Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / NetworkCredential.cs / 1305376 / NetworkCredential.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Security.Permissions; using System.Text; using System.Threading; using Microsoft.Win32; ////// public class NetworkCredential : ICredentials,ICredentialsByHost { private static EnvironmentPermission m_environmentUserNamePermission; private static EnvironmentPermission m_environmentDomainNamePermission; private static readonly object lockingObject = new object(); private string m_domain; private string m_userName; #if !FEATURE_PAL private SecureString m_password; #else //FEATURE_PAL private string m_password; #endif //FEATURE_PAL public NetworkCredential() { } ///Provides credentials for password-based /// authentication schemes such as basic, digest, NTLM and Kerberos. ////// public NetworkCredential(string userName, string password) : this(userName, password, string.Empty) { } #if !FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, SecureString password) : this(userName, password, string.Empty) { } #endif //!FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, string password, string domain) { UserName = userName; Password = password; Domain = domain; } #if !FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, SecureString password, string domain) { UserName = userName; SecurePassword = password; Domain = domain; } #endif //!FEATURE_PAL void InitializePart1() { if (m_environmentUserNamePermission == null) { lock(lockingObject) { if (m_environmentUserNamePermission == null) { m_environmentDomainNamePermission = new EnvironmentPermission(EnvironmentPermissionAccess.Read, "USERDOMAIN"); m_environmentUserNamePermission = new EnvironmentPermission(EnvironmentPermissionAccess.Read, "USERNAME"); } } } } ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public string UserName { get { InitializePart1(); m_environmentUserNamePermission.Demand(); return InternalGetUserName(); } set { if (value == null) m_userName = String.Empty; else m_userName = value; // GlobalLog.Print("NetworkCredential::set_UserName: m_userName: \"" + m_userName + "\"" ); } } ////// The user name associated with this credential. /// ////// public string Password { get { ExceptionHelper.UnmanagedPermission.Demand(); return InternalGetPassword(); } set { #if FEATURE_PAL if (value == null) m_password = String.Empty; else m_password = value; // GlobalLog.Print("NetworkCredential::set_Password: m_password: \"" + m_password + "\"" ); #else //!FEATURE_PAL m_password = UnsafeNclNativeMethods.SecureStringHelper.CreateSecureString(value); // GlobalLog.Print("NetworkCredential::set_Password: value = " + value); // GlobalLog.Print("NetworkCredential::set_Password: m_password:"); // GlobalLog.Dump(m_password); #endif //!FEATURE_PAL } } #if !FEATURE_PAL ////// The password for the user name. /// ////// public SecureString SecurePassword { get { ExceptionHelper.UnmanagedPermission.Demand(); return InternalGetSecurePassword().Copy(); } set { if (value == null) m_password = new SecureString(); // makes 0 length string else m_password = value.Copy(); } } #endif //!FEATURE_PAL ////// The password for the user name. /// ////// public string Domain { get { InitializePart1(); m_environmentDomainNamePermission.Demand(); return InternalGetDomain(); } set { if (value == null) m_domain = String.Empty; else m_domain = value; // GlobalLog.Print("NetworkCredential::set_Domain: m_domain: \"" + m_domain + "\"" ); } } internal string InternalGetUserName() { // GlobalLog.Print("NetworkCredential::get_UserName: returning \"" + m_userName + "\""); return m_userName; } internal string InternalGetPassword() { #if FEATURE_PAL // GlobalLog.Print("NetworkCredential::get_Password: returning \"" + m_password + "\""); return m_password; #else //!FEATURE_PAL string decryptedString = UnsafeNclNativeMethods.SecureStringHelper.CreateString(m_password); // GlobalLog.Print("NetworkCredential::get_Password: returning \"" + decryptedString + "\""); return decryptedString; #endif //!FEATURE_PAL } #if !FEATURE_PAL internal SecureString InternalGetSecurePassword() { return m_password; } #endif //!FEATURE_PAL internal string InternalGetDomain() { // GlobalLog.Print("NetworkCredential::get_Domain: returning \"" + m_domain + "\""); return m_domain; } internal string InternalGetDomainUserName() { string domainUserName = InternalGetDomain(); if (domainUserName.Length != 0) domainUserName += "\\"; domainUserName += InternalGetUserName(); return domainUserName; } ////// The machine name that verifies /// the credentials. Usually this is the host machine. /// ////// public NetworkCredential GetCredential(Uri uri, String authType) { return this; } public NetworkCredential