Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Tracking / TrackingRecord.cs / 1305376 / TrackingRecord.cs
using System; using System.Collections; using System.Collections.Generic; using System.Workflow.ComponentModel; using System.Runtime.Serialization; using System.Security.Permissions; using System.Workflow.Runtime.Hosting; namespace System.Workflow.Runtime.Tracking { public abstract class TrackingRecord { protected TrackingRecord() { } public abstract DateTime EventDateTime { get; set; } public abstract int EventOrder { get; set; } public abstract EventArgs EventArgs { get; set; } public abstract TrackingAnnotationCollection Annotations { get; } } ////// Contains data for a specific extraction point. /// public class ActivityTrackingRecord : TrackingRecord { #region Data Members private string _qualifiedID = null; private Type _activityType = null; private ActivityExecutionStatus _status; private List_body = new List (); private Guid _contextGuid = Guid.Empty, _parentContextGuid = Guid.Empty; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private EventArgs _args = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); #endregion #region Constructors public ActivityTrackingRecord() { } public ActivityTrackingRecord(Type activityType, string qualifiedName, Guid contextGuid, Guid parentContextGuid, ActivityExecutionStatus executionStatus, DateTime eventDateTime, int eventOrder, EventArgs eventArgs) { _activityType = activityType; _qualifiedID = qualifiedName; _status = executionStatus; _eventDateTime = eventDateTime; _contextGuid = contextGuid; _parentContextGuid = parentContextGuid; _eventOrder = eventOrder; _args = eventArgs; } #endregion #region Public Properties public string QualifiedName { get { return _qualifiedID; } set { _qualifiedID = value; } } public Guid ContextGuid { get { return _contextGuid; } set { _contextGuid = value; } } public Guid ParentContextGuid { get { return _parentContextGuid; } set { _parentContextGuid = value; } } public Type ActivityType { get { return _activityType; } set { _activityType = value; } } public ActivityExecutionStatus ExecutionStatus { get { return _status; } set { _status = value; } } public IList Body { get { return _body; } } #endregion #region TrackingRecord public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } /// /// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } #endregion } public class UserTrackingRecord : TrackingRecord { #region Data Members private string _qualifiedID = null; private Type _activityType = null; private List_body = new List (); private Guid _contextGuid = Guid.Empty, _parentContextGuid = Guid.Empty; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private object _userData = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); private EventArgs _args = null; private string _key = null; #endregion #region Constructors public UserTrackingRecord() { } public UserTrackingRecord(Type activityType, string qualifiedName, Guid contextGuid, Guid parentContextGuid, DateTime eventDateTime, int eventOrder, string userDataKey, object userData) { _activityType = activityType; _qualifiedID = qualifiedName; _eventDateTime = eventDateTime; _contextGuid = contextGuid; _parentContextGuid = parentContextGuid; _eventOrder = eventOrder; _userData = userData; _key = userDataKey; } #endregion #region Public Properties public string QualifiedName { get { return _qualifiedID; } set { _qualifiedID = value; } } public Guid ContextGuid { get { return _contextGuid; } set { _contextGuid = value; } } public Guid ParentContextGuid { get { return _parentContextGuid; } set { _parentContextGuid = value; } } public Type ActivityType { get { return _activityType; } set { _activityType = value; } } public IList Body { get { return _body; } } public string UserDataKey { get { return _key; } set { _key = value; } } public object UserData { get { return _userData; } set { _userData = value; } } #endregion #region TrackingRecord public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } /// /// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } #endregion } public class WorkflowTrackingRecord : TrackingRecord { #region Private Data Members private TrackingWorkflowEvent _event; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private