Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / TextParagraphCache.cs / 1 / TextParagraphCache.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextParagraphCache.cs // // Contents: Cache object of paragraph content used to improve performance // of optimal paragraph formatting // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-4-2005 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Diagnostics; using System.Collections.Generic; using System.Security; using System.Windows; using System.Windows.Media; using MS.Internal; using MS.Internal.TextFormatting; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// Text formatter caches all potential breakpoints within a paragraph in this cache object /// during FormatParagraphContent call. This object is to be managed by text layout client. /// The use of this cache is to improve performance of line construction in optimal paragraph /// line formatting. /// #if OPTIMALBREAK_API public sealed class TextParagraphCache : IDisposable #else [FriendAccessAllowed] internal sealed class TextParagraphCache : IDisposable #endif { private FullTextState _fullText; // full text state of the whole paragraph private SecurityCriticalDataForSet_ploparabreak; // unmanaged LS resource for parabreak session private int _finiteFormatWidth; // finite formatting ideal width private bool _penalizedAsJustified; // flag indicating whether the paragraph should be penalized as fully-justified one /// /// Construct a paragraph cache to be used during optimal paragraph formatting /// ////// Critical - as this calls the setter for _ploparabreak.Value which is type SecurityCriticalDataForSet. /// Safe - as it doesn't set security critical data to a random value passed in but rather to a value returned /// by a safe function TextFormatterContext.CreateParaBreakingSession(). /// [SecurityCritical, SecurityTreatAsSafe] internal TextParagraphCache( FormatSettings settings, int firstCharIndex, int paragraphWidth ) { Invariant.Assert(settings != null); // create full text _finiteFormatWidth = settings.GetFiniteFormatWidth(paragraphWidth); _fullText = FullTextState.Create(settings, firstCharIndex, _finiteFormatWidth); // acquiring LS context TextFormatterContext context = settings.Formatter.AcquireContext(_fullText, IntPtr.Zero); _fullText.SetTabs(context); IntPtr ploparabreakValue = IntPtr.Zero; LsErr lserr = context.CreateParaBreakingSession( firstCharIndex, _finiteFormatWidth, // breakrec is not needed before the first cp of para cache // since we handle Bidi break ourselves. IntPtr.Zero, ref ploparabreakValue, ref _penalizedAsJustified ); // get the exception in context before it is released Exception callbackException = context.CallbackException; // release the context context.Release(); if(lserr != LsErr.None) { GC.SuppressFinalize(this); if(callbackException != null) { // rethrow exception thrown in callbacks throw new InvalidOperationException(SR.Get(SRID.CreateParaBreakingSessionFailure, lserr), callbackException); } else { // throw with LS error codes TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.CreateParaBreakingSessionFailure, lserr), lserr); } } _ploparabreak.Value = ploparabreakValue; // keep context alive till here GC.KeepAlive(context); } ////// Finalizing paragraph content cache /// ~TextParagraphCache() { Dispose(false); } ////// Disposing paragraph content cache /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Client to format all feasible breakpoints for a line started at the specified character position. /// The number of breakpoints returned is restricted by penalty restr /// ////// This method is provided for direct access of PTS during optimal paragraph process. The breakpoint /// restriction handle is passed as part of the parameter to PTS callback pfnFormatLineVariants. The /// value comes from earlier steps in PTS code to compute the accumulated penalty of the entire paragraph /// using 'best-fit' algorithm. /// internal IListFormatBreakpoints( int firstCharIndex, TextLineBreak previousLineBreak, IntPtr breakpointRestrictionHandle, double maxLineWidth, out int bestFitIndex ) { // format all potential breakpoints starting from the specified firstCharIndex. // The number of breakpoints returned is restricted by penaltyRestriction. return FullTextBreakpoint.CreateMultiple( this, firstCharIndex, VerifyMaxLineWidth(maxLineWidth), previousLineBreak, breakpointRestrictionHandle, out bestFitIndex ); } /// /// Releasing LS unmanaged resource on paragraph content /// ////// Critical - as this sets critical data _ploparabreak /// Safe - as it does not set critical data thru incoming parameter /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { if(_ploparabreak.