GetCredential(string host, int port, String authenticationType) { return this; } #if DEBUG // this method is only called as part of an assert internal bool IsEqualTo(object compObject) { if ((object)compObject == null) return false; if ((object)this == (object)compObject) return true; NetworkCredential compCred = compObject as NetworkCredential; if ((object)compCred == null) return false; #if FEATURE_PAL return(InternalGetUserName() == compCred.InternalGetUserName() && InternalGetPassword() == compCred.InternalGetPassword() && InternalGetDomain() == compCred.InternalGetDomain()); #else //!FEATURE_PAL return (InternalGetUserName() == compCred.InternalGetUserName() && InternalGetDomain() == compCred.InternalGetDomain() && UnsafeNclNativeMethods.SecureStringHelper.AreEqualValues(InternalGetSecurePassword(), compCred.InternalGetSecurePassword())); #endif //!FEATURE_PAL } #endif //DEBUG } // class NetworkCredential } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns an instance of the NetworkCredential class for a Uri and /// authentication type. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Security.Permissions; using System.Text; using System.Threading; using Microsoft.Win32; ////// public class NetworkCredential : ICredentials,ICredentialsByHost { private static EnvironmentPermission m_environmentUserNamePermission; private static EnvironmentPermission m_environmentDomainNamePermission; private static readonly object lockingObject = new object(); private string m_domain; private string m_userName; #if !FEATURE_PAL private SecureString m_password; #else //FEATURE_PAL private string m_password; #endif //FEATURE_PAL public NetworkCredential() { } ///Provides credentials for password-based /// authentication schemes such as basic, digest, NTLM and Kerberos. ////// public NetworkCredential(string userName, string password) : this(userName, password, string.Empty) { } #if !FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, SecureString password) : this(userName, password, string.Empty) { } #endif //!FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, string password, string domain) { UserName = userName; Password = password; Domain = domain; } #if !FEATURE_PAL ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public NetworkCredential(string userName, SecureString password, string domain) { UserName = userName; SecurePassword = password; Domain = domain; } #endif //!FEATURE_PAL void InitializePart1() { if (m_environmentUserNamePermission == null) { lock(lockingObject) { if (m_environmentUserNamePermission == null) { m_environmentDomainNamePermission = new EnvironmentPermission(EnvironmentPermissionAccess.Read, "USERDOMAIN"); m_environmentUserNamePermission = new EnvironmentPermission(EnvironmentPermissionAccess.Read, "USERNAME"); } } } } ////// Initializes a new instance of the ////// class with name and password set as specified. /// /// public string UserName { get { InitializePart1(); m_environmentUserNamePermission.Demand(); return InternalGetUserName(); } set { if (value == null) m_userName = String.Empty; else m_userName = value; // GlobalLog.Print("NetworkCredential::set_UserName: m_userName: \"" + m_userName + "\"" ); } } ////// The user name associated with this credential. /// ////// public string Password { get { ExceptionHelper.UnmanagedPermission.Demand(); return InternalGetPassword(); } set { #if FEATURE_PAL if (value == null) m_password = String.Empty; else m_password = value; // GlobalLog.Print("NetworkCredential::set_Password: m_password: \"" + m_password + "\"" ); #else //!FEATURE_PAL m_password = UnsafeNclNativeMethods.SecureStringHelper.CreateSecureString(value); // GlobalLog.Print("NetworkCredential::set_Password: value = " + value); // GlobalLog.Print("NetworkCredential::set_Password: m_password:"); // GlobalLog.Dump(m_password); #endif //!FEATURE_PAL } } #if !FEATURE_PAL ////// The password for the user name. /// ////// public SecureString SecurePassword { get { ExceptionHelper.UnmanagedPermission.Demand(); return InternalGetSecurePassword().Copy(); } set { if (value == null) m_password = new SecureString(); // makes 0 length string else m_password = value.Copy(); } } #endif //!FEATURE_PAL ////// The password for the user name. /// ////// public string Domain { get { InitializePart1(); m_environmentDomainNamePermission.Demand(); return InternalGetDomain(); } set { if (value == null) m_domain = String.Empty; else m_domain = value; // GlobalLog.Print("NetworkCredential::set_Domain: m_domain: \"" + m_domain + "\"" ); } } internal string InternalGetUserName() { // GlobalLog.Print("NetworkCredential::get_UserName: returning \"" + m_userName + "\""); return m_userName; } internal string