Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Reflection / Emit / MethodBuilderInstantiation.cs / 1 / MethodBuilderInstantiation.cs
using System; using System.Reflection; using System.Reflection.Emit; using System.Collections; using System.Globalization; namespace System.Reflection.Emit { internal sealed class MethodBuilderInstantiation : MethodInfo { #region Static Members internal static MethodInfo MakeGenericMethod(MethodInfo method, Type[] inst) { if (!method.IsGenericMethodDefinition) throw new InvalidOperationException(); return new MethodBuilderInstantiation(method, inst); } #endregion #region Private Data Mebers internal MethodInfo m_method; private Type[] m_inst; #endregion #region Constructor internal MethodBuilderInstantiation(MethodInfo method, Type[] inst) { m_method = method; m_inst = inst; } #endregion internal override Type[] GetParameterTypes() { return m_method.GetParameterTypes(); } #region MemberBase public override MemberTypes MemberType { get { return m_method.MemberType; } } public override String Name { get { return m_method.Name; } } public override Type DeclaringType { get { return m_method.DeclaringType; } } public override Type ReflectedType { get { return m_method.ReflectedType; } } public override Object[] GetCustomAttributes(bool inherit) { return m_method.GetCustomAttributes(inherit); } public override Object[] GetCustomAttributes(Type attributeType, bool inherit) { return m_method.GetCustomAttributes(attributeType, inherit); } public override bool IsDefined(Type attributeType, bool inherit) { return m_method.IsDefined(attributeType, inherit); } internal override int MetadataTokenInternal { get { throw new NotSupportedException(); } } public override Module Module { get { return m_method.Module; } } public new Type GetType() { return base.GetType(); } #endregion #region MethodBase Members public override ParameterInfo[] GetParameters() { throw new NotSupportedException(); } public override MethodImplAttributes GetMethodImplementationFlags() { return m_method.GetMethodImplementationFlags(); } public override RuntimeMethodHandle MethodHandle { get { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicModule")); } } public override MethodAttributes Attributes { get { return m_method.Attributes; } } public override Object Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture) { throw new NotSupportedException(); } public override CallingConventions CallingConvention { get { return m_method.CallingConvention; } } public override Type[] GetGenericArguments() { return m_inst; } public override MethodInfo GetGenericMethodDefinition() { return m_method; } public override bool IsGenericMethodDefinition { get { return false; } } public override bool ContainsGenericParameters { get { for (int i = 0; i < m_inst.Length; i++) { if (m_inst[i].ContainsGenericParameters) return true; } if (DeclaringType != null && DeclaringType.ContainsGenericParameters) return true; return false; } } public override MethodInfo MakeGenericMethod(params Type[] arguments) { throw new InvalidOperationException(Environment.GetResourceString("Arg_NotGenericMethodDefinition")); } public override bool IsGenericMethod { get { return true; } } #endregion #region Public Abstract\Virtual Members internal override Type GetReturnType() { return m_method.GetReturnType(); } public override ParameterInfo ReturnParameter { get { throw new NotSupportedException(); } } public override ICustomAttributeProvider ReturnTypeCustomAttributes { get { throw new NotSupportedException(); } } public override MethodInfo GetBaseDefinition() { throw new NotSupportedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Reflection; using System.Reflection.Emit; using System.Collections; using System.Globalization; namespace System.Reflection.Emit { internal sealed class MethodBuilderInstantiation : MethodInfo { #region Static Members internal static MethodInfo MakeGenericMethod(MethodInfo method, Type[] inst) { if (!method.IsGenericMethodDefinition) throw new InvalidOperationException(); return new MethodBuilderInstantiation(method, inst); } #endregion #region Private Data Mebers internal MethodInfo m_method; private Type[] m_inst; #endregion #region Constructor internal MethodBuilderInstantiation(MethodInfo method, Type[] inst) { m_method = method; m_inst = inst; } #endregion internal override Type[] GetParameterTypes() { return m_method.GetParameterTypes(); } #region MemberBase public override MemberTypes MemberType { get { return m_method.MemberType; } } public override String Name { get { return m_method.Name; } } public override Type DeclaringType { get { return m_method.DeclaringType; } } public override Type ReflectedType { get { return m_method.ReflectedType; } } public override Object[] GetCustomAttributes(bool inherit) { return m_method.GetCustomAttributes(inherit); } public