Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / _IPv4Address.cs / 1 / _IPv4Address.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System { // The class designed as to keep minimal the working set of Uri class. // The idea is to stay with static helper methods and strings internal class IPv4AddressHelper { // fields private IPv4AddressHelper() { } private const int NumberOfLabels = 4; // methods internal static string ParseCanonicalName(string str, int start, int end, ref bool isLoopback) { unsafe { byte* numbers = stackalloc byte[NumberOfLabels]; isLoopback = Parse(str, numbers, start, end); return numbers[0] + "." + numbers[1] + "." + numbers[2] + "." + numbers[3]; } } internal static int ParseHostNumber(string str, int start, int end) { unsafe { byte* numbers = stackalloc byte[NumberOfLabels]; Parse(str, numbers, start, end); return (numbers[0] << 24) + (numbers[1] << 16) + (numbers[2] << 8) + numbers[3]; } } // // IsValid // // Performs IsValid on a substring. Updates the index to where we // believe the IPv4 address ends // // Inputs: //name // string containing possible IPv4 address // // start // offset in to start checking for IPv4 address // // end // offset in of the last character we can touch in the check // // Outputs: // end // index of last character in we checked // // Assumes: // The address string is terminated by either // end of the string, characters ':' '/' '\' '?' // // // Returns: // bool // // Throws: // Nothing // //internal unsafe static bool IsValid(char* name, int start, ref int end) { // return IsValid(name, start, ref end, false); //} //Remark: MUST NOT be used unless all input indexes are are verified and trusted. internal unsafe static bool IsValid(char* name, int start, ref int end, bool allowIPv6, bool notImplicitFile) { int dots = 0; int number = 0; bool haveNumber = false; while (start < end) { char ch = name[start]; if (allowIPv6) { // for ipv4 inside ipv6 the terminator is either ScopeId, prefix or ipv6 terminator if(ch == ']' || ch == '/' || ch == '%') break; } else if (ch == '/' || ch == '\\' || (notImplicitFile && (ch == ':' || ch == '?' || ch == '#'))) { break; } if (ch <= '9' && ch >= '0') { haveNumber = true; number = number * 10 + (name[start] - '0'); if (number > 255) { return false; } } else if (ch == '.') { if (!haveNumber) { return false; } ++dots; haveNumber = false; number = 0; } else { return false; } ++start; } bool res = (dots == 3) && haveNumber; if (res) { end = start; } return res; } // // Parse // // Convert this IPv4 address into a sequence of 4 8-bit numbers // // Assumes: // has been validated and contains only decimal digits in groups // of 8-bit numbers and the characters '.' // Address may terminate with ':' or with the end of the string // unsafe private static bool Parse(string name, byte* numbers, int start, int end) { for (int i = 0; i < NumberOfLabels; ++i) { byte b = 0; char ch; for (; (start < end) && (ch = name[start]) != '.' && ch != ':'; ++start) { b = (byte)(b * 10 + (byte)(ch - '0')); } numbers[i] = b; ++start; } return numbers[0] == 127; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System { // The class designed as to keep minimal the working set of Uri class. // The idea is to stay with static helper methods and strings internal class IPv4AddressHelper { // fields private IPv4AddressHelper() { } private const int NumberOfLabels = 4; // methods internal static string ParseCanonicalName(string str, int start, int end, ref bool isLoopback) { unsafe { byte* numbers = stackalloc byte[NumberOfLabels]; isLoopback = Parse(str, numbers, start, end); return numbers[0] + "." + numbers[1] + "." + numbers[2] + "." + numbers[3]; } } internal static int ParseHostNumber(string str, int start, int end) { unsafe { byte* numbers = stackalloc byte[NumberOfLabels]; Parse(str, numbers, start, end); return (numbers[0] << 24) + (numbers[1] << 16) + (numbers[2] << 8) + numbers[3]; } } // // IsValid // // Performs IsValid on a substring. Updates the index to where we // believe the IPv4 address ends // // Inputs: //name // string containing possible IPv4 address // // start // offset in to start checking for IPv4 address // // end // offset in of the last character we can touch in the check // // Outputs: // end // index of last character in we checked // // Assumes: // The address string is terminated by either // end of the string, characters ':' '/' '\' '?' // // // Returns: // bool // // Throws: // Nothing // //internal unsafe static bool IsValid(char* name, int start, ref int end) { // return IsValid(name, start, ref end, false); //} //Remark: MUST NOT be used unless all input indexes are are verified and trusted. internal unsafe static bool IsValid(char* name, int start, ref int end, bool allowIPv6, bool notImplicitFile) { int dots = 0; int number = 0; bool haveNumber = false; while (start < end) { char ch = name[start]; if (allowIPv6) { // for ipv4 inside ipv6 the terminator is either ScopeId, prefix or ipv6 terminator if(ch == ']' || ch == '/' || ch == '%') break; } else if (ch == '/' || ch == '\\' || (notImplicitFile && (ch == ':' || ch == '?' || ch == '#'))) { break; } if (ch <= '9' && ch >= '0') { haveNumber = true; number = number * 10 + (name[start] - '0'); if (number > 255) { return false; } } else if (ch == '.') { if (!haveNumber) { return false; } ++dots; haveNumber = false; number = 0; } else { return false; } ++start; } bool res = (dots == 3) && haveNumber; if (res) { end = start; } return res; } // // Parse // // Convert this IPv4 address into a sequence of 4 8-bit numbers // // Assumes: // has been validated and contains only decimal digits in groups // of 8-bit numbers and the characters '.' // Address may terminate with ':' or with the end of the string // unsafe private static bool Parse(string name, byte* numbers, int start, int end) { for (int i = 0; i < NumberOfLabels; ++i) { byte b = 0; char ch; for (; (start < end) && (ch = name[start]) != '.' && ch != ':'; ++start) { b = (byte)(b * 10 + (byte)(ch - '0')); } numbers[i] = b; ++start; } return numbers[0] == 127; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
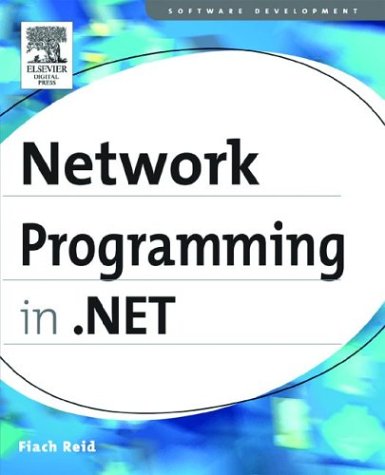
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPHostEntry.cs
- TreeViewImageKeyConverter.cs
- CatalogPartCollection.cs
- TreeView.cs
- ThreadInterruptedException.cs
- COM2ComponentEditor.cs
- PointLightBase.cs
- DataDesignUtil.cs
- SpanIndex.cs
- EditCommandColumn.cs
- ObservableDictionary.cs
- XPathDocumentBuilder.cs
- IdentityValidationException.cs
- CompiledAction.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- RegexCode.cs
- TypeReference.cs
- ObjectStateManagerMetadata.cs
- ContainerSelectorGlyph.cs
- SharedPerformanceCounter.cs
- FaultFormatter.cs
- CommonXSendMessage.cs
- Material.cs
- OperatorExpressions.cs
- RunWorkerCompletedEventArgs.cs
- DetailsViewActionList.cs
- InputLangChangeRequestEvent.cs
- DoubleLinkList.cs
- CompareInfo.cs
- KeyConverter.cs
- EncoderBestFitFallback.cs
- XmlSchemaImporter.cs
- ICspAsymmetricAlgorithm.cs
- Binding.cs
- IsolatedStorageSecurityState.cs
- WebBodyFormatMessageProperty.cs
- SettingsPropertyWrongTypeException.cs
- _NestedSingleAsyncResult.cs
- ButtonColumn.cs
- LinkArea.cs
- SqlConnectionString.cs
- ActivationArguments.cs
- Point3DKeyFrameCollection.cs
- FormClosingEvent.cs
- PropertyValue.cs
- KeyInfo.cs
- RegionInfo.cs
- NavigationService.cs
- PeerNameRecordCollection.cs
- VirtualizedItemPattern.cs
- ZipIOBlockManager.cs
- Emitter.cs
- SchemaElement.cs
- EntityDataSourceContextCreatingEventArgs.cs
- MsmqOutputChannel.cs
- RunWorkerCompletedEventArgs.cs
- AddInToken.cs
- LockRenewalTask.cs
- GregorianCalendar.cs
- RootProfilePropertySettingsCollection.cs
- Separator.cs
- CodeMethodInvokeExpression.cs
- ThreadBehavior.cs
- CodeRegionDirective.cs
- MissingFieldException.cs
- CustomAttributeSerializer.cs
- XDeferredAxisSource.cs
- LocationInfo.cs
- GroupByQueryOperator.cs
- BitmapCodecInfo.cs
- Stack.cs
- OleDbWrapper.cs
- XNodeValidator.cs
- IPipelineRuntime.cs
- InputGestureCollection.cs
- MaskDescriptors.cs
- HtmlLinkAdapter.cs
- VectorAnimationBase.cs
- WindowsGraphics.cs
- listitem.cs
- AsymmetricAlgorithm.cs
- SchemaInfo.cs
- Debug.cs
- SelectionHighlightInfo.cs
- UriTemplateHelpers.cs
- NavigationPropertyEmitter.cs
- ConfigXmlText.cs
- SqlException.cs
- WizardPanel.cs
- KeysConverter.cs
- TypeNameConverter.cs
- ToolCreatedEventArgs.cs
- InlineUIContainer.cs
- TileModeValidation.cs
- userdatakeys.cs
- MethodCallConverter.cs
- LoadRetryStrategyFactory.cs
- XMLSchema.cs
- AnnotationAuthorChangedEventArgs.cs
- AuthorizationSection.cs