Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartVerbCollection.cs / 1305376 / WebPartVerbCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; public sealed class WebPartVerbCollection : ReadOnlyCollectionBase { private HybridDictionary _ids; public static readonly WebPartVerbCollection Empty = new WebPartVerbCollection(); public WebPartVerbCollection() { Initialize(null, null); } public WebPartVerbCollection(ICollection verbs) { Initialize(null, verbs); } public WebPartVerbCollection(WebPartVerbCollection existingVerbs, ICollection verbs) { Initialize(existingVerbs, verbs); } public WebPartVerb this[int index] { get { return (WebPartVerb) InnerList[index]; } } // Currently, the WebPartVerbCollection class allows verbs to be added with duplicate IDs. // Ideally, we would not allow this. However, since the WebPartChrome.GetWebParts() method // returns both the Part and Zone verbs, there is the potential to have multiple verbs // with the same ID. So this property must be internal, since we are guaranteed to never // call it on a collection with duplicate IDs. internal WebPartVerb this[string id] { get { return (WebPartVerb)_ids[id]; } } // Do not throw for duplicate IDs, since we call this method to add items // to the collection internally, and we need to allow duplicate IDs. internal int Add(WebPartVerb value) { return InnerList.Add(value); } public bool Contains(WebPartVerb value) { return InnerList.Contains(value); } public void CopyTo(WebPartVerb[] array, int index) { InnerList.CopyTo(array, index); } public int IndexOf(WebPartVerb value) { return InnerList.IndexOf(value); } private void Initialize(WebPartVerbCollection existingVerbs, ICollection verbs) { int count = ((existingVerbs != null) ? existingVerbs.Count : 0) + ((verbs != null) ? verbs.Count : 0); _ids = new HybridDictionary(count, /* caseInsensitive */ true); if (existingVerbs != null) { foreach (WebPartVerb existingVerb in existingVerbs) { // Don't need to check arg, since we know it is valid since it came // from a CatalogPartCollection. if (_ids.Contains(existingVerb.ID)) { throw new ArgumentException(SR.GetString( SR.WebPart_Collection_DuplicateID, "WebPartVerb", existingVerb.ID), "existingVerbs"); } _ids.Add(existingVerb.ID, existingVerb); InnerList.Add(existingVerb); } } if (verbs != null) { foreach (object obj in verbs) { if (obj == null) { throw new ArgumentException(SR.GetString(SR.Collection_CantAddNull), "verbs"); } WebPartVerb webPartVerb = obj as WebPartVerb; if (webPartVerb == null) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartVerb"), "verbs"); } if (_ids.Contains(webPartVerb.ID)) { throw new ArgumentException(SR.GetString( SR.WebPart_Collection_DuplicateID, "WebPartVerb", webPartVerb.ID), "verbs"); } _ids.Add(webPartVerb.ID, webPartVerb); InnerList.Add(webPartVerb); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; public sealed class WebPartVerbCollection : ReadOnlyCollectionBase { private HybridDictionary _ids; public static readonly WebPartVerbCollection Empty = new WebPartVerbCollection(); public WebPartVerbCollection() { Initialize(null, null); } public WebPartVerbCollection(ICollection verbs) { Initialize(null, verbs); } public WebPartVerbCollection(WebPartVerbCollection existingVerbs, ICollection verbs) { Initialize(existingVerbs, verbs); } public WebPartVerb this[int index] { get { return (WebPartVerb) InnerList[index]; } } // Currently, the WebPartVerbCollection class allows verbs to be added with duplicate IDs. // Ideally, we would not allow this. However, since the WebPartChrome.GetWebParts() method // returns both the Part and Zone verbs, there is the potential to have multiple verbs // with the same ID. So this property must be internal, since we are guaranteed to never // call it on a collection with duplicate IDs. internal WebPartVerb this[string id] { get { return (WebPartVerb)_ids[id]; } } // Do not throw for duplicate IDs, since we call this method to add items // to the collection internally, and we need to allow duplicate IDs. internal int Add(WebPartVerb