.cs
- FrameworkElementAutomationPeer.cs
- CodePropertyReferenceExpression.cs
- WebBrowserDocumentCompletedEventHandler.cs
- LeftCellWrapper.cs
- ContainerSelectorBehavior.cs
- SpecularMaterial.cs
- ValuePatternIdentifiers.cs
- ConfigXmlWhitespace.cs
- PrefixQName.cs
- smtppermission.cs
- BufferedGraphicsContext.cs
- Metadata.cs
- PersonalizationProviderHelper.cs
- PermissionSetEnumerator.cs
- ClientTarget.cs
- AuthorizationPolicyTypeElement.cs
- AuthorizationRuleCollection.cs
- CodeRegionDirective.cs
- DataSourceSerializationException.cs
- SmtpReplyReader.cs
- XpsException.cs
- RtfControlWordInfo.cs
- DatagridviewDisplayedBandsData.cs
- SoapMessage.cs
- ObjectPersistData.cs
- FormatterConverter.cs
- DefaultSection.cs
- EventRecordWrittenEventArgs.cs
- DataGridViewComboBoxCell.cs
- MobileUserControl.cs
- _LocalDataStoreMgr.cs
- ComplexTypeEmitter.cs
- BuildResultCache.cs
- DataKey.cs
- StreamHelper.cs
- TemplateModeChangedEventArgs.cs
- CurrentTimeZone.cs
- FixedSOMGroup.cs
- RenderData.cs
- ProfileEventArgs.cs
- RestHandler.cs
- EntityWithKeyStrategy.cs
- _LocalDataStore.cs
- FileInfo.cs
- CommandBinding.cs
- ImageKeyConverter.cs
- BamlLocalizationDictionary.cs
- ProgressBar.cs
- ContainerCodeDomSerializer.cs
- EnglishPluralizationService.cs
- SessionIDManager.cs
- StateItem.cs
- SafeRightsManagementHandle.cs
- DATA_BLOB.cs
- ImageCodecInfo.cs
- ListViewGroupConverter.cs
- CodeIterationStatement.cs
- HotCommands.cs
- IArgumentProvider.cs
- EncodingNLS.cs
- CorrelationValidator.cs
- NTAccount.cs
- KeyEventArgs.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- clipboard.cs
- IProvider.cs
- SocketAddress.cs
- UInt64Storage.cs
- SAPIEngineTypes.cs
- UrlPath.cs