Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Generated / GradientStop.cs / 1 / GradientStop.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class GradientStop : Animatable, IFormattable { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GradientStop Clone() { return (GradientStop)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GradientStop CloneCurrentValue() { return (GradientStop)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Color - Color. Default value is Colors.Transparent. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Offset - double. Default value is 0.0. /// public double Offset { get { return (double) GetValue(OffsetProperty); } set { SetValueInternal(OffsetProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GradientStop(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}", separator, Color, Offset); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GradientStop.Color property. /// public static readonly DependencyProperty ColorProperty = RegisterProperty("Color", typeof(Color), typeof(GradientStop), Colors.Transparent, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GradientStop.Offset property. /// public static readonly DependencyProperty OffsetProperty = RegisterProperty("Offset", typeof(double), typeof(GradientStop), 0.0, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal static Color s_Color = Colors.Transparent; internal const double c_Offset = 0.0; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class GradientStop : Animatable, IFormattable { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GradientStop Clone() { return (GradientStop)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GradientStop CloneCurrentValue() { return (GradientStop)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Color - Color. Default value is Colors.Transparent. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// Offset - double. Default value is 0.0. /// public double Offset { get { return (double) GetValue(OffsetProperty); } set { SetValueInternal(OffsetProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GradientStop(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}", separator, Color, Offset); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GradientStop.Color property. /// public static readonly DependencyProperty ColorProperty = RegisterProperty("Color", typeof(Color), typeof(GradientStop), Colors.Transparent, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ////// The DependencyProperty for the GradientStop.Offset property. /// public static readonly DependencyProperty OffsetProperty = RegisterProperty("Offset", typeof(double), typeof(GradientStop), 0.0, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal static Color s_Color = Colors.Transparent; internal const double c_Offset = 0.0; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
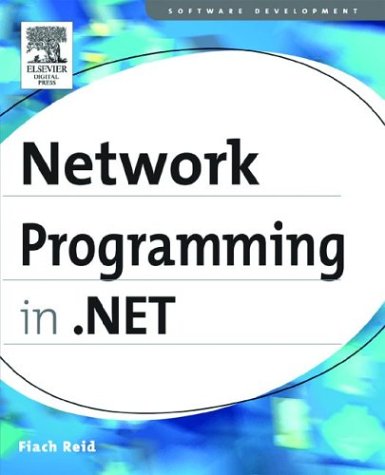
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpHandlerActionCollection.cs
- WindowsListViewSubItem.cs
- GroupItem.cs
- ArgumentDesigner.xaml.cs
- MdImport.cs
- ButtonRenderer.cs
- DataGridViewDataErrorEventArgs.cs
- PackagingUtilities.cs
- BufferModesCollection.cs
- validationstate.cs
- TextTreeNode.cs
- QilTernary.cs
- ToolStripGrip.cs
- TextureBrush.cs
- CachingHintValidation.cs
- SkewTransform.cs
- Emitter.cs
- LinqDataSourceInsertEventArgs.cs
- FilterableAttribute.cs
- AsyncOperationManager.cs
- TerminatorSinks.cs
- DocumentOrderQuery.cs
- MonthChangedEventArgs.cs
- DocumentSchemaValidator.cs
- UnsafeNativeMethods.cs
- XamlTemplateSerializer.cs
- ModelVisual3D.cs
- TextSchema.cs
- BaseCodeDomTreeGenerator.cs
- CodeValidator.cs
- PerformanceCounterPermissionAttribute.cs
- SafeArrayRankMismatchException.cs
- BrushValueSerializer.cs
- WebConvert.cs
- versioninfo.cs
- FloaterBaseParagraph.cs
- WebControlAdapter.cs
- ObjectViewListener.cs
- SQLChars.cs
- PrintDialog.cs
- XmlConvert.cs
- ValidationSummary.cs
- SchemaType.cs
- Positioning.cs
- PinProtectionHelper.cs
- QueryOutputWriterV1.cs
- BitmapScalingModeValidation.cs
- TableCell.cs
- ContextDataSourceContextData.cs
- IdentifierCreationService.cs
- DragEvent.cs
- XmlUtil.cs
- MultiView.cs
- DynamicResourceExtensionConverter.cs
- TitleStyle.cs
- RelationshipManager.cs
- WsatServiceCertificate.cs
- PageAsyncTaskManager.cs
- sqlnorm.cs
- JumpList.cs
- ButtonRenderer.cs
- MemberPathMap.cs
- DirectoryInfo.cs
- parserscommon.cs
- WebPartTransformerAttribute.cs
- DbResourceAllocator.cs
- DataSourceXmlSubItemAttribute.cs
- AsymmetricSecurityProtocolFactory.cs
- DispatchWrapper.cs
- BulletDecorator.cs
- HttpResponseInternalBase.cs
- DetailsViewDesigner.cs
- BamlTreeUpdater.cs
- StreamSecurityUpgradeAcceptorAsyncResult.cs
- DataGridParentRows.cs
- DetailsViewPagerRow.cs
- DbMetaDataFactory.cs
- Win32KeyboardDevice.cs
- EventSinkHelperWriter.cs
- TransformerConfigurationWizardBase.cs
- NopReturnReader.cs
- ProcessHostFactoryHelper.cs
- ToolBarButtonClickEvent.cs
- Configuration.cs
- TextBoxBase.cs
- DataBindingCollection.cs
- UnicodeEncoding.cs
- ObjectKeyFrameCollection.cs
- streamingZipPartStream.cs
- PropertyTabAttribute.cs
- DataSourceUtil.cs
- Peer.cs
- OutputCacheSettingsSection.cs
- TimeSpanMinutesConverter.cs
- TextServicesLoader.cs
- XmlStreamNodeWriter.cs
- IisTraceListener.cs
- DocumentApplicationJournalEntry.cs
- NonBatchDirectoryCompiler.cs
- SmiMetaData.cs