override Object[] GetCustomAttributes(Type attributeType, bool inherit) { return m_method.GetCustomAttributes(attributeType, inherit); } public override bool IsDefined(Type attributeType, bool inherit) { return m_method.IsDefined(attributeType, inherit); } internal override int MetadataTokenInternal { get { throw new NotSupportedException(); } } public override Module Module { get { return m_method.Module; } } public new Type GetType() { return base.GetType(); } #endregion #region MethodBase Members public override ParameterInfo[] GetParameters() { throw new NotSupportedException(); } public override MethodImplAttributes GetMethodImplementationFlags() { return m_method.GetMethodImplementationFlags(); } public override RuntimeMethodHandle MethodHandle { get { throw new NotSupportedException(Environment.GetResourceString("NotSupported_DynamicModule")); } } public override MethodAttributes Attributes { get { return m_method.Attributes; } } public override Object Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture) { throw new NotSupportedException(); } public override CallingConventions CallingConvention { get { return m_method.CallingConvention; } } public override Type[] GetGenericArguments() { return m_inst; } public override MethodInfo GetGenericMethodDefinition() { return m_method; } public override bool IsGenericMethodDefinition { get { return false; } } public override bool ContainsGenericParameters { get { for (int i = 0; i < m_inst.Length; i++) { if (m_inst[i].ContainsGenericParameters) return true; } if (DeclaringType != null && DeclaringType.ContainsGenericParameters) return true; return false; } } public override MethodInfo MakeGenericMethod(params Type[] arguments) { throw new InvalidOperationException(Environment.GetResourceString("Arg_NotGenericMethodDefinition")); } public override bool IsGenericMethod { get { return true; } } #endregion #region Public Abstract\Virtual Members internal override Type GetReturnType() { return m_method.GetReturnType(); } public override ParameterInfo ReturnParameter { get { throw new NotSupportedException(); } } public override ICustomAttributeProvider ReturnTypeCustomAttributes { get { throw new NotSupportedException(); } } public override MethodInfo GetBaseDefinition() { throw new NotSupportedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
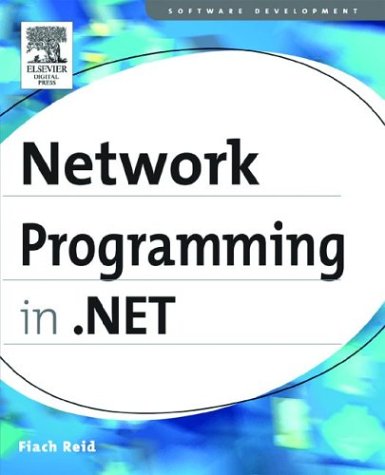
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDisplayModeCancelEventArgs.cs
- MetadataWorkspace.cs
- SubclassTypeValidatorAttribute.cs
- TraceHandlerErrorFormatter.cs
- FocusChangedEventArgs.cs
- FamilyMap.cs
- DoubleLinkListEnumerator.cs
- SchemaManager.cs
- ExpressionDumper.cs
- SafeNativeMethods.cs
- ScriptingAuthenticationServiceSection.cs
- LongMinMaxAggregationOperator.cs
- DNS.cs
- ColorConvertedBitmap.cs
- LinqDataSourceUpdateEventArgs.cs
- GPRECTF.cs
- ImageSourceTypeConverter.cs
- Rfc2898DeriveBytes.cs
- GridViewColumnHeader.cs
- serverconfig.cs
- TableDetailsRow.cs
- OdbcUtils.cs
- Color.cs
- BaseComponentEditor.cs
- EventWaitHandleSecurity.cs
- TypedElement.cs
- ModuleBuilderData.cs
- RequestCachePolicy.cs
- SchemaComplexType.cs
- BaseAsyncResult.cs
- FixedSOMPageElement.cs
- FormConverter.cs
- HttpCacheParams.cs
- AssemblyBuilder.cs
- WindowsListViewGroup.cs
- SessionPageStateSection.cs
- WindowsStartMenu.cs
- MutexSecurity.cs
- ApplicationServiceHelper.cs
- IdentityManager.cs
- DashStyle.cs
- DeclarativeCatalogPart.cs
- ReaderWriterLockWrapper.cs
- InternalPolicyElement.cs
- __Error.cs
- TargetConverter.cs
- SQLBinaryStorage.cs
- ButtonFlatAdapter.cs
- EntityViewGenerationConstants.cs
- Matrix3DStack.cs
- HTTPNotFoundHandler.cs
- DelimitedListTraceListener.cs
- SecurityTokenTypes.cs
- ScrollBar.cs
- ThreadStateException.cs
- CompositionDesigner.cs
- DateTimeStorage.cs
- ClientRequest.cs
- CheckBox.cs
- WebServiceEnumData.cs
- objectquery_tresulttype.cs
- ExternalFile.cs
- BitmapMetadata.cs
- StylusCaptureWithinProperty.cs
- ConfigViewGenerator.cs
- EdmError.cs
- ProfileInfo.cs
- UnmanagedMarshal.cs
- StateItem.cs
- ValidationError.cs
- LinqDataSourceStatusEventArgs.cs
- EntityDataSourceEntitySetNameItem.cs
- ActivityCollectionMarkupSerializer.cs
- PeerEndPoint.cs
- ItemCheckEvent.cs
- DataServiceHostFactory.cs
- entitydatasourceentitysetnameconverter.cs
- InputMethodStateTypeInfo.cs
- StreamSecurityUpgradeProvider.cs
- BamlVersionHeader.cs
- XamlReaderHelper.cs
- RewritingValidator.cs
- WebContext.cs
- Tile.cs
- HuffCodec.cs
- Process.cs
- ChangeProcessor.cs
- BrowserDefinition.cs
- DesignerListAdapter.cs
- DataGridViewButtonColumn.cs
- SoapReflectionImporter.cs
- NavigatingCancelEventArgs.cs
- Int32RectValueSerializer.cs
- TextTreeNode.cs
- XamlBrushSerializer.cs
- OleDbRowUpdatingEvent.cs
- AtomServiceDocumentSerializer.cs
- GridViewPageEventArgs.cs
- NumericUpDownAccelerationCollection.cs
- SettingsSection.cs