Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / AsyncOperation.cs / 1 / AsyncOperation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System.Security.Permissions; using System.Threading; [HostProtection(SharedState = true)] public sealed class AsyncOperation { private SynchronizationContext syncContext; private object userSuppliedState; private bool alreadyCompleted; ////// Constructor. Protected to avoid unwitting usage - AsyncOperation objects /// are typically created by AsyncOperationManager calling CreateOperation. /// private AsyncOperation(object userSuppliedState, SynchronizationContext syncContext) { this.userSuppliedState = userSuppliedState; this.syncContext = syncContext; this.alreadyCompleted = false; this.syncContext.OperationStarted(); } ////// Destructor. Guarantees that [....] context will always get notified of completion. /// ~AsyncOperation() { if (!alreadyCompleted && syncContext != null) { syncContext.OperationCompleted(); } } public object UserSuppliedState { get { return userSuppliedState; } } ///public SynchronizationContext SynchronizationContext { get { return syncContext; } } public void Post(SendOrPostCallback d, object arg) { VerifyNotCompleted(); VerifyDelegateNotNull(d); syncContext.Post(d, arg); } public void PostOperationCompleted(SendOrPostCallback d, object arg) { Post(d, arg); OperationCompletedCore(); } public void OperationCompleted() { VerifyNotCompleted(); OperationCompletedCore(); } private void OperationCompletedCore() { try { syncContext.OperationCompleted(); } finally { alreadyCompleted = true; GC.SuppressFinalize(this); } } private void VerifyNotCompleted() { if (alreadyCompleted) { throw new InvalidOperationException(SR.GetString(SR.Async_OperationAlreadyCompleted)); } } private void VerifyDelegateNotNull(SendOrPostCallback d) { if (d == null) { throw new ArgumentNullException(SR.GetString(SR.Async_NullDelegate), "d"); } } /// /// Only for use by AsyncOperationManager to create new AsyncOperation objects /// internal static AsyncOperation CreateOperation(object userSuppliedState, SynchronizationContext syncContext) { AsyncOperation newOp = new AsyncOperation(userSuppliedState, syncContext); return newOp; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System.Security.Permissions; using System.Threading; [HostProtection(SharedState = true)] public sealed class AsyncOperation { private SynchronizationContext syncContext; private object userSuppliedState; private bool alreadyCompleted; ////// Constructor. Protected to avoid unwitting usage - AsyncOperation objects /// are typically created by AsyncOperationManager calling CreateOperation. /// private AsyncOperation(object userSuppliedState, SynchronizationContext syncContext) { this.userSuppliedState = userSuppliedState; this.syncContext = syncContext; this.alreadyCompleted = false; this.syncContext.OperationStarted(); } ////// Destructor. Guarantees that [....] context will always get notified of completion. /// ~AsyncOperation() { if (!alreadyCompleted && syncContext != null) { syncContext.OperationCompleted(); } } public object UserSuppliedState { get { return userSuppliedState; } } ///public SynchronizationContext SynchronizationContext { get { return syncContext; } } public void Post(SendOrPostCallback d, object arg) { VerifyNotCompleted(); VerifyDelegateNotNull(d); syncContext.Post(d, arg); } public void PostOperationCompleted(SendOrPostCallback d, object arg) { Post(d, arg); OperationCompletedCore(); } public