Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / ListControls / DataGridGeneralPage.cs / 1 / DataGridGeneralPage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls.ListControls { using System; using System.Design; using System.Collections; using System.ComponentModel; using System.Data; using System.Diagnostics; using System.Drawing; using System.Web.UI; using System.Web.UI.Design.Util; using System.Web.UI.WebControls; using System.Windows.Forms; using WebControls = System.Web.UI.WebControls; using DataBinding = System.Web.UI.DataBinding; using DataGrid = System.Web.UI.WebControls.DataGrid; using CheckBox = System.Windows.Forms.CheckBox; using Control = System.Windows.Forms.Control; using Label = System.Windows.Forms.Label; using PropertyDescriptor = System.ComponentModel.PropertyDescriptor; using System.Globalization; ////// /// The General page for the DataGrid control. /// ///[System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] internal sealed class DataGridGeneralPage : BaseDataListPage { private CheckBox showHeaderCheck; private CheckBox showFooterCheck; private CheckBox allowSortingCheck; /// protected override string HelpKeyword { get { return "net.Asp.DataGridProperties.General"; } } /// /// /// Initializes the UI of the form. /// private void InitForm() { GroupLabel headerFooterGroup = new GroupLabel(); this.showHeaderCheck = new CheckBox(); this.showFooterCheck = new CheckBox(); GroupLabel behaviorGroup = new GroupLabel(); this.allowSortingCheck = new CheckBox(); headerFooterGroup.SetBounds(4, 4, 431, 16); headerFooterGroup.Text = SR.GetString(SR.DGGen_HeaderFooterGroup); headerFooterGroup.TabIndex = 8; headerFooterGroup.TabStop = false; showHeaderCheck.SetBounds(12, 24, 160, 16); showHeaderCheck.TabIndex = 9; showHeaderCheck.Text = SR.GetString(SR.DGGen_ShowHeader); showHeaderCheck.TextAlign = ContentAlignment.MiddleLeft; showHeaderCheck.FlatStyle = FlatStyle.System; showHeaderCheck.CheckedChanged += new EventHandler(this.OnCheckChangedShowHeader); showFooterCheck.SetBounds(12, 44, 160, 16); showFooterCheck.TabIndex = 10; showFooterCheck.Text = SR.GetString(SR.DGGen_ShowFooter); showFooterCheck.TextAlign = ContentAlignment.MiddleLeft; showFooterCheck.FlatStyle = FlatStyle.System; showFooterCheck.CheckedChanged += new EventHandler(this.OnCheckChangedShowFooter); behaviorGroup.SetBounds(4, 70, 431, 16); behaviorGroup.Text = SR.GetString(SR.DGGen_BehaviorGroup); behaviorGroup.TabIndex = 11; behaviorGroup.TabStop = false; allowSortingCheck.SetBounds(12, 88, 160, 16); allowSortingCheck.Text = SR.GetString(SR.DGGen_AllowSorting); allowSortingCheck.TabIndex = 12; allowSortingCheck.TextAlign = ContentAlignment.MiddleLeft; allowSortingCheck.FlatStyle = FlatStyle.System; allowSortingCheck.CheckedChanged += new EventHandler(this.OnCheckChangedAllowSorting); this.Text = SR.GetString(SR.DGGen_Text); this.AccessibleDescription = SR.GetString(SR.DGGen_Desc); this.Size = new Size(464, 272); this.CommitOnDeactivate = true; this.Icon = new Icon(this.GetType(), "DataGridGeneralPage.ico"); Controls.Clear(); Controls.AddRange(new Control[] { allowSortingCheck, behaviorGroup, showFooterCheck, showHeaderCheck, headerFooterGroup }); } ////// /// Initializes the page before it can be loaded with the component. /// private void InitPage() { showHeaderCheck.Checked = false; showFooterCheck.Checked = false; allowSortingCheck.Checked = false; } ////// /// Loads the component into the page. /// protected override void