Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Synthesis / VoiceInfo.cs / 1 / VoiceInfo.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.Synthesis; using System.Speech.Internal.ObjectTokens; using System.Xml; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Synthesis { ////// TODOC /// [DebuggerDisplay ("{(_name != null ? \"'\" + _name + \"' \" : \"\") + (_culture != null ? \" '\" + _culture.ToString () + \"' \" : \"\") + (_gender != VoiceGender.NotSet ? \" '\" + _gender.ToString () + \"' \" : \"\") + (_age != VoiceAge.NotSet ? \" '\" + _age.ToString () + \"' \" : \"\") + (_variant > 0 ? \" \" + _variant.ToString () : \"\")}")] [Serializable] public class VoiceInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// TODOC /// /// internal VoiceInfo (string name) { Helpers.ThrowIfEmptyOrNull (name, "name"); _name = name; } ////// TODOC /// /// internal VoiceInfo (CultureInfo culture) { // Fails if no culture is provided Helpers.ThrowIfNull (culture, "culture"); if (culture.Equals (CultureInfo.InvariantCulture)) { throw new ArgumentException (SR.Get (SRID.InvariantCultureInfo), "culture"); } _culture = culture; } internal VoiceInfo (VoiceObjectToken token) { _registryKeyPath = token._sKeyId; // Retriece the token name _id = token.Name; // Retrieve default display name _description = token.Description; // Get other attributes _name = token.TokenName (); SsmlParserHelpers.TryConvertAge (token.Age.ToLowerInvariant (), out _age); SsmlParserHelpers.TryConvertGender (token.Gender.ToLowerInvariant (), out _gender); string langId; if (token.Attributes.TryGetString ("Language", out langId)) { _culture = SapiAttributeParser.GetCultureInfoFromLanguageString (langId); } string assemblyName; if (token.TryGetString ("Assembly", out assemblyName)) { _assemblyName = assemblyName; } string clsid; if (token.TryGetString ("CLSID", out clsid)) { _clsid = clsid; } if (token.Attributes != null) { // Enum all values and add to custom table foreach (string keyName in token.Attributes.GetValueNames ()) { string attributeValue; if (token.Attributes.TryGetString (keyName, out attributeValue)) { _attributes.InternalDictionary.Add (keyName, attributeValue); } } } #if !SPEECHSERVER string audioFormats; if (token.Attributes != null && token.Attributes.TryGetString ("AudioFormats", out audioFormats)) { _audioFormats = new ReadOnlyCollection(SapiAttributeParser.GetAudioFormatsFromString (audioFormats)); } else { _audioFormats = new ReadOnlyCollection (new List ()); } #else _category = token.VoiceCategory; #endif } /// /// TODOC /// /// internal VoiceInfo (VoiceGender gender) { _gender = gender; } ////// TODOC /// /// /// internal VoiceInfo (VoiceGender gender, VoiceAge age) { _gender = gender; _age = age; } ////// TODOC /// /// /// /// internal VoiceInfo (VoiceGender gender, VoiceAge age, int voiceAlternate) { if (voiceAlternate < 0) { throw new ArgumentOutOfRangeException ("voiceAlternate", SR.Get (SRID.PromptBuilderInvalidVariant)); } _gender = gender; _age = age; _variant = voiceAlternate + 1; } #if SPEECHSERVER ////// TODOC /// /// /// /// /// internal VoiceInfo (CultureInfo culture, VoiceGender gender, string name, VoiceAge age) : this (culture) { _name = name; _gender = gender; _age = age; } #endif #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods ////// Tests whether two AutomationIdentifier objects are equivalent /// public override bool Equals (object obj) { // PreSharp doesn't understand that if obj is null then this will return false. #pragma warning disable 6506 VoiceInfo voice = obj as VoiceInfo; return voice != null && _name == voice._name && (_age == voice._age || _age == VoiceAge.NotSet || voice._age == VoiceAge.NotSet) && (_gender == voice._gender || _gender == VoiceGender.NotSet || voice._gender == VoiceGender.NotSet) && (_culture == null || voice._culture == null || _culture.Equals (voice._culture)); #pragma warning restore 6506 } ////// Overrides