Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / TypeSystem / AssemblyLoader.cs / 1305376 / AssemblyLoader.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections.Generic; using System.Reflection; using System.ComponentModel.Design; using System.IO; internal class AssemblyLoader { private Assembly assembly = null; private AssemblyName assemblyName = null; private TypeProvider typeProvider = null; private bool isLocalAssembly; internal AssemblyLoader(TypeProvider typeProvider, string filePath) { this.isLocalAssembly = false; this.typeProvider = typeProvider; if (File.Exists(filePath)) { AssemblyName asmName = AssemblyName.GetAssemblyName(filePath); if (asmName != null) { // Try loading the assembly using type resolution service first. ITypeResolutionService trs = (ITypeResolutionService)typeProvider.GetService(typeof(ITypeResolutionService)); if (trs != null) { try { this.assembly = trs.GetAssembly(asmName); // if (this.assembly == null && asmName.GetPublicKeyToken() != null && (asmName.GetPublicKeyToken().GetLength(0) == 0) && asmName.GetPublicKey() != null && (asmName.GetPublicKey().GetLength(0) == 0)) { AssemblyName partialName = (AssemblyName)asmName.Clone(); partialName.SetPublicKey(null); partialName.SetPublicKeyToken(null); this.assembly = trs.GetAssembly(partialName); } } catch { // Eat up any exceptions! } } // If type resolution service wasn't available or it failed use Assembly.Load if (this.assembly == null) { try { if (MultiTargetingInfo.MultiTargetingUtilities.IsFrameworkReferenceAssembly(filePath)) { this.assembly = Assembly.Load(asmName.FullName); } else { this.assembly = Assembly.Load(asmName); } } catch { // Eat up any exceptions! } } } // If Assembly.Load also failed, use Assembly.LoadFrom if (this.assembly == null) { this.assembly = Assembly.LoadFrom(filePath); } } else { // TypeProvider will handle this and report the error throw new FileNotFoundException(); } } internal AssemblyLoader(TypeProvider typeProvider, Assembly assembly, bool isLocalAssembly) { this.isLocalAssembly = isLocalAssembly; this.typeProvider = typeProvider; this.assembly = assembly; } internal Type GetType(string typeName) { // if (this.assembly != null) { Type type = null; try { type = this.assembly.GetType(typeName); } catch (ArgumentException) { // we eat these exeptions in our type system } if ((type != null) && (type.IsPublic || type.IsNestedPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate))) return type; } return null; } internal Type[] GetTypes() { ListfilteredTypes = new List (); if (this.assembly != null) { // foreach (Type type in this.assembly.GetTypes()) { // if(type.IsPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate)) filteredTypes.Add(type); } } return filteredTypes.ToArray(); } // we cache the AssemblyName as Assembly.GetName() is expensive internal AssemblyName AssemblyName { get { if (this.assemblyName == null) this.assemblyName = this.assembly.GetName(true); return this.assemblyName; } } internal Assembly Assembly { get { return this.assembly; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections.Generic; using System.Reflection; using System.ComponentModel.Design; using System.IO; internal class AssemblyLoader { private Assembly assembly = null; private AssemblyName assemblyName = null; private TypeProvider typeProvider = null; private bool isLocalAssembly; internal AssemblyLoader(TypeProvider typeProvider, string filePath) { this.isLocalAssembly = false; this.typeProvider = typeProvider; if (File.Exists(filePath)) { AssemblyName asmName = AssemblyName.GetAssemblyName(filePath); if (asmName != null) { // Try loading the assembly using type resolution service first. ITypeResolutionService trs = (ITypeResolutionService)typeProvider.GetService(typeof(ITypeResolutionService)); if (trs != null) { try { this.assembly = trs.GetAssembly(asmName); // if (this.assembly == null && asmName.GetPublicKeyToken() != null && (asmName.GetPublicKeyToken().GetLength(0) == 0) && asmName.GetPublicKey() != null && (asmName.GetPublicKey().GetLength(0) == 0)) { AssemblyName partialName = (AssemblyName)asmName.Clone(); partialName.SetPublicKey(null); partialName.SetPublicKeyToken(null); this.assembly = trs.GetAssembly(partialName); } } catch { // Eat up any