Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / XPath / Internal / precedingsibling.cs / 1 / precedingsibling.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
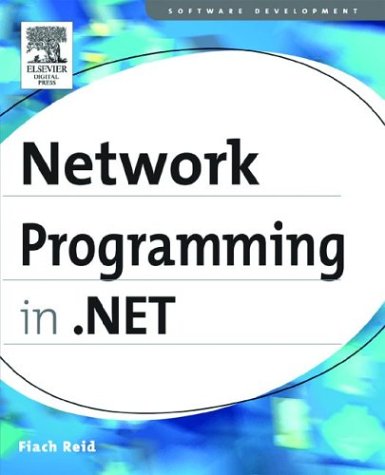
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OrderedDictionary.cs
- HtmlString.cs
- AlgoModule.cs
- sqlinternaltransaction.cs
- SectionVisual.cs
- PolicyValidationException.cs
- GACMembershipCondition.cs
- DelegatingConfigHost.cs
- WpfWebRequestHelper.cs
- HWStack.cs
- ProtocolsSection.cs
- LinqDataView.cs
- DrawToolTipEventArgs.cs
- IndexerNameAttribute.cs
- SecurityDescriptor.cs
- PropertyDescriptor.cs
- LambdaCompiler.cs
- FormViewDeletedEventArgs.cs
- MenuItem.cs
- EdmScalarPropertyAttribute.cs
- DataControlHelper.cs
- RegistryDataKey.cs
- DataGridColumn.cs
- ListViewInsertionMark.cs
- WindowsListViewItemStartMenu.cs
- ParenthesizePropertyNameAttribute.cs
- DocumentPaginator.cs
- Win32Exception.cs
- GlobalProxySelection.cs
- __Filters.cs
- FrameworkEventSource.cs
- InlinedLocationReference.cs
- Tuple.cs
- SqlUDTStorage.cs
- TemplateGroupCollection.cs
- SourceElementsCollection.cs
- MemoryRecordBuffer.cs
- Visual3D.cs
- DesignerProperties.cs
- Range.cs
- MessageBodyMemberAttribute.cs
- Simplifier.cs
- ParentControlDesigner.cs
- InvokeHandlers.cs
- PropertyGrid.cs
- ParseElementCollection.cs
- MarkupExtensionSerializer.cs
- ConfigurationStrings.cs
- OutputCacheSettingsSection.cs
- PropertyDescriptor.cs
- HtmlHead.cs
- Internal.cs
- AdornerDecorator.cs
- TimeIntervalCollection.cs
- CryptoStream.cs
- BaseComponentEditor.cs
- AsyncStreamReader.cs
- StyleBamlRecordReader.cs
- WhitespaceRuleReader.cs
- DocumentViewerConstants.cs
- SharedStatics.cs
- XamlBrushSerializer.cs
- VerificationAttribute.cs
- MultiSelector.cs
- SafeBitVector32.cs
- ExpressionContext.cs
- FlowchartStart.xaml.cs
- TransformDescriptor.cs
- EdmProperty.cs
- ActionFrame.cs
- NotCondition.cs
- _TransmitFileOverlappedAsyncResult.cs
- ADConnectionHelper.cs
- ResourceAssociationSetEnd.cs
- StateMachine.cs
- ObjectParameter.cs
- controlskin.cs
- WebPartDisplayModeCollection.cs
- counter.cs
- SmiEventStream.cs
- PenContext.cs
- InitializerFacet.cs
- DayRenderEvent.cs
- ExtendedPropertyCollection.cs
- BoundingRectTracker.cs
- WebPartTracker.cs
- SqlAliaser.cs
- SqlBulkCopy.cs
- DateTimeConstantAttribute.cs
- SimplePropertyEntry.cs
- SqlCacheDependencyDatabaseCollection.cs
- TracePayload.cs
- PrefixHandle.cs
- RetrieveVirtualItemEventArgs.cs
- InvalidPrinterException.cs
- Int16Animation.cs
- CounterCreationDataConverter.cs
- SemanticTag.cs
- DataGridViewCellPaintingEventArgs.cs
- SpellerStatusTable.cs