Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Interop / BrowserInteropHelper.cs / 1 / BrowserInteropHelper.cs
//---------------------------------------------------------------------------- // // File: BrowserInteropHelper.cs // // Description: Implements Avalon BrowserInteropHelper class, which helps // interop with the browser // // Created: 08/02/05 // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using MS.Internal; using MS.Internal.PresentationFramework; // SecurityHelper using MS.Win32; using System.Windows.Input; using MS.Internal.AppModel; namespace System.Windows.Interop { ////// Implements Avalon BrowserInteropHelper, which helps interop with the browser /// public static class BrowserInteropHelper { ////// Critical because it sets critical data. /// Safe because it is the static ctor, and the data doesn't go anywhere. /// [SecurityCritical, SecurityTreatAsSafe] static BrowserInteropHelper() { SetBrowserHosted(false); IsViewer = false; IsInitialViewerNavigation = true; } ////// Returns the IOleClientSite interface /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical: Exposes a COM interface pointer to the IOleClientSite where the app is hosted /// PublicOK: It is public, but there is a demand /// public static object ClientSite { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); object oleClientSite = null; if (IsBrowserHosted) { Application.Current.BrowserCallbackServices.GetOleClientSite(out oleClientSite); } return oleClientSite; } } ////// Returns true if the app is a browser hosted app. /// public static bool IsBrowserHosted { get { return _isBrowserHosted.Value; } } ////// This is critical because setting the BrowserHosted status is a critical resource. /// [SecurityCritical] internal static void SetBrowserHosted(bool value) { _isBrowserHosted.Value = value; } ////// Returns the Uri used to launch the application. /// public static Uri Source { get { return _source.Value; } } ////// Sets the Source URI /// ////// Critical: critical because setting the source is critical. /// [SecurityCritical] internal static void SetSource(Uri source) { _source.Value = source; } ////// Returns true if the app is a viewer app. /// ////// Critical: critical because setting the viewer status is a critical resource. /// internal static bool IsViewer { get { return _isViewer.Value; } [SecurityCritical] set { _isViewer.Value = value; } } ////// Returns true if avalon it top level. /// Also returns true if not browser-hosted. /// ////// Critical: Calls the SUCd IBrowserCallbackServices.IsAvalonTopLevel(). /// Safe: This is okay to dislose. /// internal static bool IsAvalonTopLevel { [SecurityCritical, SecurityTreatAsSafe] get { if (!IsBrowserHosted) return true; IBrowserCallbackServices bcs = Application.Current.BrowserCallbackServices; return bcs != null && bcs.IsAvalonTopLevel(); } } ////// Returns true if we are in viewer mode AND this is the first time that a viewer has been navigated. /// Including IsViewer is defense-in-depth in case somebody forgets to check IsViewer. There are other /// reasons why both IsViewer and IsViewerNavigation are necessary, however. /// ////// Critical: setting this information is a critical resource. /// internal static bool IsInitialViewerNavigation { get { return IsViewer && _isInitialViewerNavigation.Value; } [SecurityCritical] set { _isInitialViewerNavigation.Value = value; } } ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] private static void HostFilterInput(ref MSG msg, ref bool handled) { // The host gets to see input, keyboard and mouse messages. if (msg.message == MS.Win32.NativeMethods.WM_INPUT || (msg.message >= MS.Win32.NativeMethods.WM_KEYFIRST && msg.message <= MS.Win32.NativeMethods.WM_IME_KEYLAST) || (msg.message >= MS.Win32.NativeMethods.WM_MOUSEFIRST && msg.message <= MS.Win32.NativeMethods.WM_MOUSELAST)) { if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref msg, false)) { handled = true; } } } ///This hook gets a "last chance" to handle a key. Such applicaton-unhandled /// keys are