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposeParaBreakingSession(_ploparabreak.Value, !disposing); _ploparabreak.Value = IntPtr.Zero; GC.KeepAlive(this); } } ////// Verify that the input line format width is within the maximum ideal value /// private int VerifyMaxLineWidth(double maxLineWidth) { double realInfiniteWidth = FullText.Formatter.IdealToReal(Constants.InfiniteWidth); if (DoubleUtil.IsNaN(maxLineWidth)) throw new ArgumentOutOfRangeException("maxLineWidth", SR.Get(SRID.ParameterValueCannotBeNaN)); if (maxLineWidth == 0 || double.IsPositiveInfinity(maxLineWidth)) { // consider 0 or positive infinity as maximum ideal width return Constants.InfiniteWidth; } if ( maxLineWidth < 0 || maxLineWidth > realInfiniteWidth) { throw new ArgumentOutOfRangeException("maxLineWidth", SR.Get(SRID.ParameterMustBeBetween, 0, realInfiniteWidth)); } // convert real value to ideal value return FullText.Formatter.RealToIdeal(maxLineWidth); } ////// Full text state of the paragraph /// internal FullTextState FullText { get { return _fullText; } } ////// Unmanaged LS parabreak session object /// internal SecurityCriticalDataForSetPloparabreak { get { return _ploparabreak; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
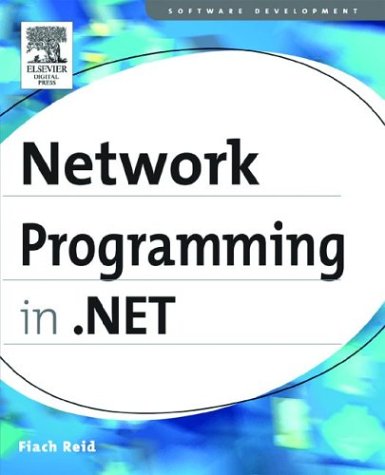
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XhtmlBasicPageAdapter.cs
- SqlMethodCallConverter.cs
- SetIterators.cs
- ResourceContainer.cs
- ListViewDeleteEventArgs.cs
- EnumerableCollectionView.cs
- ConnectionPointCookie.cs
- VirtualizedItemProviderWrapper.cs
- BevelBitmapEffect.cs
- XPathNavigator.cs
- EntityTypeEmitter.cs
- ErrorStyle.cs
- DictionaryBase.cs
- ValidationHelper.cs
- ErrorFormatterPage.cs
- ToolStripSettings.cs
- ViewLoader.cs
- PagesSection.cs
- DataGridViewRowEventArgs.cs
- ConfigurationSettings.cs
- WeakEventManager.cs
- DbQueryCommandTree.cs
- ZipIOLocalFileDataDescriptor.cs
- OutOfMemoryException.cs
- BufferAllocator.cs
- ServerValidateEventArgs.cs
- TrackingConditionCollection.cs
- GrammarBuilderPhrase.cs
- UriScheme.cs
- XsdBuildProvider.cs
- WriteableBitmap.cs
- ClockGroup.cs
- EnvironmentPermission.cs
- ListViewUpdateEventArgs.cs
- Comparer.cs
- XmlEntityReference.cs
- ListBindingConverter.cs
- AssertSection.cs
- RawStylusSystemGestureInputReport.cs
- XPathNodeHelper.cs
- CodeRemoveEventStatement.cs
- TransportBindingElementImporter.cs
- X509ChainElement.cs
- XhtmlBasicObjectListAdapter.cs
- MapPathBasedVirtualPathProvider.cs
- IgnoreSectionHandler.cs
- Material.cs
- IsolatedStorageFilePermission.cs
- MultipleFilterMatchesException.cs
- XamlTreeBuilderBamlRecordWriter.cs
- Page.cs
- XD.cs
- EnumType.cs
- PrinterUnitConvert.cs
- WindowsFormsEditorServiceHelper.cs
- TypedTableGenerator.cs
- SurrogateSelector.cs
- DoubleAnimation.cs
- ThumbButtonInfo.cs
- TickBar.cs
- StartUpEventArgs.cs
- PropertyPathWorker.cs
- ProfileEventArgs.cs
- CodeExpressionRuleDeclaration.cs
- BitmapEffectDrawingContextWalker.cs
- DashStyles.cs
- ThicknessAnimationBase.cs
- path.cs
- LocatorGroup.cs
- AmbientLight.cs
- SourceLocation.cs
- SrgsOneOf.cs
- DataPointer.cs
- PaperSize.cs
- AnnotationResource.cs
- ControlAdapter.cs
- TraceSwitch.cs
- Stackframe.cs
- ReadOnlyHierarchicalDataSourceView.cs
- DataGridItemAttachedStorage.cs
- GB18030Encoding.cs
- TabletCollection.cs
- DocumentXmlWriter.cs
- ReservationCollection.cs
- ButtonColumn.cs
- ConfigurationStrings.cs
- AlphabeticalEnumConverter.cs
- Menu.cs
- ResourceExpressionBuilder.cs
- CheckBox.cs
- PropertyRecord.cs
- WaitForChangedResult.cs
- CodeGeneratorOptions.cs
- TypedColumnHandler.cs
- RepeatBehavior.cs
- QuadraticBezierSegment.cs
- FileCodeGroup.cs
- PropertyValueUIItem.cs
- ADConnectionHelper.cs
- ProjectionPlanCompiler.cs