Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Adapters / ChtmlTextBoxAdapter.cs / 1305376 / ChtmlTextBoxAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security.Permissions; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource #else namespace System.Web.UI.MobileControls.Adapters #endif { /* * ChtmlTextBoxAdapter class. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class ChtmlTextBoxAdapter : HtmlTextBoxAdapter { private static Random _random = new Random(); /// protected override void AddAttributes(HtmlMobileTextWriter writer) { if (Control.Numeric) { if (Device.SupportsInputIStyle) { // The default input mode is always numeric if the // type is password. if (!Control.Password) { writer.WriteAttribute("istyle", "4"); } } else if (Device.SupportsInputMode) { writer.WriteAttribute("mode", "numeric"); } } AddAccesskeyAttribute(writer); AddJPhoneMultiMediaAttributes(writer); } /// public override bool RequiresFormTag { get { return true; } } private String GetRandomID(int length) { Byte[] randomBytes = new Byte[length]; _random.NextBytes(randomBytes); char[] randomChars = new char[length]; for (int i = 0; i < length; i++) { randomChars[i] = (char)((((int)randomBytes[i]) % 26) + 'a'); } return new String(randomChars); } internal override String GetRenderName() { String renderName = base.GetRenderName(); if (Device.RequiresUniqueHtmlInputNames) { renderName += Constants.SelectionListSpecialCharacter + GetRandomID(4); } return renderName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security.Permissions; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource #else namespace System.Web.UI.MobileControls.Adapters #endif { /* * ChtmlTextBoxAdapter class. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class ChtmlTextBoxAdapter : HtmlTextBoxAdapter { private static Random _random = new Random(); /// protected override void AddAttributes(HtmlMobileTextWriter writer) { if (Control.Numeric) { if (Device.SupportsInputIStyle) { // The default input mode is always numeric if the // type is password. if (!Control.Password) { writer.WriteAttribute("istyle", "4"); } } else if (Device.SupportsInputMode) { writer.WriteAttribute("mode", "numeric"); } } AddAccesskeyAttribute(writer); AddJPhoneMultiMediaAttributes(writer); } /// public override bool RequiresFormTag { get { return true; } } private String GetRandomID(int length) { Byte[] randomBytes = new Byte[length]; _random.NextBytes(randomBytes); char[] randomChars = new char[length]; for (int i = 0; i < length; i++) { randomChars[i] = (char)((((int)randomBytes[i]) % 26) + 'a'); } return new String(randomChars); } internal override String GetRenderName() { String renderName = base.GetRenderName(); if (Device.RequiresUniqueHtmlInputNames) { renderName += Constants.SelectionListSpecialCharacter + GetRandomID(4); } return renderName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
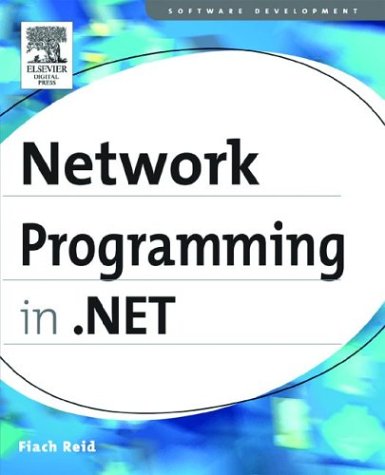
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserNavigatingEventHandler.cs
- TimeIntervalCollection.cs
- Setter.cs
- SqlNodeAnnotation.cs
- SimpleNameService.cs
- VisualBasicImportReference.cs
- OperationContractAttribute.cs
- ListItemParagraph.cs
- BinaryFormatterWriter.cs
- TwoPhaseCommit.cs
- GridViewUpdateEventArgs.cs
- TypedTableHandler.cs
- VirtualDirectoryMappingCollection.cs
- RenderTargetBitmap.cs
- GPRECTF.cs
- RepeaterCommandEventArgs.cs
- ZipFileInfo.cs
- DataObjectAttribute.cs
- ThrowHelper.cs
- StreamResourceInfo.cs
- RtfToken.cs
- EditorPartDesigner.cs
- SqlMultiplexer.cs
- MessageEventSubscriptionService.cs
- ClientProtocol.cs
- TemplatedMailWebEventProvider.cs
- BaseDataList.cs
- InteropBitmapSource.cs
- SiteMapNodeCollection.cs
- LinkDescriptor.cs
- SortQuery.cs
- ConstructorExpr.cs
- PageParser.cs
- ObjectStateManager.cs
- DrawingVisualDrawingContext.cs
- ClientScriptManagerWrapper.cs
- BatchParser.cs
- XmlToDatasetMap.cs
- BooleanSwitch.cs
- CommonDialog.cs
- ObservableDictionary.cs
- FormConverter.cs
- ConnectionOrientedTransportBindingElement.cs
- FieldAccessException.cs
- DesignTimeParseData.cs
- ObjectItemConventionAssemblyLoader.cs
- CqlLexer.cs
- Roles.cs
- TextFindEngine.cs
- MenuAutomationPeer.cs
- Size.cs
- XmlWrappingReader.cs
- FontCacheLogic.cs
- EDesignUtil.cs
- CompilerScopeManager.cs
- GB18030Encoding.cs
- ImageAttributes.cs
- MembershipAdapter.cs
- SynchronizationContext.cs
- MonitoringDescriptionAttribute.cs
- InkCanvas.cs
- ChildDocumentBlock.cs
- ContainerVisual.cs
- DatatypeImplementation.cs
- TrackingRecord.cs
- SerialStream.cs
- compensatingcollection.cs
- XmlSchemaComplexContentExtension.cs
- MenuItemCollection.cs
- IProducerConsumerCollection.cs
- NetSectionGroup.cs
- Missing.cs
- SrgsText.cs
- StorageEntityContainerMapping.cs
- StateBag.cs
- safelinkcollection.cs
- DataTableMappingCollection.cs
- TouchEventArgs.cs
- DesignerActionPropertyItem.cs
- XPathBinder.cs
- VectorCollectionConverter.cs
- CqlLexerHelpers.cs
- RoutedEventConverter.cs
- SetterBase.cs
- DictionaryEntry.cs
- GeometryConverter.cs
- XmlAtomicValue.cs
- RequestDescription.cs
- XPathSelectionIterator.cs
- TextControl.cs
- AmbientValueAttribute.cs
- FtpWebRequest.cs
- QueueProcessor.cs
- EditableTreeList.cs
- CharEnumerator.cs
- TrustLevel.cs
- BinaryMessageFormatter.cs
- DbConnectionPoolCounters.cs
- EntitySqlQueryState.cs
- WebConfigurationFileMap.cs