Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / Primitives / Thumb.cs / 2 / Thumb.cs
using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Runtime.InteropServices; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Input; using System.Windows.Media; using MS.Utility; using MS.Win32; using MS.Internal; using MS.Internal.PresentationFramework; // SafeSecurityHelper namespace System.Windows.Controls.Primitives { ////// The thumb control enables basic drag-movement functionality for scrollbars and window resizing widgets. /// ////// The thumb can receive mouse focus but it cannot receive keyboard focus. /// As well, there is no threshhold at which the control stops firing its DragDeltaEvent. /// Once in mouse capture, the DragDeltaEvent fires until the mouse button is released. /// [DefaultEvent("DragDelta")] [Localizability(LocalizationCategory.NeverLocalize)] public class Thumb : Control { #region Constructors ////// Default Thumb constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Thumb() : base() { } static Thumb() { // Register metadata for dependency properties DefaultStyleKeyProperty.OverrideMetadata(typeof(Thumb), new FrameworkPropertyMetadata(typeof(Thumb))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(Thumb)); FocusableProperty.OverrideMetadata(typeof(Thumb), new FrameworkPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); EventManager.RegisterClassHandler(typeof(Thumb), Mouse.LostMouseCaptureEvent, new MouseEventHandler(OnLostMouseCapture)); } #endregion #region Properties and Events ////// Event fires when user press mouse's left button on the thumb. /// public static readonly RoutedEvent DragStartedEvent = EventManager.RegisterRoutedEvent("DragStarted", RoutingStrategy.Bubble, typeof(DragStartedEventHandler), typeof(Thumb)); ////// Event fires when the thumb is in a mouse capture state and the user moves the mouse around. /// public static readonly RoutedEvent DragDeltaEvent = EventManager.RegisterRoutedEvent("DragDelta", RoutingStrategy.Bubble, typeof(DragDeltaEventHandler), typeof(Thumb)); ////// Event fires when user released mouse's left button or when CancelDrag method is called. /// public static readonly RoutedEvent DragCompletedEvent = EventManager.RegisterRoutedEvent("DragCompleted", RoutingStrategy.Bubble, typeof(DragCompletedEventHandler), typeof(Thumb)); ////// Add / Remove DragStartedEvent handler /// [Category("Behavior")] public event DragStartedEventHandler DragStarted { add { AddHandler(DragStartedEvent, value); } remove { RemoveHandler(DragStartedEvent, value); } } ////// Add / Remove DragDeltaEvent handler /// [Category("Behavior")] public event DragDeltaEventHandler DragDelta { add { AddHandler(DragDeltaEvent, value); } remove { RemoveHandler(DragDeltaEvent, value); } } ////// Add / Remove DragCompletedEvent handler /// [Category("Behavior")] public event DragCompletedEventHandler DragCompleted { add { AddHandler(DragCompletedEvent, value); } remove { RemoveHandler(DragCompletedEvent, value); } } private static readonly DependencyPropertyKey IsDraggingPropertyKey = DependencyProperty.RegisterReadOnly( "IsDragging", typeof(bool), typeof(Thumb), new FrameworkPropertyMetadata( MS.Internal.KnownBoxes.BooleanBoxes.FalseBox, new PropertyChangedCallback(OnIsDraggingPropertyChanged))); ////// DependencyProperty for the IsDragging property. /// Flags: None /// Default Value: false /// public static readonly DependencyProperty IsDraggingProperty = IsDraggingPropertyKey.DependencyProperty; ////// IsDragging