Object.GetHashCode() /// public override int GetHashCode () { return _name.GetHashCode (); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region public Properties ////// TODOC /// ///public VoiceGender Gender { get { return _gender; } } /// /// TODOC /// ///public VoiceAge Age { get { return _age; } } /// /// TODOC /// ///public string Name { get { return _name; } } /// /// TODOC /// /// Return a copy of the internal Language set. This disable client /// applications to modify the internal languages list. /// ///public CultureInfo Culture { get { return _culture; } } /// /// TODOC /// ///public string Id { get { return _id; } } /// /// TODOC /// ///public string Description { get { return _description != null ? _description : string.Empty; } } #if !SPEECHSERVER /// /// TODOC /// ///[EditorBrowsable (EditorBrowsableState.Advanced)] public ReadOnlyCollection SupportedAudioFormats { get { return _audioFormats; } } #endif /// /// TODOC /// ///[EditorBrowsable (EditorBrowsableState.Advanced)] public IDictionary AdditionalInfo { get { return _attributes; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods internal static bool ValidateGender (VoiceGender gender) { return gender == VoiceGender.Female || gender == VoiceGender.Male || gender == VoiceGender.Neutral || gender == VoiceGender.NotSet; } internal static bool ValidateAge (VoiceAge age) { return age == VoiceAge.Adult || age == VoiceAge.Child || age == VoiceAge.NotSet || age == VoiceAge.Senior || age == VoiceAge.Teen; } #endregion //******************************************************************* // // Internal Property // //******************************************************************* #region Internal Property /// /// TODOC /// ///internal int Variant { get { return _variant; } } /// /// TODOC /// ///internal string AssemblyName { get { return _assemblyName; } } /// /// TODOC /// ///internal string Clsid { get { return _clsid; } } /// /// TODOC /// ///internal string RegistryKeyPath { get { return _registryKeyPath; } } #if SPEECHSERVER internal VoiceCategory VoiceCategory { get { return _category; } } #endif #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _name; private CultureInfo _culture; private VoiceGender _gender = VoiceGender.NotSet; private VoiceAge _age; private int _variant = -1; [NonSerialized] private string _id; [NonSerialized] private string _registryKeyPath; [NonSerialized] private string _assemblyName; [NonSerialized] private string _clsid; [NonSerialized] private string _description; [NonSerialized] private ReadOnlyDictionary _attributes = new ReadOnlyDictionary (); #if !SPEECHSERVER [NonSerialized] private ReadOnlyCollection _audioFormats; #else VoiceCategory _category; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Speech.AudioFormat; using System.Speech.Internal; using System.Speech.Internal.Synthesis; using System.Speech.Internal.ObjectTokens; using System.Xml; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Synthesis { ////// TODOC /// [DebuggerDisplay ("{(_name != null ? \"'\" + _name + \"' \" : \"\") + (_culture != null ? \" '\" + _culture.ToString () + \"' \" : \"\") + (_gender != VoiceGender.NotSet ? \" '\" + _gender.ToString () + \"' \" : \"\") + (_age != VoiceAge.NotSet ? \" '\" + _age.ToString () + \"' \" : \"\") + (_variant > 0 ? \" \" + _variant.ToString () : \"\")}")] [Serializable] public class VoiceInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// TODOC /// /// internal VoiceInfo (string name) { Helpers.ThrowIfEmptyOrNull (name, "name"); _name = name; } ////// TODOC /// /// internal VoiceInfo (CultureInfo culture) { // Fails if no culture is provided Helpers.ThrowIfNull (culture, "culture"); if (culture.Equals (CultureInfo.InvariantCulture)) { throw new ArgumentException (SR.Get (SRID.InvariantCultureInfo), "culture"); } _culture = culture; } internal VoiceInfo (VoiceObjectToken token) { _registryKeyPath = token._sKeyId; // Retriece the token name _id = token.Name; // Retrieve default display name _description = token.Description; // Get other attributes _name = token.TokenName (); SsmlParserHelpers.TryConvertAge (token.Age.ToLowerInvariant (), out _age); SsmlParserHelpers.TryConvertGender (token.Gender.ToLowerInvariant (), out _gender); string langId; if (token.Attributes.TryGetString ("Language", out langId)) { _culture = SapiAttributeParser.GetCultureInfoFromLanguageString (langId); } string assemblyName; if (token.TryGetString ("Assembly", out assemblyName)) { _assemblyName = assemblyName; } string clsid; if (token.TryGetString ("CLSID", out clsid)) { _clsid = clsid; } if (token.Attributes != null) { // Enum all values and add to custom table foreach (string keyName in token.Attributes.GetValueNames ()) { string attributeValue; if (token.Attributes.TryGetString (keyName, out attributeValue)) { _attributes.InternalDictionary.Add (keyName, attributeValue); } } } #if !SPEECHSERVER string audioFormats; if (token.Attributes != null && token.Attributes.TryGetString ("AudioFormats", out audioFormats)) { _audioFormats = new ReadOnlyCollection(SapiAttributeParser.GetAudioFormatsFromString (audioFormats)); } else { _audioFormats = new ReadOnlyCollection (new List ()); } #else _category = token.VoiceCategory; #endif } /// /// TODOC /// /// internal VoiceInfo (VoiceGender gender) { _gender = gender; } ////// TODOC /// /// /// internal VoiceInfo (VoiceGender gender, VoiceAge age) { _gender = gender; _age = age; } ////// TODOC /// /// /// /// internal VoiceInfo (VoiceGender gender, VoiceAge age, int voiceAlternate) { if (voiceAlternate < 0) { throw new ArgumentOutOfRangeException ("voiceAlternate", SR.Get (SRID.PromptBuilderInvalidVariant)); } _gender = gender; _age = age; _variant = voiceAlternate + 1; } #if SPEECHSERVER ////// TODOC /// /// /// /// /// internal VoiceInfo (CultureInfo culture, VoiceGender gender, string name, VoiceAge age) : this (culture) { _name = name; _gender = gender; _age = age; } #endif #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods ////// Tests whether two AutomationIdentifier objects are equivalent /// public override bool Equals (object obj) { // PreSharp doesn't understand that if obj is null then this will return false. #pragma warning disable 6506 VoiceInfo voice = obj as VoiceInfo; return voice != null && _name == voice._name && (_age == voice._age || _age == VoiceAge.NotSet || voice._age == VoiceAge.NotSet) && (_gender == voice._gender || _gender == VoiceGender.NotSet || voice._gender == VoiceGender.NotSet) && (_culture == null || voice._culture == null || _culture.Equals (voice._culture)); #pragma warning restore 6506 } ////// Overrides Object.GetHashCode() /// public override int GetHashCode () { return _name.GetHashCode (); } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region public Properties ////// TODOC /// ///public VoiceGender Gender { get { return _gender; } } /// /// TODOC /// ///public VoiceAge Age { get { return _age; } } /// /// TODOC /// ///public string Name { get { return _name; } } /// /// TODOC /// /// Return a copy of the internal Language set. This disable client /// applications to modify the internal languages list. /// ///public CultureInfo Culture { get { return _culture; } } /// /// TODOC /// ///public string Id { get { return _id; } } /// /// TODOC /// ///public string Description { get { return _description != null ? _description : string.Empty; } } #if !SPEECHSERVER /// /// TODOC /// ///[EditorBrowsable (EditorBrowsableState.Advanced)] public ReadOnlyCollection SupportedAudioFormats { get { return _audioFormats; } } #endif /// /// TODOC /// ///[EditorBrowsable (EditorBrowsableState.Advanced)] public IDictionary AdditionalInfo { get { return _attributes; } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods internal static bool ValidateGender (VoiceGender gender) { return gender == VoiceGender.Female || gender == VoiceGender.Male || gender == VoiceGender.Neutral || gender == VoiceGender.NotSet; } internal static bool ValidateAge (VoiceAge age) { return age == VoiceAge.Adult || age == VoiceAge.Child || age == VoiceAge.NotSet || age == VoiceAge.Senior || age == VoiceAge.Teen; } #endregion //******************************************************************* // // Internal Property // //******************************************************************* #region Internal Property /// /// TODOC /// ///internal int Variant { get { return _variant; } } /// /// TODOC /// ///internal string AssemblyName { get { return _assemblyName; } } /// /// TODOC /// ///internal string Clsid { get { return _clsid; } } /// /// TODOC /// ///internal string RegistryKeyPath { get { return _registryKeyPath; } } #if SPEECHSERVER internal VoiceCategory VoiceCategory { get { return _category; } } #endif #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private string _name; private CultureInfo _culture; private VoiceGender _gender = VoiceGender.NotSet; private VoiceAge _age; private int _variant = -1; [NonSerialized] private string _id; [NonSerialized] private string _registryKeyPath; [NonSerialized] private string _assemblyName; [NonSerialized] private string _clsid; [NonSerialized] private string _description; [NonSerialized] private ReadOnlyDictionary _attributes = new ReadOnlyDictionary (); #if !SPEECHSERVER [NonSerialized] private ReadOnlyCollection _audioFormats; #else VoiceCategory _category; #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
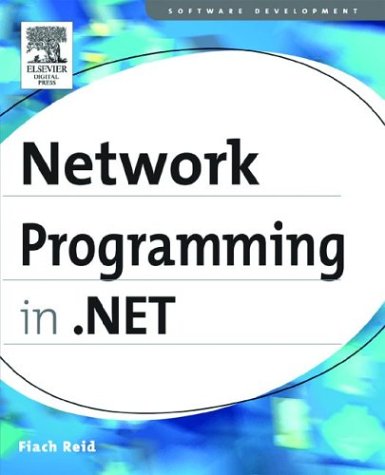
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripSplitButton.cs
- DbProviderFactoriesConfigurationHandler.cs
- CornerRadiusConverter.cs
- TemporaryBitmapFile.cs
- AggregatePushdown.cs
- BamlLocalizabilityResolver.cs
- CapabilitiesState.cs
- WinFormsSpinner.cs
- securitymgrsite.cs
- ListSortDescription.cs
- ThreadExceptionDialog.cs
- ModuleConfigurationInfo.cs
- HandlerBase.cs
- PersonalizationProviderHelper.cs
- MonikerProxyAttribute.cs
- ControlAdapter.cs
- DataGridViewRowStateChangedEventArgs.cs
- NetStream.cs
- SourceItem.cs
- TimeoutValidationAttribute.cs
- AssemblyLoader.cs
- SqlCachedBuffer.cs
- TreeNode.cs
- ByteStorage.cs
- DataTableTypeConverter.cs
- Help.cs
- DataGridViewImageColumn.cs
- UnSafeCharBuffer.cs
- StylusPointDescription.cs
- CodeConditionStatement.cs
- DebugView.cs
- SystemIPGlobalStatistics.cs
- StructuralCache.cs
- CompositeScriptReference.cs
- GPStream.cs
- ContainerControlDesigner.cs
- TimeManager.cs
- DbTransaction.cs
- Wizard.cs
- TreeBuilder.cs
- RubberbandSelector.cs
- ObjectCloneHelper.cs
- MasterPage.cs
- CustomAttribute.cs
- DoneReceivingAsyncResult.cs
- ClientSponsor.cs
- ContentControl.cs
- HtmlControlDesigner.cs
- Schema.cs
- Helpers.cs
- GeneratedContractType.cs
- Calendar.cs
- EntityKeyElement.cs
- AdapterUtil.cs
- HttpEncoder.cs
- MaskInputRejectedEventArgs.cs
- DefaultTraceListener.cs
- SqlFormatter.cs
- BadImageFormatException.cs
- AxisAngleRotation3D.cs
- Win32Exception.cs
- HMACSHA256.cs
- BitStack.cs
- MailWriter.cs
- ResolveNameEventArgs.cs
- ImageListStreamer.cs
- ProcessingInstructionAction.cs
- SourceFilter.cs
- UriExt.cs
- XmlEntityReference.cs
- WebZone.cs
- TcpChannelListener.cs
- DelegateSerializationHolder.cs
- EasingKeyFrames.cs
- HttpWriter.cs
- SchemaElementDecl.cs
- Process.cs
- XmlArrayItemAttribute.cs
- RemotingServices.cs
- FileUtil.cs
- ToolStripGrip.cs
- AuthenticationService.cs
- EventProviderTraceListener.cs
- RegexTree.cs
- ConfigXmlElement.cs
- FieldDescriptor.cs
- RawContentTypeMapper.cs
- SmiRecordBuffer.cs
- QilCloneVisitor.cs
- ToolBarOverflowPanel.cs
- PackageDigitalSignatureManager.cs
- ValidatedControlConverter.cs
- SystemTcpConnection.cs
- EmissiveMaterial.cs
- FloaterParaClient.cs
- SpellCheck.cs
- XPathNode.cs
- safelink.cs
- IdentitySection.cs
- KeyValueConfigurationCollection.cs