void OperationCompleted() { VerifyNotCompleted(); OperationCompletedCore(); } private void OperationCompletedCore() { try { syncContext.OperationCompleted(); } finally { alreadyCompleted = true; GC.SuppressFinalize(this); } } private void VerifyNotCompleted() { if (alreadyCompleted) { throw new InvalidOperationException(SR.GetString(SR.Async_OperationAlreadyCompleted)); } } private void VerifyDelegateNotNull(SendOrPostCallback d) { if (d == null) { throw new ArgumentNullException(SR.GetString(SR.Async_NullDelegate), "d"); } } /// /// Only for use by AsyncOperationManager to create new AsyncOperation objects /// internal static AsyncOperation CreateOperation(object userSuppliedState, SynchronizationContext syncContext) { AsyncOperation newOp = new AsyncOperation(userSuppliedState, syncContext); return newOp; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
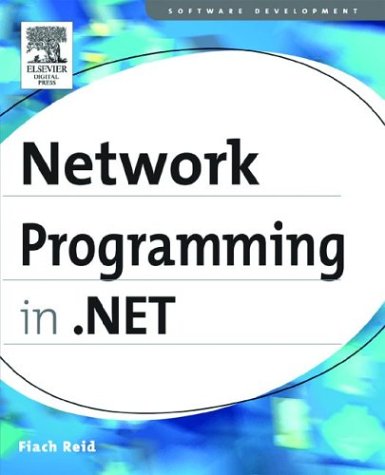
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypedAsyncResult.cs
- StreamInfo.cs
- RowToParametersTransformer.cs
- ProcessModelSection.cs
- SeparatorAutomationPeer.cs
- CaseInsensitiveHashCodeProvider.cs
- DesigntimeLicenseContextSerializer.cs
- DataObjectSettingDataEventArgs.cs
- WebBrowserNavigatingEventHandler.cs
- WebBrowserBase.cs
- Annotation.cs
- XmlSchemaSimpleTypeUnion.cs
- UrlMapping.cs
- AssemblyAssociatedContentFileAttribute.cs
- EventMappingSettings.cs
- StandardCommands.cs
- EventTrigger.cs
- RayMeshGeometry3DHitTestResult.cs
- CqlErrorHelper.cs
- regiisutil.cs
- CaseCqlBlock.cs
- ObjectContext.cs
- StylusEventArgs.cs
- StyleBamlRecordReader.cs
- OdbcConnectionPoolProviderInfo.cs
- EngineSiteSapi.cs
- ToolstripProfessionalRenderer.cs
- MetadataItemEmitter.cs
- SQLBoolean.cs
- MinimizableAttributeTypeConverter.cs
- StickyNoteContentControl.cs
- OutputBuffer.cs
- HashStream.cs
- ExpressionBuilder.cs
- DbXmlEnabledProviderManifest.cs
- CachedCompositeFamily.cs
- EnumValAlphaComparer.cs
- SBCSCodePageEncoding.cs
- AssociatedControlConverter.cs
- EventLogEntry.cs
- GlobalAllocSafeHandle.cs
- WebBrowsableAttribute.cs
- RowSpanVector.cs
- SuppressMergeCheckAttribute.cs
- Parser.cs
- SqlDataReader.cs
- TypeDescriptionProviderAttribute.cs
- AttributeUsageAttribute.cs
- VSDExceptions.cs
- DiagnosticsConfiguration.cs
- WindowsTooltip.cs
- NamespaceDecl.cs
- SqlDataSourceCache.cs
- PipelineModuleStepContainer.cs
- FlowLayoutSettings.cs
- CustomSignedXml.cs
- ColorConvertedBitmap.cs
- NameSpaceEvent.cs
- EmbeddedObject.cs
- ArraySegment.cs
- Condition.cs
- DataSourceCache.cs
- MessageSmuggler.cs
- TextModifierScope.cs
- ScriptManager.cs
- IPPacketInformation.cs
- CodeAssignStatement.cs
- GridViewUpdatedEventArgs.cs
- XmlUnspecifiedAttribute.cs
- FontResourceCache.cs
- PersonalizationStateInfo.cs
- SafeNativeMethods.cs
- ContainerUtilities.cs
- DataGridViewImageCell.cs
- ChoiceConverter.cs
- Timer.cs
- TransformerConfigurationWizardBase.cs
- ValidatorCompatibilityHelper.cs
- NameValueCollection.cs
- DesignTimeTemplateParser.cs
- DependencyObject.cs
- PropertyFilterAttribute.cs
- DeclaredTypeElementCollection.cs
- TypeGeneratedEventArgs.cs
- WindowsAuthenticationModule.cs
- PointCollection.cs
- RadioButtonRenderer.cs
- InstanceDataCollectionCollection.cs
- DictionaryBase.cs
- Compiler.cs
- DefaultCommandConverter.cs
- ping.cs
- MultiPageTextView.cs
- ScriptResourceInfo.cs
- DbConnectionPoolGroupProviderInfo.cs
- SocketInformation.cs
- InstanceStore.cs
- IUnknownConstantAttribute.cs
- DataObject.cs
- EventLogPermission.cs