EventArgs _args = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); #endregion #region Constructors public WorkflowTrackingRecord() { } public WorkflowTrackingRecord(TrackingWorkflowEvent trackingWorkflowEvent, DateTime eventDateTime, int eventOrder, EventArgs eventArgs) { _event = trackingWorkflowEvent; _eventDateTime = eventDateTime; _eventOrder = eventOrder; _args = eventArgs; } #endregion #region TrackingRecord public TrackingWorkflowEvent TrackingWorkflowEvent { get { return _event; } set { _event = value; } } public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } ////// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Workflow.ComponentModel; using System.Runtime.Serialization; using System.Security.Permissions; using System.Workflow.Runtime.Hosting; namespace System.Workflow.Runtime.Tracking { public abstract class TrackingRecord { protected TrackingRecord() { } public abstract DateTime EventDateTime { get; set; } public abstract int EventOrder { get; set; } public abstract EventArgs EventArgs { get; set; } public abstract TrackingAnnotationCollection Annotations { get; } } ////// Contains data for a specific extraction point. /// public class ActivityTrackingRecord : TrackingRecord { #region Data Members private string _qualifiedID = null; private Type _activityType = null; private ActivityExecutionStatus _status; private List_body = new List (); private Guid _contextGuid = Guid.Empty, _parentContextGuid = Guid.Empty; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private EventArgs _args = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); #endregion #region Constructors public ActivityTrackingRecord() { } public ActivityTrackingRecord(Type activityType, string qualifiedName, Guid contextGuid, Guid parentContextGuid, ActivityExecutionStatus executionStatus, DateTime eventDateTime, int eventOrder, EventArgs eventArgs) { _activityType = activityType; _qualifiedID = qualifiedName; _status = executionStatus; _eventDateTime = eventDateTime; _contextGuid = contextGuid; _parentContextGuid = parentContextGuid; _eventOrder = eventOrder; _args = eventArgs; } #endregion #region Public Properties public string QualifiedName { get { return _qualifiedID; } set { _qualifiedID = value; } } public Guid ContextGuid { get { return _contextGuid; } set { _contextGuid = value; } } public Guid ParentContextGuid { get { return _parentContextGuid; } set { _parentContextGuid = value; } } public Type ActivityType { get { return _activityType; } set { _activityType = value; } } public ActivityExecutionStatus ExecutionStatus { get { return _status; } set { _status = value; } } public IList Body { get { return _body; } } #endregion #region TrackingRecord public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } /// /// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } #endregion } public class UserTrackingRecord : TrackingRecord { #region Data Members private string _qualifiedID = null; private Type _activityType = null; private List_body = new List (); private Guid _contextGuid = Guid.Empty, _parentContextGuid = Guid.Empty; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private object _userData = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); private EventArgs _args = null; private string _key = null; #endregion #region Constructors public UserTrackingRecord() { } public UserTrackingRecord(Type activityType, string qualifiedName, Guid contextGuid, Guid parentContextGuid, DateTime eventDateTime, int eventOrder, string userDataKey, object userData) { _activityType = activityType; _qualifiedID = qualifiedName; _eventDateTime = eventDateTime; _contextGuid = contextGuid; _parentContextGuid = parentContextGuid; _eventOrder = eventOrder; _userData = userData; _key = userDataKey; } #endregion #region Public Properties public string QualifiedName { get { return _qualifiedID; } set { _qualifiedID = value; } } public Guid ContextGuid { get { return _contextGuid; } set { _contextGuid = value; } } public Guid ParentContextGuid { get { return _parentContextGuid; } set { _parentContextGuid = value; } } public Type ActivityType { get { return _activityType; } set { _activityType = value; } } public IList Body { get { return _body; } } public string