Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / FontCache / FontResourceCache.cs / 1305600 / FontResourceCache.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: FontResourceCache class maintains the list of font resources included with an application. // // // History: // 08/09/2005 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.IO.Packaging; using System.Reflection; using System.Windows.Navigation; using MS.Internal.Resources; namespace MS.Internal.FontCache { internal static class FontResourceCache { private static void ConstructFontResourceCache(Assembly entryAssembly, Dictionary> folderResourceMap) { // For entryAssembly build a set of mapping from paths to entries that describe each resource. Dictionary contentFiles = ContentFileHelper.GetContentFiles(entryAssembly); if (contentFiles != null) { foreach (string contentFile in contentFiles.Keys) { AddResourceToFolderMap(folderResourceMap, contentFile); } } IList resourceList = new ResourceManagerWrapper(entryAssembly).ResourceList; if (resourceList != null) { foreach (string resource in resourceList) { AddResourceToFolderMap(folderResourceMap, resource); } } } internal static List LookupFolder(Uri uri) { // The input URI may specify a folder which is invalid for pack URIs. If so, // create a valid pack URI by adding a fake filename. bool isFolder = IsFolderUri(uri); if (isFolder) { uri = new Uri(uri, FakeFileName); } // The input Uri should now be a valid absolute pack application Uri. // The caller (FontSourceCollection) guarantees that. // Perform a sanity check to make sure the assumption stays the same. Debug.Assert(uri.IsAbsoluteUri && uri.Scheme == PackUriHelper.UriSchemePack && BaseUriHelper.IsPackApplicationUri(uri)); Assembly uriAssembly; string escapedPath; BaseUriHelper.GetAssemblyAndPartNameFromPackAppUri(uri, out uriAssembly, out escapedPath); if (uriAssembly == null) return null; // If we added a fake filename to the uri, remove it from the escaped path. if (isFolder) { Debug.Assert(escapedPath.EndsWith(FakeFileName, StringComparison.OrdinalIgnoreCase)); escapedPath = escapedPath.Substring(0, escapedPath.Length - FakeFileName.Length); } Dictionary > folderResourceMap; lock (_assemblyCaches) { if (!_assemblyCaches.TryGetValue(uriAssembly, out folderResourceMap)) { folderResourceMap = new Dictionary >(StringComparer.OrdinalIgnoreCase); ConstructFontResourceCache(uriAssembly, folderResourceMap); _assemblyCaches.Add(uriAssembly, folderResourceMap); } } List ret; folderResourceMap.TryGetValue(escapedPath, out ret); return ret; } /// /// Determines if a font family URI is a folder. Folder URIs are not valid pack URIs /// and therefore require special handling. /// private static bool IsFolderUri(Uri uri) { string path = uri.GetComponents(UriComponents.Path, UriFormat.SafeUnescaped); return path.Length == 0 || path[path.Length - 1] == '/'; } // Fake file name, which we add to a folder URI and then remove from the resulting // escaped path. This is to work around the fact that pack URIs cannot be folder URIs. // This string could be any valid file name that does not require escaping. private const string FakeFileName = "X"; private static void AddResourceToFolderMap(Dictionary> folderResourceMap, string resourceFullName) { // Split the resource path into a directory and file name part. string folderName; string fileName; int indexOfLastSlash = resourceFullName.LastIndexOf('/'); if (indexOfLastSlash == -1) { // The resource is in the root directory, folderName is empty. folderName = String.Empty; fileName = resourceFullName; } else { folderName = resourceFullName.Substring(0, indexOfLastSlash + 1); fileName = resourceFullName.Substring(indexOfLastSlash + 1); } string extension = Path.GetExtension(fileName); bool isComposite; if (!Util.IsSupportedFontExtension(extension, out isComposite)) return; // We add entries for a font file to two collections: // one is the folder containing the file, another is the file itself, // since we allow explicit file names in FontFamily syntax if (!folderResourceMap.ContainsKey(folderName)) folderResourceMap[folderName] = new List (1); folderResourceMap[folderName].Add(fileName); Debug.Assert(!folderResourceMap.ContainsKey(resourceFullName)); folderResourceMap[resourceFullName] = new List (1); folderResourceMap[resourceFullName].Add(String.Empty); } private static Dictionary >> _assemblyCaches = new Dictionary >>(1); // Set the initial capacity to 1 because a single entry assembly is the most common case. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
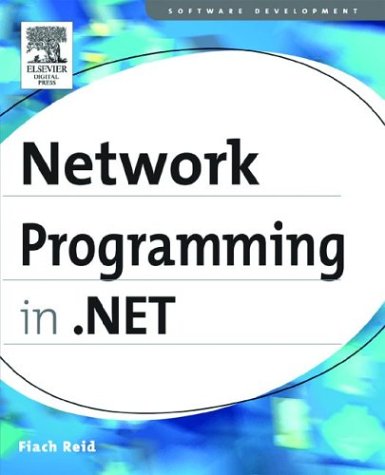
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MaxSessionCountExceededException.cs
- WindowsRebar.cs
- PresentationUIStyleResources.cs
- LinqDataSourceSelectEventArgs.cs
- JavaScriptString.cs
- FactoryGenerator.cs
- GridViewRowCollection.cs
- PasswordBox.cs
- ProfileService.cs
- SqlTriggerAttribute.cs
- _DynamicWinsockMethods.cs
- PairComparer.cs
- SamlSubject.cs
- ListenerElementsCollection.cs
- RootNamespaceAttribute.cs
- DataServiceEntityAttribute.cs
- RawMouseInputReport.cs
- SaveFileDialogDesigner.cs
- RadialGradientBrush.cs
- XmlSortKey.cs
- TemplateXamlParser.cs
- Line.cs
- ConfigDefinitionUpdates.cs
- WeakReference.cs
- MetadataException.cs
- ValueProviderWrapper.cs
- CommandLineParser.cs
- XPathPatternBuilder.cs
- WebServiceData.cs
- SiteIdentityPermission.cs
- Knowncolors.cs
- SqlWorkflowPersistenceService.cs
- FocusManager.cs
- ObjectListGeneralPage.cs
- ScalarConstant.cs
- ZipIOCentralDirectoryFileHeader.cs
- StringFreezingAttribute.cs
- XmlElementElement.cs
- DistributedTransactionPermission.cs
- DataSet.cs
- DocumentOrderQuery.cs
- ColorPalette.cs
- Panel.cs
- DataColumn.cs
- PersistenceProviderDirectory.cs
- SecurityUniqueId.cs
- Wildcard.cs
- ControlOperationInvoker.cs
- UIElementCollection.cs
- MethodCallTranslator.cs
- ReflectionTypeLoadException.cs
- BindingExpressionBase.cs
- EditorZoneAutoFormat.cs
- SystemIPv6InterfaceProperties.cs
- Operand.cs
- TemplateControlCodeDomTreeGenerator.cs
- NativeWindow.cs
- WindowsStatusBar.cs
- FrameAutomationPeer.cs
- RuntimeResourceSet.cs
- CharEnumerator.cs
- SequenceDesigner.cs
- PropertyEntry.cs
- InstanceStore.cs
- TextHidden.cs
- ResourceAttributes.cs
- ConfigXmlSignificantWhitespace.cs
- WebPartHeaderCloseVerb.cs
- SHA512Managed.cs
- TableLayoutColumnStyleCollection.cs
- CommentEmitter.cs
- BufferedWebEventProvider.cs
- SoapHeaderException.cs
- OleDbFactory.cs
- SessionParameter.cs
- TimerElapsedEvenArgs.cs
- CaseInsensitiveComparer.cs
- ClonableStack.cs
- XmlUrlResolver.cs
- ConstantProjectedSlot.cs
- ExtensionCollection.cs
- TcpConnectionPool.cs
- Semaphore.cs
- DateTimeFormat.cs
- HashCryptoHandle.cs
- dataobject.cs
- DataSet.cs
- DiffuseMaterial.cs
- RTLAwareMessageBox.cs
- Viewport3DVisual.cs
- DiscoveryEndpoint.cs
- MonthChangedEventArgs.cs
- DataObjectEventArgs.cs
- StoreItemCollection.Loader.cs
- activationcontext.cs
- ObjectToken.cs
- ProxyHwnd.cs
- DataGridViewLinkColumn.cs
- FontFamilyValueSerializer.cs
- DataGridCaption.cs