Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / CaseCqlBlock.cs / 1 / CaseCqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class to capture CqlBlocks responsible for case statements -- these // are statements needed for generating the variables in the // multiconstants, i.e., complex types, entities, discriminators, etc internal class CaseCqlBlock : CqlBlock { #region Constructors // effects: Creates a case statememt CqlBlock with SELECT (slots), // FROM (child -- note has only has one child), WHERE (true), AS // (blockAliasNum). caseSlot indicates which slot in slots // corresponds to the single case statement being generated by this block internal CaseCqlBlock(SlotInfo[] slots, int caseSlot, CqlBlock child, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) : base(slots, new List(new CqlBlock[]{child}), whereClause, identifiers, blockAliasNum) { m_caseSlotInfo = slots[caseSlot]; } #endregion #region Fields private SlotInfo m_caseSlotInfo; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part StringUtil.IndentNewLine(builder, indentLevel); // Since we know that the case statement starts on a new line, we // can add a comment after SELECT Debug.Assert(m_caseSlotInfo.MemberPath != null, "We only construct real slots not boolean slots"); builder.Append("SELECT "); if (isTopLevel) { builder.Append("VALUE "); } builder.Append("-- Constructing ") .Append(m_caseSlotInfo.MemberPath.LastComponentName); Debug.Assert(Children.Count == 1, "Case can have exactly one child"); CqlBlock childBlock = Children[0]; // First emit the case statement "CASE ... END AS Alias" m_caseSlotInfo.AsCql(builder, childBlock.CqlAlias, indentLevel); if (false == isTopLevel) { builder.Append(" AS ") .Append(m_caseSlotInfo.CqlFieldAlias); } // Now get the remaining slots bool isFirst = true; // CHANGE_[....]_IMPROVE: Try to leverage GenerateProjectedtList foreach (SlotInfo slot in Slots) { if (false == slot.IsRequiredByParent || object.ReferenceEquals(slot, m_caseSlotInfo)) { continue; } if (isFirst == true) { // Add , after END and then start new line for first field builder.Append(", "); StringUtil.IndentNewLine(builder, indentLevel + 1); } else { // Simply add a comma for the next field builder.Append(", "); } slot.AsCql(builder, childBlock.CqlAlias, indentLevel); isFirst = false; } // The FROM part: FROM (ChildView) AS AliasName StringUtil.IndentNewLine(builder, indentLevel); builder.Append("FROM ("); // Get the child childBlock.AsCql(builder, false, indentLevel + 1); StringUtil.IndentNewLine(builder, indentLevel); builder.Append(") AS ") .Append(childBlock.CqlAlias); // Get the WHERE part only when the expression is not simply TRUE if (false == BoolExpression.EqualityComparer.Equals(WhereClause, BoolExpression.True)) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("WHERE "); WhereClause.AsCql(builder, childBlock.CqlAlias); } return builder; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class to capture CqlBlocks responsible for case statements -- these // are statements needed for generating the variables in the // multiconstants, i.e., complex types, entities, discriminators, etc internal class CaseCqlBlock : CqlBlock { #region Constructors // effects: Creates a case statememt CqlBlock with SELECT (slots), // FROM (child -- note has only has one child), WHERE (true), AS // (blockAliasNum). caseSlot indicates which slot in slots // corresponds to the single case statement being generated by this block internal CaseCqlBlock(SlotInfo[] slots, int caseSlot, CqlBlock child, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) : base(slots, new List(new CqlBlock[]{child}), whereClause, identifiers, blockAliasNum) { m_caseSlotInfo = slots[caseSlot]; } #endregion #region Fields private SlotInfo m_caseSlotInfo; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part StringUtil.IndentNewLine(builder, indentLevel); // Since we know that the case statement starts on a new line, we // can add a comment after SELECT Debug.Assert(m_caseSlotInfo.MemberPath != null, "We only construct real slots not boolean slots"); builder.Append("SELECT "); if (isTopLevel) { builder.Append("VALUE "); } builder.Append("-- Constructing ") .Append(m_caseSlotInfo.MemberPath.LastComponentName); Debug.Assert(Children.Count == 1, "Case can have exactly one child"); CqlBlock childBlock = Children[0]; // First emit the case statement "CASE ... END AS Alias" m_caseSlotInfo.AsCql(builder, childBlock.CqlAlias, indentLevel); if (false == isTopLevel) { builder.Append(" AS ") .Append(m_caseSlotInfo.CqlFieldAlias); } // Now get the remaining slots bool isFirst = true; // CHANGE_[....]