UserDataKey { get { return _key; } set { _key = value; } } public object UserData { get { return _userData; } set { _userData = value; } } #endregion #region TrackingRecord public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } /// /// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } #endregion } public class WorkflowTrackingRecord : TrackingRecord { #region Private Data Members private TrackingWorkflowEvent _event; private DateTime _eventDateTime = DateTime.MinValue; private int _eventOrder = -1; private EventArgs _args = null; private TrackingAnnotationCollection _annotations = new TrackingAnnotationCollection(); #endregion #region Constructors public WorkflowTrackingRecord() { } public WorkflowTrackingRecord(TrackingWorkflowEvent trackingWorkflowEvent, DateTime eventDateTime, int eventOrder, EventArgs eventArgs) { _event = trackingWorkflowEvent; _eventDateTime = eventDateTime; _eventOrder = eventOrder; _args = eventArgs; } #endregion #region TrackingRecord public TrackingWorkflowEvent TrackingWorkflowEvent { get { return _event; } set { _event = value; } } public override DateTime EventDateTime { get { return _eventDateTime; } set { _eventDateTime = value; } } ////// Contains a value indicating the relative order of this event within the context of a workflow instance. /// Value will be unique within a workflow instance but is not guaranteed to be sequential. /// public override int EventOrder { get { return _eventOrder; } set { _eventOrder = value; } } public override EventArgs EventArgs { get { return _args; } set { _args = value; } } public override TrackingAnnotationCollection Annotations { get { return _annotations; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
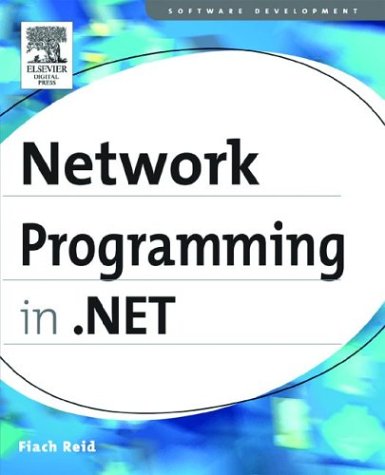
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RichTextBoxConstants.cs
- TagNameToTypeMapper.cs
- PcmConverter.cs
- DataGridTemplateColumn.cs
- EdmScalarPropertyAttribute.cs
- DataGridGeneralPage.cs
- TextTreeUndoUnit.cs
- CacheHelper.cs
- MessageDecoder.cs
- TypeSystem.cs
- SqlCommandBuilder.cs
- GridEntry.cs
- MetafileHeaderEmf.cs
- PeerFlooder.cs
- ArrayElementGridEntry.cs
- RadioButtonList.cs
- ConnectionPoint.cs
- HtmlImage.cs
- BuilderInfo.cs
- ProtocolsConfigurationEntry.cs
- MexBindingElement.cs
- Brush.cs
- ClientTargetSection.cs
- KeyValuePairs.cs
- DataContractSerializerElement.cs
- ProtocolViolationException.cs
- PassportAuthentication.cs
- SplashScreenNativeMethods.cs
- ProcessHostServerConfig.cs
- MembershipSection.cs
- AnnotationAdorner.cs
- ThreadStaticAttribute.cs
- ChannelTracker.cs
- DesignerActionService.cs
- DataSourceListEditor.cs
- _LazyAsyncResult.cs
- GuidelineSet.cs
- SortQuery.cs
- DataObject.cs
- Vector3DConverter.cs
- oledbconnectionstring.cs
- ControlBuilderAttribute.cs
- TableCellAutomationPeer.cs
- CustomWebEventKey.cs
- OuterGlowBitmapEffect.cs
- ColorBuilder.cs
- DesignerAutoFormatStyle.cs
- MsmqHostedTransportConfiguration.cs
- DataSysAttribute.cs
- BitmapMetadata.cs
- HttpPostClientProtocol.cs
- DataGridViewTextBoxColumn.cs
- ConsumerConnectionPointCollection.cs
- Durable.cs
- NameSpaceEvent.cs
- TransformerInfoCollection.cs
- RemoteCryptoDecryptRequest.cs
- DiffuseMaterial.cs
- TouchEventArgs.cs
- HostedHttpRequestAsyncResult.cs
- TemplateKey.cs
- ReadOnlyNameValueCollection.cs
- MaxMessageSizeStream.cs
- BitmapEffectGeneralTransform.cs
- CapabilitiesPattern.cs
- XDRSchema.cs
- AuthenticationModuleElement.cs
- Vector3DAnimationBase.cs
- EndpointConfigContainer.cs
- InputProcessorProfilesLoader.cs
- DataGridViewImageCell.cs
- ListViewInsertionMark.cs
- TransportContext.cs
- ComboBox.cs
- DragDeltaEventArgs.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- InfocardInteractiveChannelInitializer.cs
- IODescriptionAttribute.cs
- TypeBuilder.cs
- XmlSchemaException.cs
- GeometryHitTestParameters.cs
- ISessionStateStore.cs
- Instrumentation.cs
- RenderingEventArgs.cs
- IssuedSecurityTokenParameters.cs
- Monitor.cs
- TextEditorLists.cs
- ValidationEventArgs.cs
- SemanticBasicElement.cs
- ParserContext.cs
- DbConnectionPoolIdentity.cs
- RadioButton.cs
- _StreamFramer.cs
- TextFormatterImp.cs
- FastPropertyAccessor.cs
- DoubleAnimationBase.cs
- NamespaceList.cs
- SortedSet.cs
- GradientBrush.cs
- TextElementCollection.cs