forwarded to the browser frame. /// ////// Critical: this calls ForwardTranslateAccelerator, which is SUC'ed. /// [SecurityCritical] internal static IntPtr PostFilterInput(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { if (!handled) { if (msg >= MS.Win32.NativeMethods.WM_KEYFIRST && msg <= MS.Win32.NativeMethods.WM_IME_KEYLAST) { MSG m = new MSG(hwnd, msg, wParam, lParam, SafeNativeMethods.GetMessageTime(), 0, 0); if (MS.Win32.NativeMethods.S_OK == ForwardTranslateAccelerator(ref m, true)) { handled = true; } } } return IntPtr.Zero; } ////// Critical: this attaches an event to ThreadFilterMessage, which requires an assert /// Safe: doesn't expose anything, just does some internal plumbing stuff /// [SecurityCritical, SecurityTreatAsSafe] internal static void InitializeHostFilterInput() { (new UIPermission(PermissionState.Unrestricted)).Assert(); // Blessed assert try { ComponentDispatcher.ThreadFilterMessage += new ThreadMessageEventHandler(HostFilterInput); } finally { UIPermission.RevertAssert(); } } private static SecurityCriticalDataForSet_isBrowserHosted; private static SecurityCriticalDataForSet _isViewer; private static SecurityCriticalDataForSet _isInitialViewerNavigation; private static SecurityCriticalDataForSet _source; /// /// Critical - call is SUC'ed /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("PresentationHostDLL.dll", EntryPoint = "ForwardTranslateAccelerator")] private static extern int ForwardTranslateAccelerator(ref MSG pMsg, bool appUnhandled); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
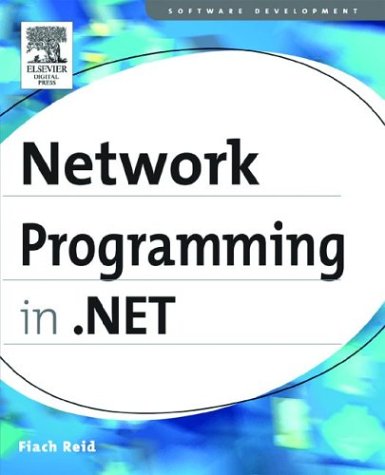
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Utility.cs
- SqlBuilder.cs
- XmlSchemaElement.cs
- DelegatingMessage.cs
- UntypedNullExpression.cs
- SqlConnectionPoolProviderInfo.cs
- MenuItemBindingCollection.cs
- TransactionalPackage.cs
- CodeDOMProvider.cs
- TailCallAnalyzer.cs
- TraceContext.cs
- RuntimeConfigurationRecord.cs
- ConfigurationProperty.cs
- EmptyImpersonationContext.cs
- AssemblyBuilder.cs
- EventLogTraceListener.cs
- StrongTypingException.cs
- MulticastDelegate.cs
- FloaterBaseParagraph.cs
- MemberBinding.cs
- HtmlInputImage.cs
- ToolStripSettings.cs
- ChineseLunisolarCalendar.cs
- TextPatternIdentifiers.cs
- AncestorChangedEventArgs.cs
- CustomValidator.cs
- NativeMethods.cs
- PageBreakRecord.cs
- CacheSection.cs
- TextSelection.cs
- XamlBrushSerializer.cs
- MobileTextWriter.cs
- FileDialog.cs
- HtmlTableCell.cs
- HTMLTextWriter.cs
- WsrmTraceRecord.cs
- WebPermission.cs
- PasswordBox.cs
- RegexNode.cs
- MetaModel.cs
- IPCCacheManager.cs
- PropertiesTab.cs
- ScriptingSectionGroup.cs
- UnSafeCharBuffer.cs
- AppDomainProtocolHandler.cs
- MDIControlStrip.cs
- DataGridViewImageColumn.cs
- IndicShape.cs
- CodeBlockBuilder.cs
- NavigationPropertyEmitter.cs
- BCryptNative.cs
- AspCompat.cs
- StringDictionaryCodeDomSerializer.cs
- BindingListCollectionView.cs
- TextHidden.cs
- indexingfiltermarshaler.cs
- StringDictionaryWithComparer.cs
- EventSetter.cs
- IERequestCache.cs
- InputBindingCollection.cs
- InkCanvasInnerCanvas.cs
- ThousandthOfEmRealPoints.cs
- IRCollection.cs
- RandomNumberGenerator.cs
- TransactionScopeDesigner.cs
- RouteValueDictionary.cs
- XmlDataCollection.cs
- StringToken.cs
- SapiInterop.cs
- FormViewCommandEventArgs.cs
- UriTemplateTrieNode.cs
- _IPv4Address.cs
- EntitySet.cs
- CheckPair.cs
- SetIterators.cs
- ClientSideProviderDescription.cs
- AuthorizationSection.cs
- ControlCachePolicy.cs
- UIElementParagraph.cs
- Emitter.cs
- Knowncolors.cs
- ConfigXmlWhitespace.cs
- ClockController.cs
- ProjectionPlanCompiler.cs
- TypedTableGenerator.cs
- NavigatingCancelEventArgs.cs
- FormsAuthenticationUserCollection.cs
- ParsedAttributeCollection.cs
- LogSwitch.cs
- GuidelineSet.cs
- CodeRemoveEventStatement.cs
- NetworkInformationPermission.cs
- ObjectDisposedException.cs
- ResXResourceWriter.cs
- HostingEnvironment.cs
- ProviderConnectionPoint.cs
- LineProperties.cs
- DataGridViewAutoSizeModeEventArgs.cs
- GetPageNumberCompletedEventArgs.cs
- X509ChainElement.cs