_IMPROVE: Try to leverage GenerateProjectedtList foreach (SlotInfo slot in Slots) { if (false == slot.IsRequiredByParent || object.ReferenceEquals(slot, m_caseSlotInfo)) { continue; } if (isFirst == true) { // Add , after END and then start new line for first field builder.Append(", "); StringUtil.IndentNewLine(builder, indentLevel + 1); } else { // Simply add a comma for the next field builder.Append(", "); } slot.AsCql(builder, childBlock.CqlAlias, indentLevel); isFirst = false; } // The FROM part: FROM (ChildView) AS AliasName StringUtil.IndentNewLine(builder, indentLevel); builder.Append("FROM ("); // Get the child childBlock.AsCql(builder, false, indentLevel + 1); StringUtil.IndentNewLine(builder, indentLevel); builder.Append(") AS ") .Append(childBlock.CqlAlias); // Get the WHERE part only when the expression is not simply TRUE if (false == BoolExpression.EqualityComparer.Equals(WhereClause, BoolExpression.True)) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("WHERE "); WhereClause.AsCql(builder, childBlock.CqlAlias); } return builder; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
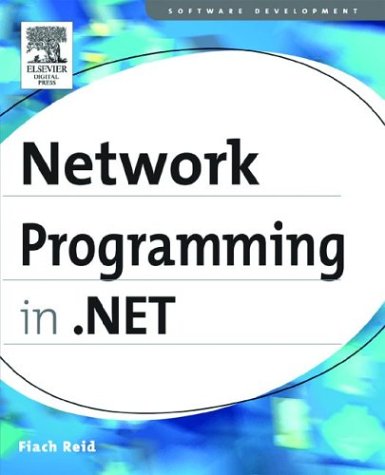
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceFilteringEventArgs.cs
- BitmapEffectRenderDataResource.cs
- ColumnMapCopier.cs
- IDQuery.cs
- QilFactory.cs
- CommentAction.cs
- BehaviorEditorPart.cs
- Bits.cs
- ClientTargetCollection.cs
- WsdlExporter.cs
- QueryReaderSettings.cs
- DBParameter.cs
- PathSegmentCollection.cs
- BindingExpressionBase.cs
- Size.cs
- AuthorizationRule.cs
- TriggerBase.cs
- PropertyGridEditorPart.cs
- InvokeMethodDesigner.xaml.cs
- TabControlCancelEvent.cs
- StubHelpers.cs
- AttributedMetaModel.cs
- ExceptionValidationRule.cs
- _NtlmClient.cs
- PageRequestManager.cs
- OutputCacheModule.cs
- RegexCharClass.cs
- EntityContainer.cs
- NativeMethods.cs
- SqlStream.cs
- RijndaelManagedTransform.cs
- DependencySource.cs
- BuilderPropertyEntry.cs
- ThemeConfigurationDialog.cs
- InvalidCastException.cs
- AutomationPeer.cs
- DataGridViewRowPostPaintEventArgs.cs
- SchemaNamespaceManager.cs
- StorageModelBuildProvider.cs
- ShutDownListener.cs
- HuffmanTree.cs
- MenuItem.cs
- TabletDeviceInfo.cs
- SrgsElementFactory.cs
- ImageMetadata.cs
- WorkflowOwnershipException.cs
- FixedSOMFixedBlock.cs
- Type.cs
- SyndicationItem.cs
- serverconfig.cs
- AddressUtility.cs
- XmlKeywords.cs
- UrlMappingsModule.cs
- ZipIOExtraField.cs
- StyleXamlParser.cs
- TemplateComponentConnector.cs
- ScaleTransform.cs
- DesignerGeometryHelper.cs
- ButtonRenderer.cs
- MetabaseServerConfig.cs
- ImageListImage.cs
- ClockController.cs
- _AutoWebProxyScriptEngine.cs
- Label.cs
- ConstantExpression.cs
- SmtpReplyReader.cs
- tibetanshape.cs
- SectionVisual.cs
- XPathMultyIterator.cs
- TransformerConfigurationWizardBase.cs
- BindingSource.cs
- ParseHttpDate.cs
- safex509handles.cs
- Win32SafeHandles.cs
- GradientBrush.cs
- DescendantQuery.cs
- WindowsStartMenu.cs
- AlternationConverter.cs
- ViewStateException.cs
- SqlParameterCollection.cs
- RootAction.cs
- SQLBinaryStorage.cs
- Cell.cs
- ComboBoxRenderer.cs
- SQLMoney.cs
- MeasureData.cs
- Literal.cs
- Propagator.JoinPropagator.cs
- securitycriticaldata.cs
- DictionaryGlobals.cs
- DragDrop.cs
- FlagPanel.cs
- ParameterToken.cs
- CompilerState.cs
- MembershipValidatePasswordEventArgs.cs
- NameSpaceExtractor.cs
- ListenerPerfCounters.cs
- BinaryReader.cs
- TextElementAutomationPeer.cs
- WindowPatternIdentifiers.cs