InternalGetPassword() { #if FEATURE_PAL // GlobalLog.Print("NetworkCredential::get_Password: returning \"" + m_password + "\""); return m_password; #else //!FEATURE_PAL string decryptedString = UnsafeNclNativeMethods.SecureStringHelper.CreateString(m_password); // GlobalLog.Print("NetworkCredential::get_Password: returning \"" + decryptedString + "\""); return decryptedString; #endif //!FEATURE_PAL } #if !FEATURE_PAL internal SecureString InternalGetSecurePassword() { return m_password; } #endif //!FEATURE_PAL internal string InternalGetDomain() { // GlobalLog.Print("NetworkCredential::get_Domain: returning \"" + m_domain + "\""); return m_domain; } internal string InternalGetDomainUserName() { string domainUserName = InternalGetDomain(); if (domainUserName.Length != 0) domainUserName += "\\"; domainUserName += InternalGetUserName(); return domainUserName; } ////// The machine name that verifies /// the credentials. Usually this is the host machine. /// ////// public NetworkCredential GetCredential(Uri uri, String authType) { return this; } public NetworkCredential GetCredential(string host, int port, String authenticationType) { return this; } #if DEBUG // this method is only called as part of an assert internal bool IsEqualTo(object compObject) { if ((object)compObject == null) return false; if ((object)this == (object)compObject) return true; NetworkCredential compCred = compObject as NetworkCredential; if ((object)compCred == null) return false; #if FEATURE_PAL return(InternalGetUserName() == compCred.InternalGetUserName() && InternalGetPassword() == compCred.InternalGetPassword() && InternalGetDomain() == compCred.InternalGetDomain()); #else //!FEATURE_PAL return (InternalGetUserName() == compCred.InternalGetUserName() && InternalGetDomain() == compCred.InternalGetDomain() && UnsafeNclNativeMethods.SecureStringHelper.AreEqualValues(InternalGetSecurePassword(), compCred.InternalGetSecurePassword())); #endif //!FEATURE_PAL } #endif //DEBUG } // class NetworkCredential } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns an instance of the NetworkCredential class for a Uri and /// authentication type. /// ///
Link Menu
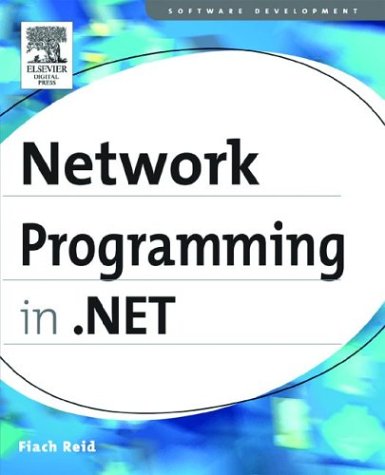
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RbTree.cs
- TaskResultSetter.cs
- TextServicesManager.cs
- TerminatingOperationBehavior.cs
- HtmlInputControl.cs
- GcSettings.cs
- RelationshipDetailsCollection.cs
- DataGridCellClipboardEventArgs.cs
- BitmapEffectOutputConnector.cs
- ElementUtil.cs
- Sql8ExpressionRewriter.cs
- _HelperAsyncResults.cs
- MobileCategoryAttribute.cs
- tabpagecollectioneditor.cs
- Lease.cs
- ButtonBaseAutomationPeer.cs
- SmiEventSink_Default.cs
- HandoffBehavior.cs
- Floater.cs
- IPEndPoint.cs
- XmlNodeChangedEventManager.cs
- SafeRightsManagementSessionHandle.cs
- ImplicitInputBrush.cs
- TypeLibConverter.cs
- PriorityItem.cs
- WpfXamlMember.cs
- RegexGroupCollection.cs
- GestureRecognizer.cs
- Style.cs
- Command.cs
- VisualTarget.cs
- SQLSingleStorage.cs
- XmlWriterSettings.cs
- SqlDataSourceCommandEventArgs.cs
- PropertyPath.cs
- Polyline.cs
- ParentUndoUnit.cs
- LongMinMaxAggregationOperator.cs
- NoneExcludedImageIndexConverter.cs
- Parameter.cs
- DataGridViewCellParsingEventArgs.cs
- ExpressionsCollectionEditor.cs
- WCFServiceClientProxyGenerator.cs
- RegexReplacement.cs
- _SslState.cs
- SByte.cs
- CrossContextChannel.cs
- Win32NamedPipes.cs
- AssemblyName.cs
- SqlConnectionString.cs
- ReferenceConverter.cs
- SuppressMergeCheckAttribute.cs
- ImageListStreamer.cs
- ISO2022Encoding.cs
- ThousandthOfEmRealPoints.cs
- XmlCharacterData.cs
- RegisteredHiddenField.cs
- XDRSchema.cs
- DbModificationClause.cs
- HandlerBase.cs
- ListSortDescriptionCollection.cs
- XPathPatternParser.cs
- OleAutBinder.cs
- BaseDataListComponentEditor.cs
- ProgramNode.cs
- ByteFacetDescriptionElement.cs
- DrawingGroup.cs
- NotifyInputEventArgs.cs
- PartialCachingAttribute.cs
- ScriptingScriptResourceHandlerSection.cs
- BorderGapMaskConverter.cs
- XmlQueryCardinality.cs
- SqlConnectionStringBuilder.cs
- ValidationPropertyAttribute.cs
- CustomErrorsSectionWrapper.cs
- DisplayNameAttribute.cs
- SignedInfo.cs
- WebPartConnectionCollection.cs
- QuestionEventArgs.cs
- DBDataPermissionAttribute.cs
- PseudoWebRequest.cs
- DeobfuscatingStream.cs
- ConsoleCancelEventArgs.cs
- PerformanceCounterLib.cs
- LocatorPartList.cs
- XmlSchemaSimpleContentRestriction.cs
- StatusBar.cs
- ColumnResizeUndoUnit.cs
- ChtmlPhoneCallAdapter.cs
- PagerSettings.cs
- Rect3DValueSerializer.cs
- Matrix.cs
- ObfuscationAttribute.cs
- WebEvents.cs
- InputLanguageProfileNotifySink.cs
- HealthMonitoringSectionHelper.cs
- PrintPreviewControl.cs
- QilXmlReader.cs
- LineProperties.cs
- WmlValidationSummaryAdapter.cs