indicates that left mouse button is pressed over the thumb. /// [Bindable(true), Browsable(false), Category("Appearance")] public bool IsDragging { get { return (bool) GetValue(IsDraggingProperty); } protected set { SetValue(IsDraggingPropertyKey, MS.Internal.KnownBoxes.BooleanBoxes.Box(value)); } } #endregion Properties and Events ////// Called when IsDraggingProperty is changed on "d." /// /// The object on which the property was changed. /// EventArgs that contains the old and new values for this property private static void OnIsDraggingPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((Thumb) d).OnDraggingChanged(e); } #region Public methods ////// This method cancels the dragging operation. /// public void CancelDrag() { if (IsDragging) { if (IsMouseCaptured) { ReleaseMouseCapture(); } ClearValue(IsDraggingPropertyKey); RaiseEvent(new DragCompletedEventArgs(_previousScreenCoordPosition.X - _originScreenCoordPosition.X, _previousScreenCoordPosition.Y - _originScreenCoordPosition.Y, true)); } } #endregion Public methods #region Virtual methods ////// This method is invoked when the IsDragging property changes. /// /// DependencyPropertyChangedEventArgs for IsDragging property. protected virtual void OnDraggingChanged(DependencyPropertyChangedEventArgs e) { } #endregion Virtual methods #region Override methods ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ThumbAutomationPeer(this); } ///) /// /// This is the method that responds to the MouseButtonEvent event. /// /// protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { if (!IsDragging) { e.Handled = true; Focus(); CaptureMouse(); SetValue(IsDraggingPropertyKey, true); _originThumbPoint = e.GetPosition(this); _previousScreenCoordPosition = _originScreenCoordPosition = SafeSecurityHelper.ClientToScreen(this,_originThumbPoint); bool exceptionThrown = true; try { RaiseEvent(new DragStartedEventArgs(_originThumbPoint.X, _originThumbPoint.Y)); exceptionThrown = false; } finally { if (exceptionThrown) { CancelDrag(); } } } else { // This is weird, Thumb shouldn't get MouseLeftButtonDown event while dragging. // This may be the case that something ate MouseLeftButtonUp event, so Thumb never had a chance to // reset IsDragging property Debug.Assert(false,"Got MouseLeftButtonDown event while dragging!"); } base.OnMouseLeftButtonDown(e); } ////// This is the method that responds to the MouseButtonEvent event. /// /// protected override void OnMouseLeftButtonUp(MouseButtonEventArgs e) { if (IsMouseCaptured && IsDragging) { e.Handled = true; ClearValue(IsDraggingPropertyKey); ReleaseMouseCapture(); Point pt = SafeSecurityHelper.ClientToScreen(this, e.MouseDevice.GetPosition(this)); RaiseEvent(new DragCompletedEventArgs(pt.X - _originScreenCoordPosition.X, pt.Y - _originScreenCoordPosition.Y, false)); } base.OnMouseLeftButtonUp(e); } // Cancel Drag if we lost capture private static void OnLostMouseCapture(object sender, MouseEventArgs e) { Thumb thumb = (Thumb)sender; if (Mouse.Captured != thumb) { thumb.CancelDrag(); } } ////// This is the method that responds to the MouseEvent event. /// /// protected override void OnMouseMove(MouseEventArgs e) { base.OnMouseMove(e); if (IsDragging) { if (e.MouseDevice.LeftButton == MouseButtonState.Pressed) { Point thumbCoordPosition = e.GetPosition(this); // Get client point then convert to screen point Point screenCoordPosition = SafeSecurityHelper.ClientToScreen(this, thumbCoordPosition); // We will fire DragDelta event only when