LoadComponent() { InitPage(); DataGrid dataGrid = (DataGrid)GetBaseControl(); showHeaderCheck.Checked = dataGrid.ShowHeader; showFooterCheck.Checked = dataGrid.ShowFooter; allowSortingCheck.Checked = dataGrid.AllowSorting; } ////// /// private void OnCheckChangedShowHeader(object source, EventArgs e) { if (IsLoading()) return; SetDirty(); } ////// /// private void OnCheckChangedShowFooter(object source, EventArgs e) { if (IsLoading()) return; SetDirty(); } ////// /// private void OnCheckChangedAllowSorting(object source, EventArgs e) { if (IsLoading()) return; SetDirty(); } ////// /// Saves the component loaded into the page. /// protected override void SaveComponent() { DataGrid dataGrid = (DataGrid)GetBaseControl(); dataGrid.ShowHeader = showHeaderCheck.Checked; dataGrid.ShowFooter = showFooterCheck.Checked; dataGrid.AllowSorting = allowSortingCheck.Checked; } ////// /// Sets the component that is to be edited in the page. /// public override void SetComponent(IComponent component) { base.SetComponent(component); InitForm(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
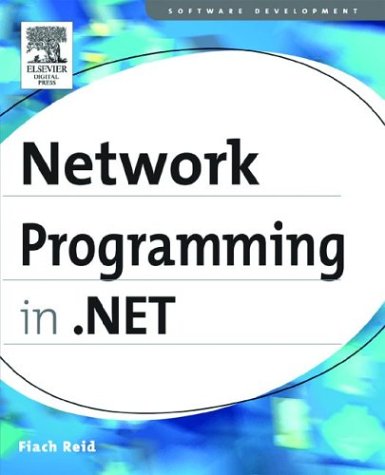
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LowerCaseStringConverter.cs
- ConsoleCancelEventArgs.cs
- ItemList.cs
- DependencyPropertyChangedEventArgs.cs
- Window.cs
- RulePatternOps.cs
- StructuredTypeEmitter.cs
- TypeDelegator.cs
- BaseCollection.cs
- ContractReference.cs
- DataGridViewRowsAddedEventArgs.cs
- CodeCommentStatement.cs
- CommonGetThemePartSize.cs
- EntityCollection.cs
- ArraySubsetEnumerator.cs
- NativeMethods.cs
- PlainXmlDeserializer.cs
- MediaElement.cs
- KnownIds.cs
- ExtensionsSection.cs
- HttpCookie.cs
- InputBindingCollection.cs
- LinearKeyFrames.cs
- BindingContext.cs
- FacetDescriptionElement.cs
- OrderedEnumerableRowCollection.cs
- CharacterMetricsDictionary.cs
- ApplicationTrust.cs
- FormCollection.cs
- WsdlExporter.cs
- EntitySet.cs
- ToolStripSystemRenderer.cs
- LostFocusEventManager.cs
- XslTransform.cs
- ExcludePathInfo.cs
- EventTrigger.cs
- KeyValueConfigurationCollection.cs
- BaseConfigurationRecord.cs
- ParseHttpDate.cs
- XmlSignificantWhitespace.cs
- LinqDataSourceInsertEventArgs.cs
- BufferBuilder.cs
- SqlDelegatedTransaction.cs
- ReadOnlyCollectionBase.cs
- WebPartDisplayModeCollection.cs
- XmlAttributes.cs
- EventToken.cs
- Int16Converter.cs
- SqlDataSourceCache.cs
- ExpressionPrinter.cs
- AspNetHostingPermission.cs
- ImageMap.cs
- Thread.cs
- DrawingAttributeSerializer.cs
- TreeNodeConverter.cs
- EventProviderWriter.cs
- TimeSpanSecondsConverter.cs
- DataBoundControlActionList.cs
- Crc32Helper.cs
- CodeAttachEventStatement.cs
- SqlMetaData.cs
- XmlMemberMapping.cs
- Label.cs
- Pen.cs
- FileNotFoundException.cs
- HierarchicalDataBoundControl.cs
- ReadWriteObjectLock.cs
- ThreadAbortException.cs
- XmlSchemaException.cs
- PathStreamGeometryContext.cs
- ColumnMap.cs
- ApplicationProxyInternal.cs
- SymbolType.cs
- DataObjectFieldAttribute.cs
- CellTreeNode.cs
- ConfigurationStrings.cs
- FileInfo.cs
- EmptyEnumerable.cs
- RegistrySecurity.cs
- Popup.cs
- WebServiceData.cs
- VBIdentifierNameEditor.cs
- AudioFormatConverter.cs
- ModelItemDictionaryImpl.cs
- CodeBinaryOperatorExpression.cs
- CallSiteBinder.cs
- OdbcConnectionString.cs
- IChannel.cs
- RecipientIdentity.cs
- GridEntryCollection.cs
- Vector3dCollection.cs
- ArgumentNullException.cs
- ConstrainedGroup.cs
- XmlBaseReader.cs
- TagMapInfo.cs
- KeyboardEventArgs.cs
- EditorPartChrome.cs
- Timer.cs
- CssTextWriter.cs
- SamlAuthorityBinding.cs