Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Size.cs / 1 / Size.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * Size.cs * * Abstract: * * Integer-point coordinate size class * * Revision History: * * 2/4/2k [....] * Created it. * \**************************************************************************/ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a dimension in 2D coordinate space */ ////// /// Represents the size of a rectangular region /// with an ordered pair of width and height. /// [ TypeConverterAttribute(typeof(SizeConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [SuppressMessage("Microsoft.Usage", "CA2225:OperatorOverloadsHaveNamedAlternates")] public struct Size { ////// /// Initializes a new instance of the public static readonly Size Empty = new Size(); private int width; private int height; /** * Create a new Size object from a point */ ///class. /// /// /// public Size(Point pt) { width = pt.X; height = pt.Y; } /** * Create a new Size object of the specified dimension */ ////// Initializes a new instance of the ///class from /// the specified . /// /// /// Initializes a new instance of the public Size(int width, int height) { this.width = width; this.height = height; } ///class from /// the specified dimensions. /// /// /// Converts the specified public static implicit operator SizeF(Size p) { return new SizeF(p.Width, p.Height); } ///to a /// . /// /// /// public static Size operator +(Size sz1, Size sz2) { return Add(sz1, sz2); } ////// Performs vector addition of two ///objects. /// /// /// public static Size operator -(Size sz1, Size sz2) { return Subtract(sz1, sz2); } ////// Contracts a ///by another /// . /// /// /// Tests whether two public static bool operator ==(Size sz1, Size sz2) { return sz1.Width == sz2.Width && sz1.Height == sz2.Height; } ///objects /// are identical. /// /// /// public static bool operator !=(Size sz1, Size sz2) { return !(sz1 == sz2); } ////// Tests whether two ///objects are different. /// /// /// Converts the specified public static explicit operator Point(Size size) { return new Point(size.Width, size.Height); } ///to a /// . /// /// /// Tests whether this [Browsable(false)] public bool IsEmpty { get { return width == 0 && height == 0; } } /** * Horizontal dimension */ ///has zero /// width and height. /// /// /// public int Width { get { return width; } set { width = value; } } /** * Vertical dimension */ ////// Represents the horizontal component of this /// ///. /// /// /// Represents the vertical component of this /// public int Height { get { return height; } set { height = value; } } ///. /// /// public static Size Add(Size sz1, Size sz2) { return new Size(sz1.Width + sz2.Width, sz1.Height + sz2.Height); } ////// Performs vector addition of two ///objects. /// /// /// Converts a SizeF to a Size by performing a ceiling operation on /// all the coordinates. /// public static Size Ceiling(SizeF value) { return new Size((int)Math.Ceiling(value.Width), (int)Math.Ceiling(value.Height)); } ////// public static Size Subtract(Size sz1, Size sz2) { return new Size(sz1.Width - sz2.Width, sz1.Height - sz2.Height); } ////// Contracts a ///by another . /// /// /// Converts a SizeF to a Size by performing a truncate operation on /// all the coordinates. /// public static Size Truncate(SizeF value) { return new Size((int)value.Width, (int)value.Height); } ////// /// Converts a SizeF to a Size by performing a round operation on /// all the coordinates. /// public static Size Round(SizeF value) { return new Size((int)Math.Round(value.Width), (int)Math.Round(value.Height)); } ////// /// public override bool Equals(object obj) { if (!