value) { return InnerList.Add(value); } public bool Contains(WebPartVerb value) { return InnerList.Contains(value); } public void CopyTo(WebPartVerb[] array, int index) { InnerList.CopyTo(array, index); } public int IndexOf(WebPartVerb value) { return InnerList.IndexOf(value); } private void Initialize(WebPartVerbCollection existingVerbs, ICollection verbs) { int count = ((existingVerbs != null) ? existingVerbs.Count : 0) + ((verbs != null) ? verbs.Count : 0); _ids = new HybridDictionary(count, /* caseInsensitive */ true); if (existingVerbs != null) { foreach (WebPartVerb existingVerb in existingVerbs) { // Don't need to check arg, since we know it is valid since it came // from a CatalogPartCollection. if (_ids.Contains(existingVerb.ID)) { throw new ArgumentException(SR.GetString( SR.WebPart_Collection_DuplicateID, "WebPartVerb", existingVerb.ID), "existingVerbs"); } _ids.Add(existingVerb.ID, existingVerb); InnerList.Add(existingVerb); } } if (verbs != null) { foreach (object obj in verbs) { if (obj == null) { throw new ArgumentException(SR.GetString(SR.Collection_CantAddNull), "verbs"); } WebPartVerb webPartVerb = obj as WebPartVerb; if (webPartVerb == null) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartVerb"), "verbs"); } if (_ids.Contains(webPartVerb.ID)) { throw new ArgumentException(SR.GetString( SR.WebPart_Collection_DuplicateID, "WebPartVerb", webPartVerb.ID), "verbs"); } _ids.Add(webPartVerb.ID, webPartVerb); InnerList.Add(webPartVerb); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
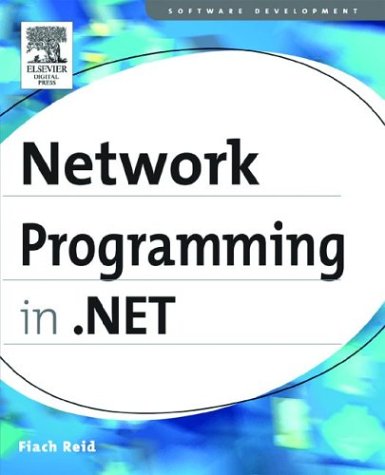
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DisableDpiAwarenessAttribute.cs
- AssemblyBuilder.cs
- StretchValidation.cs
- File.cs
- BatchStream.cs
- Rect.cs
- DataTable.cs
- RegexFCD.cs
- odbcmetadatacollectionnames.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- LinkButton.cs
- XmlText.cs
- WizardForm.cs
- ProcessThreadCollection.cs
- XmlLinkedNode.cs
- XsdDateTime.cs
- FtpWebRequest.cs
- TranslateTransform.cs
- IndentedTextWriter.cs
- IsolatedStorageFileStream.cs
- EmissiveMaterial.cs
- ExponentialEase.cs
- ParsedRoute.cs
- XamlReaderHelper.cs
- DataServiceResponse.cs
- XmlSchemaAnyAttribute.cs
- TraceLog.cs
- FormViewDeleteEventArgs.cs
- SByteStorage.cs
- CodeSubDirectoriesCollection.cs
- XmlSchemaExporter.cs
- FloaterBaseParaClient.cs
- KerberosSecurityTokenAuthenticator.cs
- CodeDirectoryCompiler.cs
- ComponentResourceKeyConverter.cs
- ToolboxItemAttribute.cs
- TextEffect.cs
- SingleAnimationBase.cs
- SurrogateDataContract.cs
- smtpconnection.cs
- ElementAtQueryOperator.cs
- SharedDp.cs
- SizeConverter.cs
- WebBrowsableAttribute.cs
- ExpressionNormalizer.cs
- ScriptControlManager.cs
- TemplateNodeContextMenu.cs
- WebPartDisplayModeCancelEventArgs.cs
- ProfileSettings.cs
- SequenceNumber.cs
- ContainerTracking.cs
- Brush.cs
- GeneralTransform.cs
- WsatProxy.cs
- CodeTypeReferenceCollection.cs
- QuotedPairReader.cs
- DbProviderSpecificTypePropertyAttribute.cs
- InlineObject.cs
- ChoiceConverter.cs
- BitmapInitialize.cs
- XmlSchemaObjectTable.cs
- JoinTreeSlot.cs
- WSFederationHttpSecurity.cs
- VerticalAlignConverter.cs
- BoundPropertyEntry.cs
- OutOfMemoryException.cs
- ReflectionTypeLoadException.cs
- WebPageTraceListener.cs
- JsonDataContract.cs
- EntityFunctions.cs
- OdbcReferenceCollection.cs
- CriticalFinalizerObject.cs
- InputBindingCollection.cs
- DataBoundLiteralControl.cs
- SoapDocumentMethodAttribute.cs
- WebPartZoneBase.cs
- SystemDiagnosticsSection.cs
- updateconfighost.cs
- contentDescriptor.cs
- TypeReference.cs
- EndpointAddressElementBase.cs
- TabItemWrapperAutomationPeer.cs
- PageCatalogPart.cs
- ConfigurationLockCollection.cs
- FilterElement.cs
- StickyNote.cs
- CachedCompositeFamily.cs
- XmlObjectSerializerReadContext.cs
- handlecollector.cs
- ConfigXmlCDataSection.cs
- HwndSourceParameters.cs
- PersonalizableAttribute.cs
- ManualResetEventSlim.cs
- NumberSubstitution.cs
- Compilation.cs
- Documentation.cs
- TextPenaltyModule.cs
- WebPartZone.cs
- RequiredAttributeAttribute.cs
- _IPv4Address.cs