the mouse is really moved if (screenCoordPosition != _previousScreenCoordPosition) { _previousScreenCoordPosition = screenCoordPosition; e.Handled = true; RaiseEvent(new DragDeltaEventArgs(thumbCoordPosition.X - _originThumbPoint.X, thumbCoordPosition.Y - _originThumbPoint.Y)); } } else { if (e.MouseDevice.Captured == this) ReleaseMouseCapture(); ClearValue(IsDraggingPropertyKey); _originThumbPoint.X = 0; _originThumbPoint.Y = 0; } } } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 19; } } #endregion #region Data ////// The point where the mouse was clicked down (Thumb's co-ordinate). /// private Point _originThumbPoint; // ////// The position of the mouse (screen co-ordinate) where the mouse was clicked down. /// private Point _originScreenCoordPosition; ////// The position of the mouse (screen co-ordinate) when the previous DragDelta event was fired /// private Point _previousScreenCoordPosition; #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
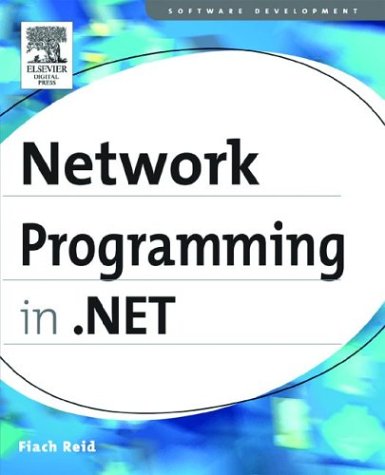
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinqDataSourceDisposeEventArgs.cs
- DecoderReplacementFallback.cs
- DocumentPageView.cs
- FormsAuthenticationCredentials.cs
- Block.cs
- CommunicationException.cs
- AudioDeviceOut.cs
- HttpListenerRequest.cs
- NamespaceCollection.cs
- CommandDevice.cs
- OciHandle.cs
- EntityContainerEmitter.cs
- AsyncCompletedEventArgs.cs
- DoubleAnimationUsingKeyFrames.cs
- WsdlParser.cs
- connectionpool.cs
- WebPartManagerInternals.cs
- NodeInfo.cs
- FontFamily.cs
- FactoryMaker.cs
- BufferModeSettings.cs
- AsyncContentLoadedEventArgs.cs
- ObjectCacheSettings.cs
- SamlAuthenticationClaimResource.cs
- RemotingConfigParser.cs
- WebPartCloseVerb.cs
- DefaultClaimSet.cs
- RequestCacheEntry.cs
- WindowsUpDown.cs
- TextRangeSerialization.cs
- ComponentCommands.cs
- ListViewUpdateEventArgs.cs
- TextEndOfSegment.cs
- SourceSwitch.cs
- CompilerTypeWithParams.cs
- ParseHttpDate.cs
- XmlAttributeCache.cs
- TextLineBreak.cs
- PageParserFilter.cs
- DiscoveryCallbackBehavior.cs
- SqlConnection.cs
- CodeArrayIndexerExpression.cs
- InstanceKeyCompleteException.cs
- ProfileInfo.cs
- VisualBrush.cs
- VectorValueSerializer.cs
- CommandTreeTypeHelper.cs
- AnchoredBlock.cs
- XPathAncestorQuery.cs
- xmlfixedPageInfo.cs
- Application.cs
- SamlAuthorityBinding.cs
- MobileControlsSectionHandler.cs
- _NegoState.cs
- MouseCaptureWithinProperty.cs
- InvalidateEvent.cs
- MediaPlayerState.cs
- wmiprovider.cs
- DocumentReference.cs
- RelationshipWrapper.cs
- InternalResources.cs
- MarkedHighlightComponent.cs
- Psha1DerivedKeyGenerator.cs
- InkCanvasAutomationPeer.cs
- ErrorWrapper.cs
- NullableIntMinMaxAggregationOperator.cs
- CryptoApi.cs
- RTLAwareMessageBox.cs
- TreeViewAutomationPeer.cs
- ConnectionManagementElementCollection.cs
- WebPartManager.cs
- TextTreeRootTextBlock.cs
- CodeTypeMember.cs
- connectionpool.cs
- Label.cs
- MetadataWorkspace.cs
- ManifestResourceInfo.cs
- SchemaEntity.cs
- XmlSchemaIdentityConstraint.cs
- XmlDictionaryReaderQuotas.cs
- ReaderContextStackData.cs
- TraceUtility.cs
- Pair.cs
- OuterGlowBitmapEffect.cs
- MultipleCopiesCollection.cs
- DeferredElementTreeState.cs
- LogEntryHeaderv1Deserializer.cs
- SafeUserTokenHandle.cs
- IntranetCredentialPolicy.cs
- LostFocusEventManager.cs
- ExpressionConverter.cs
- TagMapCollection.cs
- HttpRequest.cs
- CodeTypeOfExpression.cs
- MenuBindingsEditor.cs
- DropShadowBitmapEffect.cs
- RadioButton.cs
- XPathSelectionIterator.cs
- hwndwrapper.cs
- ListViewPagedDataSource.cs