Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / TextServicesDisplayAttribute.cs / 1 / TextServicesDisplayAttribute.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } return Color.FromArgb(0xff,0,0,0); } ////// Line color of the composition line draw /// internal Color LineColor { get { return GetColor(_attr.crLine); } } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } return Color.FromArgb(0xff,0,0,0); } ////// Line color of the composition line draw /// internal Color LineColor { get { return GetColor(_attr.crLine); } } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
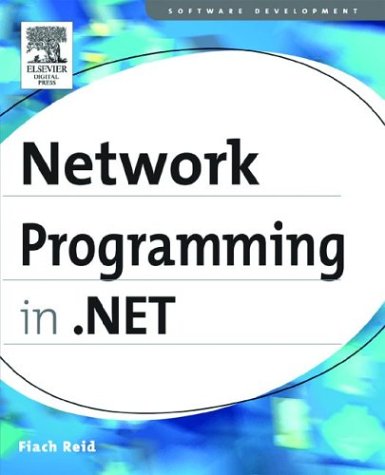
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthorizationRuleCollection.cs
- GeneralTransform3DGroup.cs
- URL.cs
- Stack.cs
- NameValueSectionHandler.cs
- ResourceDefaultValueAttribute.cs
- XmlSchemaAppInfo.cs
- CodeChecksumPragma.cs
- SqlStatistics.cs
- SqlLiftWhereClauses.cs
- ByteConverter.cs
- DataGridItemEventArgs.cs
- Thumb.cs
- ComponentGuaranteesAttribute.cs
- StringAnimationBase.cs
- ClientSettingsSection.cs
- securitycriticaldata.cs
- XmlSchemaAttributeGroupRef.cs
- GeometryConverter.cs
- GACMembershipCondition.cs
- QueryOptionExpression.cs
- ChildrenQuery.cs
- GroupStyle.cs
- BitmapFrameEncode.cs
- CreateUserWizardStep.cs
- TargetControlTypeAttribute.cs
- DrawingDrawingContext.cs
- TextTabProperties.cs
- AuthenticatingEventArgs.cs
- ResourcePermissionBaseEntry.cs
- PseudoWebRequest.cs
- Byte.cs
- XsdValidatingReader.cs
- NetworkInformationException.cs
- BitmapInitialize.cs
- TreeNodeCollection.cs
- PseudoWebRequest.cs
- StringResourceManager.cs
- UriParserTemplates.cs
- WmlObjectListAdapter.cs
- FileUtil.cs
- LambdaCompiler.Generated.cs
- SatelliteContractVersionAttribute.cs
- IPHostEntry.cs
- ToolStripLabel.cs
- ValidationSummary.cs
- HwndTarget.cs
- FixedPageAutomationPeer.cs
- DefaultHttpHandler.cs
- XsltException.cs
- ReadOnlyPropertyMetadata.cs
- ProjectionCamera.cs
- ToolboxItemCollection.cs
- TabControl.cs
- SelectedGridItemChangedEvent.cs
- NumericPagerField.cs
- MailAddress.cs
- XsdDateTime.cs
- ReturnEventArgs.cs
- Composition.cs
- ListSurrogate.cs
- NotificationContext.cs
- CodeCommentStatement.cs
- CryptoSession.cs
- DeviceContext2.cs
- wgx_commands.cs
- ContextTokenTypeConverter.cs
- InkSerializer.cs
- ClientType.cs
- TraceProvider.cs
- OverrideMode.cs
- Hex.cs
- Thread.cs
- PropertyItem.cs
- EndEvent.cs
- MessageDescription.cs
- MatrixUtil.cs
- Baml2006ReaderContext.cs
- DesignerActionMethodItem.cs
- DataGridColumnHeadersPresenter.cs
- HttpClientCertificate.cs
- TemplateContainer.cs
- CommandEventArgs.cs
- RenderingEventArgs.cs
- TextTreeRootTextBlock.cs
- ProfilePropertyNameValidator.cs
- RegexNode.cs
- OdbcEnvironmentHandle.cs
- ResourceContainer.cs
- HtmlToClrEventProxy.cs
- FrameworkElementFactory.cs
- ToolStripSplitButton.cs
- DocumentViewer.cs
- EnterpriseServicesHelper.cs
- UrlPath.cs
- ConstructorExpr.cs
- PageThemeCodeDomTreeGenerator.cs
- prompt.cs
- ModuleBuilderData.cs
- NotCondition.cs