exceptions! } } // If type resolution service wasn't available or it failed use Assembly.Load if (this.assembly == null) { try { if (MultiTargetingInfo.MultiTargetingUtilities.IsFrameworkReferenceAssembly(filePath)) { this.assembly = Assembly.Load(asmName.FullName); } else { this.assembly = Assembly.Load(asmName); } } catch { // Eat up any exceptions! } } } // If Assembly.Load also failed, use Assembly.LoadFrom if (this.assembly == null) { this.assembly = Assembly.LoadFrom(filePath); } } else { // TypeProvider will handle this and report the error throw new FileNotFoundException(); } } internal AssemblyLoader(TypeProvider typeProvider, Assembly assembly, bool isLocalAssembly) { this.isLocalAssembly = isLocalAssembly; this.typeProvider = typeProvider; this.assembly = assembly; } internal Type GetType(string typeName) { // if (this.assembly != null) { Type type = null; try { type = this.assembly.GetType(typeName); } catch (ArgumentException) { // we eat these exeptions in our type system } if ((type != null) && (type.IsPublic || type.IsNestedPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate))) return type; } return null; } internal Type[] GetTypes() { List filteredTypes = new List (); if (this.assembly != null) { // foreach (Type type in this.assembly.GetTypes()) { // if(type.IsPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate)) filteredTypes.Add(type); } } return filteredTypes.ToArray(); } // we cache the AssemblyName as Assembly.GetName() is expensive internal AssemblyName AssemblyName { get { if (this.assemblyName == null) this.assemblyName = this.assembly.GetName(true); return this.assemblyName; } } internal Assembly Assembly { get { return this.assembly; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
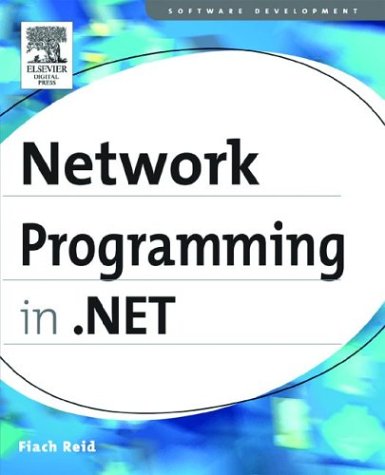
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlServer2KCompatibilityAnnotation.cs
- SafeCryptoHandles.cs
- CreateUserWizardAutoFormat.cs
- SQLBytes.cs
- SharedPersonalizationStateInfo.cs
- DataTableClearEvent.cs
- WebDescriptionAttribute.cs
- ConfigurationProviderException.cs
- PresentationAppDomainManager.cs
- ProcessHostFactoryHelper.cs
- LookupBindingPropertiesAttribute.cs
- SecurityPolicySection.cs
- BamlBinaryReader.cs
- InvokeProviderWrapper.cs
- WeakReference.cs
- Helpers.cs
- TranslateTransform3D.cs
- SafeFindHandle.cs
- AssemblyResourceLoader.cs
- Visual3DCollection.cs
- PassportPrincipal.cs
- ToolStripItemBehavior.cs
- UnionExpr.cs
- AccessedThroughPropertyAttribute.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- ListBase.cs
- DataGridViewRowStateChangedEventArgs.cs
- ListViewUpdatedEventArgs.cs
- Menu.cs
- JsonObjectDataContract.cs
- ExpanderAutomationPeer.cs
- SqlDataSourceView.cs
- CallbackHandler.cs
- WebHttpElement.cs
- AssignDesigner.xaml.cs
- ScriptModule.cs
- AmbientLight.cs
- ModifierKeysValueSerializer.cs
- ReflectionHelper.cs
- Camera.cs
- Win32SafeHandles.cs
- SafeWaitHandle.cs
- Executor.cs
- Assembly.cs
- TextTreeInsertUndoUnit.cs
- SQLBinaryStorage.cs
- SqlAggregateChecker.cs
- CssStyleCollection.cs
- Base64Encoding.cs
- PageParserFilter.cs
- SqlClientPermission.cs
- ApplicationActivator.cs
- OperatorExpressions.cs
- DependencyPropertyAttribute.cs
- ExtensionFile.cs
- InstancePersistenceContext.cs
- Range.cs
- Padding.cs
- ConfigXmlComment.cs
- XmlElementElementCollection.cs
- Compiler.cs
- SqlExpander.cs
- ComponentResourceKey.cs
- OperationCanceledException.cs
- ReaderContextStackData.cs
- HttpCapabilitiesBase.cs
- WebPartVerbCollection.cs
- DataGridViewHitTestInfo.cs
- BufferedGraphicsManager.cs
- SpecialFolderEnumConverter.cs
- MouseActionConverter.cs
- TogglePattern.cs
- HttpValueCollection.cs
- BitmapCodecInfoInternal.cs
- CodeAttributeDeclaration.cs
- WindowsScrollBar.cs
- LineUtil.cs
- SoapFormatter.cs
- SchemaImporter.cs
- InlineCollection.cs
- MethodExpr.cs
- XslAst.cs
- PropertyEmitterBase.cs
- LayoutTableCell.cs
- XsltFunctions.cs
- ExpressionBuilder.cs
- _NegoStream.cs
- CqlParserHelpers.cs
- SafeFileHandle.cs
- HyperLinkField.cs
- handlecollector.cs
- SubpageParagraph.cs
- DataServiceRequestOfT.cs
- RightsManagementProvider.cs
- ComponentEditorPage.cs
- AdapterDictionary.cs
- AppDomainManager.cs
- DoubleSumAggregationOperator.cs
- DataContractSerializer.cs
- Sql8ConformanceChecker.cs