(obj is Size)) return false; Size comp = (Size)obj; // Note value types can't have derived classes, so we don't need to // check the types of the objects here. -- [....], 2/21/2001 return (comp.width == this.width) && (comp.height == this.height); } ////// Tests to see whether the specified object is a /// ////// with the same dimensions as this . /// /// /// public override int GetHashCode() { return width ^ height; } ////// Returns a hash code. /// ////// /// public override string ToString() { return "{Width=" + width.ToString(CultureInfo.CurrentCulture) + ", Height=" + height.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Creates a human-readable string that represents this /// ///. ///
Link Menu
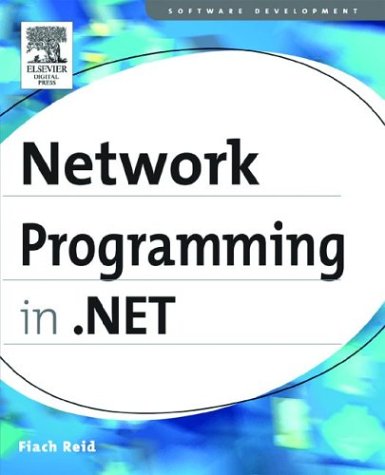
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VirtualStackFrame.cs
- DrawingContextWalker.cs
- xmlsaver.cs
- PlaceHolder.cs
- LicFileLicenseProvider.cs
- IBuiltInEvidence.cs
- OleDbErrorCollection.cs
- StorageMappingFragment.cs
- ButtonChrome.cs
- XmlUtil.cs
- CancelEventArgs.cs
- DataGridViewButtonColumn.cs
- ViewBase.cs
- SingleKeyFrameCollection.cs
- XmlEntity.cs
- UIntPtr.cs
- TrackingValidationObjectDictionary.cs
- TitleStyle.cs
- LogLogRecordEnumerator.cs
- HttpFileCollectionWrapper.cs
- Table.cs
- ConfigXmlCDataSection.cs
- FormViewCommandEventArgs.cs
- EditingCoordinator.cs
- NodeFunctions.cs
- ResourceReader.cs
- SystemWebSectionGroup.cs
- VerticalAlignConverter.cs
- COM2ExtendedBrowsingHandler.cs
- NamedElement.cs
- DecimalConverter.cs
- XslTransform.cs
- GiveFeedbackEvent.cs
- SecurityUniqueId.cs
- ResourceCategoryAttribute.cs
- SqlConnectionPoolProviderInfo.cs
- AttachInfo.cs
- DropShadowBitmapEffect.cs
- NoResizeHandleGlyph.cs
- RoutedEventArgs.cs
- DocumentViewerConstants.cs
- TypeSystem.cs
- XmlWriterSettings.cs
- TableStyle.cs
- FileLogRecord.cs
- UpdateTracker.cs
- ActivitySurrogateSelector.cs
- DataGridViewButtonColumn.cs
- SqlConnectionHelper.cs
- FormViewUpdatedEventArgs.cs
- ToggleButtonAutomationPeer.cs
- HttpContextWrapper.cs
- DefaultWorkflowSchedulerService.cs
- ImpersonationContext.cs
- SearchForVirtualItemEventArgs.cs
- SQLBinary.cs
- SqlPersonalizationProvider.cs
- RegistryConfigurationProvider.cs
- FixedSOMTable.cs
- StrokeIntersection.cs
- HwndSource.cs
- DependencyObject.cs
- OleDbDataReader.cs
- RangeValuePatternIdentifiers.cs
- IntSecurity.cs
- ThreadSafeList.cs
- WebPartEventArgs.cs
- DataBinding.cs
- ScriptRegistrationManager.cs
- ListViewInsertEventArgs.cs
- TypeValidationEventArgs.cs
- Token.cs
- RectangleHotSpot.cs
- RightsManagementErrorHandler.cs
- OracleTransaction.cs
- HttpWebRequestElement.cs
- MenuAdapter.cs
- PersonalizationStateInfoCollection.cs
- DeviceContexts.cs
- ResXFileRef.cs
- EntityDataSourceSelectedEventArgs.cs
- AllMembershipCondition.cs
- _AcceptOverlappedAsyncResult.cs
- SqlHelper.cs
- ToolStripContentPanelRenderEventArgs.cs
- Timer.cs
- AssemblyNameProxy.cs
- ReadOnlyDataSource.cs
- SchemaObjectWriter.cs
- Root.cs
- ImageListUtils.cs
- ToolStripPanelSelectionGlyph.cs
- XmlChildNodes.cs
- ConfigXmlElement.cs
- FilterQueryOptionExpression.cs
- DecodeHelper.cs
- PropertyStore.cs
- ScriptingWebServicesSectionGroup.cs
- ExpressionEditorAttribute.cs
- PerformanceCounterLib.cs