Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Data / PropertyGroupDescription.cs / 1 / PropertyGroupDescription.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Description of grouping based on a property value. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; // StringComparison using System.ComponentModel; // [DefaultValue] using System.Globalization; // CultureInfo using System.Reflection; // PropertyInfo using System.Windows; // SR using System.Xml; // XmlNode using MS.Internal; // XmlHelper namespace System.Windows.Data { ////// Description of grouping based on a property value. /// public class PropertyGroupDescription : GroupDescription { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Initializes a new instance of PropertyGroupDescription. /// public PropertyGroupDescription() { } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// public PropertyGroupDescription(string propertyName) { UpdatePropertyName(propertyName); } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// public PropertyGroupDescription(string propertyName, IValueConverter converter) { UpdatePropertyName(propertyName); _converter = converter; } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// /// /// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// public PropertyGroupDescription(string propertyName, IValueConverter converter, StringComparison stringComparison) { UpdatePropertyName(propertyName); _converter = converter; _stringComparison = stringComparison; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// [DefaultValue(null)] public string PropertyName { get { return _propertyName; } set { UpdatePropertyName(value); OnPropertyChanged("PropertyName"); } } ////// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// [DefaultValue(null)] public IValueConverter Converter { get { return _converter; } set { _converter = value; OnPropertyChanged("Converter"); } } ////// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// [DefaultValue(StringComparison.Ordinal)] public StringComparison StringComparison { get { return _stringComparison; } set { _stringComparison = value; OnPropertyChanged("StringComparison"); } } #endregion Public Properties #region Public Methods //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ ////// Return the group name(s) for the given item /// public override object GroupNameFromItem(object item, int level, CultureInfo culture) { object value; // get the property value if (String.IsNullOrEmpty(PropertyName)) { value = item; } else if (XmlHelper.IsXmlNode(item)) { value = GetValueFromXmlNode(item); } else if (item != null) { using (_propertyPath.SetContext(item)) { value = _propertyPath.GetValue(); } } else { value = null; } // apply the converter to the value if (Converter != null) { value = Converter.Convert(value, typeof(object), level, culture); } return value; } ////// Return true if the names match (i.e the item should belong to the group). /// public override bool NamesMatch(object groupName, object itemName) { string s1 = groupName as string; string s2 = itemName as string; if (s1 != null && s2 != null) { return String.Equals(s1, s2, StringComparison); } else { return Object.Equals(groupName, itemName); } } #endregion Public Methods #region Private Methods private void UpdatePropertyName(string propertyName) { _propertyName = propertyName; _propertyPath = !String.IsNullOrEmpty(propertyName) ? new PropertyPath(propertyName) : null; } private void OnPropertyChanged(string propertyName) { OnPropertyChanged(new PropertyChangedEventArgs(propertyName)); } #endregion Private Methods // separate method to avoid loading System.Xml [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private object GetValueFromXmlNode(object item) { return XmlHelper.SelectStringValue((XmlNode)item, PropertyName); } #region Private Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ string _propertyName; PropertyPath _propertyPath; IValueConverter _converter; StringComparison _stringComparison = StringComparison.Ordinal; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Description of grouping based on a property value. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; // StringComparison using System.ComponentModel; // [DefaultValue] using System.Globalization; // CultureInfo using System.Reflection; // PropertyInfo using System.Windows; // SR using System.Xml; // XmlNode using MS.Internal; // XmlHelper namespace System.Windows.Data { ////// Description of grouping based on a property value. /// public class PropertyGroupDescription : GroupDescription { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Initializes a new instance of PropertyGroupDescription. /// public PropertyGroupDescription() { } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// public PropertyGroupDescription(string propertyName) { UpdatePropertyName(propertyName); } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// public PropertyGroupDescription(string propertyName, IValueConverter converter) { UpdatePropertyName(propertyName); _converter = converter; } ////// Initializes a new instance of PropertyGroupDescription. /// /// /// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// /// /// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// /// /// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// public PropertyGroupDescription(string propertyName, IValueConverter converter, StringComparison stringComparison) { UpdatePropertyName(propertyName); _converter = converter; _stringComparison = stringComparison; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The name of the property whose value is used to determine which group(s) /// an item belongs to. /// If PropertyName is null, the item itself is used. /// [DefaultValue(null)] public string PropertyName { get { return _propertyName; } set { UpdatePropertyName(value); OnPropertyChanged("PropertyName"); } } ////// This converter is applied to the property value (or the item) to /// produce the final value used to determine which group(s) an item /// belongs to. /// If the delegate returns an ICollection, the item is added to /// multiple groups - one for each member of the collection. /// [DefaultValue(null)] public IValueConverter Converter { get { return _converter; } set { _converter = value; OnPropertyChanged("Converter"); } } ////// This governs the comparison between an item's value (as determined /// by PropertyName and Converter) and a group's name. /// It is ignored unless both comparands are strings. /// The default value is StringComparison.Ordinal. /// [DefaultValue(StringComparison.Ordinal)] public StringComparison StringComparison { get { return _stringComparison; } set { _stringComparison = value; OnPropertyChanged("StringComparison"); } } #endregion Public Properties #region Public Methods //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ ////// Return the group name(s) for the given item /// public override object GroupNameFromItem(object item, int level, CultureInfo culture) { object value; // get the property value if (String.IsNullOrEmpty(PropertyName)) { value = item; } else if (XmlHelper.IsXmlNode(item)) { value = GetValueFromXmlNode(item); } else if (item != null) { using (_propertyPath.SetContext(item)) { value = _propertyPath.GetValue(); } } else { value = null; } // apply the converter to the value if (Converter != null) { value = Converter.Convert(value, typeof(object), level, culture); } return value; } ////// Return true if the names match (i.e the item should belong to the group). /// public override bool NamesMatch(object groupName, object itemName) { string s1 = groupName as string; string s2 = itemName as string; if (s1 != null && s2 != null) { return String.Equals(s1, s2, StringComparison); } else { return Object.Equals(groupName, itemName); } } #endregion Public Methods #region Private Methods private void UpdatePropertyName(string propertyName) { _propertyName = propertyName; _propertyPath = !String.IsNullOrEmpty(propertyName) ? new PropertyPath(propertyName) : null; } private void OnPropertyChanged(string propertyName) { OnPropertyChanged(new PropertyChangedEventArgs(propertyName)); } #endregion Private Methods // separate method to avoid loading System.Xml [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private object GetValueFromXmlNode(object item) { return XmlHelper.SelectStringValue((XmlNode)item, PropertyName); } #region Private Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ string _propertyName; PropertyPath _propertyPath; IValueConverter _converter; StringComparison _stringComparison = StringComparison.Ordinal; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
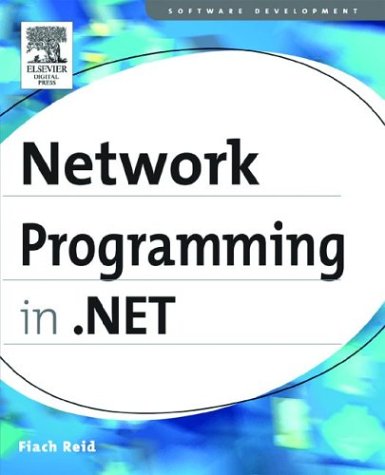
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataException.cs
- DataTemplateKey.cs
- Lasso.cs
- PartialCachingControl.cs
- WebPartHelpVerb.cs
- ConsoleCancelEventArgs.cs
- Point3DValueSerializer.cs
- ListViewInsertEventArgs.cs
- SecurityProtocolFactory.cs
- BitmapEffectInputData.cs
- SqlXml.cs
- XmlBindingWorker.cs
- StrokeIntersection.cs
- XmlQueryTypeFactory.cs
- ServiceActivationException.cs
- TrimSurroundingWhitespaceAttribute.cs
- BitmapData.cs
- HtmlShimManager.cs
- MouseGesture.cs
- Visual3D.cs
- OleDbParameter.cs
- ChildrenQuery.cs
- DocumentDesigner.cs
- AdjustableArrowCap.cs
- RegexGroup.cs
- StrongNameIdentityPermission.cs
- XmlMapping.cs
- Debug.cs
- CompiledELinqQueryState.cs
- HttpVersion.cs
- DelegateInArgument.cs
- RecordManager.cs
- NTAccount.cs
- SystemIPGlobalStatistics.cs
- MimeMapping.cs
- SelectionProcessor.cs
- AnimationClockResource.cs
- TdsEnums.cs
- PathSegment.cs
- MobileControlsSectionHelper.cs
- RequestResizeEvent.cs
- AuthenticationServiceManager.cs
- NGCUIElementCollectionSerializerAsync.cs
- JsonSerializer.cs
- SqlServices.cs
- QilReplaceVisitor.cs
- XmlMtomWriter.cs
- TableCell.cs
- NativeMethods.cs
- followingsibling.cs
- AttachInfo.cs
- SmtpNegotiateAuthenticationModule.cs
- KoreanLunisolarCalendar.cs
- FlowLayoutPanel.cs
- TitleStyle.cs
- ScriptServiceAttribute.cs
- PropertyInformation.cs
- SelectionRange.cs
- GifBitmapDecoder.cs
- CodeAccessSecurityEngine.cs
- ServicesUtilities.cs
- DataPointer.cs
- TransformPattern.cs
- DivideByZeroException.cs
- CompilationSection.cs
- EventMappingSettingsCollection.cs
- TimeoutValidationAttribute.cs
- FileLoadException.cs
- SerialPinChanges.cs
- WebBrowserEvent.cs
- QueuePathDialog.cs
- BuildProviderCollection.cs
- ImageCodecInfo.cs
- FrameAutomationPeer.cs
- Int16KeyFrameCollection.cs
- HMACSHA1.cs
- Variant.cs
- XPathEmptyIterator.cs
- RegistrySecurity.cs
- GlobalizationSection.cs
- TrackingProfile.cs
- Keywords.cs
- ComplexTypeEmitter.cs
- OleDbPropertySetGuid.cs
- TextFormatterImp.cs
- FixedSOMPageElement.cs
- ListenerSessionConnection.cs
- SerialReceived.cs
- WebBrowserDocumentCompletedEventHandler.cs
- ImageListStreamer.cs
- SqlConnectionStringBuilder.cs
- XmlJsonReader.cs
- RectAnimationUsingKeyFrames.cs
- DataObject.cs
- GlyphCollection.cs
- UnitControl.cs
- RepeatEnumerable.cs
- EventRecord.cs
- OdbcEnvironmentHandle.cs
- BadImageFormatException.cs